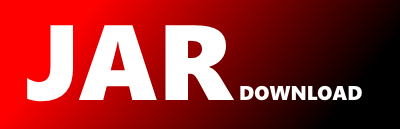
commonMain.com.soywiz.korev.EventDispatcher.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of korgw Show documentation
Show all versions of korgw Show documentation
Portable UI with accelerated graphics support for Kotlin
package com.soywiz.korev
import com.soywiz.kds.*
import com.soywiz.korio.lang.*
import kotlin.reflect.*
interface EventDispatcher {
fun addEventListener(clazz: KClass, handler: (T) -> Unit): Closeable
fun dispatch(clazz: KClass, event: T)
fun copyFrom(other: EventDispatcher) = Unit
open class Mixin : EventDispatcher {
private var handlers: LinkedHashMap, FastArrayList<(Event) -> Unit>>? = null
private fun getHandlersFor(clazz: KClass): FastArrayList<(T) -> Unit>? {
if (handlers == null) return null
@Suppress("UNCHECKED_CAST")
return handlers?.get(clazz) as? FastArrayList<(T) -> Unit>?
}
private fun getHandlersForCreate(clazz: KClass): FastArrayList<(T) -> Unit> {
if (handlers == null) handlers = LinkedHashMap()
@Suppress("UNCHECKED_CAST")
return handlers!!.getOrPut(clazz) { FastArrayList() } as FastArrayList<(T) -> Unit>
}
override fun addEventListener(clazz: KClass, handler: (T) -> Unit): Closeable {
val handlers = getHandlersForCreate(clazz)
handlers += handler
return Closeable { handlers -= handler }
}
final override fun copyFrom(other: EventDispatcher) {
handlers?.clear()
if (other is Mixin) {
val otherHandlers = other.handlers
if (otherHandlers != null) {
for ((clazz, events) in otherHandlers) {
for (event in events) {
addEventListener(clazz, event)
}
}
}
}
}
override fun dispatch(clazz: KClass, event: T) {
if (handlers == null) return
getHandlersFor(clazz)?.fastForEach { handler ->
handler(event)
}
}
}
companion object {
operator fun invoke(): EventDispatcher = Mixin()
}
}
object DummyEventDispatcher : EventDispatcher, Closeable {
override fun close() {
}
override fun addEventListener(clazz: KClass, handler: (T) -> Unit): Closeable {
return this
}
override fun dispatch(clazz: KClass, event: T) {
}
}
inline fun EventDispatcher.addEventListener(noinline handler: (T) -> Unit) = addEventListener(T::class, handler)
inline fun EventDispatcher.dispatch(event: T) = dispatch(T::class, event)
inline operator fun T.invoke(callback: T.() -> Unit): T = this.apply(callback)
open class Event {
var target: Any? = null
var _stopPropagation = false
fun stopPropagation() {
_stopPropagation = true
}
}
fun Event.preventDefault(reason: Any? = null): Nothing = throw PreventDefaultException(reason)
fun preventDefault(reason: Any? = null): Nothing = throw PreventDefaultException(reason)
class PreventDefaultException(val reason: Any? = null) : Exception()
/*
//interface Cancellable
interface EventDispatcher {
class Mixin : EventDispatcher {
val events = hashMapOf, ArrayList<(Any) -> Unit>>()
override fun addEventListener(clazz: KClass, handler: (T) -> Unit): Cancellable {
val handlers = events.getOrPut(clazz) { arrayListOf() }
val chandler = handler as ((Any) -> Unit)
handlers += chandler
return Cancellable { handlers -= chandler }
}
override fun dispatch(event: T, clazz: KClass) {
val handlers = events[clazz]
if (handlers != null) {
for (handler in handlers.toList()) {
handler(event)
}
}
}
}
fun addEventListener(clazz: KClass, handler: (T) -> Unit): Cancellable
fun dispatch(event: T, clazz: KClass = event::class): Unit
}
interface Event
inline fun EventDispatcher.addEventListener(noinline handler: (T) -> Unit): Cancellable =
this.addEventListener(T::class, handler)
inline suspend fun EventDispatcher.addEventListenerSuspend(noinline handler: suspend (T) -> Unit): Cancellable {
val context = getCoroutineContext()
return this.addEventListener(T::class) { event ->
context.go {
handler(event)
}
}
}
/*
class ED : EventDispatcher by EventDispatcher.Mixin() {
override fun dispatch(event: Any) {
//super.dispatch(event) // ERROR!
println("dispatched: $event!")
}
}
open class ED1 : EventDispatcher by EventDispatcher.Mixin()
open class ED2 : ED1() {
override fun dispatch(event: Any) {
super.dispatch(event) // WORKS
println("dispatched: $event!")
}
}
class ED2(val ed: EventDispatcher = EventDispatcher.Mixin()) : EventDispatcher by ed {
override fun dispatch(event: Any) {
ed.dispatch(event)
println("dispatched: $event!")
}
}
*/
*/
© 2015 - 2025 Weber Informatics LLC | Privacy Policy