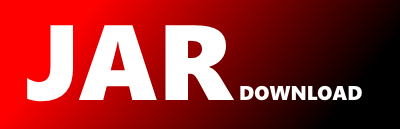
org.gjt.sp.jedit.gui.InputHandler Maven / Gradle / Ivy
/*
* InputHandler.java - Manages key bindings and executes actions
* :tabSize=8:indentSize=8:noTabs=false:
* :folding=explicit:collapseFolds=1:
*
* Copyright (C) 1999, 2003 Slava Pestov
*
* This program is free software; you can redistribute it and/or
* modify it under the terms of the GNU General Public License
* as published by the Free Software Foundation; either version 2
* of the License, or any later version.
*
* This program is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
* GNU General Public License for more details.
*
* You should have received a copy of the GNU General Public License
* along with this program; if not, write to the Free Software
* Foundation, Inc., 59 Temple Place - Suite 330, Boston, MA 02111-1307, USA.
*/
package org.gjt.sp.jedit.gui;
//{{{ Imports
import javax.swing.*;
import javax.swing.text.JTextComponent;
import org.gjt.sp.jedit.textarea.JEditTextArea;
import org.gjt.sp.jedit.*;
import org.gjt.sp.jedit.buffer.JEditBuffer;
import org.gjt.sp.jedit.input.AbstractInputHandler;
import org.gjt.sp.util.Log;
import org.gjt.sp.util.StandardUtilities;
import java.awt.event.KeyEvent;
import java.awt.*;
//}}}
/**
* An input handler converts the user's key strokes into concrete actions.
* It also takes care of macro recording and action repetition.
*
* This class provides all the necessary support code for an input
* handler, but doesn't actually do any key binding logic. It is up
* to the implementations of this class to do so.
*
* @author Slava Pestov
* @version $Id: InputHandler.java 12942 2008-06-27 08:33:55Z kpouer $
* @see org.gjt.sp.jedit.gui.DefaultInputHandler
*/
public abstract class InputHandler extends AbstractInputHandler
{
//{{{ InputHandler constructor
/**
* Creates a new input handler.
* @param view The view
*/
protected InputHandler(View view)
{
this.view = view;
} //}}}
//{{{ processKeyEventSub() method
private void processKeyEventSub(boolean focusOnTextArea)
{
// we might have been closed as a result of
// the above
if(view.isClosed())
return;
// this is a weird hack.
// we don't want C+e a to insert 'a' in the
// search bar if the search bar has focus...
if(isPrefixActive())
{
Component focusOwner = view.getFocusOwner();
if(focusOwner instanceof JTextComponent)
{
view.setPrefixFocusOwner(focusOwner);
view.getTextArea().requestFocus();
}
else if(focusOnTextArea)
{
view.getTextArea().requestFocus();
}
else
{
view.setPrefixFocusOwner(null);
}
}
else
{
view.setPrefixFocusOwner(null);
}
}
//}}}
//{{{ getRepeatCount() method
/**
* Returns the number of times the next action will be repeated.
*/
public int getRepeatCount()
{
return repeatCount;
} //}}}
//{{{ setRepeatCount() method
/**
* Sets the number of times the next action will be repeated.
* @param repeatCount The repeat count
*/
public void setRepeatCount(int repeatCount)
{
} //}}}
//{{{ readNextChar() method
/**
* Invokes the specified BeanShell code, replacing __char__ in the
* code with the next input character.
* @param msg The prompt to display in the status bar
* @param code The code
* @since jEdit 3.2pre2
*/
public void readNextChar(String msg, String code)
{
readNextChar = code;
} //}}}
//{{{ readNextChar() method
/**
* @deprecated Use the other form of this method instead
*/
@Deprecated
public void readNextChar(String code)
{
readNextChar = code;
} //}}}
//{{{ invokeLastAction() method
public void invokeLastAction()
{
if(lastAction == null)
view.getToolkit().beep();
else
invokeAction(lastAction);
} //}}}
//{{{ Instance variables
protected final View view;
//}}}
//{{{ userInput() method
protected void userInput(char ch)
{
} //}}}
//{{{ invokeReadNextChar() method
protected void invokeReadNextChar(char ch)
{
JEditBuffer buffer = view.getBuffer();
/* if(buffer.insideCompoundEdit())
buffer.endCompoundEdit(); */
String charStr = StandardUtilities.charsToEscapes(String.valueOf(ch));
// this might be a bit slow if __char__ occurs a lot
int index;
while((index = readNextChar.indexOf("__char__")) != -1)
{
readNextChar = readNextChar.substring(0,index)
+ '\'' + charStr + '\''
+ readNextChar.substring(index + 8);
}
readNextChar = null;
} //}}}
}