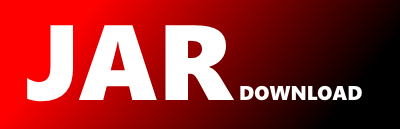
com.somospnt.signature.TimeStamper Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pdfbox-signature-api Show documentation
Show all versions of pdfbox-signature-api Show documentation
This is a libray to digital signing a PDF and a PFX valid certificate.
Based in PDFBox official SVN example.
The newest version!
/*
* Copyright 2015 The Apache Software Foundation.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package com.somospnt.signature;
import java.io.IOException;
import java.io.InputStream;
import java.io.OutputStream;
import java.security.NoSuchAlgorithmException;
import org.apache.commons.logging.Log;
import org.apache.commons.logging.LogFactory;
import org.apache.pdfbox.cos.COSName;
import org.apache.pdfbox.pdmodel.PDDocument;
import org.apache.pdfbox.pdmodel.interactive.digitalsignature.PDSignature;
/**
* A simple wrapper class for timestamping PDF files using PDFBOX Example usage:
*
*
* {@code
* FileInputStream inputStream = new FileInputStream(INPUT_FILE);
* FileOutputStream outputStream = new FileOutputStream(OUTPUT_FILE);
* TimeStamper timeStamper = new TimeStamper(TSA_URL);
* timeStamper.addTimeStamp(inputStream, outputStream);
* timeStamper.embedTimeStamp(inputStream, outputStream);
* }
*
*
* @author SomosPNT
*/
public class TimeStamper {
private static final Log LOG = LogFactory.getLog(TimeStamper.class);
private final String tsaUrl;
private final TimeStamperEmbedder timeStamperEmbedder;
public TimeStamper(String tsaUrl) {
this.tsaUrl = tsaUrl;
this.timeStamperEmbedder = new TimeStamperEmbedder(tsaUrl);
}
/**
* Signs the given PDF file. Adds timeStamp to the signature.
*
* @param inputStream the input stream that contains the PDF file
* @param outputStream the output stream that contains the PDF signed file
* @throws IOException if the file could not be read or signed
*/
public void addTimeStamp(InputStream inputStream, OutputStream outputStream) throws IOException {
PDDocument document = PDDocument.load(inputStream);
int accessPermissions = SigUtils.getMDPPermission(document);
if (accessPermissions == 1) {
throw new IllegalStateException(
"No changes to the document are permitted due to DocMDP transform parameters dictionary");
}
// create signature dictionary
PDSignature signature = new PDSignature();
signature.setType(COSName.DOC_TIME_STAMP);
signature.setFilter(PDSignature.FILTER_ADOBE_PPKLITE);
signature.setSubFilter(COSName.getPDFName("ETSI.RFC3161"));
// No certification allowed because /Reference not allowed in signature directory
// see ETSI EN 319 142-1 Part 1 and ETSI TS 102 778-4
// http://www.etsi.org/deliver/etsi_en%5C319100_319199%5C31914201%5C01.01.00_30%5Cen_31914201v010100v.pdf
// http://www.etsi.org/deliver/etsi_ts/102700_102799/10277804/01.01.01_60/ts_10277804v010101p.pdf
// register signature dictionary and sign interface
document.addSignature(signature, (content) -> {
ValidationTimeStamp validation;
try {
validation = new ValidationTimeStamp(tsaUrl);
return validation.getTimeStampToken(content);
} catch (NoSuchAlgorithmException e) {
LOG.error("Hashing-Algorithm not found for TimeStamping", e);
}
return new byte[]{};
});
// write incremental (only for signing purpose)
document.saveIncremental(outputStream);
}
/**
* Add timeStamp to an existing signature.
*
* @param inputStream the input stream that contains the PDF file
* @param outputStream the output stream that contains the PDF signed file
* @throws IOException if the file could not be read or signed
*/
public void embedTimeStamp(InputStream inputStream, OutputStream outputStream) throws IOException {
timeStamperEmbedder.embedTimeStamp(inputStream, outputStream);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy