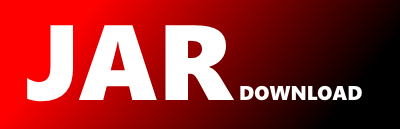
org.gjt.sp.jedit.gui.DefaultInputHandler Maven / Gradle / Ivy
Go to download
This project aims to build a command line tool that can create
HTML view with syntax highlighted source code.
It uses Jedit syntax highlighting engine and support all languages that are supported in JEdit.
Which are currently: ActionScript, Ada 95, ANTLR, Apache HTTPD, APDL, AppleScript, ASP, Aspect-J, Assembly, AWK, B formal method, Batch, BBj, BCEL, BibTeX, C, C++, C#, CHILL, CIL, COBOL, ColdFusion, CSS, CVS Commit, D, DOxygen, DSSSL, Eiffel, EmbPerl, Erlang, Factor, Fortran, Foxpro, FreeMarker, Fortran, Gettext, Groovy, Haskell, HTML, Icon, IDL, Inform, INI, Inno Setup, Informix 4GL, Interlis, Io, Java, JavaScript, JCL, JHTML, JMK, JSP, Latex, Lilypond, Lisp, LOTOS, Lua, Makefile, Maple, ML, Modula-3, MoinMoin, MQSC, NetRexx, NQC, NSIS2, Objective C, ObjectRexx, Occam, Omnimark, Parrot, Pascal, Patch, Perl, PHP, Pike, PL-SQL, PL/I, Pop11, PostScript, Povray, PowerDynamo, Progress 4GL, Prolog, Properties, PSP, PV-WAVE, Pyrex, Python, REBOL, Redcode, Relax-NG, RelationalView, Rest, Rib, RPM spec, RTF, Ruby, Ruby-HTML, RView, S+, S#, SAS, Scheme, SDL/PL, SGML, Shell Script, SHTML, Smalltalk, SMI MIB, SQR, Squidconf, SVN Commit, Swig, TCL, TeX, Texinfo, TPL, Transact-SQL, UnrealScript, VBScript, Velocity, Verilog, VHDL, XML, XSL, ZPT
The newest version!
/*
* DefaultInputHandler.java - Default implementation of an input handler
* :tabSize=8:indentSize=8:noTabs=false:
* :folding=explicit:collapseFolds=1:
*
* Copyright (C) 1999, 2003 Slava Pestov
*
* This program is free software; you can redistribute it and/or
* modify it under the terms of the GNU General Public License
* as published by the Free Software Foundation; either version 2
* of the License, or any later version.
*
* This program is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
* GNU General Public License for more details.
*
* You should have received a copy of the GNU General Public License
* along with this program; if not, write to the Free Software
* Foundation, Inc., 59 Temple Place - Suite 330, Boston, MA 02111-1307, USA.
*/
package org.gjt.sp.jedit.gui;
//{{{ Imports
import java.awt.event.InputEvent;
import java.awt.event.KeyEvent;
import java.awt.Toolkit;
import java.util.Hashtable;
import org.gjt.sp.jedit.*;
//}}}
/**
* The default input handler. It maps sequences of keystrokes into actions
* and inserts key typed events into the text area.
* @author Slava Pestov
* @version $Id: DefaultInputHandler.java 12504 2008-04-22 23:12:43Z ezust $
*/
public class DefaultInputHandler extends InputHandler
{
//{{{ DefaultInputHandler constructor
/**
* Creates a new input handler with no key bindings defined.
* @param view The view
* @param bindings An explicitly-specified set of key bindings,
* must not be null.
* @since jEdit 4.3pre1
*/
public DefaultInputHandler(View view, Hashtable bindings)
{
super(view);
if(bindings == null)
throw new NullPointerException();
this.bindings = this.currentBindings = bindings;
} //}}}
//{{{ DefaultInputHandler constructor
/**
* Creates a new input handler with no key bindings defined.
* @param view The view
*/
public DefaultInputHandler(View view)
{
this(view,new Hashtable());
} //}}}
//{{{ DefaultInputHandler constructor
/**
* Creates a new input handler with the same set of key bindings
* as the one specified. Note that both input handlers share
* a pointer to exactly the same key binding table; so adding
* a key binding in one will also add it to the other.
* @param copy The input handler to copy key bindings from
* @param view The view
*/
public DefaultInputHandler(View view, DefaultInputHandler copy)
{
this(view,copy.bindings);
} //}}}
//{{{ isPrefixActive() method
/**
* Returns if a prefix key has been pressed.
*/
@Override
public boolean isPrefixActive()
{
return bindings != currentBindings
|| super.isPrefixActive();
} //}}}
//{{{ setCurrentBindings() method
@Override
public void setCurrentBindings(Hashtable bindings)
{
currentBindings = bindings;
} //}}}
@Override
public void invokeAction(Object action) {
// TODO Auto-generated method stub
}
@Override
public void invokeAction(String action) {
// TODO Auto-generated method stub
}
@Override
public void processKeyEvent(KeyEvent evt, int from, boolean global) {
// TODO Auto-generated method stub
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy