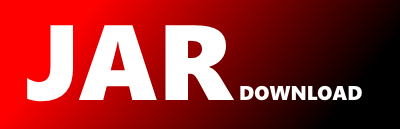
com.softlayer.api.service.network.bandwidth.Usage Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of softlayer-api-client Show documentation
Show all versions of softlayer-api-client Show documentation
API client for accessing the SoftLayer API
The newest version!
package com.softlayer.api.service.network.bandwidth;
import com.softlayer.api.annotation.ApiProperty;
import com.softlayer.api.annotation.ApiType;
import com.softlayer.api.service.Entity;
import com.softlayer.api.service.metric.tracking.Object;
import com.softlayer.api.service.network.bandwidth.version1.usage.detail.Type;
import java.math.BigDecimal;
/**
* The SoftLayer_Network_Bandwidth_Usage data type contains specific information relating to bandwidth utilization at a specific point in time on a given network interface.
*
* @see SoftLayer_Network_Bandwidth_Usage
*/
@ApiType("SoftLayer_Network_Bandwidth_Usage")
public class Usage extends Entity {
/**
* The tracking object this bandwidth usage record describes.
*/
@ApiProperty
protected Object trackingObject;
public Object getTrackingObject() {
return trackingObject;
}
public void setTrackingObject(Object trackingObject) {
this.trackingObject = trackingObject;
}
/**
* In and out bandwidth utilization for a specified time stamp.
*/
@ApiProperty
protected Type type;
public Type getType() {
return type;
}
public void setType(Type type) {
this.type = type;
}
/**
* Incoming bandwidth utilization.
*/
@ApiProperty(canBeNullOrNotSet = true)
protected BigDecimal amountIn;
public BigDecimal getAmountIn() {
return amountIn;
}
public void setAmountIn(BigDecimal amountIn) {
amountInSpecified = true;
this.amountIn = amountIn;
}
protected boolean amountInSpecified;
public boolean isAmountInSpecified() {
return amountInSpecified;
}
public void unsetAmountIn() {
amountIn = null;
amountInSpecified = false;
}
/**
* Outgoing bandwidth utilization.
*/
@ApiProperty(canBeNullOrNotSet = true)
protected BigDecimal amountOut;
public BigDecimal getAmountOut() {
return amountOut;
}
public void setAmountOut(BigDecimal amountOut) {
amountOutSpecified = true;
this.amountOut = amountOut;
}
protected boolean amountOutSpecified;
public boolean isAmountOutSpecified() {
return amountOutSpecified;
}
public void unsetAmountOut() {
amountOut = null;
amountOutSpecified = false;
}
/**
* ID of the bandwidth usage detail type for this record.
*/
@ApiProperty(canBeNullOrNotSet = true)
protected BigDecimal bandwidthUsageDetailTypeId;
public BigDecimal getBandwidthUsageDetailTypeId() {
return bandwidthUsageDetailTypeId;
}
public void setBandwidthUsageDetailTypeId(BigDecimal bandwidthUsageDetailTypeId) {
bandwidthUsageDetailTypeIdSpecified = true;
this.bandwidthUsageDetailTypeId = bandwidthUsageDetailTypeId;
}
protected boolean bandwidthUsageDetailTypeIdSpecified;
public boolean isBandwidthUsageDetailTypeIdSpecified() {
return bandwidthUsageDetailTypeIdSpecified;
}
public void unsetBandwidthUsageDetailTypeId() {
bandwidthUsageDetailTypeId = null;
bandwidthUsageDetailTypeIdSpecified = false;
}
public static class Mask extends com.softlayer.api.service.Entity.Mask {
public com.softlayer.api.service.metric.tracking.Object.Mask trackingObject() {
return withSubMask("trackingObject", com.softlayer.api.service.metric.tracking.Object.Mask.class);
}
public com.softlayer.api.service.network.bandwidth.version1.usage.detail.Type.Mask type() {
return withSubMask("type", com.softlayer.api.service.network.bandwidth.version1.usage.detail.Type.Mask.class);
}
public Mask amountIn() {
withLocalProperty("amountIn");
return this;
}
public Mask amountOut() {
withLocalProperty("amountOut");
return this;
}
public Mask bandwidthUsageDetailTypeId() {
withLocalProperty("bandwidthUsageDetailTypeId");
return this;
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy