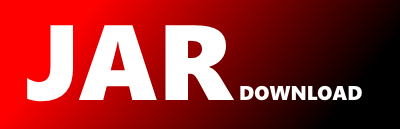
com.sourceclear.plugins.config.ConsoleConfig Maven / Gradle / Ivy
/*
Copyright (c) 2015 SourceClear Inc.
Licensed under the Apache License, Version 2.0 (the "License");
you may not use this file except in compliance with the License.
You may obtain a copy of the License at
http://www.apache.org/licenses/LICENSE-2.0
Unless required by applicable law or agreed to in writing, software
distributed under the License is distributed on an "AS IS" BASIS,
WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
See the License for the specific language governing permissions and
limitations under the License.
*/
package com.sourceclear.plugins.config;
import com.fasterxml.jackson.annotation.JsonIgnoreProperties;
import com.fasterxml.jackson.annotation.JsonProperty;
import com.sourceclear.scm.core.SCMType;
import javax.annotation.Nonnull;
/**
* Contains the configuration knobs that alter the behavior of the Terminal.
* This class is the POJO representation of the on-disk file, so try not to
* use un-mappable instance fields.
*/
@JsonIgnoreProperties(ignoreUnknown=true)
public class ConsoleConfig {
@SuppressWarnings("unused")
public static class Builder {
/** Holds the user API key. */
private String userToken;
/**
* Holds the organizational API key,
* which permits a user to interact with repositories which belong to the organization.
*/
private String orgToken;
private String scmToken;
private String scmUsername;
private char[] scmPassword;
private SCMType defaultScmType;
private String proxyServerAddress;
private Integer proxyServerPort;
private String apiUrl;
public Builder () {}
public Builder (ConsoleConfig config) {
userToken = config.getUserToken();
orgToken = config.getOrgToken();
scmToken = config.getScmToken();
scmUsername = config.getScmUsername();
scmPassword = config.getScmPassword();
proxyServerAddress = config.getProxyServerAddress();
proxyServerPort = config.getProxyServerPort();
defaultScmType = config.getDefaultScmType();
apiUrl = config.getApiUrl();
}
public Builder withUserToken(String value) {
this.userToken = value;
return this;
}
public Builder withOrgToken(String value) {
this.orgToken = value;
return this;
}
public Builder withScmToken(String scmToken) {
this.scmToken = scmToken;
return this;
}
public Builder withScmUsername(String scmUsername) {
this.scmUsername = scmUsername;
return this;
}
public Builder withScmPassword(char[] scmPassword) {
this.scmPassword = scmPassword;
return this;
}
public Builder withDefaultScmType(SCMType defaultScmType) {
this.defaultScmType = defaultScmType;
return this;
}
public Builder withApiUrl(String apiUrl) {
this.apiUrl = apiUrl;
return this;
}
public Builder withProxyServerAddress(String proxyServerAddress) {
this.proxyServerAddress = proxyServerAddress;
return this;
}
public Builder withProxyServerPort(Integer proxyServerPort) {
this.proxyServerPort = proxyServerPort;
return this;
}
/**
* Applies the given config against the data so far, applying an non-{@code null}
* values and ignoring any {@code null} ones.
* @param config the "updated" config
*/
public Builder withConsoleConfig(@Nonnull ConsoleConfig config) {
userToken = coalesce(config.getUserToken(), userToken);
orgToken = coalesce(config.getOrgToken(), orgToken);
scmToken = coalesce(config.getScmToken(), scmToken);
scmUsername = coalesce(config.getScmUsername(), scmUsername);
scmPassword = coalesce(config.getScmPassword(), scmPassword);
proxyServerAddress = coalesce(config.getProxyServerAddress(), proxyServerAddress);
proxyServerPort = coalesce(config.getProxyServerPort(), proxyServerPort);
defaultScmType = coalesce(config.getDefaultScmType(), defaultScmType);
apiUrl = coalesce(config.getApiUrl(), apiUrl);
return this;
}
public ConsoleConfig build() {
return new ConsoleConfig(this);
}
/**
* Implements the PostgreSQL function of the same name, with the same semantics: first non-{@code null} wins.
* This is preferable to {@code com.google.common.base.MoreObjects.firstNonNull} because that one
* throws {@code NullPointerException} if they are both {@code null}.
* @param the type of both A and B
* @return the first, left to right, non-{@code null} value.
*/
static T coalesce(T a, T b) {
if (null != a) {
return a;
} else {
return b;
}
}
}
///////////////////////////// Class Attributes \\\\\\\\\\\\\\\\\\\\\\\\\\\\\\
private static final String DEFAULT_API_URL = "https://api.srcclr.com";
////////////////////////////// Class Methods \\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\
//////////////////////////////// Attributes \\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\
/** Holds the user API key. */
@JsonProperty("userToken")
private final String userToken;
/**
* Holds the organizational API key,
* which permits a user to interact with repositories which belong to the organization.
*/
@JsonProperty("orgToken")
private final String orgToken;
@JsonProperty("scmToken")
private final String scmToken;
@JsonProperty("scmUsername")
private final String scmUsername;
@JsonProperty("scmPassword")
private final char[] scmPassword;
@JsonProperty("defaultScmType")
private final SCMType defaultScmType;
@JsonProperty("proxyServerAddress")
private final String proxyServerAddress;
@JsonProperty("proxyServerPort")
private final Integer proxyServerPort;
@JsonProperty("apiUrl")
private final String apiUrl;
/////////////////////////////// Constructors \\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\
@SuppressWarnings("unused")
private ConsoleConfig() {
// this is only to make javac happy;
// Jackson still puts the values into the fields as expected
this(new Builder(), false);
}
private ConsoleConfig(Builder builder) {
this(builder, true);
}
/**
*
* @param setDefaultApiUrl a bit of trickery because of the default constructor above; it isn't user data
* so don't coalesce to the default api
*/
private ConsoleConfig(Builder builder, boolean setDefaultApiUrl) {
userToken = builder.userToken;
orgToken = builder.orgToken;
scmToken = builder.scmToken;
scmUsername = builder.scmUsername;
scmPassword = builder.scmPassword;
defaultScmType = builder.defaultScmType;
proxyServerAddress = builder.proxyServerAddress;
proxyServerPort = builder.proxyServerPort;
if (setDefaultApiUrl) {
// that Builder is entirely blank and is just to make javac happy
// don't treat it as if it is real data
apiUrl = Builder.coalesce(builder.apiUrl, DEFAULT_API_URL);
} else {
apiUrl = null;
}
}
////////////////////////////////// Methods \\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\
//------------------------ Implements:
//------------------------ Overrides:
//---------------------------- Abstract Methods -----------------------------
//---------------------------- Utility Methods ------------------------------
//---------------------------- Property Methods -----------------------------
/** Returns the User API key. */
public String getUserToken() {
return userToken;
}
/**
* Returns the organizational API key,
* which permits a user to interact with repositories which belong to the organization.
*/
public String getOrgToken() {
return orgToken;
}
/**
* @return the scmToken
*/
public String getScmToken() {
return scmToken;
}
/**
* @return the scmUsername
*/
public String getScmUsername() {
return scmUsername;
}
/**
* @return the scmPassword
*/
public char[] getScmPassword() {
return scmPassword;
}
/**
* @return the defaultScmType
*/
public SCMType getDefaultScmType() {
return defaultScmType;
}
/**
* @return the apiUrl
*/
public String getApiUrl() {
return apiUrl;
}
/**
* @return the proxy server address
*/
public String getProxyServerAddress() {
return proxyServerAddress;
}
/**
* @return the port of the server
*/
public Integer getProxyServerPort() {
return proxyServerPort;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy