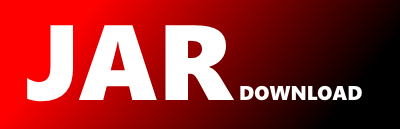
com.speedment.jpastreamer.field.internal.predicate.floats.FloatBetweenPredicate Maven / Gradle / Ivy
/*
* JPAstreamer - Express JPA queries with Java Streams
* Copyright (c) 2020-2020, Speedment, Inc. All Rights Reserved.
*
* License: GNU Lesser General Public License (LGPL), version 2.1 or later.
*
* This library is distributed in the hope that it will be useful, but WITHOUT ANY WARRANTY;
* without even the implied warranty of MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE.
* See the GNU Lesser General Public License for more details.
*
* See: https://github.com/speedment/jpa-streamer/blob/master/LICENSE
*/
package com.speedment.jpastreamer.field.internal.predicate.floats;
import com.speedment.jpastreamer.field.predicate.trait.HasInclusion;
import com.speedment.jpastreamer.field.trait.HasArg0;
import com.speedment.jpastreamer.field.trait.HasArg1;
import com.speedment.jpastreamer.field.trait.HasFloatValue;
import com.speedment.jpastreamer.field.internal.predicate.AbstractFieldPredicate;
import com.speedment.jpastreamer.field.predicate.Inclusion;
import com.speedment.jpastreamer.field.predicate.PredicateType;
import static java.util.Objects.requireNonNull;
/**
* A predicate that evaluates if a value is between two floats.
*
* @param entity type
*
* @author Emil Forslund
* @since 3.0.0
*/
public final class FloatBetweenPredicate
extends AbstractFieldPredicate>
implements HasInclusion,
HasArg0,
HasArg1 {
private final float start;
private final float end;
private final Inclusion inclusion;
public FloatBetweenPredicate(
HasFloatValue field,
float start,
float end,
Inclusion inclusion) {
super(PredicateType.BETWEEN, field, entity -> {
final float fieldValue = field.getAsFloat(entity);
switch (inclusion) {
case START_EXCLUSIVE_END_EXCLUSIVE :
return (start < fieldValue && end > fieldValue);
case START_EXCLUSIVE_END_INCLUSIVE :
return (start < fieldValue && end >= fieldValue);
case START_INCLUSIVE_END_EXCLUSIVE :
return (start <= fieldValue && end > fieldValue);
case START_INCLUSIVE_END_INCLUSIVE :
return (start <= fieldValue && end >= fieldValue);
default : throw new IllegalStateException("Inclusion unknown: " + inclusion);
}
});
this.start = start;
this.end = end;
this.inclusion = requireNonNull(inclusion);
}
@Override
public Float get0() {
return start;
}
@Override
public Float get1() {
return end;
}
@Override
public Inclusion getInclusion() {
return inclusion;
}
@Override
public FloatNotBetweenPredicate negate() {
return new FloatNotBetweenPredicate<>(getField(), start, end, inclusion);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy