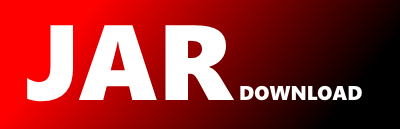
com.somospnt.signature.CreateSignature Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pdfbox-signature-api Show documentation
Show all versions of pdfbox-signature-api Show documentation
This is a libray to digital signing a PDF and a PFX valid certificate.
Based in PDFBox official SVN example.
The newest version!
/*
* Licensed to the Apache Software Foundation (ASF) under one or more
* contributor license agreements. See the NOTICE file distributed with
* this work for additional information regarding copyright ownership.
* The ASF licenses this file to You under the Apache License, Version 2.0
* (the "License"); you may not use this file except in compliance with
* the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package com.somospnt.signature;
import java.io.IOException;
import java.io.InputStream;
import java.io.OutputStream;
import java.security.GeneralSecurityException;
import java.security.KeyStore;
import java.security.KeyStoreException;
import java.security.NoSuchAlgorithmException;
import java.security.UnrecoverableKeyException;
import java.security.cert.CertificateException;
import java.util.Calendar;
import org.apache.pdfbox.pdmodel.PDDocument;
import org.apache.pdfbox.pdmodel.interactive.digitalsignature.ExternalSigningSupport;
import org.apache.pdfbox.pdmodel.interactive.digitalsignature.PDSignature;
/**
* An example for signing a PDF with bouncy castle. A keystore can be created
* with the java keytool, for example:
*
* {@code keytool -genkeypair -storepass 123456 -storetype pkcs12 -alias test -validity 365
* -v -keyalg RSA -keystore keystore.p12 }
*
* @author Thomas Chojecki
* @author Vakhtang Koroghlishvili
* @author John Hewson
* @author SomosPNT
*/
class CreateSignature extends CreateSignatureBase {
private String location;
private String reason;
/**
* Initialize the signature creator with a keystore and certficate password.
*
* @param keystore the pkcs12 keystore containing the signing certificate
* @param pin the password for recovering the key
* @throws KeyStoreException if the keystore has not been initialized
* (loaded)
* @throws NoSuchAlgorithmException if the algorithm for recovering the key
* cannot be found
* @throws UnrecoverableKeyException if the given password is wrong
* @throws CertificateException if the certificate is not valid as signing
* time
* @throws IOException if no certificate could be found
*/
public CreateSignature(KeyStore keystore, char[] pin) throws GeneralSecurityException, IOException {
super(keystore, pin);
}
/**
* Signs the given PDF file. Adds today date to the signature.
*
* @param file the PDF file to sign
* @throws IOException if the file could not be read or written
*/
public void signDetached(InputStream input, OutputStream output) throws IOException {
try (PDDocument document = PDDocument.load(input)) {
int accessPermissions = SigUtils.getMDPPermission(document);
if (accessPermissions == 1) {
throw new IllegalStateException("No changes to the document are permitted due to DocMDP transform parameters dictionary");
}
PDSignature signature = new PDSignature();
signature.setFilter(PDSignature.FILTER_ADOBE_PPKLITE);
signature.setSubFilter(PDSignature.SUBFILTER_ADBE_PKCS7_DETACHED);
signature.setLocation(location);
signature.setReason(reason);
signature.setSignDate(Calendar.getInstance());
if (accessPermissions == 0) {
SigUtils.setMDPPermission(document, signature, 2);
}
document.addSignature(signature);
ExternalSigningSupport externalSigning = document.saveIncrementalForExternalSigning(output);
byte[] cmsSignature = sign(externalSigning.getContent());
externalSigning.setSignature(cmsSignature);
}
}
public void setLocation(String location) {
this.location = location;
}
public void setReason(String reason) {
this.reason = reason;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy