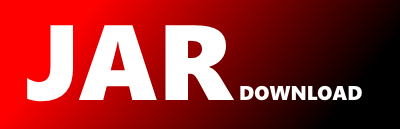
com.softlayer.api.service.container.network.intrusionprotection.Event Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of softlayer-api-client Show documentation
Show all versions of softlayer-api-client Show documentation
API client for accessing the SoftLayer API
package com.softlayer.api.service.container.network.intrusionprotection;
import com.softlayer.api.annotation.ApiProperty;
import com.softlayer.api.annotation.ApiType;
import com.softlayer.api.service.Entity;
/**
* The IntrusionProtection_Event object stores information about individual intrusion protection events.
*
* It is a data container that cannot be edited, deleted, or saved.
*
* It is returned by many methods in the TippingPointReporting object, but never directly, always as a child of another container object.
*
* @see SoftLayer_Container_Network_IntrusionProtection_Event
*/
@ApiType("SoftLayer_Container_Network_IntrusionProtection_Event")
public class Event extends Entity {
/**
* The CVE ID(s), if any, associated with this attack signature.
*/
@ApiProperty(canBeNullOrNotSet = true)
protected String CVEId;
public String getCVEId() {
return CVEId;
}
public void setCVEId(String CVEId) {
CVEIdSpecified = true;
this.CVEId = CVEId;
}
protected boolean CVEIdSpecified;
public boolean isCVEIdSpecified() {
return CVEIdSpecified;
}
public void unsetCVEId() {
CVEId = null;
CVEIdSpecified = false;
}
/**
* The action that was taken when this attack was discovered. Can be either "Block" or "Permit"
*/
@ApiProperty(canBeNullOrNotSet = true)
protected String actionTaken;
public String getActionTaken() {
return actionTaken;
}
public void setActionTaken(String actionTaken) {
actionTakenSpecified = true;
this.actionTaken = actionTaken;
}
protected boolean actionTakenSpecified;
public boolean isActionTakenSpecified() {
return actionTakenSpecified;
}
public void unsetActionTaken() {
actionTaken = null;
actionTakenSpecified = false;
}
/**
* The number of attacks in this block. Attacks are grouped differently based on the query performed on the tippingPointReporting object.
*/
@ApiProperty(canBeNullOrNotSet = true)
protected Long attackCount;
public Long getAttackCount() {
return attackCount;
}
public void setAttackCount(Long attackCount) {
attackCountSpecified = true;
this.attackCount = attackCount;
}
protected boolean attackCountSpecified;
public boolean isAttackCountSpecified() {
return attackCountSpecified;
}
public void unsetAttackCount() {
attackCount = null;
attackCountSpecified = false;
}
/**
* Long description of the attack. May contain links to more information
*/
@ApiProperty(canBeNullOrNotSet = true)
protected String attackLongDescription;
public String getAttackLongDescription() {
return attackLongDescription;
}
public void setAttackLongDescription(String attackLongDescription) {
attackLongDescriptionSpecified = true;
this.attackLongDescription = attackLongDescription;
}
protected boolean attackLongDescriptionSpecified;
public boolean isAttackLongDescriptionSpecified() {
return attackLongDescriptionSpecified;
}
public void unsetAttackLongDescription() {
attackLongDescription = null;
attackLongDescriptionSpecified = false;
}
/**
* Name of the attack
*/
@ApiProperty(canBeNullOrNotSet = true)
protected String attackName;
public String getAttackName() {
return attackName;
}
public void setAttackName(String attackName) {
attackNameSpecified = true;
this.attackName = attackName;
}
protected boolean attackNameSpecified;
public boolean isAttackNameSpecified() {
return attackNameSpecified;
}
public void unsetAttackName() {
attackName = null;
attackNameSpecified = false;
}
/**
* The starting timestamp of the attack recorded, in Y-m-d H:i:s format. May not be set, depending on the type of query performed.
*/
@ApiProperty(canBeNullOrNotSet = true)
protected String beginTime;
public String getBeginTime() {
return beginTime;
}
public void setBeginTime(String beginTime) {
beginTimeSpecified = true;
this.beginTime = beginTime;
}
protected boolean beginTimeSpecified;
public boolean isBeginTimeSpecified() {
return beginTimeSpecified;
}
public void unsetBeginTime() {
beginTime = null;
beginTimeSpecified = false;
}
/**
* The BugTraq ID(s), if any, associated with this attack signature.
*/
@ApiProperty(canBeNullOrNotSet = true)
protected String bugtraqId;
public String getBugtraqId() {
return bugtraqId;
}
public void setBugtraqId(String bugtraqId) {
bugtraqIdSpecified = true;
this.bugtraqId = bugtraqId;
}
protected boolean bugtraqIdSpecified;
public boolean isBugtraqIdSpecified() {
return bugtraqIdSpecified;
}
public void unsetBugtraqId() {
bugtraqId = null;
bugtraqIdSpecified = false;
}
/**
* The human-readable classification of the attack
*/
@ApiProperty(canBeNullOrNotSet = true)
protected String classification;
public String getClassification() {
return classification;
}
public void setClassification(String classification) {
classificationSpecified = true;
this.classification = classification;
}
protected boolean classificationSpecified;
public boolean isClassificationSpecified() {
return classificationSpecified;
}
public void unsetClassification() {
classification = null;
classificationSpecified = false;
}
/**
* The IP Address (as a dotted decimal string) of the machine that was the target of the attack
*/
@ApiProperty(canBeNullOrNotSet = true)
protected String destinationIpAddress;
public String getDestinationIpAddress() {
return destinationIpAddress;
}
public void setDestinationIpAddress(String destinationIpAddress) {
destinationIpAddressSpecified = true;
this.destinationIpAddress = destinationIpAddress;
}
protected boolean destinationIpAddressSpecified;
public boolean isDestinationIpAddressSpecified() {
return destinationIpAddressSpecified;
}
public void unsetDestinationIpAddress() {
destinationIpAddress = null;
destinationIpAddressSpecified = false;
}
/**
* The port the attack was directed at
*/
@ApiProperty(canBeNullOrNotSet = true)
protected Long destinationPort;
public Long getDestinationPort() {
return destinationPort;
}
public void setDestinationPort(Long destinationPort) {
destinationPortSpecified = true;
this.destinationPort = destinationPort;
}
protected boolean destinationPortSpecified;
public boolean isDestinationPortSpecified() {
return destinationPortSpecified;
}
public void unsetDestinationPort() {
destinationPort = null;
destinationPortSpecified = false;
}
/**
* The ending timestamp of the attack recorded, in Y-m-d H:i:s format. May not be set, depending on the type of query performed.
*/
@ApiProperty(canBeNullOrNotSet = true)
protected String endTime;
public String getEndTime() {
return endTime;
}
public void setEndTime(String endTime) {
endTimeSpecified = true;
this.endTime = endTime;
}
protected boolean endTimeSpecified;
public boolean isEndTimeSpecified() {
return endTimeSpecified;
}
public void unsetEndTime() {
endTime = null;
endTimeSpecified = false;
}
/**
* The platform affected by the attack
*/
@ApiProperty(canBeNullOrNotSet = true)
protected String platform;
public String getPlatform() {
return platform;
}
public void setPlatform(String platform) {
platformSpecified = true;
this.platform = platform;
}
protected boolean platformSpecified;
public boolean isPlatformSpecified() {
return platformSpecified;
}
public void unsetPlatform() {
platform = null;
platformSpecified = false;
}
/**
* The protocol used in the attack
*/
@ApiProperty(canBeNullOrNotSet = true)
protected String protocol;
public String getProtocol() {
return protocol;
}
public void setProtocol(String protocol) {
protocolSpecified = true;
this.protocol = protocol;
}
protected boolean protocolSpecified;
public boolean isProtocolSpecified() {
return protocolSpecified;
}
public void unsetProtocol() {
protocol = null;
protocolSpecified = false;
}
/**
* The human-readable severity of this attack, from "Low" to "Critical"
*/
@ApiProperty(canBeNullOrNotSet = true)
protected String severity;
public String getSeverity() {
return severity;
}
public void setSeverity(String severity) {
severitySpecified = true;
this.severity = severity;
}
protected boolean severitySpecified;
public boolean isSeveritySpecified() {
return severitySpecified;
}
public void unsetSeverity() {
severity = null;
severitySpecified = false;
}
/**
* Unique ID of the "Signature" in question. The signature determines the type of attack recorded. SignatureId is used in the drillDown() function on the TippingPointReporting service
*/
@ApiProperty(canBeNullOrNotSet = true)
protected String signatureId;
public String getSignatureId() {
return signatureId;
}
public void setSignatureId(String signatureId) {
signatureIdSpecified = true;
this.signatureId = signatureId;
}
protected boolean signatureIdSpecified;
public boolean isSignatureIdSpecified() {
return signatureIdSpecified;
}
public void unsetSignatureId() {
signatureId = null;
signatureIdSpecified = false;
}
/**
* The IP Address (as a dotted decimal string) of the machine originating the attack
*/
@ApiProperty(canBeNullOrNotSet = true)
protected String sourceIpAddress;
public String getSourceIpAddress() {
return sourceIpAddress;
}
public void setSourceIpAddress(String sourceIpAddress) {
sourceIpAddressSpecified = true;
this.sourceIpAddress = sourceIpAddress;
}
protected boolean sourceIpAddressSpecified;
public boolean isSourceIpAddressSpecified() {
return sourceIpAddressSpecified;
}
public void unsetSourceIpAddress() {
sourceIpAddress = null;
sourceIpAddressSpecified = false;
}
/**
* The port the attack originated from
*/
@ApiProperty(canBeNullOrNotSet = true)
protected Long sourcePort;
public Long getSourcePort() {
return sourcePort;
}
public void setSourcePort(Long sourcePort) {
sourcePortSpecified = true;
this.sourcePort = sourcePort;
}
protected boolean sourcePortSpecified;
public boolean isSourcePortSpecified() {
return sourcePortSpecified;
}
public void unsetSourcePort() {
sourcePort = null;
sourcePortSpecified = false;
}
public static class Mask extends com.softlayer.api.service.Entity.Mask {
public Mask CVEId() {
withLocalProperty("CVEId");
return this;
}
public Mask actionTaken() {
withLocalProperty("actionTaken");
return this;
}
public Mask attackCount() {
withLocalProperty("attackCount");
return this;
}
public Mask attackLongDescription() {
withLocalProperty("attackLongDescription");
return this;
}
public Mask attackName() {
withLocalProperty("attackName");
return this;
}
public Mask beginTime() {
withLocalProperty("beginTime");
return this;
}
public Mask bugtraqId() {
withLocalProperty("bugtraqId");
return this;
}
public Mask classification() {
withLocalProperty("classification");
return this;
}
public Mask destinationIpAddress() {
withLocalProperty("destinationIpAddress");
return this;
}
public Mask destinationPort() {
withLocalProperty("destinationPort");
return this;
}
public Mask endTime() {
withLocalProperty("endTime");
return this;
}
public Mask platform() {
withLocalProperty("platform");
return this;
}
public Mask protocol() {
withLocalProperty("protocol");
return this;
}
public Mask severity() {
withLocalProperty("severity");
return this;
}
public Mask signatureId() {
withLocalProperty("signatureId");
return this;
}
public Mask sourceIpAddress() {
withLocalProperty("sourceIpAddress");
return this;
}
public Mask sourcePort() {
withLocalProperty("sourcePort");
return this;
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy