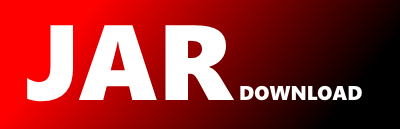
com.softlayer.api.service.billing.order.Quote Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of softlayer-api-client Show documentation
Show all versions of softlayer-api-client Show documentation
API client for accessing the SoftLayer API
package com.softlayer.api.service.billing.order;
import com.softlayer.api.ApiClient;
import com.softlayer.api.ResponseHandler;
import com.softlayer.api.annotation.ApiMethod;
import com.softlayer.api.annotation.ApiProperty;
import com.softlayer.api.annotation.ApiType;
import com.softlayer.api.service.Account;
import com.softlayer.api.service.Entity;
import com.softlayer.api.service.container.product.Order;
import com.softlayer.api.service.container.product.order.Receipt;
import java.util.ArrayList;
import java.util.GregorianCalendar;
import java.util.List;
import java.util.concurrent.Future;
/**
* The SoftLayer_Billing_Oder_Quote data type contains general information relating to an individual order applied to a SoftLayer customer account or to a new customer. Personal information in this type such as names, addresses, and phone numbers are taken from the account's contact information at the time the quote is generated for existing SoftLayer customer.
*
* @see SoftLayer_Billing_Order_Quote
*/
@ApiType("SoftLayer_Billing_Order_Quote")
public class Quote extends Entity {
/**
* A quote's corresponding account.
*/
@ApiProperty
protected Account account;
public Account getAccount() {
return account;
}
public void setAccount(Account account) {
this.account = account;
}
/**
* This order contains the records for which products were selected for this quote.
*/
@ApiProperty
protected com.softlayer.api.service.billing.Order order;
public com.softlayer.api.service.billing.Order getOrder() {
return order;
}
public void setOrder(com.softlayer.api.service.billing.Order order) {
this.order = order;
}
/**
* These are all the orders that were created from this quote.
*/
@ApiProperty
protected List ordersFromQuote;
public List getOrdersFromQuote() {
if (ordersFromQuote == null) {
ordersFromQuote = new ArrayList();
}
return ordersFromQuote;
}
/**
* Identification Number of the account record tied to the quote
*/
@ApiProperty(canBeNullOrNotSet = true)
protected Long accountId;
public Long getAccountId() {
return accountId;
}
public void setAccountId(Long accountId) {
accountIdSpecified = true;
this.accountId = accountId;
}
protected boolean accountIdSpecified;
public boolean isAccountIdSpecified() {
return accountIdSpecified;
}
public void unsetAccountId() {
accountId = null;
accountIdSpecified = false;
}
/**
* Identification Number of the order record tied to the quote.
*/
@ApiProperty(canBeNullOrNotSet = true)
protected Long completedPurchaseDataId;
public Long getCompletedPurchaseDataId() {
return completedPurchaseDataId;
}
public void setCompletedPurchaseDataId(Long completedPurchaseDataId) {
completedPurchaseDataIdSpecified = true;
this.completedPurchaseDataId = completedPurchaseDataId;
}
protected boolean completedPurchaseDataIdSpecified;
public boolean isCompletedPurchaseDataIdSpecified() {
return completedPurchaseDataIdSpecified;
}
public void unsetCompletedPurchaseDataId() {
completedPurchaseDataId = null;
completedPurchaseDataIdSpecified = false;
}
/**
* Holds the date the quote record was created
*/
@ApiProperty(canBeNullOrNotSet = true)
protected GregorianCalendar createDate;
public GregorianCalendar getCreateDate() {
return createDate;
}
public void setCreateDate(GregorianCalendar createDate) {
createDateSpecified = true;
this.createDate = createDate;
}
protected boolean createDateSpecified;
public boolean isCreateDateSpecified() {
return createDateSpecified;
}
public void unsetCreateDate() {
createDate = null;
createDateSpecified = false;
}
/**
* This property holds the date of expiration of a quote, after that date the quote would be deem expired
*/
@ApiProperty(canBeNullOrNotSet = true)
protected GregorianCalendar expirationDate;
public GregorianCalendar getExpirationDate() {
return expirationDate;
}
public void setExpirationDate(GregorianCalendar expirationDate) {
expirationDateSpecified = true;
this.expirationDate = expirationDate;
}
protected boolean expirationDateSpecified;
public boolean isExpirationDateSpecified() {
return expirationDateSpecified;
}
public void unsetExpirationDate() {
expirationDate = null;
expirationDateSpecified = false;
}
/**
* The id use to identify a quote.
*/
@ApiProperty(canBeNullOrNotSet = true)
protected Long id;
public Long getId() {
return id;
}
public void setId(Long id) {
idSpecified = true;
this.id = id;
}
protected boolean idSpecified;
public boolean isIdSpecified() {
return idSpecified;
}
public void unsetId() {
id = null;
idSpecified = false;
}
/**
* Holds the date when the quote record was modified with reference to its creation date
*/
@ApiProperty(canBeNullOrNotSet = true)
protected GregorianCalendar modifyDate;
public GregorianCalendar getModifyDate() {
return modifyDate;
}
public void setModifyDate(GregorianCalendar modifyDate) {
modifyDateSpecified = true;
this.modifyDate = modifyDate;
}
protected boolean modifyDateSpecified;
public boolean isModifyDateSpecified() {
return modifyDateSpecified;
}
public void unsetModifyDate() {
modifyDate = null;
modifyDateSpecified = false;
}
/**
* The name given to quote by the initiator
*/
@ApiProperty(canBeNullOrNotSet = true)
protected String name;
public String getName() {
return name;
}
public void setName(String name) {
nameSpecified = true;
this.name = name;
}
protected boolean nameSpecified;
public boolean isNameSpecified() {
return nameSpecified;
}
public void unsetName() {
name = null;
nameSpecified = false;
}
/**
* This property Holds system generated notes. In our case if a quote is tied to an order where one of the order item has an inactive promotion code, the quote will be considered invalid.
*/
@ApiProperty(canBeNullOrNotSet = true)
protected String publicNote;
public String getPublicNote() {
return publicNote;
}
public void setPublicNote(String publicNote) {
publicNoteSpecified = true;
this.publicNote = publicNote;
}
protected boolean publicNoteSpecified;
public boolean isPublicNoteSpecified() {
return publicNoteSpecified;
}
public void unsetPublicNote() {
publicNote = null;
publicNoteSpecified = false;
}
/**
* Holds system generated hash password for the Quote
*/
@ApiProperty(canBeNullOrNotSet = true)
protected String quoteKey;
public String getQuoteKey() {
return quoteKey;
}
public void setQuoteKey(String quoteKey) {
quoteKeySpecified = true;
this.quoteKey = quoteKey;
}
protected boolean quoteKeySpecified;
public boolean isQuoteKeySpecified() {
return quoteKeySpecified;
}
public void unsetQuoteKey() {
quoteKey = null;
quoteKeySpecified = false;
}
/**
* This property Holds the current status of a Quote: pending,expired, saved or deleted
*/
@ApiProperty(canBeNullOrNotSet = true)
protected String status;
public String getStatus() {
return status;
}
public void setStatus(String status) {
statusSpecified = true;
this.status = status;
}
protected boolean statusSpecified;
public boolean isStatusSpecified() {
return statusSpecified;
}
public void unsetStatus() {
status = null;
statusSpecified = false;
}
/**
* A count of these are all the orders that were created from this quote.
*/
@ApiProperty
protected Long ordersFromQuoteCount;
public Long getOrdersFromQuoteCount() {
return ordersFromQuoteCount;
}
public void setOrdersFromQuoteCount(Long ordersFromQuoteCount) {
this.ordersFromQuoteCount = ordersFromQuoteCount;
}
public Service asService(ApiClient client) {
return service(client, id);
}
public static Service service(ApiClient client) {
return client.createService(Service.class, null);
}
public static Service service(ApiClient client, Long id) {
return client.createService(Service.class, id == null ? null : id.toString());
}
/**
* The SoftLayer_Billing_Order_Quote service controls the quoted orders that are created whenever a SoftLayer customer's places a purchase. Quotes exist in several states. The ones of concern are:
* *'''PENDING''': Quotes which have not been paid yet. Quotes pending approval from a Softlayer customer.
*
*
* Once an order is placed from a quote it moves from PENDING to EXPIRED state 2 days after its creation and it is removed from the system after 5 days unless otherwise the SoftLayer customer saved the quote.
*
* Quotes could are created with contact information duplicated from the [[SoftLayer_Account (type)|SoftLayer_Account data type]] or by manual entry. We do this in order to maintain a history of an account's contact information as quotes are generated.
*
* Query the [[SoftLayer_Account]] service to get a list of quotes for your account.
*
* @see SoftLayer_Billing_Order_Quote
*/
@com.softlayer.api.annotation.ApiService("SoftLayer_Billing_Order_Quote")
public static interface Service extends com.softlayer.api.Service {
public ServiceAsync asAsync();
public Mask withNewMask();
public Mask withMask();
public void setMask(Mask mask);
/**
* This method is used to transfer an anonymous quote to the active user and associated account. An anonymous quote is one that was created by a user without being authenticated. If a quote was created anonymously and then the customer attempts to access that anonymous quote via the API (which requires authentication), the customer will be unable to retrieve the quote due to the security restrictions in place. By providing the ability for a customer to claim a quote, s/he will be able to pull the anonymous quote onto his/her account and successfully view the quote.
*
* To claim a quote, both the quote id and the quote key (the 32-character random string) must be provided.
*
* @see SoftLayer_Billing_Order_Quote::claim
*/
@ApiMethod
public Quote claim(String quoteKey, Long quoteId);
/**
* Account master users and sub-users in the SoftLayer customer portal can delete the quote of an order.
*
* @see SoftLayer_Billing_Order_Quote::deleteQuote
*/
@ApiMethod(instanceRequired = true)
public Quote deleteQuote();
/**
* getObject retrieves the SoftLayer_Billing_Order_Quote object whose ID number corresponds to the ID number of the init parameter passed to the SoftLayer_Billing_Order_Quote service. You can only retrieve quotes that are assigned to your portal user's account.
*
* @see SoftLayer_Billing_Order_Quote::getObject
*/
@ApiMethod(instanceRequired = true)
public Quote getObject();
/**
* Retrieve a PDF record of a SoftLayer quoted order. SoftLayer keeps PDF records of all quoted orders for customer retrieval from the portal and API. You must have a PDF reader installed in order to view these quoted order files.
*
* @see SoftLayer_Billing_Order_Quote::getPdf
*/
@ApiMethod(instanceRequired = true)
public byte[] getPdf();
/**
* This method will return a [[SoftLayer_Billing_Order_Quote]] that is identified by the quote key specified. If you do not have access to the quote or it does not exist, an exception will be thrown indicating so.
*
* @see SoftLayer_Billing_Order_Quote::getQuoteByQuoteKey
*/
@ApiMethod
public Quote getQuoteByQuoteKey(String quoteKey);
/**
* Generate an [[SoftLayer_Container_Product_Order|order container]] from the previously-created quote. This will take into account promotions, reseller status, estimated taxes and all other standard order verification processes.
*
* @see SoftLayer_Billing_Order_Quote::getRecalculatedOrderContainer
*/
@ApiMethod(instanceRequired = true)
public Order getRecalculatedOrderContainer(Order userOrderData, Boolean orderBeingPlacedFlag);
/**
* Use this method for placing server orders and additional services orders. The same applies for this as with verifyOrder. Send in the SoftLayer_Container_Product_Order_Hardware_Server for server orders. In addition to verifying the order, placeOrder() also makes an initial authorization on the SoftLayer_Account tied to this order, if a credit card is on file. If the account tied to this order is a paypal customer, an URL will also be returned to the customer. After placing the order, you must go to this URL to finish the authorization process. This tells paypal that you indeed want to place the order. After going to this URL, it will direct you back to a SoftLayer webpage that tells us you have finished the process. After this, it will go to sales for final approval.
*
* @see SoftLayer_Billing_Order_Quote::placeOrder
*/
@ApiMethod(instanceRequired = true)
public Receipt placeOrder(Order orderData);
/**
* Use this method for placing server quotes and additional services quotes. The same applies for this as with verifyOrder. Send in the SoftLayer_Container_Product_Order_Hardware_Server for server quotes. In addition to verifying the quote, placeQuote() also makes an initial authorization on the SoftLayer_Account tied to this order, if a credit card is on file. If the account tied to this order is a paypal customer, an URL will also be returned to the customer. After placing the order, you must go to this URL to finish the authorization process. This tells paypal that you indeed want to place the order. After going to this URL, it will direct you back to a SoftLayer webpage that tells us you have finished the process.
*
* @see SoftLayer_Billing_Order_Quote::placeQuote
*/
@ApiMethod(instanceRequired = true)
public Order placeQuote(Order orderData);
/**
* Account master users and sub-users in the SoftLayer customer portal can save the quote of an order to avoid its deletion after 5 days or its expiration after 2 days.
*
* @see SoftLayer_Billing_Order_Quote::saveQuote
*/
@ApiMethod(instanceRequired = true)
public Quote saveQuote();
/**
* Use this method for placing server orders and additional services orders. The same applies for this as with verifyOrder. Send in the SoftLayer_Container_Product_Order_Hardware_Server for server orders. In addition to verifying the order, placeOrder() also makes an initial authorization on the SoftLayer_Account tied to this order, if a credit card is on file. If the account tied to this order is a paypal customer, an URL will also be returned to the customer. After placing the order, you must go to this URL to finish the authorization process. This tells paypal that you indeed want to place the order. After going to this URL, it will direct you back to a SoftLayer webpage that tells us you have finished the process. After this, it will go to sales for final approval.
*
* @see SoftLayer_Billing_Order_Quote::verifyOrder
*/
@ApiMethod(instanceRequired = true)
public Order verifyOrder(Order orderData);
/**
* A quote's corresponding account.
*
* @see SoftLayer_Billing_Order_Quote::getAccount
*/
@ApiMethod(instanceRequired = true)
public Account getAccount();
/**
* This order contains the records for which products were selected for this quote.
*
* @see SoftLayer_Billing_Order_Quote::getOrder
*/
@ApiMethod(instanceRequired = true)
public com.softlayer.api.service.billing.Order getOrder();
/**
* These are all the orders that were created from this quote.
*
* @see SoftLayer_Billing_Order_Quote::getOrdersFromQuote
*/
@ApiMethod(instanceRequired = true)
public List getOrdersFromQuote();
}
public static interface ServiceAsync extends com.softlayer.api.ServiceAsync {
public Mask withNewMask();
public Mask withMask();
public void setMask(Mask mask);
/**
* Async version of {@link Service#claim}
*/
public Future claim(String quoteKey, Long quoteId);
public Future> claim(String quoteKey, Long quoteId, ResponseHandler callback);
/**
* Async version of {@link Service#deleteQuote}
*/
public Future deleteQuote();
public Future> deleteQuote(ResponseHandler callback);
/**
* Async version of {@link Service#getObject}
*/
public Future getObject();
public Future> getObject(ResponseHandler callback);
/**
* Async version of {@link Service#getPdf}
*/
public Future getPdf();
public Future> getPdf(ResponseHandler callback);
/**
* Async version of {@link Service#getQuoteByQuoteKey}
*/
public Future getQuoteByQuoteKey(String quoteKey);
public Future> getQuoteByQuoteKey(String quoteKey, ResponseHandler callback);
/**
* Async version of {@link Service#getRecalculatedOrderContainer}
*/
public Future getRecalculatedOrderContainer(Order userOrderData, Boolean orderBeingPlacedFlag);
public Future> getRecalculatedOrderContainer(Order userOrderData, Boolean orderBeingPlacedFlag, ResponseHandler callback);
/**
* Async version of {@link Service#placeOrder}
*/
public Future placeOrder(Order orderData);
public Future> placeOrder(Order orderData, ResponseHandler callback);
/**
* Async version of {@link Service#placeQuote}
*/
public Future placeQuote(Order orderData);
public Future> placeQuote(Order orderData, ResponseHandler callback);
/**
* Async version of {@link Service#saveQuote}
*/
public Future saveQuote();
public Future> saveQuote(ResponseHandler callback);
/**
* Async version of {@link Service#verifyOrder}
*/
public Future verifyOrder(Order orderData);
public Future> verifyOrder(Order orderData, ResponseHandler callback);
/**
* Async version of {@link Service#getAccount}
*/
public Future getAccount();
/**
* Async callback version of {@link Service#getAccount}
*/
public Future> getAccount(ResponseHandler callback);
/**
* Async version of {@link Service#getOrder}
*/
public Future getOrder();
/**
* Async callback version of {@link Service#getOrder}
*/
public Future> getOrder(ResponseHandler callback);
/**
* Async version of {@link Service#getOrdersFromQuote}
*/
public Future> getOrdersFromQuote();
/**
* Async callback version of {@link Service#getOrdersFromQuote}
*/
public Future> getOrdersFromQuote(ResponseHandler> callback);
}
public static class Mask extends com.softlayer.api.service.Entity.Mask {
public com.softlayer.api.service.Account.Mask account() {
return withSubMask("account", com.softlayer.api.service.Account.Mask.class);
}
public com.softlayer.api.service.billing.Order.Mask order() {
return withSubMask("order", com.softlayer.api.service.billing.Order.Mask.class);
}
public com.softlayer.api.service.billing.Order.Mask ordersFromQuote() {
return withSubMask("ordersFromQuote", com.softlayer.api.service.billing.Order.Mask.class);
}
public Mask accountId() {
withLocalProperty("accountId");
return this;
}
public Mask completedPurchaseDataId() {
withLocalProperty("completedPurchaseDataId");
return this;
}
public Mask createDate() {
withLocalProperty("createDate");
return this;
}
public Mask expirationDate() {
withLocalProperty("expirationDate");
return this;
}
public Mask id() {
withLocalProperty("id");
return this;
}
public Mask modifyDate() {
withLocalProperty("modifyDate");
return this;
}
public Mask name() {
withLocalProperty("name");
return this;
}
public Mask publicNote() {
withLocalProperty("publicNote");
return this;
}
public Mask quoteKey() {
withLocalProperty("quoteKey");
return this;
}
public Mask status() {
withLocalProperty("status");
return this;
}
public Mask ordersFromQuoteCount() {
withLocalProperty("ordersFromQuoteCount");
return this;
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy