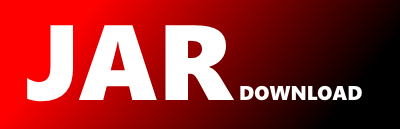
com.company.sakila.db0.sakila.film.generated.GeneratedFilm Maven / Gradle / Ivy
package com.company.sakila.db0.sakila.film.generated;
import com.company.sakila.db0.sakila.film.Film;
import com.company.sakila.db0.sakila.language.Language;
import com.speedment.common.annotation.GeneratedCode;
import com.speedment.runtime.config.identifier.ColumnIdentifier;
import com.speedment.runtime.config.identifier.TableIdentifier;
import com.speedment.runtime.core.manager.Manager;
import com.speedment.runtime.core.util.OptionalUtil;
import com.speedment.runtime.field.ComparableField;
import com.speedment.runtime.field.ComparableForeignKeyField;
import com.speedment.runtime.field.IntField;
import com.speedment.runtime.field.ShortField;
import com.speedment.runtime.field.ShortForeignKeyField;
import com.speedment.runtime.field.StringField;
import com.speedment.runtime.typemapper.TypeMapper;
import java.math.BigDecimal;
import java.sql.Date;
import java.sql.Timestamp;
import java.util.Optional;
import java.util.OptionalInt;
/**
* The generated base for the {@link
* com.company.sakila.db0.sakila.film.Film}-interface representing entities of
* the {@code film}-table in the database.
*
* This file has been automatically generated by Speedment. Any changes made to
* it will be overwritten.
*
* @author Speedment
*/
@GeneratedCode("Speedment")
public interface GeneratedFilm {
/**
* This Field corresponds to the {@link Film} field that can be obtained
* using the {@link Film#getFilmId()} method.
*/
IntField FILM_ID = IntField.create(
Identifier.FILM_ID,
Film::getFilmId,
Film::setFilmId,
TypeMapper.primitive(),
true
);
/**
* This Field corresponds to the {@link Film} field that can be obtained
* using the {@link Film#getTitle()} method.
*/
StringField TITLE = StringField.create(
Identifier.TITLE,
Film::getTitle,
Film::setTitle,
TypeMapper.identity(),
false
);
/**
* This Field corresponds to the {@link Film} field that can be obtained
* using the {@link Film#getDescription()} method.
*/
StringField DESCRIPTION = StringField.create(
Identifier.DESCRIPTION,
o -> OptionalUtil.unwrap(o.getDescription()),
Film::setDescription,
TypeMapper.identity(),
false
);
/**
* This Field corresponds to the {@link Film} field that can be obtained
* using the {@link Film#getReleaseYear()} method.
*/
ComparableField RELEASE_YEAR = ComparableField.create(
Identifier.RELEASE_YEAR,
o -> OptionalUtil.unwrap(o.getReleaseYear()),
Film::setReleaseYear,
TypeMapper.identity(),
false
);
/**
* This Field corresponds to the {@link Film} field that can be obtained
* using the {@link Film#getLanguageId()} method.
*/
ShortForeignKeyField LANGUAGE_ID = ShortForeignKeyField.create(
Identifier.LANGUAGE_ID,
Film::getLanguageId,
Film::setLanguageId,
Language.LANGUAGE_ID,
TypeMapper.primitive(),
false
);
/**
* This Field corresponds to the {@link Film} field that can be obtained
* using the {@link Film#getOriginalLanguageId()} method.
*/
ComparableForeignKeyField ORIGINAL_LANGUAGE_ID = ComparableForeignKeyField.create(
Identifier.ORIGINAL_LANGUAGE_ID,
o -> OptionalUtil.unwrap(o.getOriginalLanguageId()),
Film::setOriginalLanguageId,
Language.LANGUAGE_ID,
TypeMapper.identity(),
false
);
/**
* This Field corresponds to the {@link Film} field that can be obtained
* using the {@link Film#getRentalDuration()} method.
*/
ShortField RENTAL_DURATION = ShortField.create(
Identifier.RENTAL_DURATION,
Film::getRentalDuration,
Film::setRentalDuration,
TypeMapper.primitive(),
false
);
/**
* This Field corresponds to the {@link Film} field that can be obtained
* using the {@link Film#getRentalRate()} method.
*/
ComparableField RENTAL_RATE = ComparableField.create(
Identifier.RENTAL_RATE,
Film::getRentalRate,
Film::setRentalRate,
TypeMapper.identity(),
false
);
/**
* This Field corresponds to the {@link Film} field that can be obtained
* using the {@link Film#getLength()} method.
*/
ComparableField LENGTH = ComparableField.create(
Identifier.LENGTH,
o -> OptionalUtil.unwrap(o.getLength()),
Film::setLength,
TypeMapper.identity(),
false
);
/**
* This Field corresponds to the {@link Film} field that can be obtained
* using the {@link Film#getReplacementCost()} method.
*/
ComparableField REPLACEMENT_COST = ComparableField.create(
Identifier.REPLACEMENT_COST,
Film::getReplacementCost,
Film::setReplacementCost,
TypeMapper.identity(),
false
);
/**
* This Field corresponds to the {@link Film} field that can be obtained
* using the {@link Film#getRating()} method.
*/
StringField RATING = StringField.create(
Identifier.RATING,
o -> OptionalUtil.unwrap(o.getRating()),
Film::setRating,
TypeMapper.identity(),
false
);
/**
* This Field corresponds to the {@link Film} field that can be obtained
* using the {@link Film#getSpecialFeatures()} method.
*/
StringField SPECIAL_FEATURES = StringField.create(
Identifier.SPECIAL_FEATURES,
o -> OptionalUtil.unwrap(o.getSpecialFeatures()),
Film::setSpecialFeatures,
TypeMapper.identity(),
false
);
/**
* This Field corresponds to the {@link Film} field that can be obtained
* using the {@link Film#getLastUpdate()} method.
*/
ComparableField LAST_UPDATE = ComparableField.create(
Identifier.LAST_UPDATE,
Film::getLastUpdate,
Film::setLastUpdate,
TypeMapper.identity(),
false
);
/**
* Returns the filmId of this Film. The filmId field corresponds to the
* database column db0.sakila.film.film_id.
*
* @return the filmId of this Film
*/
int getFilmId();
/**
* Returns the title of this Film. The title field corresponds to the
* database column db0.sakila.film.title.
*
* @return the title of this Film
*/
String getTitle();
/**
* Returns the description of this Film. The description field corresponds
* to the database column db0.sakila.film.description.
*
* @return the description of this Film
*/
Optional getDescription();
/**
* Returns the releaseYear of this Film. The releaseYear field corresponds
* to the database column db0.sakila.film.release_year.
*
* @return the releaseYear of this Film
*/
Optional getReleaseYear();
/**
* Returns the languageId of this Film. The languageId field corresponds to
* the database column db0.sakila.film.language_id.
*
* @return the languageId of this Film
*/
short getLanguageId();
/**
* Returns the originalLanguageId of this Film. The originalLanguageId field
* corresponds to the database column db0.sakila.film.original_language_id.
*
* @return the originalLanguageId of this Film
*/
Optional getOriginalLanguageId();
/**
* Returns the rentalDuration of this Film. The rentalDuration field
* corresponds to the database column db0.sakila.film.rental_duration.
*
* @return the rentalDuration of this Film
*/
short getRentalDuration();
/**
* Returns the rentalRate of this Film. The rentalRate field corresponds to
* the database column db0.sakila.film.rental_rate.
*
* @return the rentalRate of this Film
*/
BigDecimal getRentalRate();
/**
* Returns the length of this Film. The length field corresponds to the
* database column db0.sakila.film.length.
*
* @return the length of this Film
*/
OptionalInt getLength();
/**
* Returns the replacementCost of this Film. The replacementCost field
* corresponds to the database column db0.sakila.film.replacement_cost.
*
* @return the replacementCost of this Film
*/
BigDecimal getReplacementCost();
/**
* Returns the rating of this Film. The rating field corresponds to the
* database column db0.sakila.film.rating.
*
* @return the rating of this Film
*/
Optional getRating();
/**
* Returns the specialFeatures of this Film. The specialFeatures field
* corresponds to the database column db0.sakila.film.special_features.
*
* @return the specialFeatures of this Film
*/
Optional getSpecialFeatures();
/**
* Returns the lastUpdate of this Film. The lastUpdate field corresponds to
* the database column db0.sakila.film.last_update.
*
* @return the lastUpdate of this Film
*/
Timestamp getLastUpdate();
/**
* Sets the filmId of this Film. The filmId field corresponds to the
* database column db0.sakila.film.film_id.
*
* @param filmId to set of this Film
* @return this Film instance
*/
Film setFilmId(int filmId);
/**
* Sets the title of this Film. The title field corresponds to the database
* column db0.sakila.film.title.
*
* @param title to set of this Film
* @return this Film instance
*/
Film setTitle(String title);
/**
* Sets the description of this Film. The description field corresponds to
* the database column db0.sakila.film.description.
*
* @param description to set of this Film
* @return this Film instance
*/
Film setDescription(String description);
/**
* Sets the releaseYear of this Film. The releaseYear field corresponds to
* the database column db0.sakila.film.release_year.
*
* @param releaseYear to set of this Film
* @return this Film instance
*/
Film setReleaseYear(Date releaseYear);
/**
* Sets the languageId of this Film. The languageId field corresponds to the
* database column db0.sakila.film.language_id.
*
* @param languageId to set of this Film
* @return this Film instance
*/
Film setLanguageId(short languageId);
/**
* Sets the originalLanguageId of this Film. The originalLanguageId field
* corresponds to the database column db0.sakila.film.original_language_id.
*
* @param originalLanguageId to set of this Film
* @return this Film instance
*/
Film setOriginalLanguageId(Short originalLanguageId);
/**
* Sets the rentalDuration of this Film. The rentalDuration field
* corresponds to the database column db0.sakila.film.rental_duration.
*
* @param rentalDuration to set of this Film
* @return this Film instance
*/
Film setRentalDuration(short rentalDuration);
/**
* Sets the rentalRate of this Film. The rentalRate field corresponds to the
* database column db0.sakila.film.rental_rate.
*
* @param rentalRate to set of this Film
* @return this Film instance
*/
Film setRentalRate(BigDecimal rentalRate);
/**
* Sets the length of this Film. The length field corresponds to the
* database column db0.sakila.film.length.
*
* @param length to set of this Film
* @return this Film instance
*/
Film setLength(Integer length);
/**
* Sets the replacementCost of this Film. The replacementCost field
* corresponds to the database column db0.sakila.film.replacement_cost.
*
* @param replacementCost to set of this Film
* @return this Film instance
*/
Film setReplacementCost(BigDecimal replacementCost);
/**
* Sets the rating of this Film. The rating field corresponds to the
* database column db0.sakila.film.rating.
*
* @param rating to set of this Film
* @return this Film instance
*/
Film setRating(String rating);
/**
* Sets the specialFeatures of this Film. The specialFeatures field
* corresponds to the database column db0.sakila.film.special_features.
*
* @param specialFeatures to set of this Film
* @return this Film instance
*/
Film setSpecialFeatures(String specialFeatures);
/**
* Sets the lastUpdate of this Film. The lastUpdate field corresponds to the
* database column db0.sakila.film.last_update.
*
* @param lastUpdate to set of this Film
* @return this Film instance
*/
Film setLastUpdate(Timestamp lastUpdate);
/**
* Queries the specified manager for the referenced Language. If no such
* Language exists, an {@code NullPointerException} will be thrown.
*
* @param foreignManager the manager to query for the entity
* @return the foreign entity referenced
*/
Language findLanguageId(Manager foreignManager);
/**
* Queries the specified manager for the referenced Language. If no such
* Language exists, an {@code NullPointerException} will be thrown.
*
* @param foreignManager the manager to query for the entity
* @return the foreign entity referenced
*/
Optional findOriginalLanguageId(Manager foreignManager);
enum Identifier implements ColumnIdentifier {
FILM_ID ("film_id"),
TITLE ("title"),
DESCRIPTION ("description"),
RELEASE_YEAR ("release_year"),
LANGUAGE_ID ("language_id"),
ORIGINAL_LANGUAGE_ID ("original_language_id"),
RENTAL_DURATION ("rental_duration"),
RENTAL_RATE ("rental_rate"),
LENGTH ("length"),
REPLACEMENT_COST ("replacement_cost"),
RATING ("rating"),
SPECIAL_FEATURES ("special_features"),
LAST_UPDATE ("last_update");
private final String columnId;
private final TableIdentifier tableIdentifier;
Identifier(String columnId) {
this.columnId = columnId;
this.tableIdentifier = TableIdentifier.of( getDbmsId(),
getSchemaId(),
getTableId());
}
@Override
public String getDbmsId() {
return "db0";
}
@Override
public String getSchemaId() {
return "sakila";
}
@Override
public String getTableId() {
return "film";
}
@Override
public String getColumnId() {
return this.columnId;
}
@Override
public TableIdentifier asTableIdentifier() {
return this.tableIdentifier;
}
}
}