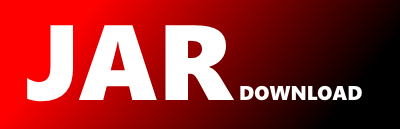
macosMain.korlibs.render.KeyMapping.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of korgw Show documentation
Show all versions of korgw Show documentation
Portable UI with accelerated graphics support for Kotlin
package korlibs.render
import korlibs.event.*
// https://stackoverflow.com/questions/3202629/where-can-i-find-a-list-of-mac-virtual-key-codes/16125341
internal val KeyCodesToKeys = mapOf(
0x00 to Key.A,
0x01 to Key.S,
0x02 to Key.D,
0x03 to Key.F,
0x04 to Key.H,
0x05 to Key.G,
0x06 to Key.Z,
0x07 to Key.X,
0x08 to Key.C,
0x09 to Key.V,
0x0B to Key.B,
0x0C to Key.Q,
0x0D to Key.W,
0x0E to Key.E,
0x0F to Key.R,
0x10 to Key.Y,
0x11 to Key.T,
0x12 to Key.N1,
0x13 to Key.N2,
0x14 to Key.N3,
0x15 to Key.N4,
0x16 to Key.N6,
0x17 to Key.N5,
0x18 to Key.EQUAL,
0x19 to Key.N9,
0x1A to Key.N7,
0x1B to Key.MINUS,
0x1C to Key.N8,
0x1D to Key.N0,
0x1E to Key.RIGHT_BRACKET,
0x1F to Key.O,
0x20 to Key.U,
0x21 to Key.LEFT_BRACKET,
0x22 to Key.I,
0x23 to Key.P,
0x25 to Key.L,
0x26 to Key.J,
0x27 to Key.QUOTE,
0x28 to Key.K,
0x29 to Key.SEMICOLON,
0x2A to Key.BACKSLASH,
0x2B to Key.COMMA,
0x2C to Key.SLASH,
0x2D to Key.N,
0x2E to Key.M,
0x2F to Key.PERIOD,
0x32 to Key.GRAVE_ACCENT,
0x41 to Key.KP_DECIMAL,
0x43 to Key.KP_MULTIPLY,
0x45 to Key.PLUS,
0x47 to Key.CLEAR,
0x4B to Key.KP_DIVIDE,
0x4C to Key.ENTER,
0x4E to Key.MINUS,
0x51 to Key.EQUAL,
0x52 to Key.KP_0,
0x53 to Key.KP_1,
0x54 to Key.KP_2,
0x55 to Key.KP_3,
0x56 to Key.KP_4,
0x57 to Key.KP_5,
0x58 to Key.KP_6,
0x59 to Key.KP_7,
0x5B to Key.KP_8,
0x5C to Key.KP_9,
////
0x24 to Key.ENTER,
0x4C to Key.ENTER,
0x30 to Key.TAB,
0x31 to Key.SPACE,
//0x33 to Key.DELETE,
0x33 to Key.BACKSPACE,
0x35 to Key.ESCAPE,
0x37 to Key.META,
0x38 to Key.LEFT_SHIFT,
0x39 to Key.CAPS_LOCK,
0x3A to Key.LEFT_ALT,
0x3B to Key.LEFT_CONTROL,
0x3C to Key.RIGHT_SHIFT,
0x3D to Key.RIGHT_ALT,
0x3E to Key.RIGHT_CONTROL,
0x7B to Key.LEFT,
0x7C to Key.RIGHT,
0x7D to Key.DOWN,
0x7E to Key.UP,
0x48 to Key.VOLUME_UP,
0x49 to Key.VOLUME_DOWN,
0x4A to Key.MUTE,
0x72 to Key.HELP,
0x73 to Key.HOME,
0x74 to Key.PAGE_UP,
0x75 to Key.DELETE,
0x77 to Key.END,
0x79 to Key.PAGE_DOWN,
0x3F to Key.FUNCTION,
0x7A to Key.F1,
0x78 to Key.F2,
0x76 to Key.F4,
0x60 to Key.F5,
0x61 to Key.F6,
0x62 to Key.F7,
0x63 to Key.F3,
0x64 to Key.F8,
0x65 to Key.F9,
0x6D to Key.F10,
0x67 to Key.F11,
0x6F to Key.F12,
0x69 to Key.F13,
0x6B to Key.F14,
0x71 to Key.F15,
0x6A to Key.F16,
0x40 to Key.F17,
0x4F to Key.F18,
0x50 to Key.F19,
0x5A to Key.F20
)
internal val CharToKeys = mapOf(
'a' to Key.A, 'A' to Key.A,
'b' to Key.B, 'B' to Key.B,
'c' to Key.C, 'C' to Key.C,
'd' to Key.D, 'D' to Key.D,
'e' to Key.E, 'E' to Key.E,
'f' to Key.F, 'F' to Key.F,
'g' to Key.G, 'G' to Key.G,
'h' to Key.H, 'H' to Key.H,
'i' to Key.I, 'I' to Key.I,
'j' to Key.J, 'J' to Key.J,
'k' to Key.K, 'K' to Key.K,
'l' to Key.L, 'L' to Key.L,
'm' to Key.M, 'M' to Key.M,
'n' to Key.N, 'N' to Key.N,
'o' to Key.O, 'O' to Key.O,
'p' to Key.P, 'P' to Key.P,
'q' to Key.Q, 'Q' to Key.Q,
'r' to Key.R, 'R' to Key.R,
's' to Key.S, 'S' to Key.S,
't' to Key.T, 'T' to Key.T,
'u' to Key.U, 'U' to Key.U,
'v' to Key.V, 'V' to Key.V,
'w' to Key.W, 'W' to Key.W,
'x' to Key.X, 'X' to Key.X,
'y' to Key.Y, 'Y' to Key.Y,
'z' to Key.Z, 'Z' to Key.Z,
'0' to Key.N0, '1' to Key.N1, '2' to Key.N2, '3' to Key.N3, '4' to Key.N4,
'5' to Key.N5, '6' to Key.N6, '7' to Key.N7, '8' to Key.N8, '9' to Key.N9
)
© 2015 - 2025 Weber Informatics LLC | Privacy Policy