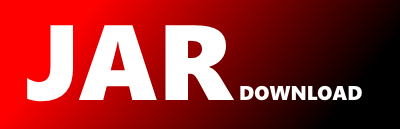
com.softlayer.api.service.Network Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of softlayer-api-client Show documentation
Show all versions of softlayer-api-client Show documentation
API client for accessing the SoftLayer API
package com.softlayer.api.service;
import com.softlayer.api.ApiClient;
import com.softlayer.api.ResponseHandler;
import com.softlayer.api.annotation.ApiMethod;
import com.softlayer.api.annotation.ApiProperty;
import com.softlayer.api.annotation.ApiType;
import com.softlayer.api.service.Entity;
import com.softlayer.api.service.network.Pod;
import com.softlayer.api.service.network.Subnet;
import java.util.ArrayList;
import java.util.List;
import java.util.concurrent.Future;
/**
* Provides services oriented to network-centric discovery and manipulation.
*
* @see SoftLayer_Network
*/
@ApiType("SoftLayer_Network")
public class Network extends Entity {
/**
* The size of the Network specified in CIDR notation. Specified in conjunction with the ``networkIdentifier`` to describe the bounding subnet size for the Network. Required for creation. See [[SoftLayer_Network/createObject]] documentation for creation details.
*/
@ApiProperty
protected Long cidr;
public Long getCidr() {
return cidr;
}
public void setCidr(Long cidr) {
this.cidr = cidr;
}
/**
* A name for the Network. This is required during creation of a Network and is entirely user defined.
*/
@ApiProperty
protected String name;
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
/**
* The starting IP address of the Network. Specified in conjunction with the ``cidr`` property to specify the bounding IP address space for the Network. Required for creation. See [[SoftLayer_Network/createObject]] documentation for creation details.
*/
@ApiProperty
protected String networkIdentifier;
public String getNetworkIdentifier() {
return networkIdentifier;
}
public void setNetworkIdentifier(String networkIdentifier) {
this.networkIdentifier = networkIdentifier;
}
/**
* Notes, or a description of the Network. This is entirely user defined.
*/
@ApiProperty
protected String notes;
public String getNotes() {
return notes;
}
public void setNotes(String notes) {
this.notes = notes;
}
/**
* The Subnets within the Network. These represent the realized segments of the Network and reside within a [[SoftLayer_Network_Pod|Pod]]. A Subnet must be specified when provisioning a compute resource within a Network.
*/
@ApiProperty
protected List subnets;
public List getSubnets() {
if (subnets == null) {
subnets = new ArrayList();
}
return subnets;
}
/**
* The owning account identifier.
*/
@ApiProperty(canBeNullOrNotSet = true)
protected Long accountId;
public Long getAccountId() {
return accountId;
}
public void setAccountId(Long accountId) {
accountIdSpecified = true;
this.accountId = accountId;
}
protected boolean accountIdSpecified;
public boolean isAccountIdSpecified() {
return accountIdSpecified;
}
public void unsetAccountId() {
accountId = null;
accountIdSpecified = false;
}
/**
* Unique identifier for the network.
*/
@ApiProperty(canBeNullOrNotSet = true)
protected Long id;
public Long getId() {
return id;
}
public void setId(Long id) {
idSpecified = true;
this.id = id;
}
protected boolean idSpecified;
public boolean isIdSpecified() {
return idSpecified;
}
public void unsetId() {
id = null;
idSpecified = false;
}
/**
* A count of the Subnets within the Network. These represent the realized segments of the Network and reside within a [[SoftLayer_Network_Pod|Pod]]. A Subnet must be specified when provisioning a compute resource within a Network.
*/
@ApiProperty
protected Long subnetCount;
public Long getSubnetCount() {
return subnetCount;
}
public void setSubnetCount(Long subnetCount) {
this.subnetCount = subnetCount;
}
public Service asService(ApiClient client) {
return service(client, id);
}
public static Service service(ApiClient client) {
return client.createService(Service.class, null);
}
public static Service service(ApiClient client, Long id) {
return client.createService(Service.class, id == null ? null : id.toString());
}
/**
* Provides services oriented to network-centric discovery and manipulation.
*
* @see SoftLayer_Network
*/
@com.softlayer.api.annotation.ApiService("SoftLayer_Network")
public static interface Service extends com.softlayer.api.Service {
public ServiceAsync asAsync();
public Mask withNewMask();
public Mask withMask();
public void setMask(Mask mask);
/**
* Initiate the automated process to establish connectivity granting the account private back-end network access to the services available through IBM Cloud Service Endpoint. Once initiated, the configuration process occurs asynchronously in the background.
*
*
*
* Responses
*
* True
The request to connect was successfully initiated.
*
* False
The account and Service Endpoint networks are already connected.
*
*
*
* Exceptions
*
* SoftLayer_Exception_NotReady
Thrown when the current network configuration will not support connection alteration.
*
*
*
*
*
* @see SoftLayer_Network::connectPrivateEndpointService
*/
@ApiMethod
public Boolean connectPrivateEndpointService();
/**
* Provide a template containing the following properties to create a Network:
* * networkIdentifier
* * cidr
* * name
*
*
* The ``networkIdentifier`` must be an IP address within RFC 1918 blocks:
* * 192.168.0.0/16
* * 172.16.0.0/12
* * 10.0.0.0/8
* The ``cidr`` must be an integer between 16 and 24, inclusive. The ``networkIdentifier``/``cidr`` must represent a valid subnet specification. The ``name`` must not be empty, but otherwise can contain up to 50 characters of user specified information to identify the Network.
*
* The subnet specification of the Network bounds the IP address space which can be utilized and constrains the creation of Subnets within the Network.
*
* Example networkIdentifier/CIDR combinations:
* * 192.168.0.0/16
* * 192.168.0.0/17
* * 172.16.0.0/16
* * 172.31.0.0/16
* * 10.0.0.0/16
* * 10.255.0.0/16
*
* @see SoftLayer_Network::createObject
*/
@ApiMethod
public Network createObject(Network templateObject);
/**
* Creation of a Subnet is necessary prior to provisioning compute resources into a Network. In order to create a Subnet, both a [[SoftLayer_Network_Subnet|Subnet]] and [[SoftLayer_Network_Pod|Pod]] must be specified. The Pod determines where the Subnet will be available for use by compute resources.
*
* Provide a Subnet template containing the following properties:
* * networkIdentifier
* * cidr
* The ``networkIdentifier`` must represent an IP address within that specified by the Network. The ``cidr`` must be an integer between 24 and 29, inclusive, and represent a subnet size smaller than the Network's. The ``networkIdentifier``/``cidr`` must represent a valid subnet specification.
*
* Provide a Pod template containing the following property:
* * name
* The ``name`` must represent a valid Pod e.g. sjc01.pod02. See [[SoftLayer_Network_Pod (type)]] for more information.
*
* The following constraints apply to Subnet creation:
* * It must fit within the bounds of the Network.
* * It must be no larger than /24 and no smaller than /29.
* * Its size must not equal that of the Network. This implies that a fully
* utilized Network will have a minimum of two Subnets.
* * The Pod must support the ability to create Networks by having the
* SUPPORTS_CUSTOMER_DEFINED_NETWORK capability. See [[SoftLayer_Network_Pod/getCapabilities]].
*
* @see SoftLayer_Network::createSubnet
*/
@ApiMethod(instanceRequired = true)
public Subnet createSubnet(Subnet subnet, Pod pod);
/**
* Remove the specified Network along with any Subnets.
*
* @see SoftLayer_Network::deleteObject
*/
@ApiMethod(instanceRequired = true)
public Boolean deleteObject();
/**
*
*
* Provide a Subnet template containing the following properties:
* * networkIdentifier
* * cidr
* The ``networkIdentifier`` must represent an IP address within that specified by the Network. The ``cidr`` must be an integer between 24 and 29, inclusive, and represent a subnet size smaller than the Network's. The ``networkIdentifier``/``cidr`` must represent a valid subnet specification. Or:
* * id
* The ``id`` must identify a Subnet in the Network. If the ``id`` is provided, the ``networkIdentifier``/``cidr`` will be ignored.
*
* Subnets may only be removed when no compute resources are utilizing them.
*
* @see SoftLayer_Network::deleteSubnet
*/
@ApiMethod(instanceRequired = true)
public Boolean deleteSubnet(Subnet subnet);
/**
* Initiate the automated process to revoke mutual connectivity from the account network and IBM Cloud Service Endpoint network. Once initiated, the configuration process occurs asynchronously in the background.
*
*
*
* Responses
*
* True
The request to disconnect was successfully initiated.
*
* False
The account and Service Endpoint networks are already disconnected.
*
*
*
* Exceptions
*
* SoftLayer_Exception_NotReady
Thrown when the current network configuration will not support connection alteration.
*
*
*
*
*
* @see SoftLayer_Network::disconnectPrivateEndpointService
*/
@ApiMethod
public Boolean disconnectPrivateEndpointService();
/**
* Modify either the ``name`` or ``notes`` properties of a Network.
*
* @see SoftLayer_Network::editObject
*/
@ApiMethod(instanceRequired = true)
public Boolean editObject(Network templateObject);
/**
* @see SoftLayer_Network::getAllObjects
*/
@ApiMethod
public List getAllObjects();
/**
* @see SoftLayer_Network::getObject
*/
@ApiMethod(instanceRequired = true)
public Network getObject();
/**
* Accessing select IBM Cloud services attached to the private back-end network is made possible by establishing a network relationship between an account's private network and the Service Endpoint network.
*
*
*
* Responses
*
* True
The account and Service Endpoint networks are currently connected.
*
* False
The account and Service Endpoint networks are not connected; both networks are properly configured to connect.
*
*
*
* Exceptions
*
* SoftLayer_Exception_NotReady
Thrown when the current network configuration will not support connection alteration.
*
*
*
*
*
* @see SoftLayer_Network::isConnectedToPrivateEndpointService
*/
@ApiMethod
public Boolean isConnectedToPrivateEndpointService();
/**
* The size of the Network specified in CIDR notation. Specified in conjunction with the ``networkIdentifier`` to describe the bounding subnet size for the Network. Required for creation. See [[SoftLayer_Network/createObject]] documentation for creation details.
*
* @see SoftLayer_Network::getCidr
*/
@ApiMethod(instanceRequired = true)
public Long getCidr();
/**
* A name for the Network. This is required during creation of a Network and is entirely user defined.
*
* @see SoftLayer_Network::getName
*/
@ApiMethod(instanceRequired = true)
public String getName();
/**
* The starting IP address of the Network. Specified in conjunction with the ``cidr`` property to specify the bounding IP address space for the Network. Required for creation. See [[SoftLayer_Network/createObject]] documentation for creation details.
*
* @see SoftLayer_Network::getNetworkIdentifier
*/
@ApiMethod(instanceRequired = true)
public String getNetworkIdentifier();
/**
* Notes, or a description of the Network. This is entirely user defined.
*
* @see SoftLayer_Network::getNotes
*/
@ApiMethod(instanceRequired = true)
public String getNotes();
/**
* The Subnets within the Network. These represent the realized segments of the Network and reside within a [[SoftLayer_Network_Pod|Pod]]. A Subnet must be specified when provisioning a compute resource within a Network.
*
* @see SoftLayer_Network::getSubnets
*/
@ApiMethod(instanceRequired = true)
public List getSubnets();
}
public static interface ServiceAsync extends com.softlayer.api.ServiceAsync {
public Mask withNewMask();
public Mask withMask();
public void setMask(Mask mask);
/**
* Async version of {@link Service#connectPrivateEndpointService}
*/
public Future connectPrivateEndpointService();
public Future> connectPrivateEndpointService(ResponseHandler callback);
/**
* Async version of {@link Service#createObject}
*/
public Future createObject(Network templateObject);
public Future> createObject(Network templateObject, ResponseHandler callback);
/**
* Async version of {@link Service#createSubnet}
*/
public Future createSubnet(Subnet subnet, Pod pod);
public Future> createSubnet(Subnet subnet, Pod pod, ResponseHandler callback);
/**
* Async version of {@link Service#deleteObject}
*/
public Future deleteObject();
public Future> deleteObject(ResponseHandler callback);
/**
* Async version of {@link Service#deleteSubnet}
*/
public Future deleteSubnet(Subnet subnet);
public Future> deleteSubnet(Subnet subnet, ResponseHandler callback);
/**
* Async version of {@link Service#disconnectPrivateEndpointService}
*/
public Future disconnectPrivateEndpointService();
public Future> disconnectPrivateEndpointService(ResponseHandler callback);
/**
* Async version of {@link Service#editObject}
*/
public Future editObject(Network templateObject);
public Future> editObject(Network templateObject, ResponseHandler callback);
/**
* Async version of {@link Service#getAllObjects}
*/
public Future> getAllObjects();
public Future> getAllObjects(ResponseHandler> callback);
/**
* Async version of {@link Service#getObject}
*/
public Future getObject();
public Future> getObject(ResponseHandler callback);
/**
* Async version of {@link Service#isConnectedToPrivateEndpointService}
*/
public Future isConnectedToPrivateEndpointService();
public Future> isConnectedToPrivateEndpointService(ResponseHandler callback);
/**
* Async version of {@link Service#getCidr}
*/
public Future getCidr();
/**
* Async callback version of {@link Service#getCidr}
*/
public Future> getCidr(ResponseHandler callback);
/**
* Async version of {@link Service#getName}
*/
public Future getName();
/**
* Async callback version of {@link Service#getName}
*/
public Future> getName(ResponseHandler callback);
/**
* Async version of {@link Service#getNetworkIdentifier}
*/
public Future getNetworkIdentifier();
/**
* Async callback version of {@link Service#getNetworkIdentifier}
*/
public Future> getNetworkIdentifier(ResponseHandler callback);
/**
* Async version of {@link Service#getNotes}
*/
public Future getNotes();
/**
* Async callback version of {@link Service#getNotes}
*/
public Future> getNotes(ResponseHandler callback);
/**
* Async version of {@link Service#getSubnets}
*/
public Future> getSubnets();
/**
* Async callback version of {@link Service#getSubnets}
*/
public Future> getSubnets(ResponseHandler> callback);
}
public static class Mask extends Entity.Mask {
public Mask cidr() {
withLocalProperty("cidr");
return this;
}
public Mask name() {
withLocalProperty("name");
return this;
}
public Mask networkIdentifier() {
withLocalProperty("networkIdentifier");
return this;
}
public Mask notes() {
withLocalProperty("notes");
return this;
}
public com.softlayer.api.service.network.Subnet.Mask subnets() {
return withSubMask("subnets", com.softlayer.api.service.network.Subnet.Mask.class);
}
public Mask accountId() {
withLocalProperty("accountId");
return this;
}
public Mask id() {
withLocalProperty("id");
return this;
}
public Mask subnetCount() {
withLocalProperty("subnetCount");
return this;
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy