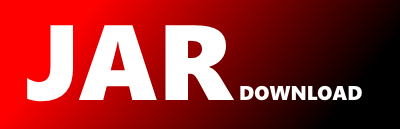
com.company.sakila.db0.sakila.customer.generated.GeneratedCustomerSqlAdapter Maven / Gradle / Ivy
package com.company.sakila.db0.sakila.customer.generated;
import com.company.sakila.db0.sakila.customer.Customer;
import com.company.sakila.db0.sakila.customer.CustomerImpl;
import com.speedment.common.annotation.GeneratedCode;
import com.speedment.common.injector.annotation.ExecuteBefore;
import com.speedment.common.injector.annotation.WithState;
import com.speedment.runtime.config.identifier.TableIdentifier;
import com.speedment.runtime.core.component.sql.SqlPersistenceComponent;
import com.speedment.runtime.core.component.sql.SqlStreamSupplierComponent;
import com.speedment.runtime.core.exception.SpeedmentException;
import java.sql.ResultSet;
import java.sql.SQLException;
import static com.speedment.common.injector.State.RESOLVED;
/**
* The generated Sql Adapter for a {@link
* com.company.sakila.db0.sakila.customer.Customer} entity.
*
* This file has been automatically generated by Speedment. Any changes made to
* it will be overwritten.
*
* @author Speedment
*/
@GeneratedCode("Speedment")
public abstract class GeneratedCustomerSqlAdapter {
private final TableIdentifier tableIdentifier;
protected GeneratedCustomerSqlAdapter() {
this.tableIdentifier = TableIdentifier.of("db0", "sakila", "customer");
}
@ExecuteBefore(RESOLVED)
void installMethodName(@WithState(RESOLVED) SqlStreamSupplierComponent streamSupplierComponent,
@WithState(RESOLVED) SqlPersistenceComponent persistenceComponent) {
streamSupplierComponent.install(tableIdentifier, this::apply);
persistenceComponent.install(tableIdentifier);
}
protected Customer apply(ResultSet resultSet) throws SpeedmentException {
final Customer entity = createEntity();
try {
entity.setCustomerId( resultSet.getInt(1) );
entity.setStoreId( resultSet.getShort(2) );
entity.setFirstName( resultSet.getString(3) );
entity.setLastName( resultSet.getString(4) );
entity.setEmail( resultSet.getString(5) );
entity.setAddressId( resultSet.getInt(6) );
entity.setActive( resultSet.getInt(7) );
entity.setCreateDate( resultSet.getTimestamp(8) );
entity.setLastUpdate( resultSet.getTimestamp(9) );
} catch (final SQLException sqle) {
throw new SpeedmentException(sqle);
}
return entity;
}
protected CustomerImpl createEntity() {
return new CustomerImpl();
}
}