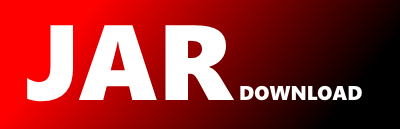
com.softlayer.api.service.user.customer.OpenIdConnect Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of softlayer-api-client Show documentation
Show all versions of softlayer-api-client Show documentation
API client for accessing the SoftLayer API
package com.softlayer.api.service.user.customer;
import com.softlayer.api.ApiClient;
import com.softlayer.api.ResponseHandler;
import com.softlayer.api.annotation.ApiMethod;
import com.softlayer.api.annotation.ApiType;
import com.softlayer.api.service.Account;
import com.softlayer.api.service.account.authentication.openidconnect.RegistrationInformation;
import com.softlayer.api.service.container.user.customer.openidconnect.LoginAccountInfo;
import com.softlayer.api.service.container.user.customer.portal.Token;
import com.softlayer.api.service.user.Customer;
import com.softlayer.api.service.user.customer.security.Answer;
import com.softlayer.api.service.user.security.Question;
import java.util.List;
import java.util.concurrent.Future;
/**
* @see SoftLayer_User_Customer_OpenIdConnect
*/
@ApiType("SoftLayer_User_Customer_OpenIdConnect")
public class OpenIdConnect extends Customer {
public Service asService(ApiClient client) {
return service(client, id);
}
public static Service service(ApiClient client) {
return client.createService(Service.class, null);
}
public static Service service(ApiClient client, Long id) {
return client.createService(Service.class, id == null ? null : id.toString());
}
/**
* @see SoftLayer_User_Customer_OpenIdConnect
*/
@com.softlayer.api.annotation.ApiService("SoftLayer_User_Customer_OpenIdConnect")
public static interface Service extends Customer.Service {
public ServiceAsync asAsync();
public Mask withNewMask();
public Mask withMask();
public void setMask(Mask mask);
/**
* @see SoftLayer_User_Customer_OpenIdConnect::completeInvitationAfterLogin
*/
@ApiMethod
public Void completeInvitationAfterLogin(String providerType, String accessToken, String emailRegistrationCode);
/**
* @see SoftLayer_User_Customer_OpenIdConnect::createOpenIdConnectUserAndCompleteInvitation
*/
@ApiMethod
public String createOpenIdConnectUserAndCompleteInvitation(String providerType, Customer user, String password, String registrationCode);
/**
* Declines an invitation to link an OpenIdConnect identity to a SoftLayer (Atlas) identity and account. Note that this uses a registration code that is likely a one-time-use-only token, so if an invitation has already been processed (accepted or previously declined) it will not be possible to process it a second time.
*
* @see SoftLayer_User_Customer_OpenIdConnect::declineInvitation
*/
@ApiMethod
public Void declineInvitation(String providerType, String registrationCode);
/**
* This API gets the default account for the OpenIdConnect identity that is linked to the current SoftLayer user identity. If there is no default present, the API returns null.
*
* @see SoftLayer_User_Customer_OpenIdConnect::getDefaultAccount
*/
@ApiMethod(instanceRequired = true)
public Account getDefaultAccount(String providerType);
/**
* Validates a supplied OpenIdConnect access token to the SoftLayer customer portal and returns the default account name and id for the active user. An exception will be thrown if no matching customer is found.
*
* @see SoftLayer_User_Customer_OpenIdConnect::getLoginAccountInfoOpenIdConnect
*/
@ApiMethod
public LoginAccountInfo getLoginAccountInfoOpenIdConnect(String providerType, String accessToken);
/**
* An OpenIdConnect identity, for example an IBMid, can be linked or mapped to one or more individual SoftLayer users, but no more than one SoftLayer user per account. This effectively links the OpenIdConnect identity to those accounts. This API returns a list of all the accounts for which there is a link between the OpenIdConnect identity and a SoftLayer user.
*
* @see SoftLayer_User_Customer_OpenIdConnect::getMappedAccounts
*/
@ApiMethod(instanceRequired = true)
public List getMappedAccounts(String providerType);
/**
* @see SoftLayer_User_Customer_OpenIdConnect::getObject
*/
@ApiMethod(value = "getObject", instanceRequired = true)
public OpenIdConnect getObjectForOpenIdConnect();
/**
* @see SoftLayer_User_Customer_OpenIdConnect::getOpenIdRegistrationInfoFromCode
*/
@ApiMethod
public RegistrationInformation getOpenIdRegistrationInfoFromCode(String providerType, String registrationCode);
/**
* Attempt to authenticate a supplied OpenIdConnect access token to the SoftLayer customer portal. If authentication is successful then the API returns a token containing the ID of the authenticated user and a hash key used by the SoftLayer customer portal to maintain authentication.
*
* @see SoftLayer_User_Customer_OpenIdConnect::getPortalLoginTokenOpenIdConnect
*/
@ApiMethod
public Token getPortalLoginTokenOpenIdConnect(String providerType, String accessToken, Long accountId, Long securityQuestionId, String securityQuestionAnswer);
/**
* This method is deprecated. Please see documentation for initiatePortalPasswordChange Retrieve a user object using a password recovery key received in an email generated by the [[SoftLayer_User_Customer::lostPassword|lostPassword]] method. The SoftLayer customer portal uses getUserFromLostPasswordRequest() to retrieve user security questions. Password recovery keys are valid for 24 hours after they're generated.
*
* @see SoftLayer_User_Customer_OpenIdConnect::getUserFromLostPasswordRequest
*/
@ApiMethod
public List getUserFromLostPasswordRequest(String key);
/**
* Determine if a string is the given user's login password to the SoftLayer customer portal.
*
* @see SoftLayer_User_Customer_OpenIdConnect::isValidPortalPassword
*/
@ApiMethod(instanceRequired = true)
public Boolean isValidPortalPassword(String password);
/**
* This method is deprecated. Please see documentation for initiatePortalPasswordChange SoftLayer provides a way for users of it's customer portal to recover lost passwords. The lostPassword() method is the first step in this process. Given a valid username and email address, the SoftLayer API will email the address provided with a URL to visit to begin the password recovery process. The last part of this URL is a hash key that's used as an identifier throughout this process. Use this hash key in the [[SoftLayer_User_Customer::setPasswordFromLostPasswordRequest|setPasswordFromLostPasswordRequest]] method to reset a user's password. Password recovery hash keys are valid for 24 hours after they're generated.
*
* @see SoftLayer_User_Customer_OpenIdConnect::lostPassword
*/
@ApiMethod
public Boolean lostPassword(String username, String email);
/**
* This method is deprecated. Please see documentation for initiatePortalPasswordChange Attempt to authenticate a username and password to the SoftLayer customer portal and reset there password. If authentication and password reset is successful then the API returns true.
*
* @see SoftLayer_User_Customer_OpenIdConnect::resetExpiredPassword
*/
@ApiMethod
public Boolean resetExpiredPassword(String username, String password, String newPassword, Long securityQuestionId, String securityQuestionAnswer);
/**
* An OpenIdConnect identity, for example an IBMid, can be linked or mapped to one or more individual SoftLayer users, but no more than one per account. If an OpenIdConnect identity is mapped to multiple accounts in this manner, one such account should be identified as the default account for that identity.
*
* @see SoftLayer_User_Customer_OpenIdConnect::setDefaultAccount
*/
@ApiMethod(instanceRequired = true)
public Account setDefaultAccount(String providerType, Long accountId);
/**
* Set a user's password via the lost password recovery system, using a password recovery key received in an email generated by the [[SoftLayer_User_Customer::lostPassword|lostPassword]] method. Password recovery keys are valid for 24 hours after they're generated.
*
* User portal passwords must match the following restrictions. Portal passwords must...
* * ...be over eight characters long.
* * ...be under twenty characters long.
* * ...contain at least one uppercase letter
* * ...contain at least one lowercase letter
* * ...contain at least one number
* * ...contain one of the special characters _ - | @ . , ? / ! ~ # $ % ^ & * ( ) { } [ ] \ + =
* * ...not match your username
* * ...not match your forum password
*
* @see SoftLayer_User_Customer_OpenIdConnect::setPasswordFromLostPasswordRequest
*/
@ApiMethod
public Boolean setPasswordFromLostPasswordRequest(String key, String password, List securityAnswers);
/**
* As master user, calling this api for the IBMid provider type when there is an existing IBMid for the email on the SL account will silently (without sending an invitation email) create a link for the IBMid. If the SoftLayer user is already linked to IBMid, this will reset the existing link. If the IBMid specified by the email of this user, is already used in a link to another user in this account, this call will fail. If there is already an open invitation from this SoftLayer user to this or any IBMid, this call will fail. If there is already an open invitation from some other SoftLayer user in this account to this IBMid, then this call will fail.
*
* @see SoftLayer_User_Customer_OpenIdConnect::silentlyMigrateUserOpenIdConnect
*/
@ApiMethod(instanceRequired = true)
public Boolean silentlyMigrateUserOpenIdConnect(String providerType);
/**
* This method is deprecated. Please see documentation for initiatePortalPasswordChange Update a user's password on the SoftLayer customer portal. As with forum passwords, user portal passwords must match the following restrictions. Portal passwords must...
* * ...be over eight characters long.
* * ...be under twenty characters long.
* * ...contain at least one uppercase letter
* * ...contain at least one lowercase letter
* * ...contain at least one number
* * ...contain one of the special characters _ - | @ . , ? / ! ~ # $ % ^ & * ( ) { } [ ] \ + =
* * ...not match your username
* * ...not match your forum password
* Finally, users can only update their own password. An account's master user can update any of their account users' passwords.
*
* @see SoftLayer_User_Customer_OpenIdConnect::updatePassword
*/
@ApiMethod(instanceRequired = true)
public Boolean updatePassword(String password);
}
public static interface ServiceAsync extends Customer.ServiceAsync {
public Mask withNewMask();
public Mask withMask();
public void setMask(Mask mask);
/**
* Async version of {@link Service#completeInvitationAfterLogin}
*/
public Future completeInvitationAfterLogin(String providerType, String accessToken, String emailRegistrationCode);
public Future> completeInvitationAfterLogin(String providerType, String accessToken, String emailRegistrationCode, ResponseHandler callback);
/**
* Async version of {@link Service#createOpenIdConnectUserAndCompleteInvitation}
*/
public Future createOpenIdConnectUserAndCompleteInvitation(String providerType, Customer user, String password, String registrationCode);
public Future> createOpenIdConnectUserAndCompleteInvitation(String providerType, Customer user, String password, String registrationCode, ResponseHandler callback);
/**
* Async version of {@link Service#declineInvitation}
*/
public Future declineInvitation(String providerType, String registrationCode);
public Future> declineInvitation(String providerType, String registrationCode, ResponseHandler callback);
/**
* Async version of {@link Service#getDefaultAccount}
*/
public Future getDefaultAccount(String providerType);
public Future> getDefaultAccount(String providerType, ResponseHandler callback);
/**
* Async version of {@link Service#getLoginAccountInfoOpenIdConnect}
*/
public Future getLoginAccountInfoOpenIdConnect(String providerType, String accessToken);
public Future> getLoginAccountInfoOpenIdConnect(String providerType, String accessToken, ResponseHandler callback);
/**
* Async version of {@link Service#getMappedAccounts}
*/
public Future> getMappedAccounts(String providerType);
public Future> getMappedAccounts(String providerType, ResponseHandler> callback);
/**
* Async version of {@link Service#getObjectForOpenIdConnect}
*/
public Future getObjectForOpenIdConnect();
public Future> getObjectForOpenIdConnect(ResponseHandler callback);
/**
* Async version of {@link Service#getOpenIdRegistrationInfoFromCode}
*/
public Future getOpenIdRegistrationInfoFromCode(String providerType, String registrationCode);
public Future> getOpenIdRegistrationInfoFromCode(String providerType, String registrationCode, ResponseHandler callback);
/**
* Async version of {@link Service#getPortalLoginTokenOpenIdConnect}
*/
public Future getPortalLoginTokenOpenIdConnect(String providerType, String accessToken, Long accountId, Long securityQuestionId, String securityQuestionAnswer);
public Future> getPortalLoginTokenOpenIdConnect(String providerType, String accessToken, Long accountId, Long securityQuestionId, String securityQuestionAnswer, ResponseHandler callback);
/**
* Async version of {@link Service#getUserFromLostPasswordRequest}
*/
public Future> getUserFromLostPasswordRequest(String key);
public Future> getUserFromLostPasswordRequest(String key, ResponseHandler> callback);
/**
* Async version of {@link Service#isValidPortalPassword}
*/
public Future isValidPortalPassword(String password);
public Future> isValidPortalPassword(String password, ResponseHandler callback);
/**
* Async version of {@link Service#lostPassword}
*/
public Future lostPassword(String username, String email);
public Future> lostPassword(String username, String email, ResponseHandler callback);
/**
* Async version of {@link Service#resetExpiredPassword}
*/
public Future resetExpiredPassword(String username, String password, String newPassword, Long securityQuestionId, String securityQuestionAnswer);
public Future> resetExpiredPassword(String username, String password, String newPassword, Long securityQuestionId, String securityQuestionAnswer, ResponseHandler callback);
/**
* Async version of {@link Service#setDefaultAccount}
*/
public Future setDefaultAccount(String providerType, Long accountId);
public Future> setDefaultAccount(String providerType, Long accountId, ResponseHandler callback);
/**
* Async version of {@link Service#setPasswordFromLostPasswordRequest}
*/
public Future setPasswordFromLostPasswordRequest(String key, String password, List securityAnswers);
public Future> setPasswordFromLostPasswordRequest(String key, String password, List securityAnswers, ResponseHandler callback);
/**
* Async version of {@link Service#silentlyMigrateUserOpenIdConnect}
*/
public Future silentlyMigrateUserOpenIdConnect(String providerType);
public Future> silentlyMigrateUserOpenIdConnect(String providerType, ResponseHandler callback);
/**
* Async version of {@link Service#updatePassword}
*/
public Future updatePassword(String password);
public Future> updatePassword(String password, ResponseHandler callback);
}
public static class Mask extends com.softlayer.api.service.user.Customer.Mask {
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy