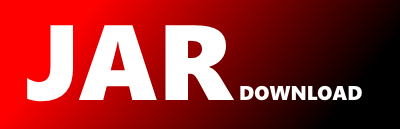
org.gjt.sp.util.WorkThread Maven / Gradle / Ivy
Go to download
This project aims to build a command line tool that can create
HTML view with syntax highlighted source code.
It uses Jedit syntax highlighting engine and support all languages that are supported in JEdit.
Which are currently: ActionScript, Ada 95, ANTLR, Apache HTTPD, APDL, AppleScript, ASP, Aspect-J, Assembly, AWK, B formal method, Batch, BBj, BCEL, BibTeX, C, C++, C#, CHILL, CIL, COBOL, ColdFusion, CSS, CVS Commit, D, DOxygen, DSSSL, Eiffel, EmbPerl, Erlang, Factor, Fortran, Foxpro, FreeMarker, Fortran, Gettext, Groovy, Haskell, HTML, Icon, IDL, Inform, INI, Inno Setup, Informix 4GL, Interlis, Io, Java, JavaScript, JCL, JHTML, JMK, JSP, Latex, Lilypond, Lisp, LOTOS, Lua, Makefile, Maple, ML, Modula-3, MoinMoin, MQSC, NetRexx, NQC, NSIS2, Objective C, ObjectRexx, Occam, Omnimark, Parrot, Pascal, Patch, Perl, PHP, Pike, PL-SQL, PL/I, Pop11, PostScript, Povray, PowerDynamo, Progress 4GL, Prolog, Properties, PSP, PV-WAVE, Pyrex, Python, REBOL, Redcode, Relax-NG, RelationalView, Rest, Rib, RPM spec, RTF, Ruby, Ruby-HTML, RView, S+, S#, SAS, Scheme, SDL/PL, SGML, Shell Script, SHTML, Smalltalk, SMI MIB, SQR, Squidconf, SVN Commit, Swig, TCL, TeX, Texinfo, TPL, Transact-SQL, UnrealScript, VBScript, Velocity, Verilog, VHDL, XML, XSL, ZPT
The newest version!
/*
* WorkThread.java - Background thread that does stuff
* Copyright (C) 2000 Slava Pestov
*
* This program is free software; you can redistribute it and/or
* modify it under the terms of the GNU General Public License
* as published by the Free Software Foundation; either version 2
* of the License, or any later version.
*
* This program is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
* GNU General Public License for more details.
*
* You should have received a copy of the GNU General Public License
* along with this program; if not, write to the Free Software
* Foundation, Inc., 59 Temple Place - Suite 330, Boston, MA 02111-1307, USA.
*/
package org.gjt.sp.util;
/**
* Services work requests in the background.
* @author Slava Pestov
* @version $Id: WorkThread.java 12504 2008-04-22 23:12:43Z ezust $
*/
public class WorkThread extends Thread implements ThreadAbortMonitor
{
/**
* Sets if the current request can be aborted.
* If set to true and already aborted, the thread will be stopped
*
* @param abortable true if the WorkThread is abortable
* @since jEdit 2.6pre1
*/
public void setAbortable(boolean abortable)
{
synchronized(abortLock)
{
this.abortable = abortable;
if(aborted)
stop(new Abort());
}
}
/**
* Returns if the work thread is currently running a request.
* @return true if a request is currently running
*/
public boolean isRequestRunning()
{
return requestRunning;
}
public boolean isAborted()
{
synchronized (abortLock)
{
return aborted;
}
}
/**
* Returns the status text.
* @return the status label
*/
public String getStatus()
{
return status;
}
/**
* Sets the status text.
* @param status the new status of the thread
* @since jEdit 2.6pre1
*/
public void setStatus(String status)
{
this.status = status;
}
/**
* Returns the progress value.
* @return the progress value
*/
public int getProgressValue()
{
return progressValue;
}
/**
* Sets the progress value.
* @param progressValue the new progress value
* @since jEdit 2.6pre1
*/
public void setProgressValue(int progressValue)
{
this.progressValue = progressValue;
}
/**
* Returns the progress maximum.
* @return the maximum value of the progression
*/
public int getProgressMaximum()
{
return progressMaximum;
}
/**
* Sets the maximum progress value.
* @param progressMaximum the maximum value of the progression
* @since jEdit 2.6pre1
*/
public void setProgressMaximum(int progressMaximum)
{
this.progressMaximum = progressMaximum;
}
/**
* Aborts the currently running request, if allowed.
* @since jEdit 2.6pre1
*/
public void abortCurrentRequest()
{
synchronized(abortLock)
{
if(abortable && !aborted)
stop(new Abort());
aborted = true;
}
}
public void run()
{
Log.log(Log.DEBUG,this,"Work request thread starting [" + getName() + "]");
for(;;)
{
doRequests();
}
}
// private members
private final Object abortLock = new Object();
private boolean requestRunning;
private boolean abortable;
private boolean aborted;
private String status;
private int progressValue;
private int progressMaximum;
private void doRequests()
{
}
public static class Abort extends Error
{
public Abort()
{
super("Work request aborted");
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy