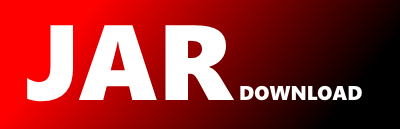
org.gjt.sp.jedit.buffer.FoldHandler Maven / Gradle / Ivy
Go to download
This project aims to build a command line tool that can create
HTML view with syntax highlighted source code.
It uses Jedit syntax highlighting engine and support all languages that are supported in JEdit.
Which are currently: ActionScript, Ada 95, ANTLR, Apache HTTPD, APDL, AppleScript, ASP, Aspect-J, Assembly, AWK, B formal method, Batch, BBj, BCEL, BibTeX, C, C++, C#, CHILL, CIL, COBOL, ColdFusion, CSS, CVS Commit, D, DOxygen, DSSSL, Eiffel, EmbPerl, Erlang, Factor, Fortran, Foxpro, FreeMarker, Fortran, Gettext, Groovy, Haskell, HTML, Icon, IDL, Inform, INI, Inno Setup, Informix 4GL, Interlis, Io, Java, JavaScript, JCL, JHTML, JMK, JSP, Latex, Lilypond, Lisp, LOTOS, Lua, Makefile, Maple, ML, Modula-3, MoinMoin, MQSC, NetRexx, NQC, NSIS2, Objective C, ObjectRexx, Occam, Omnimark, Parrot, Pascal, Patch, Perl, PHP, Pike, PL-SQL, PL/I, Pop11, PostScript, Povray, PowerDynamo, Progress 4GL, Prolog, Properties, PSP, PV-WAVE, Pyrex, Python, REBOL, Redcode, Relax-NG, RelationalView, Rest, Rib, RPM spec, RTF, Ruby, Ruby-HTML, RView, S+, S#, SAS, Scheme, SDL/PL, SGML, Shell Script, SHTML, Smalltalk, SMI MIB, SQR, Squidconf, SVN Commit, Swig, TCL, TeX, Texinfo, TPL, Transact-SQL, UnrealScript, VBScript, Velocity, Verilog, VHDL, XML, XSL, ZPT
The newest version!
/*
* FoldHandler.java - Fold handler interface
* :tabSize=8:indentSize=8:noTabs=false:
* :folding=explicit:collapseFolds=1:
*
* Copyright (C) 2001, 2005 Slava Pestov
*
* This program is free software; you can redistribute it and/or
* modify it under the terms of the GNU General Public License
* as published by the Free Software Foundation; either version 2
* of the License, or any later version.
*
* This program is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
* GNU General Public License for more details.
*
* You should have received a copy of the GNU General Public License
* along with this program; if not, write to the Free Software
* Foundation, Inc., 59 Temple Place - Suite 330, Boston, MA 02111-1307, USA.
*/
package org.gjt.sp.jedit.buffer;
import javax.swing.text.Segment;
/**
* Interface for obtaining the fold level of a specified line.
*
* Plugins can provide fold handlers by defining entries in their
* services.xml
files like so:
*
*
<SERVICE CLASS="org.gjt.sp.jedit.buffer.FoldHandler" NAME="name">
* new MyFoldHandler();
*</SERVICE>
*
* See {@link org.gjt.sp.jedit.ServiceManager} for details.
*
* @author Slava Pestov
* @version $Id: FoldHandler.java 12504 2008-04-22 23:12:43Z ezust $
* @since jEdit 4.3pre3
*/
public abstract class FoldHandler
{
/**
* The service type. See {@link org.gjt.sp.jedit.ServiceManager}.
* @since jEdit 4.2pre1
* @deprecated use {@link org.gjt.sp.jedit.ServiceManager.ServiceFoldHandlerProvider}
*/
@Deprecated
public static final String SERVICE = "org.gjt.sp.jedit.buffer.FoldHandler";
/** The FoldHandlerProvider. */
public static FoldHandlerProvider foldHandlerProvider;
//{{{ getName() method
/**
* Returns the internal name of this FoldHandler
* @return The internal name of this FoldHandler
* @since jEdit 4.0pre6
*/
public String getName()
{
return name;
}
//}}}
//{{{ getFoldLevel() method
/**
* Returns the fold level of the specified line.
* @param buffer The buffer in question
* @param lineIndex The line index
* @param seg A segment the fold handler can use to obtain any
* text from the buffer, if necessary
* @return The fold level of the specified line
* @since jEdit 4.0pre1
*/
public abstract int getFoldLevel(JEditBuffer buffer, int lineIndex, Segment seg);
//}}}
//{{{ equals() method
/**
* Returns if the specified fold handler is equal to this one.
* @param o The object
*/
public boolean equals(Object o)
{
// Default implementation... subclasses can extend this.
if(o == null)
return false;
else
return getClass() == o.getClass();
} //}}}
//{{{ hashCode() method
public int hashCode()
{
return getClass().hashCode();
} //}}}
//{{{ getFoldHandler() method
/**
* Returns the fold handler with the specified name, or null if
* there is no registered handler with that name.
* @param name The name of the desired fold handler
* @since jEdit 4.0pre6
*/
public static FoldHandler getFoldHandler(String name)
{
return foldHandlerProvider.getFoldHandler(name);
}
//}}}
//{{{ getFoldModes() method
/**
* Returns an array containing the names of all registered fold
* handlers.
*
* @since jEdit 4.0pre6
*/
public static String[] getFoldModes()
{
return foldHandlerProvider.getFoldModes();
}
//}}}
//{{{ FoldHandler() constructor
protected FoldHandler(String name)
{
this.name = name;
}
//}}}
//{{{ toString() method
public String toString()
{
return name;
} //}}}
private String name;
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy