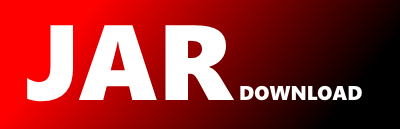
com.softwaremagico.tm.random.predefined.RandomPredefined Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of think-machine-random Show documentation
Show all versions of think-machine-random Show documentation
Think Machine - A Fading Suns character generator (random character creation)
package com.softwaremagico.tm.random.predefined;
/*-
* #%L
* Think Machine (Core)
* %%
* Copyright (C) 2017 - 2018 Softwaremagico
* %%
* This software is designed by Jorge Hortelano Otero. Jorge Hortelano Otero
* Valencia (Spain).
*
* This program is free software; you can redistribute it and/or modify it under
* the terms of the GNU General Public License as published by the Free Software
* Foundation; either version 2 of the License, or (at your option) any later
* version.
*
* This program is distributed in the hope that it will be useful, but WITHOUT
* ANY WARRANTY; without even the implied warranty of MERCHANTABILITY or FITNESS
* FOR A PARTICULAR PURPOSE. See the GNU General Public License for more
* details.
*
* You should have received a copy of the GNU General Public License along with
* this program; If not, see .
* #L%
*/
import com.softwaremagico.tm.Element;
import com.softwaremagico.tm.character.benefices.AvailableBenefice;
import com.softwaremagico.tm.character.benefices.BeneficeDefinition;
import com.softwaremagico.tm.character.blessings.Blessing;
import com.softwaremagico.tm.character.characteristics.Characteristic;
import com.softwaremagico.tm.character.characteristics.CharacteristicName;
import com.softwaremagico.tm.character.equipment.armours.Armour;
import com.softwaremagico.tm.character.equipment.shields.Shield;
import com.softwaremagico.tm.character.equipment.weapons.Weapon;
import com.softwaremagico.tm.character.factions.Faction;
import com.softwaremagico.tm.character.occultism.OccultismPath;
import com.softwaremagico.tm.character.races.Race;
import com.softwaremagico.tm.character.skills.AvailableSkill;
import com.softwaremagico.tm.json.ExcludeFromJson;
import com.softwaremagico.tm.random.selectors.IRandomPreference;
import java.util.*;
public abstract class RandomPredefined> extends Element implements IRandomPredefined {
private final Set> randomPreferences;
private final Set characteristicsMinimumValues;
private final Set requiredSkills;
private final Set suggestedSkills;
private final Set suggestedBenefices;
private final Set mandatoryBenefices;
private final Set mandatoryBeneficeSpecializations;
private final Set mandatoryBlessings;
private final Set suggestedBlessings;
private final Set mandatoryOccultismPaths;
private final Set suggestedBeneficeSpecializations;
private Faction faction;
private Race race;
private final String group;
@ExcludeFromJson
public boolean parentMerged = false;
public RandomPredefined(String id, String name, String description, String language, String moduleName,
Set> randomPreferences, Set characteristicsMinimumValues,
Set requiredSkills, Set suggestedSkills,
Set mandatoryBlessings, Set suggestedBlessings,
Set mandatoryBenefices, Set suggestedBenefices,
Set mandatoryBeneficeSpecializations, Set suggestedBeneficeSpecializations,
Set mandatoryOccultismPaths, Faction faction, Race race, String group) {
super(id, name, description, language, moduleName);
this.randomPreferences = randomPreferences;
this.characteristicsMinimumValues = characteristicsMinimumValues;
this.requiredSkills = requiredSkills;
this.suggestedSkills = suggestedSkills;
this.suggestedBenefices = suggestedBenefices;
this.mandatoryBenefices = mandatoryBenefices;
this.mandatoryBeneficeSpecializations = mandatoryBeneficeSpecializations;
this.mandatoryBlessings = mandatoryBlessings;
this.suggestedBlessings = suggestedBlessings;
this.suggestedBeneficeSpecializations = suggestedBeneficeSpecializations;
this.mandatoryOccultismPaths = mandatoryOccultismPaths;
this.faction = faction;
this.race = race;
this.group = group;
}
public RandomPredefined(String id, String name, String description, String language, String moduleName, String group) {
this(id, name, description, language, moduleName, new HashSet<>(), new HashSet<>(), new HashSet<>(), new HashSet<>(),
new HashSet<>(), new HashSet<>(), new HashSet<>(), new HashSet<>(), new HashSet<>(), new HashSet<>(), new HashSet<>(),
null, null, group);
}
public void copy(IRandomPredefined randomPredefined) {
if (randomPredefined == null) {
return;
}
randomPreferences.addAll(randomPredefined.getPreferences());
characteristicsMinimumValues.addAll(randomPredefined.getCharacteristicsMinimumValues());
requiredSkills.addAll(randomPredefined.getRequiredSkills());
suggestedSkills.addAll(randomPredefined.getSuggestedSkills());
suggestedBenefices.addAll(randomPredefined.getSuggestedBenefices());
mandatoryBenefices.addAll(randomPredefined.getMandatoryBenefices());
mandatoryBeneficeSpecializations.addAll(randomPredefined.getMandatoryBeneficeSpecializations());
suggestedBeneficeSpecializations.addAll(randomPredefined.getSuggestedBeneficeSpecializations());
mandatoryBlessings.addAll(randomPredefined.getMandatoryBlessings());
suggestedBlessings.addAll(randomPredefined.getSuggestedBlessings());
mandatoryOccultismPaths.addAll(randomPredefined.getMandatoryOccultismPaths());
if (faction == null) {
faction = randomPredefined.getFaction();
}
if (race == null) {
race = randomPredefined.getRace();
}
this.setRestricted(randomPredefined.isRestricted());
this.setOfficial(randomPredefined.isOfficial());
}
@Override
public void setParent(IRandomPredefined parentProfile) {
if (!parentMerged && parentProfile != null) {
// Merge preferences. This has preference over parent profile.
final IRandomPredefined mergedProfile = PredefinedMerger.merge(parentProfile.getLanguage(),
parentProfile.getModuleName(), parentProfile, this);
randomPreferences.clear();
randomPreferences.addAll(mergedProfile.getPreferences());
characteristicsMinimumValues.clear();
characteristicsMinimumValues.addAll(mergedProfile.getCharacteristicsMinimumValues());
requiredSkills.addAll(mergedProfile.getRequiredSkills());
suggestedSkills.addAll(mergedProfile.getSuggestedSkills());
suggestedBenefices.addAll(mergedProfile.getSuggestedBenefices());
mandatoryBenefices.addAll(mergedProfile.getMandatoryBenefices());
parentMerged = true;
}
}
@Override
public Set getCharacteristicsMinimumValues() {
return characteristicsMinimumValues;
}
@Override
public Characteristic getCharacteristicMinimumValues(CharacteristicName characteristicName) {
for (final Characteristic characteristic : getCharacteristicsMinimumValues()) {
if (Objects
.equals(characteristic.getCharacteristicDefinition().getCharacteristicName(), characteristicName)) {
return characteristic;
}
}
return null;
}
@Override
public Map getSkillsMinimumValues() {
return new HashMap<>();
}
@Override
public Set getBlessings() {
return new HashSet<>();
}
@Override
public Set getBenefices() {
return new HashSet<>();
}
@Override
public int getExperiencePoints() {
return 0;
}
@Override
public Set> getPreferences() {
return randomPreferences;
}
@Override
public boolean isParentMerged() {
return parentMerged;
}
public void setParentMerged(boolean parentMerged) {
this.parentMerged = parentMerged;
}
@Override
public Set getRequiredSkills() {
return requiredSkills;
}
@Override
public Set getSuggestedSkills() {
return suggestedSkills;
}
@Override
public Set getSuggestedBenefices() {
return suggestedBenefices;
}
@Override
public Set getMandatoryBenefices() {
return mandatoryBenefices;
}
@Override
public Set getMandatoryBeneficeSpecializations() {
return mandatoryBeneficeSpecializations;
}
@Override
public Set getSuggestedBeneficeSpecializations() {
return suggestedBeneficeSpecializations;
}
@Override
public Set getMandatoryBlessings() {
return mandatoryBlessings;
}
@Override
public Set getSuggestedBlessings() {
return suggestedBlessings;
}
@Override
public int hashCode() {
return super.hashCode();
}
@Override
public boolean equals(Object obj) {
return super.equals(obj);
}
@Override
public Set getMandatoryWeapons() {
return new HashSet<>();
}
@Override
public Set getMandatoryArmours() {
return new HashSet<>();
}
@Override
public Set getMandatoryShields() {
return new HashSet<>();
}
@Override
public Set getMandatoryOccultismPaths() {
return mandatoryOccultismPaths;
}
@Override
public Faction getFaction() {
return faction;
}
@Override
public void setFaction(Faction faction) {
this.faction = faction;
}
@Override
public Race getRace() {
return race;
}
@Override
public void setRace(Race race) {
this.race = race;
}
@Override
public boolean isOfficial() {
return super.isOfficial() && (getFaction() == null || getFaction().isOfficial()) &&
(getRace() == null || getRace().isOfficial());
}
@Override
public String getGroup() {
return group;
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy