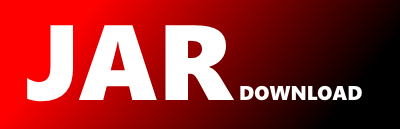
org.gjt.sp.jedit.textarea.JEditTextArea Maven / Gradle / Ivy
/*
* JEditTextArea.java - jEdit's text component
* :tabSize=8:indentSize=8:noTabs=false:
* :folding=explicit:collapseFolds=1:
*
* Copyright (C) 1999, 2005 Slava Pestov
* Portions copyright (C) 2000 Ollie Rutherfurd
*
* This program is free software; you can redistribute it and/or
* modify it under the terms of the GNU General Public License
* as published by the Free Software Foundation; either version 2
* of the License, or any later version.
*
* This program is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
* GNU General Public License for more details.
*
* You should have received a copy of the GNU General Public License
* along with this program; if not, write to the Free Software
* Foundation, Inc., 59 Temple Place - Suite 330, Boston, MA 02111-1307, USA.
*/
package org.gjt.sp.jedit.textarea;
//{{{ Imports
import java.awt.AWTEvent;
import java.awt.Point;
import java.awt.event.MouseEvent;
import javax.swing.JPopupMenu;
import org.gjt.sp.jedit.*;
/**
* jEdit's text component.
*
* Unlike most other text editors, the selection API permits selection and
* concurrent manipulation of multiple, non-contiguous regions of text.
* Methods in this class that deal with selecting text rely upon classes derived
* the {@link Selection} class.
*
* @author Slava Pestov
* @author John Gellene (API documentation)
* @version $Id: JEditTextArea.java 13005 2008-07-04 19:38:16Z ezust $
*/
public class JEditTextArea extends TextArea
{
//{{{ JEditTextArea constructor
/**
* Creates a new JEditTextArea.
*/
public JEditTextArea(View view)
{
super(jEdit.getPropertyManager(), view);
enableEvents(AWTEvent.FOCUS_EVENT_MASK | AWTEvent.KEY_EVENT_MASK);
popupEnabled = true;
this.view = view;
} //}}}
// {{{ overrides from the base class that are EditBus aware
public void goToBufferEnd(boolean select)
{
}
public void goToBufferStart(boolean select)
{
} // }}}
//{{{ showGoToLineDialog() method
/**
* Displays the 'go to line' dialog box, and moves the caret to the
* specified line number.
* @since jEdit 2.7pre2
*/
public void showGoToLineDialog()
{
} //}}}
//{{{ doWordCount() method
protected static void doWordCount(View view, String text)
{
char[] chars = text.toCharArray();
int characters = chars.length;
int words = 0;
int lines = 1;
boolean word = true;
for(int i = 0; i < chars.length; i++)
{
switch(chars[i])
{
case '\r': case '\n':
lines++;
case ' ': case '\t':
word = true;
break;
default:
if(word)
{
words++;
word = false;
}
break;
}
}
Object[] args = { characters, words, lines };
} //}}}
//{{{ Getters and setters
//{{{ getView() method
/**
* Returns this text area's view.
* @since jEdit 4.2pre5
*/
public View getView()
{
return view;
} //}}}
//}}}
//{{{ Private members
//{{{ Instance variables
private View view;
private JPopupMenu popup;
private boolean popupEnabled;
//}}}
//}}}
//{{{ isRightClickPopupEnabled() method
/**
* Returns if the right click popup menu is enabled. The Gestures
* plugin uses this API.
* @since jEdit 4.2pre13
*/
public boolean isRightClickPopupEnabled()
{
return popupEnabled;
} //}}}
//{{{ setRightClickPopupEnabled() method
/**
* Sets if the right click popup menu is enabled. The Gestures
* plugin uses this API.
* @since jEdit 4.2pre13
*/
public void setRightClickPopupEnabled(boolean popupEnabled)
{
this.popupEnabled = popupEnabled;
} //}}}
//{{{ getRightClickPopup() method
/**
* Returns the right click popup menu.
*/
public final JPopupMenu getRightClickPopup()
{
return popup;
} //}}}
//{{{ setRightClickPopup() method
/**
* Sets the right click popup menu.
* @param popup The popup
*/
public final void setRightClickPopup(JPopupMenu popup)
{
this.popup = popup;
} //}}}
//{{{ handlePopupTrigger() method
/**
* Do the same thing as right-clicking on the text area. The Gestures
* plugin uses this API.
* @since jEdit 4.2pre13
*/
public void handlePopupTrigger(MouseEvent evt)
{
} //}}}
//{{{ showPopupMenu() method
/**
* Shows the popup menu below the current caret position.
* @since 4.3pre10
*/
public void showPopupMenu()
{
} //}}}
}