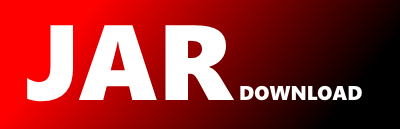
com.soulgalore.velocity.HeaderTool Maven / Gradle / Ivy
package com.soulgalore.velocity;
import org.apache.http.impl.cookie.DateParseException;
import org.apache.http.impl.cookie.DateUtils;
import org.joda.time.DateTime;
import org.joda.time.Seconds;
public class HeaderTool {
public HeaderTool() {
}
public Seconds getSeconds(int seconds) {
return Seconds.seconds(seconds);
}
public Seconds getDelta(String from, String to) {
if (from == null)
return null;
else if (to == null)
to = new DateTime().toString();
DateTime lastModified;
DateTime today;
try {
lastModified = new DateTime(DateUtils.parseDate(from));
today = new DateTime(DateUtils.parseDate(to));
return Seconds.secondsBetween(lastModified, today);
} catch (DateParseException e) {
return null;
}
}
public Seconds getCacheTime(String fullCacheControl, String expires, String now) {
if (fullCacheControl != null && !"".equals(fullCacheControl))
return getMaxAge(fullCacheControl.trim(), expires, now);
else {
if (expires != null)
return getDelta(now.trim(), expires.trim());
else
return Seconds.ZERO;
}
}
public Seconds getMaxAge(String fullCacheControl, String expires, String now) {
if (doMaxAgeExist(fullCacheControl)) {
String firstPart = fullCacheControl.substring(
fullCacheControl.indexOf("max-age=") + 8,
fullCacheControl.length());
String sec = firstPart.indexOf(",") > 0 ? firstPart.substring(0,
firstPart.indexOf(",")) : firstPart;
try {
return Seconds.seconds(Integer.parseInt(sec.trim()));
}
catch (java.lang.NumberFormatException e) {
System.err.println("Unparseable cache header:" + fullCacheControl);
return Seconds.seconds(0);
}
}
else if (fullCacheControl != null && fullCacheControl.contains("no-cache")) {
return Seconds.ZERO;
}
else
if (expires != null && now!=null)
return getDelta(now.trim(), expires.trim());
else
return Seconds.ZERO;
}
public double getDays(Seconds seconds) {
if (seconds==null)
return 0;
int days = seconds.toStandardDays().getDays();
if (days!=0)
return days;
else {
int hours = seconds.toStandardHours().getHours();
return (double) Math.round((hours/24.0) * 100) / 100;
}
}
private boolean doMaxAgeExist(String fullCacheControl) {
if (fullCacheControl == null)
return false;
else if (fullCacheControl.contains("max-age="))
return true;
else
return false;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy