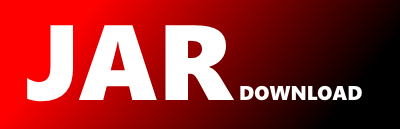
com.softwaremagico.tm.character.RandomPlanet Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of think-machine-random Show documentation
Show all versions of think-machine-random Show documentation
Think Machine - A Fading Suns character generator (random character creation)
package com.softwaremagico.tm.character;
/*-
* #%L
* Think Machine (Core)
* %%
* Copyright (C) 2017 - 2018 Softwaremagico
* %%
* This software is designed by Jorge Hortelano Otero. Jorge Hortelano Otero
* Valencia (Spain).
*
* This program is free software; you can redistribute it and/or modify it under
* the terms of the GNU General Public License as published by the Free Software
* Foundation; either version 2 of the License, or (at your option) any later
* version.
*
* This program is distributed in the hope that it will be useful, but WITHOUT
* ANY WARRANTY; without even the implied warranty of MERCHANTABILITY or FITNESS
* FOR A PARTICULAR PURPOSE. See the GNU General Public License for more
* details.
*
* You should have received a copy of the GNU General Public License along with
* this program; If not, see .
* #L%
*/
import com.softwaremagico.tm.InvalidXmlElementException;
import com.softwaremagico.tm.character.exceptions.RestrictedElementException;
import com.softwaremagico.tm.character.exceptions.UnofficialElementNotAllowedException;
import com.softwaremagico.tm.character.factions.Faction;
import com.softwaremagico.tm.character.planets.Planet;
import com.softwaremagico.tm.character.planets.PlanetFactory;
import com.softwaremagico.tm.character.races.InvalidRaceException;
import com.softwaremagico.tm.random.RandomSelector;
import com.softwaremagico.tm.random.exceptions.InvalidRandomElementSelectedException;
import com.softwaremagico.tm.random.selectors.IRandomPreference;
import java.util.Collection;
import java.util.Set;
public class RandomPlanet extends RandomSelector {
private static final int FACTION_PLANET = 50;
private static final int NEUTRAL_PLANET = 8;
private static final int ENEMY_PLANET = 1;
public RandomPlanet(CharacterPlayer characterPlayer, Set> preferences)
throws InvalidXmlElementException, RestrictedElementException, UnofficialElementNotAllowedException {
super(characterPlayer, preferences);
}
@Override
public void assign() throws InvalidRaceException, InvalidRandomElementSelectedException {
getCharacterPlayer().getInfo().setPlanet(selectElementByWeight());
}
@Override
protected Collection getAllElements() throws InvalidXmlElementException {
return PlanetFactory.getInstance().getElements(getCharacterPlayer().getLanguage(),
getCharacterPlayer().getModuleName());
}
@Override
protected int getWeight(Planet planet) {
//By race
if (!getCharacterPlayer().getRace().getPlanets().isEmpty() && !getCharacterPlayer().getRace().getPlanets().contains(planet)) {
return 0;
}
//By faction
if (planet.getFactions().contains(getCharacterPlayer().getFaction())) {
return FACTION_PLANET;
}
for (final Faction factionsOfPlanet : planet.getFactions()) {
if (factionsOfPlanet != null && getCharacterPlayer().getFaction() != null &&
factionsOfPlanet.getRestrictedToFactionGroup() == getCharacterPlayer().getFaction().getFactionGroup()) {
return ENEMY_PLANET;
}
}
return NEUTRAL_PLANET;
}
@Override
protected void assignIfMandatory(Planet element) throws InvalidXmlElementException {
return;
}
@Override
protected void assignMandatoryValues(Set mandatoryValues) throws InvalidXmlElementException {
return;
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy