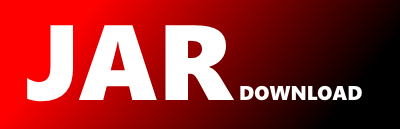
com.sourcetohtml.JEditGenerator Maven / Gradle / Ivy
Go to download
This project aims to build a command line tool that can create
HTML view with syntax highlighted source code.
It uses Jedit syntax highlighting engine and support all languages that are supported in JEdit.
Which are currently: ActionScript, Ada 95, ANTLR, Apache HTTPD, APDL, AppleScript, ASP, Aspect-J, Assembly, AWK, B formal method, Batch, BBj, BCEL, BibTeX, C, C++, C#, CHILL, CIL, COBOL, ColdFusion, CSS, CVS Commit, D, DOxygen, DSSSL, Eiffel, EmbPerl, Erlang, Factor, Fortran, Foxpro, FreeMarker, Fortran, Gettext, Groovy, Haskell, HTML, Icon, IDL, Inform, INI, Inno Setup, Informix 4GL, Interlis, Io, Java, JavaScript, JCL, JHTML, JMK, JSP, Latex, Lilypond, Lisp, LOTOS, Lua, Makefile, Maple, ML, Modula-3, MoinMoin, MQSC, NetRexx, NQC, NSIS2, Objective C, ObjectRexx, Occam, Omnimark, Parrot, Pascal, Patch, Perl, PHP, Pike, PL-SQL, PL/I, Pop11, PostScript, Povray, PowerDynamo, Progress 4GL, Prolog, Properties, PSP, PV-WAVE, Pyrex, Python, REBOL, Redcode, Relax-NG, RelationalView, Rest, Rib, RPM spec, RTF, Ruby, Ruby-HTML, RView, S+, S#, SAS, Scheme, SDL/PL, SGML, Shell Script, SHTML, Smalltalk, SMI MIB, SQR, Squidconf, SVN Commit, Swig, TCL, TeX, Texinfo, TPL, Transact-SQL, UnrealScript, VBScript, Velocity, Verilog, VHDL, XML, XSL, ZPT
The newest version!
package com.sourcetohtml;
import java.awt.Color;
import java.awt.Font;
import java.awt.FontMetrics;
import java.awt.Graphics2D;
import java.awt.image.BufferedImage;
import java.io.BufferedReader;
import java.io.BufferedWriter;
import java.io.ByteArrayOutputStream;
import java.io.File;
import java.io.FileReader;
import java.io.FileWriter;
import java.io.IOException;
import java.io.OutputStreamWriter;
import java.io.Writer;
import java.util.ArrayList;
import java.util.Iterator;
import java.util.logging.Level;
import java.util.logging.Logger;
import javax.imageio.ImageIO;
import javax.imageio.ImageWriter;
import javax.imageio.stream.ImageOutputStream;
import javax.swing.text.TabExpander;
import org.gjt.sp.jedit.Buffer;
import org.gjt.sp.jedit.Mode;
import org.gjt.sp.jedit.jEdit;
import org.gjt.sp.jedit.syntax.Chunk;
import org.gjt.sp.jedit.syntax.DisplayTokenHandler;
import org.gjt.sp.jedit.syntax.ModeProvider;
import org.gjt.sp.jedit.syntax.SyntaxStyle;
import org.gjt.sp.jedit.syntax.Token;
import org.gjt.sp.jedit.syntax.TokenMarker;
import org.gjt.sp.util.SyntaxUtilities;
/**
* Uses JEdit to determinate type of file and to parse the file into tokens
* It than iterates through the tokens and generates HTML
* and a thumbnail image if requested
* @author jcelak
*/
public class JEditGenerator extends FileDetailGenerator
{
private static final Logger log = Logger.getLogger("TextToHtml");
private static final int thumbnailWidth = 100;
private static final int thumbnailHeight = 100;
private SyntaxStyle[] syntaxStyles = null;
public static void main(String arg[]) throws Exception
{
JEditGenerator tth = new JEditGenerator();
tth.write(new File("c:\\CheckoutBeanValidatorTest.java"),new File("c:/temp/a.html"), new File("c:/temp/s.gif"));
//tth.write(new File("c:\\temp\\test\\archive\\jirka\\archive\\_ARCH_file merge.zip\\file merge\\cobal\\layout.jsp"),new File("c:/temp/b.html"));
//tth.write(new File("c:\\temp\\hchfinance\\menu_files\\ddlevelsmenu-topbar.css"),new File("c:/temp/c.html"));
//tth.write(new File("c:\\temp\\hchfinance\\menu_files\\ddlevelsmenu.js"),new File("c:/temp/d.html"));
}
public JEditGenerator()
{
init();
}
@Override
public String getHTML(File input, File root) {
ByteArrayOutputStream baos = new ByteArrayOutputStream();
write(input, new OutputStreamWriter(baos), null);
return baos.toString();
}
/**
* Initialize Jedit by loading modes and initialize syntax styles
*/
private void init()
{
jEdit.initSystemProperties();
jEdit.reloadModes();
SyntaxUtilities.propertyManager = jEdit.getPropertyManager();
String defaultFont = jEdit.getProperty("view.font");
int defaultFontSize = jEdit.getIntegerProperty("view.fontsize",12);
syntaxStyles = SyntaxUtilities.loadStyles(defaultFont,defaultFontSize);
syntaxStyles[0] = syntaxStyles[1];
}
private String readFirstLine(File f) throws Exception
{
BufferedReader in = null;
try {
in = new BufferedReader(new FileReader(f));
return in.readLine();
} finally {
if (in != null)
in.close();
}
}
public synchronized void write(File source, File destination, File thumbnailDestination)
{
try {
write(source,new FileWriter(destination),thumbnailDestination);
} catch (IOException e) {
e.printStackTrace();
}
}
public void write(File source, Writer out, File thumbnailDestination)
{
BufferedImage thumbImage = null;
try {
Graphics2D graphics2D = null;
if (thumbnailDestination != null)
{
thumbImage = new BufferedImage(thumbnailWidth, thumbnailHeight,
BufferedImage.TYPE_INT_ARGB);
graphics2D = thumbImage.createGraphics();
graphics2D.setColor(new Color(255,255,255));
graphics2D.fillRect(0, 0, thumbnailWidth, thumbnailHeight);
}
ModeProvider modeprovider = ModeProvider.instance;
Mode m = modeprovider.getModeForFile(source.getAbsolutePath(),
readFirstLine(source));
Buffer b = jEdit.openTemporary(null, source.getParentFile().getAbsolutePath(), source.getName(), false);
out = new BufferedWriter(out);
TokenMarker tm = m.getTokenMarker();
b.setTokenMarker(tm);
out.write("");
out.write("");
if (Config.getInstance().getBooleanProperty("com.sourcetohtml.JEditGenerator.generateLineNumbers", true))
{
out.write("");
for (int i=0;i"+(i+1)+"\n");
}
out.write("
");
}
out.write("\n");
int x = 1;
int y = 1;
for (int i=0;i(),0.0f);
b.markTokens(i, tokenHandler);
//System.out.print(""+(i+1)+" ");
Token t = tokenHandler.getTokens();
boolean emptyLine = true;
int maxHeight = 3;
while (t != null)
{
Chunk c = (Chunk)t;
if (c.str != null)
{
if (y < thumbnailHeight && x < thumbnailWidth && thumbnailDestination != null)
{
Font cf = c.style.getFont();
Font f = new Font("arial",cf.getStyle(),6);
graphics2D.setFont(f);
graphics2D.setColor(c.style.getForegroundColor());
FontMetrics fm = graphics2D.getFontMetrics();
int width = fm.stringWidth(c.str);
int height = fm.getAscent();
graphics2D.drawString(c.str, x, y + height);
x = x + width + 2;
maxHeight = Math.max(height, maxHeight);
}
out.write("" +
escapeSpecialCharacters(c.str) + //.replaceAll(" ", " ") +
"");
emptyLine = false;
} else
out.write("\t");
//System.out.println(" ");
t = t.next;
}
x = 1;
y = y + maxHeight + 1;
if (emptyLine)
out.write(" ");
out.write("\n");
}
out.write(" \n");
out.write("
\n");
} catch (Exception e)
{
log.log(Level.SEVERE, "Unable to generate html version of file " + source.getAbsolutePath(),e);
} finally
{
if (out != null)
{
try {
out.close();
} catch (IOException e) {}
}
if (thumbnailDestination != null)
saveImage(thumbImage,thumbnailDestination);
}
}
private void saveImage(BufferedImage thumbImage, File destination)
{
ImageWriter writer = null;
Iterator iter = ImageIO.getImageWritersByFormatName("gif");
if (iter.hasNext())
{
writer = (ImageWriter) iter.next();
}
ImageOutputStream ios = null;
try {
ios = ImageIO.createImageOutputStream(destination);
writer.setOutput(ios);
writer.write(thumbImage);
} catch (IOException e) {
log.log(Level.SEVERE, "Unable store text thumbnail " + destination.getAbsolutePath(),e);
} finally
{
if (ios != null)
{
try {
ios.flush();
ios.close();
}
catch (Exception e)
{
log.log(Level.SEVERE, "Unable close streams ",e);
}
}
writer.dispose();
}
}
private String escapeSpecialCharacters(String input)
{
return input.replaceAll("<", "<").replaceAll(">", ">");
}
private String getCSSClass(SyntaxStyle ss)
{
for (int i= 0;i
© 2015 - 2024 Weber Informatics LLC | Privacy Policy