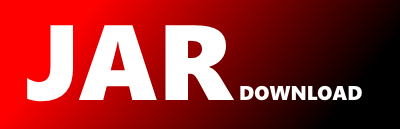
com.solidfire.element.api.SolidFireElementIF Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of solidfire-sdk-java Show documentation
Show all versions of solidfire-sdk-java Show documentation
Library for interfacing with the Public and Incubating SolidFire Element API.
The newest version!
/*
* Copyright © 2014-2016 NetApp, Inc. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
/*
* DO NOT EDIT THIS CODE BY HAND! It has been generated with jsvcgen.
*/
package com.solidfire.element.api;
import com.solidfire.core.client.Attributes;
import com.solidfire.core.annotation.*;
import com.solidfire.core.javautil.Optional;
/**
* The API for controlling a SolidFire cluster.
**/
public interface SolidFireElementIF {
/**
* You can use AddAccount to add a new account to the system. You can create new volumes under the new account. The CHAP settings you specify for the account apply to all volumes owned by the account.
**/
@Since("1.0")
@ConnectionType("Cluster")
public AddAccountResult addAccount(final AddAccountRequest request);
/**
* You can use AddAccount to add a new account to the system. You can create new volumes under the new account. The CHAP settings you specify for the account apply to all volumes owned by the account.
**/
@Since("1.0")
@ConnectionType("Cluster")
public AddAccountResult addAccount(
String username,
Optional initiatorSecret,
Optional targetSecret,
Optional attributes
);
/**
* GetAccountByID enables you to return details about a specific account, given its accountID.
**/
@Since("1.0")
@ConnectionType("Cluster")
public GetAccountResult getAccountByID(final GetAccountByIDRequest request);
/**
* GetAccountByID enables you to return details about a specific account, given its accountID.
**/
@Since("1.0")
@ConnectionType("Cluster")
public GetAccountResult getAccountByID(
Long accountID
);
/**
* GetAccountByName enables you to retrieve details about a specific account, given its username.
**/
@Since("1.0")
@ConnectionType("Cluster")
public GetAccountResult getAccountByName(final GetAccountByNameRequest request);
/**
* GetAccountByName enables you to retrieve details about a specific account, given its username.
**/
@Since("1.0")
@ConnectionType("Cluster")
public GetAccountResult getAccountByName(
String username
);
/**
* GetAccountEfficiency enables you to retrieve efficiency statistics about a volume account. This method returns efficiency information
* only for the account you specify as a parameter.
**/
@Since("6.0")
@ConnectionType("Cluster")
public GetEfficiencyResult getAccountEfficiency(final GetAccountEfficiencyRequest request);
/**
* GetAccountEfficiency enables you to retrieve efficiency statistics about a volume account. This method returns efficiency information
* only for the account you specify as a parameter.
**/
@Since("6.0")
@ConnectionType("Cluster")
public GetEfficiencyResult getAccountEfficiency(
Long accountID
);
/**
* ListAccounts returns the entire list of accounts, with optional paging support.
**/
@Since("1.0")
@ConnectionType("Cluster")
public ListAccountsResult listAccounts(final ListAccountsRequest request);
/**
* ListAccounts returns the entire list of accounts, with optional paging support.
**/
@Since("1.0")
@ConnectionType("Cluster")
public ListAccountsResult listAccounts(
Optional startAccountID,
Optional limit
);
/**
* ListAccounts returns the entire list of accounts, with optional paging support.
**/
@Since("1.0")
@ConnectionType("Cluster")
public ListAccountsResult listAccounts(
Optional startAccountID,
Optional limit,
Optional includeStorageContainers
);
/**
* ModifyAccount enables you to modify an existing account.
* When you lock an account, any existing connections from that account are immediately terminated. When you change an account's
* CHAP settings, any existing connections remain active, and the new CHAP settings are used on subsequent connections or
* reconnections.
* To clear an account's attributes, specify {} for the attributes parameter.
**/
@Since("1.0")
@ConnectionType("Cluster")
public ModifyAccountResult modifyAccount(final ModifyAccountRequest request);
/**
* ModifyAccount enables you to modify an existing account.
* When you lock an account, any existing connections from that account are immediately terminated. When you change an account's
* CHAP settings, any existing connections remain active, and the new CHAP settings are used on subsequent connections or
* reconnections.
* To clear an account's attributes, specify {} for the attributes parameter.
**/
@Since("1.0")
@ConnectionType("Cluster")
public ModifyAccountResult modifyAccount(
Long accountID,
Optional username,
Optional status,
Optional initiatorSecret,
Optional targetSecret,
Optional attributes
);
/**
* RemoveAccount enables you to remove an existing account. You must delete and purge all volumes associated with the account
* using DeleteVolume before you can remove the account. If volumes on the account are still pending deletion, you cannot use
* RemoveAccount to remove the account.
**/
@Since("1.0")
@ConnectionType("Cluster")
public RemoveAccountResult removeAccount(final RemoveAccountRequest request);
/**
* RemoveAccount enables you to remove an existing account. You must delete and purge all volumes associated with the account
* using DeleteVolume before you can remove the account. If volumes on the account are still pending deletion, you cannot use
* RemoveAccount to remove the account.
**/
@Since("1.0")
@ConnectionType("Cluster")
public RemoveAccountResult removeAccount(
Long accountID
);
/**
* You can use GetAsyncResult to retrieve the result of asynchronous method calls. Some method calls require some time to run, and
* might not be finished when the system sends the initial response. To obtain the status or result of the method call, use
* GetAsyncResult to poll the asyncHandle value returned by the method.
* GetAsyncResult returns the overall status of the operation (in progress, completed, or error) in a standard fashion, but the actual
* data returned for the operation depends on the original method call and the return data is documented with each method.
**/
@Since("1.0")
@ConnectionType("Cluster")
public Attributes getAsyncResult(final GetAsyncResultRequest request);
/**
* You can use GetAsyncResult to retrieve the result of asynchronous method calls. Some method calls require some time to run, and
* might not be finished when the system sends the initial response. To obtain the status or result of the method call, use
* GetAsyncResult to poll the asyncHandle value returned by the method.
* GetAsyncResult returns the overall status of the operation (in progress, completed, or error) in a standard fashion, but the actual
* data returned for the operation depends on the original method call and the return data is documented with each method.
**/
@Since("1.0")
@ConnectionType("Cluster")
public Attributes getAsyncResult(
Long asyncHandle,
Optional keepResult
);
/**
* You can use ListAsyncResults to list the results of all currently running and completed asynchronous methods on the system.
* Querying asynchronous results with ListAsyncResults does not cause completed asyncHandles to expire; you can use GetAsyncResult
* to query any of the asyncHandles returned by ListAsyncResults.
**/
@Since("9.0")
@ConnectionType("Cluster")
public ListAsyncResultsResult listAsyncResults(final ListAsyncResultsRequest request);
/**
* You can use ListAsyncResults to list the results of all currently running and completed asynchronous methods on the system.
* Querying asynchronous results with ListAsyncResults does not cause completed asyncHandles to expire; you can use GetAsyncResult
* to query any of the asyncHandles returned by ListAsyncResults.
**/
@Since("9.0")
@ConnectionType("Cluster")
public ListAsyncResultsResult listAsyncResults(
Optional asyncResultTypes
);
/**
* CreateBackupTarget enables you to create and store backup target information so that you do not need to re-enter it each time a backup is created.
**/
@Since("6.0")
@ConnectionType("Cluster")
public CreateBackupTargetResult createBackupTarget(final CreateBackupTargetRequest request);
/**
* CreateBackupTarget enables you to create and store backup target information so that you do not need to re-enter it each time a backup is created.
**/
@Since("6.0")
@ConnectionType("Cluster")
public CreateBackupTargetResult createBackupTarget(
String name,
Attributes attributes
);
/**
* GetBackupTarget enables you to return information about a specific backup target that you have created.
**/
@Since("6.0")
@ConnectionType("Cluster")
public GetBackupTargetResult getBackupTarget(final GetBackupTargetRequest request);
/**
* GetBackupTarget enables you to return information about a specific backup target that you have created.
**/
@Since("6.0")
@ConnectionType("Cluster")
public GetBackupTargetResult getBackupTarget(
Long backupTargetID
);
/**
* You can use ListBackupTargets to retrieve information about all backup targets that have been created.
**/
@Since("6.0")
@ConnectionType("Cluster")
public ListBackupTargetsResult listBackupTargets();
/**
* ModifyBackupTarget enables you to change attributes of a backup target.
**/
@Since("6.0")
@ConnectionType("Cluster")
public ModifyBackupTargetResult modifyBackupTarget(final ModifyBackupTargetRequest request);
/**
* ModifyBackupTarget enables you to change attributes of a backup target.
**/
@Since("6.0")
@ConnectionType("Cluster")
public ModifyBackupTargetResult modifyBackupTarget(
Long backupTargetID,
Optional name,
Optional attributes
);
/**
* RemoveBackupTarget allows you to delete backup targets.
**/
@Since("6.0")
@ConnectionType("Cluster")
public RemoveBackupTargetResult removeBackupTarget(final RemoveBackupTargetRequest request);
/**
* RemoveBackupTarget allows you to delete backup targets.
**/
@Since("6.0")
@ConnectionType("Cluster")
public RemoveBackupTargetResult removeBackupTarget(
Long backupTargetID
);
/**
* You can use the ClearClusterFaults method to clear information about both current and previously detected faults. Both resolved
* and unresolved faults can be cleared.
**/
@Since("1.0")
@ConnectionType("Cluster")
public ClearClusterFaultsResult clearClusterFaults(final ClearClusterFaultsRequest request);
/**
* You can use the ClearClusterFaults method to clear information about both current and previously detected faults. Both resolved
* and unresolved faults can be cleared.
**/
@Since("1.0")
@ConnectionType("Cluster")
public ClearClusterFaultsResult clearClusterFaults(
Optional faultTypes
);
/**
* The CreateCluster method enables you to initialize the node in a cluster that has ownership of the "mvip" and "svip" addresses. Each new cluster is initialized using the management IP (MIP) of the first node in the cluster. This method also automatically adds all the nodes being configured into the cluster. You only need to use this method once each time a new cluster is initialized.
* Note: You need to log in to the node that is used as the master node for the cluster. After you log in, run the GetBootstrapConfig method on the node to get the IP addresses for the rest of the nodes that you want to include in the
* cluster. Then, run the CreateCluster method.
**/
@Since("7.0")
@ConnectionType("Both")
public CreateClusterResult createCluster(final CreateClusterRequest request);
/**
* The CreateCluster method enables you to initialize the node in a cluster that has ownership of the "mvip" and "svip" addresses. Each new cluster is initialized using the management IP (MIP) of the first node in the cluster. This method also automatically adds all the nodes being configured into the cluster. You only need to use this method once each time a new cluster is initialized.
* Note: You need to log in to the node that is used as the master node for the cluster. After you log in, run the GetBootstrapConfig method on the node to get the IP addresses for the rest of the nodes that you want to include in the
* cluster. Then, run the CreateCluster method.
**/
@Since("7.0")
@ConnectionType("Both")
public CreateClusterResult createCluster(
Optional acceptEula,
String mvip,
String svip,
Long repCount,
String username,
String password,
String[] nodes,
Optional attributes
);
/**
* CreateSupportBundle enables you to create a support bundle file under the node's directory. After creation, the bundle is stored on the node as a tar.gz file.
**/
@Since("8.0")
@ConnectionType("Node")
public CreateSupportBundleResult createSupportBundle(final CreateSupportBundleRequest request);
/**
* CreateSupportBundle enables you to create a support bundle file under the node's directory. After creation, the bundle is stored on the node as a tar.gz file.
**/
@Since("8.0")
@ConnectionType("Node")
public CreateSupportBundleResult createSupportBundle(
Optional bundleName,
Optional extraArgs,
Optional timeoutSec
);
/**
* DeleteAllSupportBundles enables you to delete all support bundles generated with the CreateSupportBundle API method.
**/
@Since("8.0")
@ConnectionType("Node")
public DeleteAllSupportBundlesResult deleteAllSupportBundles();
/**
* The DisableEncryptionAtRest method enables you to remove the encryption that was previously applied to the cluster using the EnableEncryptionAtRest method. This disable method is asynchronous and returns a response before encryption is disabled. You can use the GetClusterInfo method to poll the system to see when the process has completed.
**/
@Since("5.0")
@ConnectionType("Cluster")
public DisableEncryptionAtRestResult disableEncryptionAtRest();
/**
* You can use the EnableEncryptionAtRest method to enable the Advanced Encryption Standard (AES) 256-bit encryption at rest on the cluster, so that the cluster can manage the encryption key used for the drives on each node. This feature is not enabled by default.
* When you enable Encryption at Rest, the cluster automatically manages encryption keys internally for the drives on each node in the cluster. Nodes do not store the keys to unlock drives and the keys are never passed over the network. Two nodes participating in a cluster are required to access the key to disable encryption on a drive. The encryption management does not affect performance or efficiency on the cluster. If an encryption-enabled drive or node is removed from the cluster with the API, Encryption at Rest is disabled and the data is not secure erased. Data can be secure erased using the SecureEraseDrives API method.
* Note: If you have a node type with a model number ending in "-NE", the EnableEncryptionAtRest method call fails with a response of "Encryption not allowed. Cluster detected non-encryptable node".
* You should only enable or disable encryption when the cluster is running and in a healthy state. You can enable or disable encryption at your discretion and as often as you need.
* Note: This process is asynchronous and returns a response before encryption is enabled. You can use the GetClusterInfo
* method to poll the system to see when the process has completed.
**/
@Since("5.0")
@ConnectionType("Cluster")
public EnableEncryptionAtRestResult enableEncryptionAtRest();
/**
* You can use the GetAPI method to return a list of all the API methods and supported API endpoints that can be used in the system.
**/
@Since("1.0")
@ConnectionType("Both")
public GetAPIResult getAPI();
/**
* You can use the GetClusterCapacity method to return the high-level capacity measurements for an entire cluster. You can use the fields returned from this method to calculate the efficiency rates that are displayed in the Element OS Web UI. You can use the following calculations in scripts to return the efficiency rates for thin provisioning, deduplication, compression, and overall efficiency.
**/
@Since("1.0")
@ConnectionType("Cluster")
public GetClusterCapacityResult getClusterCapacity();
/**
* The GetClusterConfig API method enables you to return information about the cluster configuration this node uses to communicate with the cluster that it is a part of.
**/
@Since("5.0")
@ConnectionType("Node")
public GetClusterConfigResult getClusterConfig();
/**
* You can use GetClusterFullThreshold to view the stages set for cluster fullness levels. This method returns all fullness metrics for the
* cluster.
* Note: When a cluster reaches the Error stage of block cluster fullness, the maximum IOPS on all volumes are reduced linearly to the volume's minimum IOPS as the cluster approaches the Critical stage. This helps prevent the cluster from
* reaching the Critical stage of block cluster fullness.
**/
@Since("1.0")
@ConnectionType("Cluster")
public GetClusterFullThresholdResult getClusterFullThreshold();
/**
* GetClusterInfo enables you to return configuration information about the cluster.
**/
@Since("1.0")
@ConnectionType("Cluster")
public GetClusterInfoResult getClusterInfo();
/**
* GetClusterMasterNodeID enables you to retrieve the ID of the node that can perform cluster-wide administration tasks and holds the
* storage virtual IP address (SVIP) and management virtual IP address (MVIP).
**/
@Since("1.0")
@ConnectionType("Cluster")
public GetClusterMasterNodeIDResult getClusterMasterNodeID();
/**
* The GetClusterState API method enables you to indicate if a node is part of a cluster or not. The three states are:
* Available: Node has not been configured with a cluster name.
* Pending: Node is pending for a specific named cluster and can be added.
* Active: Node is an active member of a cluster and may not be added to another
* cluster.
* Note: This method is available only through the per-node API endpoint 5.0 or later.
**/
@Since("5.0")
@ConnectionType("Cluster")
public GetClusterStateResult getClusterState(final GetClusterStateRequest request);
/**
* The GetClusterState API method enables you to indicate if a node is part of a cluster or not. The three states are:
* Available: Node has not been configured with a cluster name.
* Pending: Node is pending for a specific named cluster and can be added.
* Active: Node is an active member of a cluster and may not be added to another
* cluster.
* Note: This method is available only through the per-node API endpoint 5.0 or later.
**/
@Since("5.0")
@ConnectionType("Cluster")
public GetClusterStateResult getClusterState(
Boolean force
);
/**
* GetClusterStats enables you to retrieve high-level activity measurements for the cluster. Values returned are cumulative from the
* creation of the cluster.
**/
@Since("1.0")
@ConnectionType("Cluster")
public GetClusterStatsResult getClusterStats();
/**
* GetClusterVersionInfo enables you to retrieve information about the Element software version running on each node in the cluster.
* This method also returns information about nodes that are currently in the process of upgrading software.
**/
@Since("1.0")
@ConnectionType("Cluster")
public GetClusterVersionInfoResult getClusterVersionInfo();
/**
* NetApp engineering uses the GetCompleteStats API method to troubleshoot new features. The data returned from GetCompleteStats is not documented, changes frequently, and is not guaranteed to be accurate. NetApp does not recommend using GetCompleteStats for collecting performance data or any other
* management integration with a SolidFire cluster.
**/
@Since("1.0")
@ConnectionType("Cluster")
public Attributes getCompleteStats();
/**
* GetLimits enables you to retrieve the limit values set by the API. These values might change between releases of Element OS, but do not change without an update to the system. Knowing the limit values set by the API can be useful when writing API scripts for user-facing tools.
* Note: The GetLimits method returns the limits for the current software version regardless of the API endpoint version used to pass the method.
**/
@Since("1.0")
@ConnectionType("Cluster")
public GetLimitsResult getLimits();
/**
* GetNtpInfo enables you to return the current network time protocol (NTP) configuration information.
**/
@Since("1.0")
@ConnectionType("Cluster")
public GetNtpInfoResult getNtpInfo();
/**
* NetApp engineering uses the GetRawStats API method to troubleshoot new features. The data returned from GetRawStats is not documented, changes frequently, and is not guaranteed to be accurate. NetApp does not recommend using GetCompleteStats for collecting performance data or any other
* management integration with a SolidFire cluster.
**/
@Since("1.0")
@ConnectionType("Cluster")
public Attributes getRawStats();
/**
* You can use the GetSSLCertificate method to retrieve the SSL certificate that is currently active on the cluster.
**/
@Since("10.0")
@ConnectionType("Cluster")
public GetSSLCertificateResult getSSLCertificate();
/**
* GetSystemStatus enables you to return whether a reboot ir required or not.
**/
@Since("5.0")
@ConnectionType("Node")
public GetSystemStatusResult getSystemStatus();
/**
* ListClusterFaults enables you to retrieve information about any faults detected on the cluster. With this method, you can retrieve both current faults as well as faults that have been resolved. The system caches faults every 30 seconds.
**/
@Since("1.0")
@ConnectionType("Cluster")
public ListClusterFaultsResult listClusterFaults(final ListClusterFaultsRequest request);
/**
* ListClusterFaults enables you to retrieve information about any faults detected on the cluster. With this method, you can retrieve both current faults as well as faults that have been resolved. The system caches faults every 30 seconds.
**/
@Since("1.0")
@ConnectionType("Cluster")
public ListClusterFaultsResult listClusterFaults(
Optional bestPractices,
Optional faultTypes
);
/**
* ListEvents returns events detected on the cluster, sorted from oldest to newest.
**/
@Since("1.0")
@ConnectionType("Cluster")
public ListEventsResult listEvents(final ListEventsRequest request);
/**
* ListEvents returns events detected on the cluster, sorted from oldest to newest.
**/
@Since("1.0")
@ConnectionType("Cluster")
public ListEventsResult listEvents(
Optional maxEvents,
Optional startEventID,
Optional endEventID
);
/**
* ListEvents returns events detected on the cluster, sorted from oldest to newest.
**/
@Since("1.0")
@ConnectionType("Cluster")
public ListEventsResult listEvents(
Optional maxEvents,
Optional startEventID,
Optional endEventID,
Optional eventType
);
/**
* ListSyncJobs enables you to return information about synchronization jobs that are running on a SolidFire cluster. The type of
* synchronization jobs that are returned with this method are slice, clone, and remote.
**/
@Since("1.0")
@ConnectionType("Cluster")
public ListSyncJobsResult listSyncJobs();
/**
* You can use ModifyClusterFullThreshold to change the level at which the system generates an event when the storage cluster approaches a certain capacity utilization. You can use the threshold setting to indicate the acceptable amount of utilized block storage before the system generates a warning. For example, if you want to be alerted when the system reaches 3% below the "Error" level block storage utilization, enter a value of "3" for the stage3BlockThresholdPercent parameter. If this level is reached, the system sends an alert to the Event Log in the Cluster Management Console.
**/
@Since("1.0")
@ConnectionType("Cluster")
public ModifyClusterFullThresholdResult modifyClusterFullThreshold(final ModifyClusterFullThresholdRequest request);
/**
* You can use ModifyClusterFullThreshold to change the level at which the system generates an event when the storage cluster approaches a certain capacity utilization. You can use the threshold setting to indicate the acceptable amount of utilized block storage before the system generates a warning. For example, if you want to be alerted when the system reaches 3% below the "Error" level block storage utilization, enter a value of "3" for the stage3BlockThresholdPercent parameter. If this level is reached, the system sends an alert to the Event Log in the Cluster Management Console.
**/
@Since("1.0")
@ConnectionType("Cluster")
public ModifyClusterFullThresholdResult modifyClusterFullThreshold(
Optional stage2AwareThreshold,
Optional maxMetadataOverProvisionFactor
);
/**
* You can use ModifyClusterFullThreshold to change the level at which the system generates an event when the storage cluster approaches a certain capacity utilization. You can use the threshold setting to indicate the acceptable amount of utilized block storage before the system generates a warning. For example, if you want to be alerted when the system reaches 3% below the "Error" level block storage utilization, enter a value of "3" for the stage3BlockThresholdPercent parameter. If this level is reached, the system sends an alert to the Event Log in the Cluster Management Console.
**/
@Since("1.0")
@ConnectionType("Cluster")
public ModifyClusterFullThresholdResult modifyClusterFullThreshold(
Optional stage2AwareThreshold,
Optional stage3BlockThresholdPercent,
Optional maxMetadataOverProvisionFactor
);
/**
* You can use the RemoveSSLCertificate method to remove the user SSL certificate and private key for the cluster.
* After the certificate and private key are removed, the cluster is configured to use the default certificate and private key.
**/
@Since("10.0")
@ConnectionType("Cluster")
public RemoveSSLCertificateResult removeSSLCertificate();
/**
* The SetClusterConfig API method enables you to set the configuration this node uses to communicate with the cluster it is associated with. To see the states in which these objects can be modified, see Cluster Object Attributes. To display the current cluster
* interface settings for a node, run the GetClusterConfig API method.
* Note: This method is available only through the per-node API endpoint 5.0 or later.
**/
@Since("5.0")
@ConnectionType("Node")
public SetClusterConfigResult setClusterConfig(final SetClusterConfigRequest request);
/**
* The SetClusterConfig API method enables you to set the configuration this node uses to communicate with the cluster it is associated with. To see the states in which these objects can be modified, see Cluster Object Attributes. To display the current cluster
* interface settings for a node, run the GetClusterConfig API method.
* Note: This method is available only through the per-node API endpoint 5.0 or later.
**/
@Since("5.0")
@ConnectionType("Node")
public SetClusterConfigResult setClusterConfig(
ClusterConfig cluster
);
/**
* SetNtpInfo enables you to configure NTP on cluster nodes. The values you set with this interface apply to all nodes in the cluster. If an NTP broadcast server periodically broadcasts time information on your network, you can optionally configure nodes as broadcast clients.
* Note: NetApp recommends using NTP servers that are internal to your network, rather than the installation defaults.
**/
@Since("1.0")
@ConnectionType("Cluster")
public SetNtpInfoResult setNtpInfo(final SetNtpInfoRequest request);
/**
* SetNtpInfo enables you to configure NTP on cluster nodes. The values you set with this interface apply to all nodes in the cluster. If an NTP broadcast server periodically broadcasts time information on your network, you can optionally configure nodes as broadcast clients.
* Note: NetApp recommends using NTP servers that are internal to your network, rather than the installation defaults.
**/
@Since("1.0")
@ConnectionType("Cluster")
public SetNtpInfoResult setNtpInfo(
String[] servers,
Optional broadcastclient
);
/**
* You can use the SetSSLCertificate method to set a user SSL certificate and a private key for the cluster.
**/
@Since("10.0")
@ConnectionType("Cluster")
public SetSSLCertificateResult setSSLCertificate(final SetSSLCertificateRequest request);
/**
* You can use the SetSSLCertificate method to set a user SSL certificate and a private key for the cluster.
**/
@Since("10.0")
@ConnectionType("Cluster")
public SetSSLCertificateResult setSSLCertificate(
String certificate,
String privateKey
);
/**
* You can use AddClusterAdmin to add a new cluster admin account. A cluster ddmin can manage the cluster using the API and management tools. Cluster admins are completely separate and unrelated to standard tenant accounts.
* Each cluster admin can be restricted to a subset of the API. NetApp recommends using multiple cluster admin accounts for different users and applications. You should give each cluster admin the minimal permissions necessary; this reduces the potential impact of credential compromise.
* You must accept the End User License Agreement (EULA) by setting the acceptEula parameter to true to add a cluster administrator account to the system.
**/
@Since("1.0")
@ConnectionType("Cluster")
public AddClusterAdminResult addClusterAdmin(final AddClusterAdminRequest request);
/**
* You can use AddClusterAdmin to add a new cluster admin account. A cluster ddmin can manage the cluster using the API and management tools. Cluster admins are completely separate and unrelated to standard tenant accounts.
* Each cluster admin can be restricted to a subset of the API. NetApp recommends using multiple cluster admin accounts for different users and applications. You should give each cluster admin the minimal permissions necessary; this reduces the potential impact of credential compromise.
* You must accept the End User License Agreement (EULA) by setting the acceptEula parameter to true to add a cluster administrator account to the system.
**/
@Since("1.0")
@ConnectionType("Cluster")
public AddClusterAdminResult addClusterAdmin(
String username,
String password,
String[] access,
Optional acceptEula,
Optional attributes
);
/**
* GetCurrentClusterAdmin returns information for the current primary cluster administrator. The primary Cluster Admin was created when the cluster was created.
**/
@Since("6.0")
@ConnectionType("Cluster")
public GetCurrentClusterAdminResult getCurrentClusterAdmin();
/**
* You can use the GetLoginBanner method to get the currently active Terms of Use banner that users see when they log on to the web interface.
**/
@Since("10.0")
@ConnectionType("Cluster")
public GetLoginBannerResult getLoginBanner();
/**
* ListClusterAdmins returns the list of all cluster administrators for the cluster. There can be several cluster administrator accounts with different levels of permissions. There can be only one primary cluster administrator in the system. The primary Cluster Admin is the administrator that was created when the cluster was created. You can also create LDAP administrators when setting up an LDAP system on the cluster.
**/
@Since("1.0")
@ConnectionType("Cluster")
public ListClusterAdminsResult listClusterAdmins();
/**
* You can use ModifyClusterAdmin to change the settings for a cluster admin or LDAP cluster admin. You cannot change access for the administrator cluster admin account.
**/
@Since("1.0")
@ConnectionType("Cluster")
public ModifyClusterAdminResult modifyClusterAdmin(final ModifyClusterAdminRequest request);
/**
* You can use ModifyClusterAdmin to change the settings for a cluster admin or LDAP cluster admin. You cannot change access for the administrator cluster admin account.
**/
@Since("1.0")
@ConnectionType("Cluster")
public ModifyClusterAdminResult modifyClusterAdmin(
Long clusterAdminID,
Optional password,
Optional access,
Optional attributes
);
/**
* You can use RemoveClusterAdmin to remove a Cluster Admin. You cannot remove the administrator cluster admin account.
**/
@Since("1.0")
@ConnectionType("Cluster")
public RemoveClusterAdminResult removeClusterAdmin(final RemoveClusterAdminRequest request);
/**
* You can use RemoveClusterAdmin to remove a Cluster Admin. You cannot remove the administrator cluster admin account.
**/
@Since("1.0")
@ConnectionType("Cluster")
public RemoveClusterAdminResult removeClusterAdmin(
Long clusterAdminID
);
/**
* You can use the SetLoginBanner method to set the active Terms of Use banner users see when they log on to the web interface.
**/
@Since("10.0")
@ConnectionType("Cluster")
public SetLoginBannerResult setLoginBanner(final SetLoginBannerRequest request);
/**
* You can use the SetLoginBanner method to set the active Terms of Use banner users see when they log on to the web interface.
**/
@Since("10.0")
@ConnectionType("Cluster")
public SetLoginBannerResult setLoginBanner();
/**
* You can use the SetLoginBanner method to set the active Terms of Use banner users see when they log on to the web interface.
**/
@Since("10.0")
@ConnectionType("Cluster")
public SetLoginBannerResult setLoginBanner(
Optional banner,
Optional enabled
);
/**
* AddDrives enables you to add one or more available drives to the cluster, enabling the drives to host a portion of the cluster's data.
* When you add a node to the cluster or install new drives in an existing node, the new drives are marked as "available" and must be
* added via AddDrives before they can be utilized. Use the ListDrives method to display drives that are "available" to be added. When
* you add multiple drives, it is more efficient to add them in a single AddDrives method call rather than multiple individual methods
* with a single drive each. This reduces the amount of data balancing that must occur to stabilize the storage load on the cluster.
* When you add a drive, the system automatically determines the "type" of drive it should be.
* The method is asynchronous and returns immediately. However, it can take some time for the data in the cluster to be rebalanced
* using the newly added drives. As the new drives are syncing on the system, you can use the ListSyncJobs method to see how the
* drives are being rebalanced and the progress of adding the new drive. You can also use the GetAsyncResult method to query the
* method's returned asyncHandle.
**/
@Since("1.0")
@ConnectionType("Cluster")
public AddDrivesResult addDrives(final AddDrivesRequest request);
/**
* AddDrives enables you to add one or more available drives to the cluster, enabling the drives to host a portion of the cluster's data.
* When you add a node to the cluster or install new drives in an existing node, the new drives are marked as "available" and must be
* added via AddDrives before they can be utilized. Use the ListDrives method to display drives that are "available" to be added. When
* you add multiple drives, it is more efficient to add them in a single AddDrives method call rather than multiple individual methods
* with a single drive each. This reduces the amount of data balancing that must occur to stabilize the storage load on the cluster.
* When you add a drive, the system automatically determines the "type" of drive it should be.
* The method is asynchronous and returns immediately. However, it can take some time for the data in the cluster to be rebalanced
* using the newly added drives. As the new drives are syncing on the system, you can use the ListSyncJobs method to see how the
* drives are being rebalanced and the progress of adding the new drive. You can also use the GetAsyncResult method to query the
* method's returned asyncHandle.
**/
@Since("1.0")
@ConnectionType("Cluster")
public AddDrivesResult addDrives(
NewDrive[] drives,
Optional forceDuringUpgrade
);
/**
* AddDrives enables you to add one or more available drives to the cluster, enabling the drives to host a portion of the cluster's data.
* When you add a node to the cluster or install new drives in an existing node, the new drives are marked as "available" and must be
* added via AddDrives before they can be utilized. Use the ListDrives method to display drives that are "available" to be added. When
* you add multiple drives, it is more efficient to add them in a single AddDrives method call rather than multiple individual methods
* with a single drive each. This reduces the amount of data balancing that must occur to stabilize the storage load on the cluster.
* When you add a drive, the system automatically determines the "type" of drive it should be.
* The method is asynchronous and returns immediately. However, it can take some time for the data in the cluster to be rebalanced
* using the newly added drives. As the new drives are syncing on the system, you can use the ListSyncJobs method to see how the
* drives are being rebalanced and the progress of adding the new drive. You can also use the GetAsyncResult method to query the
* method's returned asyncHandle.
**/
@Since("1.0")
@ConnectionType("Cluster")
public AddDrivesResult addDrives(
NewDrive[] drives,
Optional forceDuringUpgrade,
Optional forceDuringBinSync
);
/**
* GetDriveConfig enables you to display drive information for expected slice and block drive counts as well as the number of slices
* and block drives that are currently connected to the node.
* Note: This method is available only through the per-node API endpoint 5.0 or later.
**/
@Since("2.0")
@ConnectionType("Node")
public GetDriveConfigResult getDriveConfig();
/**
* GetDriveHardwareInfo returns all the hardware information for the given drive. This generally includes details about manufacturers, vendors, versions, and
* other associated hardware identification information.
**/
@Since("1.0")
@ConnectionType("Cluster")
public GetDriveHardwareInfoResult getDriveHardwareInfo(final GetDriveHardwareInfoRequest request);
/**
* GetDriveHardwareInfo returns all the hardware information for the given drive. This generally includes details about manufacturers, vendors, versions, and
* other associated hardware identification information.
**/
@Since("1.0")
@ConnectionType("Cluster")
public GetDriveHardwareInfoResult getDriveHardwareInfo(
Long driveID
);
/**
* GetDriveStats returns high-level activity measurements for a single drive. Values are cumulative from the addition of the drive to the
* cluster. Some values are specific to block drives. You might not obtain statistical data for both block and metadata drives when you
* run this method.
**/
@Since("1.0")
@ConnectionType("Cluster")
public GetDriveStatsResult getDriveStats(final GetDriveStatsRequest request);
/**
* GetDriveStats returns high-level activity measurements for a single drive. Values are cumulative from the addition of the drive to the
* cluster. Some values are specific to block drives. You might not obtain statistical data for both block and metadata drives when you
* run this method.
**/
@Since("1.0")
@ConnectionType("Cluster")
public GetDriveStatsResult getDriveStats(
Long driveID
);
/**
* ListDriveHardware returns all the drives connected to a node. Use this method on individual nodes to return drive hardware
* information or use this method on the cluster master node MVIP to see information for all the drives on all nodes.
* Note: The "securitySupported": true line of the method response does not imply that the drives are capable of
* encryption; only that the security status can be queried. If you have a node type with a model number ending in "-NE",
* commands to enable security features on these drives will fail. See the EnableEncryptionAtRest method for more information.
**/
@Since("7.0")
@ConnectionType("Cluster")
public ListDriveHardwareResult listDriveHardware(final ListDriveHardwareRequest request);
/**
* ListDriveHardware returns all the drives connected to a node. Use this method on individual nodes to return drive hardware
* information or use this method on the cluster master node MVIP to see information for all the drives on all nodes.
* Note: The "securitySupported": true line of the method response does not imply that the drives are capable of
* encryption; only that the security status can be queried. If you have a node type with a model number ending in "-NE",
* commands to enable security features on these drives will fail. See the EnableEncryptionAtRest method for more information.
**/
@Since("7.0")
@ConnectionType("Cluster")
public ListDriveHardwareResult listDriveHardware(
Boolean force
);
/**
* ListDriveStats enables you to retrieve high-level activity measurements for multiple drives in the cluster. By default, this method returns statistics for all drives in the cluster, and these measurements are cumulative from the addition of the drive to the cluster. Some values this method returns are specific to block drives, and some are specific to metadata drives.
**/
@Since("9.0")
@ConnectionType("Cluster")
public ListDriveStatsResult listDriveStats(final ListDriveStatsRequest request);
/**
* ListDriveStats enables you to retrieve high-level activity measurements for multiple drives in the cluster. By default, this method returns statistics for all drives in the cluster, and these measurements are cumulative from the addition of the drive to the cluster. Some values this method returns are specific to block drives, and some are specific to metadata drives.
**/
@Since("9.0")
@ConnectionType("Cluster")
public ListDriveStatsResult listDriveStats(
Optional drives
);
/**
* ListDrives enables you to retrieve the list of the drives that exist in the cluster's active nodes. This method returns drives that have
* been added as volume metadata or block drives as well as drives that have not been added and are available.
**/
@Since("1.0")
@ConnectionType("Cluster")
public ListDrivesResult listDrives();
/**
* You can use RemoveDrives to proactively remove drives that are part of the cluster. You might want to use this method when
* reducing cluster capacity or preparing to replace drives nearing the end of their service life. Any data on the drives is removed and
* migrated to other drives in the cluster before the drive is removed from the cluster. This is an asynchronous method. Depending on
* the total capacity of the drives being removed, it might take several minutes to migrate all of the data. Use the GetAsyncResult
* method to check the status of the remove operation.
* When removing multiple drives, use a single RemoveDrives method call rather than multiple individual methods with a single drive
* each. This reduces the amount of data balancing that must occur to even stabilize the storage load on the cluster.
* You can also remove drives with a "failed" status using RemoveDrives. When you remove a drive with a "failed" status it is not
* returned to an "available" or active status. The drive is unavailable for use in the cluster.
* Use the ListDrives method to obtain the driveIDs for the drives you want to remove.
**/
@Since("1.0")
@ConnectionType("Cluster")
public AsyncHandleResult removeDrives(final RemoveDrivesRequest request);
/**
* You can use RemoveDrives to proactively remove drives that are part of the cluster. You might want to use this method when
* reducing cluster capacity or preparing to replace drives nearing the end of their service life. Any data on the drives is removed and
* migrated to other drives in the cluster before the drive is removed from the cluster. This is an asynchronous method. Depending on
* the total capacity of the drives being removed, it might take several minutes to migrate all of the data. Use the GetAsyncResult
* method to check the status of the remove operation.
* When removing multiple drives, use a single RemoveDrives method call rather than multiple individual methods with a single drive
* each. This reduces the amount of data balancing that must occur to even stabilize the storage load on the cluster.
* You can also remove drives with a "failed" status using RemoveDrives. When you remove a drive with a "failed" status it is not
* returned to an "available" or active status. The drive is unavailable for use in the cluster.
* Use the ListDrives method to obtain the driveIDs for the drives you want to remove.
**/
@Since("1.0")
@ConnectionType("Cluster")
public AsyncHandleResult removeDrives(
Long[] drives,
Optional forceDuringUpgrade
);
/**
* ResetDrives enables you to proactively initialize drives and remove all data currently residing on a drive. The drive can then be reused
* in an existing node or used in an upgraded node. This method requires the force parameter to be included in the method call.
**/
@Since("6.0")
@ConnectionType("Node")
public ResetDrivesResult resetDrives(final ResetDrivesRequest request);
/**
* ResetDrives enables you to proactively initialize drives and remove all data currently residing on a drive. The drive can then be reused
* in an existing node or used in an upgraded node. This method requires the force parameter to be included in the method call.
**/
@Since("6.0")
@ConnectionType("Node")
public ResetDrivesResult resetDrives(
String drives,
Boolean force
);
/**
* SecureEraseDrives enables you to remove any residual data from drives that have a status of "available." You might want to use this method when replacing a drive nearing the end of its service life that contained sensitive data. This method uses a Security Erase Unit command to write a predetermined pattern to the drive and resets the encryption key on the drive. This asynchronous method might take up to two minutes to complete. You can use GetAsyncResult to check on the status of the secure erase operation.
* You can use the ListDrives method to obtain the driveIDs for the drives you want to secure erase.
**/
@Since("5.0")
@ConnectionType("Cluster")
public AsyncHandleResult secureEraseDrives(final SecureEraseDrivesRequest request);
/**
* SecureEraseDrives enables you to remove any residual data from drives that have a status of "available." You might want to use this method when replacing a drive nearing the end of its service life that contained sensitive data. This method uses a Security Erase Unit command to write a predetermined pattern to the drive and resets the encryption key on the drive. This asynchronous method might take up to two minutes to complete. You can use GetAsyncResult to check on the status of the secure erase operation.
* You can use the ListDrives method to obtain the driveIDs for the drives you want to secure erase.
**/
@Since("5.0")
@ConnectionType("Cluster")
public AsyncHandleResult secureEraseDrives(
Long[] drives
);
/**
* You can use the TestDrives API method to run a hardware validation on all drives on the node. This method detects hardware
* failures on the drives (if present) and reports them in the results of the validation tests.
* You can only use the TestDrives method on nodes that are not "active" in a cluster.
* Note: This test takes approximately 10 minutes.
* Note: This method is available only through the per-node API endpoint 5.0 or later.
**/
@Since("5.0")
@ConnectionType("Node")
public TestDrivesResult testDrives(final TestDrivesRequest request);
/**
* You can use the TestDrives API method to run a hardware validation on all drives on the node. This method detects hardware
* failures on the drives (if present) and reports them in the results of the validation tests.
* You can only use the TestDrives method on nodes that are not "active" in a cluster.
* Note: This test takes approximately 10 minutes.
* Note: This method is available only through the per-node API endpoint 5.0 or later.
**/
@Since("5.0")
@ConnectionType("Node")
public TestDrivesResult testDrives(
Optional minutes,
Optional force
);
/**
* You can use the GetClusterHardwareInfo method to retrieve the hardware status and information for all Fibre Channel nodes, iSCSI
* nodes and drives in the cluster. This generally includes details about manufacturers, vendors, versions, and other associated hardware
* identification information.
**/
@Since("1.0")
@ConnectionType("Cluster")
public GetClusterHardwareInfoResult getClusterHardwareInfo(final GetClusterHardwareInfoRequest request);
/**
* You can use the GetClusterHardwareInfo method to retrieve the hardware status and information for all Fibre Channel nodes, iSCSI
* nodes and drives in the cluster. This generally includes details about manufacturers, vendors, versions, and other associated hardware
* identification information.
**/
@Since("1.0")
@ConnectionType("Cluster")
public GetClusterHardwareInfoResult getClusterHardwareInfo(
Optional type
);
/**
* GetHardwareConfig enables you to display the hardware configuration information for a node.
* Note: This method is available only through the per-node API endpoint 5.0 or later.
**/
@Since("5.0")
@ConnectionType("Node")
public GetHardwareConfigResult getHardwareConfig();
/**
* The GetHardwareInfo API method enables you to return hardware information and status for a single node. This generally includes details about manufacturers, vendors, versions, drives, and other associated hardware identification information.
**/
@Since("9.0")
@ConnectionType("Node")
public GetHardwareInfoResult getHardwareInfo();
/**
* GetNodeHardwareInfo enables you to return all the hardware information and status for the node specified. This generally includes details about
* manufacturers, vendors, versions, and other associated hardware identification information.
**/
@Since("1.0")
@ConnectionType("Cluster")
public GetNodeHardwareInfoResult getNodeHardwareInfo(final GetNodeHardwareInfoRequest request);
/**
* GetNodeHardwareInfo enables you to return all the hardware information and status for the node specified. This generally includes details about
* manufacturers, vendors, versions, and other associated hardware identification information.
**/
@Since("1.0")
@ConnectionType("Cluster")
public GetNodeHardwareInfoResult getNodeHardwareInfo(
Long nodeID
);
/**
* GetNvramInfo enables you to retrieve information from each node about the NVRAM card.
**/
@Since("5.0")
@ConnectionType("Node")
public GetNvramInfoResult getNvramInfo(final GetNvramInfoRequest request);
/**
* GetNvramInfo enables you to retrieve information from each node about the NVRAM card.
**/
@Since("5.0")
@ConnectionType("Node")
public GetNvramInfoResult getNvramInfo(
Optional force
);
/**
* AddInitiatorsToVolumeAccessGroup enables you
* to add initiators to a specified volume access group.
**/
@Since("5.0")
@ConnectionType("Cluster")
public ModifyVolumeAccessGroupResult addInitiatorsToVolumeAccessGroup(final AddInitiatorsToVolumeAccessGroupRequest request);
/**
* AddInitiatorsToVolumeAccessGroup enables you
* to add initiators to a specified volume access group.
**/
@Since("5.0")
@ConnectionType("Cluster")
public ModifyVolumeAccessGroupResult addInitiatorsToVolumeAccessGroup(
Long volumeAccessGroupID,
String[] initiators
);
/**
* CreateInitiators enables you to create multiple new initiator IQNs or World Wide Port Names (WWPNs) and optionally assign them
* aliases and attributes. When you use CreateInitiators to create new initiators, you can also add them to volume access groups.
* If CreateInitiators fails to create one of the initiators provided in the parameter, the method returns an error and does not create
* any initiators (no partial completion is possible).
**/
@Since("9.0")
@ConnectionType("Cluster")
public CreateInitiatorsResult createInitiators(final CreateInitiatorsRequest request);
/**
* CreateInitiators enables you to create multiple new initiator IQNs or World Wide Port Names (WWPNs) and optionally assign them
* aliases and attributes. When you use CreateInitiators to create new initiators, you can also add them to volume access groups.
* If CreateInitiators fails to create one of the initiators provided in the parameter, the method returns an error and does not create
* any initiators (no partial completion is possible).
**/
@Since("9.0")
@ConnectionType("Cluster")
public CreateInitiatorsResult createInitiators(
CreateInitiator[] initiators
);
/**
* DeleteInitiators enables you to delete one or more initiators from the system (and from any associated volumes or volume access
* groups).
* If DeleteInitiators fails to delete one of the initiators provided in the parameter, the system returns an error and does not delete any
* initiators (no partial completion is possible).
**/
@Since("9.0")
@ConnectionType("Cluster")
public DeleteInitiatorsResult deleteInitiators(final DeleteInitiatorsRequest request);
/**
* DeleteInitiators enables you to delete one or more initiators from the system (and from any associated volumes or volume access
* groups).
* If DeleteInitiators fails to delete one of the initiators provided in the parameter, the system returns an error and does not delete any
* initiators (no partial completion is possible).
**/
@Since("9.0")
@ConnectionType("Cluster")
public DeleteInitiatorsResult deleteInitiators(
Long[] initiators
);
/**
* ListInitiators enables you to list initiator IQNs or World Wide Port Names (WWPNs).
**/
@Since("9.0")
@ConnectionType("Cluster")
public ListInitiatorsResult listInitiators(final ListInitiatorsRequest request);
/**
* ListInitiators enables you to list initiator IQNs or World Wide Port Names (WWPNs).
**/
@Since("9.0")
@ConnectionType("Cluster")
public ListInitiatorsResult listInitiators(
Optional startInitiatorID,
Optional limit,
Optional initiators
);
/**
* ModifyInitiators enables you to change the attributes of one or more existing initiators. You cannot change the name of an existing
* initiator. If you need to change the name of an initiator, delete it first with DeleteInitiators and create a new one with
* CreateInitiators.
* If ModifyInitiators fails to change one of the initiators provided in the parameter, the method returns an error and does not modify
* any initiators (no partial completion is possible).
**/
@Since("9.0")
@ConnectionType("Cluster")
public ModifyInitiatorsResult modifyInitiators(final ModifyInitiatorsRequest request);
/**
* ModifyInitiators enables you to change the attributes of one or more existing initiators. You cannot change the name of an existing
* initiator. If you need to change the name of an initiator, delete it first with DeleteInitiators and create a new one with
* CreateInitiators.
* If ModifyInitiators fails to change one of the initiators provided in the parameter, the method returns an error and does not modify
* any initiators (no partial completion is possible).
**/
@Since("9.0")
@ConnectionType("Cluster")
public ModifyInitiatorsResult modifyInitiators(
ModifyInitiator[] initiators
);
/**
* RemoveInitiatorsFromVolumeAccessGroup enables
* you to remove initiators from a specified volume access
* group.
**/
@Since("5.0")
@ConnectionType("Cluster")
public ModifyVolumeAccessGroupResult removeInitiatorsFromVolumeAccessGroup(final RemoveInitiatorsFromVolumeAccessGroupRequest request);
/**
* RemoveInitiatorsFromVolumeAccessGroup enables
* you to remove initiators from a specified volume access
* group.
**/
@Since("5.0")
@ConnectionType("Cluster")
public ModifyVolumeAccessGroupResult removeInitiatorsFromVolumeAccessGroup(
Long volumeAccessGroupID,
String[] initiators,
Optional deleteOrphanInitiators
);
/**
* AddLdapClusterAdmin enables you to add a new LDAP cluster administrator user. An LDAP cluster administrator can manage the
* cluster via the API and management tools. LDAP cluster admin accounts are completely separate and unrelated to standard tenant
* accounts.
* You can also use this method to add an LDAP group that has been defined in Active Directory. The access level that is given to the group is passed to the individual users in the LDAP group.
**/
@Since("8.0")
@ConnectionType("Cluster")
public AddLdapClusterAdminResult addLdapClusterAdmin(final AddLdapClusterAdminRequest request);
/**
* AddLdapClusterAdmin enables you to add a new LDAP cluster administrator user. An LDAP cluster administrator can manage the
* cluster via the API and management tools. LDAP cluster admin accounts are completely separate and unrelated to standard tenant
* accounts.
* You can also use this method to add an LDAP group that has been defined in Active Directory. The access level that is given to the group is passed to the individual users in the LDAP group.
**/
@Since("8.0")
@ConnectionType("Cluster")
public AddLdapClusterAdminResult addLdapClusterAdmin(
String username,
String[] access,
Optional acceptEula,
Optional attributes
);
/**
* The DisableLdapAuthentication method enables you to disable LDAP authentication and remove all LDAP configuration settings. This method does not remove any configured cluster admin accounts (user or group). However, those cluster admin accounts will no longer be able to log in.
**/
@Since("7.0")
@ConnectionType("Cluster")
public DisableLdapAuthenticationResult disableLdapAuthentication();
/**
* The EnableLdapAuthentication method enables you to configure an LDAP directory connection to use for LDAP authentication to a cluster. Users that are members of the LDAP directory can then log in to the storage system using their LDAP credentials.
**/
@Since("7.0")
@ConnectionType("Cluster")
public EnableLdapAuthenticationResult enableLdapAuthentication(final EnableLdapAuthenticationRequest request);
/**
* The EnableLdapAuthentication method enables you to configure an LDAP directory connection to use for LDAP authentication to a cluster. Users that are members of the LDAP directory can then log in to the storage system using their LDAP credentials.
**/
@Since("7.0")
@ConnectionType("Cluster")
public EnableLdapAuthenticationResult enableLdapAuthentication(
Optional authType,
Optional groupSearchBaseDN,
Optional groupSearchCustomFilter,
Optional groupSearchType,
Optional searchBindDN,
Optional searchBindPassword,
String[] serverURIs,
Optional userDNTemplate,
Optional userSearchBaseDN,
Optional userSearchFilter
);
/**
* The GetLdapConfiguration method enables you to get the currently active LDAP configuration on the cluster.
**/
@Since("7.0")
@ConnectionType("Cluster")
public GetLdapConfigurationResult getLdapConfiguration();
/**
* The TestLdapAuthentication method enables you to validate the currently enabled LDAP authentication settings. If the configuration is
* correct, the API call returns the group membership of the tested user.
**/
@Since("7.0")
@ConnectionType("Cluster")
public TestLdapAuthenticationResult testLdapAuthentication(final TestLdapAuthenticationRequest request);
/**
* The TestLdapAuthentication method enables you to validate the currently enabled LDAP authentication settings. If the configuration is
* correct, the API call returns the group membership of the tested user.
**/
@Since("7.0")
@ConnectionType("Cluster")
public TestLdapAuthenticationResult testLdapAuthentication(
String username,
String password,
Optional ldapConfiguration
);
/**
* GetLoginSessionInfo enables you to return the period of time a log in authentication session is valid for both log in shells and the TUI.
**/
@Since("7.0")
@ConnectionType("Cluster")
public GetLoginSessionInfoResult getLoginSessionInfo();
/**
* GetRemoteLoggingHosts enables you to retrieve the current list of log servers.
**/
@Since("1.0")
@ConnectionType("Cluster")
public GetRemoteLoggingHostsResult getRemoteLoggingHosts();
/**
* You can use SetLoginSessionInfo to set the period of time that a session's login authentication is valid. After the log in period elapses without activity on the system, the authentication expires. New login credentials are required for continued access to the cluster after the timeout period has elapsed.
**/
@Since("7.0")
@ConnectionType("Cluster")
public SetLoginSessionInfoResult setLoginSessionInfo(final SetLoginSessionInfoRequest request);
/**
* You can use SetLoginSessionInfo to set the period of time that a session's login authentication is valid. After the log in period elapses without activity on the system, the authentication expires. New login credentials are required for continued access to the cluster after the timeout period has elapsed.
**/
@Since("7.0")
@ConnectionType("Cluster")
public SetLoginSessionInfoResult setLoginSessionInfo(
String timeout
);
/**
* SetRemoteLoggingHosts enables you to configure remote logging from the nodes in the storage cluster to a centralized log server or servers. Remote logging is performed over TCP using the default port 514. This API does not add to the existing logging hosts. Rather, it replaces what currently exists with new values specified by this API method. You can use GetRemoteLoggingHosts to determine what the current logging hosts are, and then use SetRemoteLoggingHosts to set the desired list of current and new logging hosts.
**/
@Since("1.0")
@ConnectionType("Cluster")
public SetRemoteLoggingHostsResult setRemoteLoggingHosts(final SetRemoteLoggingHostsRequest request);
/**
* SetRemoteLoggingHosts enables you to configure remote logging from the nodes in the storage cluster to a centralized log server or servers. Remote logging is performed over TCP using the default port 514. This API does not add to the existing logging hosts. Rather, it replaces what currently exists with new values specified by this API method. You can use GetRemoteLoggingHosts to determine what the current logging hosts are, and then use SetRemoteLoggingHosts to set the desired list of current and new logging hosts.
**/
@Since("1.0")
@ConnectionType("Cluster")
public SetRemoteLoggingHostsResult setRemoteLoggingHosts(
LoggingServer[] remoteHosts
);
/**
* ListFibreChannelPortInfo enables you to retrieve information about the Fibre Channel ports on a node. The API method is intended for use on individual nodes; userid and password authentication is required for access to individual Fibre Channel nodes.
**/
@Since("8.0")
@ConnectionType("Cluster")
public ListFibreChannelPortInfoResult listFibreChannelPortInfo();
/**
* ListFibreChannelSessions enables you to retrieve information about the active Fibre Channel sessions on a cluster.
**/
@Since("7.0")
@ConnectionType("Cluster")
public ListFibreChannelSessionsResult listFibreChannelSessions();
/**
* You can use ListISCSISessions to return iSCSI information for volumes in the cluster.
**/
@Since("1.0")
@ConnectionType("Cluster")
public ListISCSISessionsResult listISCSISessions();
/**
* ListNetworkInterfaces enables you to retrieve information about each network interface on a node. The API method is intended for use on individual nodes; userid and password authentication is required for access to individual nodes.
**/
@Since("7.0")
@ConnectionType("Node")
public ListNetworkInterfacesResult listNetworkInterfaces();
/**
* The ListNodeFibreChannelPortInfo API method enables you to retrieve information about the Fibre Channel ports on a node. The API method is intended for use on individual nodes; userid and password authentication is required for access to individual Fibre Channel nodes.
**/
@Since("7.0")
@ConnectionType("Node")
public ListNodeFibreChannelPortInfoResult listNodeFibreChannelPortInfo();
/**
* AddNodes enables you to add one or more new nodes to a cluster. When a node that is not configured starts up for the first time, you are prompted to configure the node. After you configure the node, it is registered as a "pending node" with the cluster.
* Note: It might take several seconds after adding a new node for it to start up and register its drives as available.
**/
@Since("1.0")
@ConnectionType("Cluster")
public AddNodesResult addNodes(final AddNodesRequest request);
/**
* AddNodes enables you to add one or more new nodes to a cluster. When a node that is not configured starts up for the first time, you are prompted to configure the node. After you configure the node, it is registered as a "pending node" with the cluster.
* Note: It might take several seconds after adding a new node for it to start up and register its drives as available.
**/
@Since("1.0")
@ConnectionType("Cluster")
public AddNodesResult addNodes(
Long[] pendingNodes,
Optional autoInstall
);
/**
* GetBootstrapConfig returns cluster and node information from the bootstrap configuration file. Use this API method on an individual node before it has been joined with a cluster. You can use the information this method returns in the cluster configuration interface when you create a cluster.
**/
@Since("2.0")
@ConnectionType("Both")
public GetBootstrapConfigResult getBootstrapConfig();
/**
* The GetConfig API method enables you to retrieve all configuration information for a node. This method includes the same information available in both the GetClusterConfig and GetNetworkConfig API methods.
* Note: This method is available only through the per-node API endpoint 5.0 or later.
**/
@Since("5.0")
@ConnectionType("Node")
public GetConfigResult getConfig();
/**
* The GetNetworkConfig API method enables you to display the network configuration information for a node.
* Note: This method is available only through the per-node API endpoint 5.0 or later.
**/
@Since("5.0")
@ConnectionType("Node")
public GetNetworkConfigResult getNetworkConfig();
/**
* You can use the GetNodeSSLCertificate method to retrieve the SSL certificate that is currently active on the cluster.
* You can use this method on both management and storage nodes.
**/
@Since("10.0")
@ConnectionType("Node")
public GetNodeSSLCertificateResult getNodeSSLCertificate();
/**
* GetNodeStats enables you to retrieve the high-level activity measurements for a single node.
**/
@Since("1.0")
@ConnectionType("Cluster")
public GetNodeStatsResult getNodeStats(final GetNodeStatsRequest request);
/**
* GetNodeStats enables you to retrieve the high-level activity measurements for a single node.
**/
@Since("1.0")
@ConnectionType("Cluster")
public GetNodeStatsResult getNodeStats(
Long nodeID
);
/**
* GetOrigin enables you to retrieve the origination certificate for where the node was built. This method might return null if there is no origination certification.
**/
@Since("9.0")
@ConnectionType("Cluster")
public GetOriginResult getOrigin();
/**
* You can use GetPendingOperation to detect an operation on a node that is currently in progress. You can also use this method to report back when an operation has completed.
* Note: method is available only through the per-node API endpoint 5.0 or later.
**/
@Since("5.0")
@ConnectionType("Node")
public GetPendingOperationResult getPendingOperation();
/**
* ListActiveNodes returns the list of currently active nodes that are in the cluster.
**/
@Since("1.0")
@ConnectionType("Cluster")
public ListActiveNodesResult listActiveNodes();
/**
* ListAllNodes enables you to retrieve a list of active and pending nodes in the cluster.
**/
@Since("1.0")
@ConnectionType("Cluster")
public ListAllNodesResult listAllNodes();
/**
* ListNodeStats enables you to view the high-level activity measurements for all nodes in a cluster.
**/
@Since("6.0")
@ConnectionType("Cluster")
public ListNodeStatsResult listNodeStats();
/**
* ListPendingActiveNodes returns the list of nodes in the cluster that are currently in the PendingActive state, between the pending and active states. These are nodes that are currently being returned to the factory image.
**/
@Since("9.0")
@ConnectionType("Cluster")
public ListPendingActiveNodesResult listPendingActiveNodes();
/**
* ListPendingNodes returns a list of the currently pending nodes in the system. Pending nodes are nodes that are running and configured to join the cluster, but have not yet been added via the AddNodes API method.
**/
@Since("1.0")
@ConnectionType("Cluster")
public ListPendingNodesResult listPendingNodes();
/**
* You can use the RemoveNodeSSLCertificate method to remove the user SSL certificate and private key for the management node.
* After the certificate and private key are removed, the management node is configured to use the default certificate and private key..
**/
@Since("10.0")
@ConnectionType("Node")
public RemoveNodeSSLCertificateResult removeNodeSSLCertificate();
/**
* You can use RemoveNodes to remove one or more nodes that should no longer participate in the cluster. Before removing a node, you must remove all drives the node contains using the RemoveDrives method. You cannot remove a node until the RemoveDrives process has completed and all data has been migrated away from the node.
* After you remove a node, it registers itself as a pending node. You can add the node again or shut it down (shutting the node down removes it from the Pending Node list).
**/
@Since("1.0")
@ConnectionType("Cluster")
public RemoveNodesResult removeNodes(final RemoveNodesRequest request);
/**
* You can use RemoveNodes to remove one or more nodes that should no longer participate in the cluster. Before removing a node, you must remove all drives the node contains using the RemoveDrives method. You cannot remove a node until the RemoveDrives process has completed and all data has been migrated away from the node.
* After you remove a node, it registers itself as a pending node. You can add the node again or shut it down (shutting the node down removes it from the Pending Node list).
**/
@Since("1.0")
@ConnectionType("Cluster")
public RemoveNodesResult removeNodes(
Long[] nodes
);
/**
* The SetConfig API method enables you to set all the configuration information for the node. This includes the same information available via calls to SetClusterConfig and SetNetworkConfig in one API method.
* Note: This method is available only through the per-node API endpoint 5.0 or later.
* Caution: Changing the "bond-mode" on a node can cause a temporary loss of network connectivity. Exercise caution when using this method.
**/
@Since("5.0")
@ConnectionType("Node")
public SetConfigResult setConfig(final SetConfigRequest request);
/**
* The SetConfig API method enables you to set all the configuration information for the node. This includes the same information available via calls to SetClusterConfig and SetNetworkConfig in one API method.
* Note: This method is available only through the per-node API endpoint 5.0 or later.
* Caution: Changing the "bond-mode" on a node can cause a temporary loss of network connectivity. Exercise caution when using this method.
**/
@Since("5.0")
@ConnectionType("Node")
public SetConfigResult setConfig(
ConfigParams config
);
/**
* The SetNetworkConfig API method enables you to set the network configuration for a node. To display the current network settings for a node, run the GetNetworkConfig API method.
* Note: This method is available only through the per-node API endpoint 5.0 or later.
* Changing the "bond-mode" on a node can cause a temporary loss of network connectivity. Exercise caution when using this method.
**/
@Since("5.0")
@ConnectionType("Node")
public SetNetworkConfigResult setNetworkConfig(final SetNetworkConfigRequest request);
/**
* The SetNetworkConfig API method enables you to set the network configuration for a node. To display the current network settings for a node, run the GetNetworkConfig API method.
* Note: This method is available only through the per-node API endpoint 5.0 or later.
* Changing the "bond-mode" on a node can cause a temporary loss of network connectivity. Exercise caution when using this method.
**/
@Since("5.0")
@ConnectionType("Node")
public SetNetworkConfigResult setNetworkConfig(
NetworkParams network
);
/**
* You can use the SetNodeSSLCertificate method to set a user SSL certificate and private key for the management node.
**/
@Since("10.0")
@ConnectionType("Node")
public SetNodeSSLCertificateResult setNodeSSLCertificate(final SetNodeSSLCertificateRequest request);
/**
* You can use the SetNodeSSLCertificate method to set a user SSL certificate and private key for the management node.
**/
@Since("10.0")
@ConnectionType("Node")
public SetNodeSSLCertificateResult setNodeSSLCertificate(
String certificate,
String privateKey
);
/**
* You can use the CompleteClusterPairing method with the encoded key received from the StartClusterPairing method to complete the cluster pairing process. The CompleteClusterPairing method is the second step in the cluster pairing process.
**/
@Since("6.0")
@ConnectionType("Cluster")
public CompleteClusterPairingResult completeClusterPairing(final CompleteClusterPairingRequest request);
/**
* You can use the CompleteClusterPairing method with the encoded key received from the StartClusterPairing method to complete the cluster pairing process. The CompleteClusterPairing method is the second step in the cluster pairing process.
**/
@Since("6.0")
@ConnectionType("Cluster")
public CompleteClusterPairingResult completeClusterPairing(
String clusterPairingKey
);
/**
* You can use the CompleteVolumePairing method to complete the pairing of two volumes.
**/
@Since("6.0")
@ConnectionType("Cluster")
public CompleteVolumePairingResult completeVolumePairing(final CompleteVolumePairingRequest request);
/**
* You can use the CompleteVolumePairing method to complete the pairing of two volumes.
**/
@Since("6.0")
@ConnectionType("Cluster")
public CompleteVolumePairingResult completeVolumePairing(
String volumePairingKey,
Long volumeID
);
/**
* ListActivePairedVolumes enables you to list all the active volumes paired with a volume. This method returns information about volumes with active and pending pairings.
**/
@Since("6.0")
@ConnectionType("Cluster")
public ListActivePairedVolumesResult listActivePairedVolumes(final ListActivePairedVolumesRequest request);
/**
* ListActivePairedVolumes enables you to list all the active volumes paired with a volume. This method returns information about volumes with active and pending pairings.
**/
@Since("6.0")
@ConnectionType("Cluster")
public ListActivePairedVolumesResult listActivePairedVolumes(
Optional startVolumeID,
Optional limit
);
/**
* You can use the ListClusterPairs method to list all the clusters that a cluster is paired with. This method returns information about active and pending cluster pairings, such as statistics about the current pairing as well as the connectivity and latency (in milliseconds) of the cluster pairing.
**/
@Since("6.0")
@ConnectionType("Cluster")
public ListClusterPairsResult listClusterPairs();
/**
* ModifyVolumePair enables you to pause or restart replication between a pair of volumes.
**/
@Since("6.0")
@ConnectionType("Cluster")
public ModifyVolumePairResult modifyVolumePair(final ModifyVolumePairRequest request);
/**
* ModifyVolumePair enables you to pause or restart replication between a pair of volumes.
**/
@Since("6.0")
@ConnectionType("Cluster")
public ModifyVolumePairResult modifyVolumePair(
Long volumeID,
Optional pausedManual,
Optional mode,
Optional pauseLimit
);
/**
* You can use the RemoveClusterPair method to close the open connections between two paired clusters.
* Note: Before you remove a cluster pair, you must first remove all volume pairing to the clusters with the "RemoveVolumePair" API method.
**/
@Since("6.0")
@ConnectionType("Cluster")
public RemoveClusterPairResult removeClusterPair(final RemoveClusterPairRequest request);
/**
* You can use the RemoveClusterPair method to close the open connections between two paired clusters.
* Note: Before you remove a cluster pair, you must first remove all volume pairing to the clusters with the "RemoveVolumePair" API method.
**/
@Since("6.0")
@ConnectionType("Cluster")
public RemoveClusterPairResult removeClusterPair(
Long clusterPairID
);
/**
* RemoveVolumePair enables you to remove the remote pairing between two volumes. Use this method on both the source and target volumes that are paired together. When you remove the volume pairing information, data is no longer replicated to or from the volume.
**/
@Since("6.0")
@ConnectionType("Cluster")
public RemoveVolumePairResult removeVolumePair(final RemoveVolumePairRequest request);
/**
* RemoveVolumePair enables you to remove the remote pairing between two volumes. Use this method on both the source and target volumes that are paired together. When you remove the volume pairing information, data is no longer replicated to or from the volume.
**/
@Since("6.0")
@ConnectionType("Cluster")
public RemoveVolumePairResult removeVolumePair(
Long volumeID
);
/**
* You can use the StartClusterPairing method to create an encoded key from a cluster that is used to pair with another cluster. The key created from this API method is used in the CompleteClusterPairing API method to establish a cluster pairing. You can pair a cluster with a maximum of four other clusters.
**/
@Since("6.0")
@ConnectionType("Cluster")
public StartClusterPairingResult startClusterPairing();
/**
* StartVolumePairing enables you to create an encoded key from a volume that is used to pair with another volume. The key that this
* method creates is used in the CompleteVolumePairing API method to establish a volume pairing.
**/
@Since("6.0")
@ConnectionType("Cluster")
public StartVolumePairingResult startVolumePairing(final StartVolumePairingRequest request);
/**
* StartVolumePairing enables you to create an encoded key from a volume that is used to pair with another volume. The key that this
* method creates is used in the CompleteVolumePairing API method to establish a volume pairing.
**/
@Since("6.0")
@ConnectionType("Cluster")
public StartVolumePairingResult startVolumePairing(
Long volumeID
);
/**
* StartVolumePairing enables you to create an encoded key from a volume that is used to pair with another volume. The key that this
* method creates is used in the CompleteVolumePairing API method to establish a volume pairing.
**/
@Since("6.0")
@ConnectionType("Cluster")
public StartVolumePairingResult startVolumePairing(
Long volumeID,
Optional mode
);
/**
* The ResetNode API method enables you to reset a node to the factory settings. All data, packages (software upgrades, and so on),
* configurations, and log files are deleted from the node when you call this method. However, network settings for the node are
* preserved during this operation. Nodes that are participating in a cluster cannot be reset to the factory settings.
* The ResetNode API can only be used on nodes that are in an "Available" state. It cannot be used on nodes that are "Active" in a
* cluster, or in a "Pending" state.
* Caution: This method clears any data that is on the node. Exercise caution when using this method.
* Note: This method is available only through the per-node API endpoint 5.0 or later.
**/
@Since("5.0")
@ConnectionType("Node")
public ResetNodeResult resetNode(final ResetNodeRequest request);
/**
* The ResetNode API method enables you to reset a node to the factory settings. All data, packages (software upgrades, and so on),
* configurations, and log files are deleted from the node when you call this method. However, network settings for the node are
* preserved during this operation. Nodes that are participating in a cluster cannot be reset to the factory settings.
* The ResetNode API can only be used on nodes that are in an "Available" state. It cannot be used on nodes that are "Active" in a
* cluster, or in a "Pending" state.
* Caution: This method clears any data that is on the node. Exercise caution when using this method.
* Note: This method is available only through the per-node API endpoint 5.0 or later.
**/
@Since("5.0")
@ConnectionType("Node")
public ResetNodeResult resetNode(
String build,
Boolean force
);
/**
* The ResetNode API method enables you to reset a node to the factory settings. All data, packages (software upgrades, and so on),
* configurations, and log files are deleted from the node when you call this method. However, network settings for the node are
* preserved during this operation. Nodes that are participating in a cluster cannot be reset to the factory settings.
* The ResetNode API can only be used on nodes that are in an "Available" state. It cannot be used on nodes that are "Active" in a
* cluster, or in a "Pending" state.
* Caution: This method clears any data that is on the node. Exercise caution when using this method.
* Note: This method is available only through the per-node API endpoint 5.0 or later.
**/
@Since("5.0")
@ConnectionType("Node")
public ResetNodeResult resetNode(
String build,
Boolean force,
Optional reboot,
Optional options
);
/**
* The RestartNetworking API method enables you to restart the networking services on a node.
* Warning: This method restarts all networking services on a node, causing temporary loss of networking connectivity.
* Exercise caution when using this method.
* Note: This method is available only through the per-node API endpoint 5.0 or later.
**/
@Since("5.0")
@ConnectionType("Node")
public Attributes restartNetworking(final RestartNetworkingRequest request);
/**
* The RestartNetworking API method enables you to restart the networking services on a node.
* Warning: This method restarts all networking services on a node, causing temporary loss of networking connectivity.
* Exercise caution when using this method.
* Note: This method is available only through the per-node API endpoint 5.0 or later.
**/
@Since("5.0")
@ConnectionType("Node")
public Attributes restartNetworking(
Boolean force
);
/**
* The Shutdown API method enables you to restart or shutdown a node that has not yet been added to a cluster. To use this method,
* log in to the MIP for the pending node, and enter the "shutdown" method with either the "restart" or "halt" options.
**/
@Since("1.0")
@ConnectionType("Cluster")
public ShutdownResult shutdown(final ShutdownRequest request);
/**
* The Shutdown API method enables you to restart or shutdown a node that has not yet been added to a cluster. To use this method,
* log in to the MIP for the pending node, and enter the "shutdown" method with either the "restart" or "halt" options.
**/
@Since("1.0")
@ConnectionType("Cluster")
public ShutdownResult shutdown(
Long[] nodes,
Optional option
);
/**
* This will invoke any API method supported by the SolidFire API for the version and port the connection is using.
* Returns a nested hashtable of key/value pairs that contain the result of the invoked method.
**/
@Since("1.0")
@ConnectionType("Both")
public Attributes invokeSFApi(final InvokeSFApiRequest request);
/**
* This will invoke any API method supported by the SolidFire API for the version and port the connection is using.
* Returns a nested hashtable of key/value pairs that contain the result of the invoked method.
**/
@Since("1.0")
@ConnectionType("Both")
public Attributes invokeSFApi(
String method,
Optional