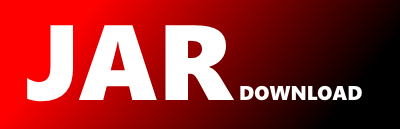
com.speedment.plugins.json.JsonEncoder Maven / Gradle / Ivy
Show all versions of json-stream Show documentation
/**
*
* Copyright (c) 2006-2018, Speedment, Inc. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"); You may not
* use this file except in compliance with the License. You may obtain a copy of
* the License at:
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS, WITHOUT
* WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. See the
* License for the specific language governing permissions and limitations under
* the License.
*/
package com.speedment.plugins.json;
import com.speedment.runtime.core.manager.Manager;
import com.speedment.runtime.field.*;
import com.speedment.runtime.field.method.*;
import com.speedment.runtime.field.trait.HasFinder;
import java.util.function.Function;
import java.util.stream.Stream;
/**
* An encoder that can transform Speedment entities to JSON.
*
* Example usage:
* {@code
* Manager
addresses = app.managerOf(Address.class);
* Manager employees = app.managerOf(Employee.class);
* Manager departments = app.managerOf(Department.class);
*
* // Include street, zip-code and city only.
* JconEncoder addrEncoder = JsonEncoder.noneOf(addresses)
* .add(Address.STREET)
* .add(Address.ZIPCODE)
* .add(Address.CITY);
*
* // Do not expose SSN but do inline the home address.
* JconEncoder empEncoder = JsonEncoder.allOf(employees)
* .remove(Employee.SSN)
* .remove(Employee.DEPARTMENT); // Go the other way around
* .add(Employee.HOME_ADDRESS, addrEncoder); // Foreign key
*
* // Inline every employee in the department.
* JconEncoder depEncoder = JsonEncoder.allOf(departments)
* .putStreamer("employees", Department::employees, empEncoder);
*
* String json = depEncoder.apply(departments.findAny().get());
* }
*
* @param entity type
*
* @author Emil Forslund
* @since 2.1.0
*/
public interface JsonEncoder {
/**************************************************************************/
/* Getters */
/**************************************************************************/
/**
* Returns the manager for the entity type that this encoder works with.
*
* @return the manager
*/
Manager getManager();
/**************************************************************************/
/* Field Putters */
/**************************************************************************/
/**
* Include the specified field in the encoder.
*
* @param the database type
* @param the java type
* @param field the field type
* @return a reference to this encoder
*/
JsonEncoder put(ReferenceField field);
/**
* Include the specified field in the encoder.
*
* @param the database type
* @param field the field type
* @return a reference to this encoder
*/
JsonEncoder putByte(ByteField field);
/**
* Include the specified field in the encoder.
*
* @param the database type
* @param field the field type
* @return a reference to this encoder
*/
JsonEncoder putShort(ShortField field);
/**
* Include the specified field in the encoder.
*
* @param the database type
* @param field the field type
* @return a reference to this encoder
*/
JsonEncoder putInt(IntField field);
/**
* Include the specified field in the encoder.
*
* @param the database type
* @param field the field type
* @return a reference to this encoder
*/
JsonEncoder putLong(LongField field);
/**
* Include the specified field in the encoder.
*
* @param the database type
* @param field the field type
* @return a reference to this encoder
*/
JsonEncoder putFloat(FloatField field);
/**
* Include the specified field in the encoder.
*
* @param the database type
* @param field the field type
* @return a reference to this encoder
*/
JsonEncoder putDouble(DoubleField field);
/**
* Include the specified field in the encoder.
*
* @param the database type
* @param field the field type
* @return a reference to this encoder
*/
JsonEncoder putChar(CharField field);
/**
* Include the specified field in the encoder.
*
* @param the database type
* @param field the field type
* @return a reference to this encoder
*/
JsonEncoder putBoolean(BooleanField field);
/**************************************************************************/
/* Put Labels with Getters */
/**************************************************************************/
/**
* Include the specified label in the encoder, using the specified getter to
* determine its value. If the label is the same as an existing field name,
* it will be replaced.
*
* @param the reference type of the field
* @param label the label to store it under
* @param getter how to retreive the value to store
* @return a reference to this encoder
*/
JsonEncoder put(String label, ReferenceGetter getter);
/**
* Include the specified label in the encoder, using the specified getter to
* determine its value. If the label is the same as an existing field name,
* it will be replaced.
*
* @param label the label to store it under
* @param getter how to retreive the value to store
* @return a reference to this encoder
*/
JsonEncoder putByte(String label, ByteGetter getter);
/**
* Include the specified label in the encoder, using the specified getter to
* determine its value. If the label is the same as an existing field name,
* it will be replaced.
*
* @param label the label to store it under
* @param getter how to retreive the value to store
* @return a reference to this encoder
*/
JsonEncoder putShort(String label, ShortGetter getter);
/**
* Include the specified label in the encoder, using the specified getter to
* determine its value. If the label is the same as an existing field name,
* it will be replaced.
*
* @param label the label to store it under
* @param getter how to retreive the value to store
* @return a reference to this encoder
*/
JsonEncoder putInt(String label, IntGetter getter);
/**
* Include the specified label in the encoder, using the specified getter to
* determine its value. If the label is the same as an existing field name,
* it will be replaced.
*
* @param label the label to store it under
* @param getter how to retreive the value to store
* @return a reference to this encoder
*/
JsonEncoder putLong(String label, LongGetter getter);
/**
* Include the specified label in the encoder, using the specified getter to
* determine its value. If the label is the same as an existing field name,
* it will be replaced.
*
* @param label the label to store it under
* @param getter how to retreive the value to store
* @return a reference to this encoder
*/
JsonEncoder putFloat(String label, FloatGetter getter);
/**
* Include the specified label in the encoder, using the specified getter to
* determine its value. If the label is the same as an existing field name,
* it will be replaced.
*
* @param label the label to store it under
* @param getter how to retreive the value to store
* @return a reference to this encoder
*/
JsonEncoder putDouble(String label, DoubleGetter getter);
/**
* Include the specified label in the encoder, using the specified getter to
* determine its value. If the label is the same as an existing field name,
* it will be replaced.
*
* @param label the label to store it under
* @param getter how to retreive the value to store
* @return a reference to this encoder
*/
JsonEncoder putChar(String label, CharGetter getter);
/**
* Include the specified label in the encoder, using the specified getter to
* determine its value. If the label is the same as an existing field name,
* it will be replaced.
*
* @param label the label to store it under
* @param getter how to retreive the value to store
* @return a reference to this encoder
*/
JsonEncoder putBoolean(String label, BooleanGetter getter);
/**************************************************************************/
/* Put Fields with Finders */
/**************************************************************************/
/**
* Include the specified foreign key field in the encoder, embedding foreign
* entities as inner JSON objects.
*
* @param the type of the foreign entity
* @param the type of the field itself
*
* @param field the foreign key field
* @param encoder encoder for the foreign entity
* @return a reference to this encoder
*/
& HasFinder>
JsonEncoder put(FIELD field, JsonEncoder encoder);
/**************************************************************************/
/* Put Labels with Finders */
/**************************************************************************/
/**
* Include the specified label in this encoder, populating it with an inner
* JSON object representing a foreign entity. This can be used to create
* custom object hierarchies. If the specified label already exists in the
* encoder, it will be replaced.
*
* @param the foreign entity type
* @param label the label to store it under
* @param finder a finder method used to retrieve the foreign entity
* @param fkEncoder an encoder for the foreign entity type
* @return a reference to this encoder
*/
JsonEncoder put(
String label,
FindFrom finder,
JsonEncoder fkEncoder);
/**************************************************************************/
/* Put Labels with Find Many */
/**************************************************************************/
/**
* Include the specified label in this encoder, using it to store an array
* of inner JSON objects determined by applying the specified streaming
* method. If the specified label already exists in the encoder, it will be
* replaced.
*
* @param the foreign entity type
* @param label the label to store it under
* @param streamer the streaming method to use
* @param fkEncoder encoder for the foreign entity type
* @return a reference to this encoder
*/
JsonEncoder putStreamer(
String label,
Function> streamer,
JsonEncoder fkEncoder);
/**
* Include the specified label in this encoder, using it to store an array
* of inner JSON objects determined by applying the specified streaming
* method. If the specified label already exists in the encoder, it will be
* replaced.
*
* @param the foreign entity type
* @param label the label to store it under
* @param streamer the streaming method to use
* @param fkEncoder encoder for the foreign entity type
* @return a reference to this encoder
*/
JsonEncoder putStreamer(
String label,
Function> streamer,
Function fkEncoder);
/**************************************************************************/
/* Remove by Label */
/**************************************************************************/
/**
* Exclude the specified label from this encoder. If the label was not
* already included, this method will have no effect.
*
* This method can be used to remove fields as well if the correct field
* name is specified.
*
* @param label the label to remove
* @return a reference to this encoder
*/
JsonEncoder remove(String label);
/**
* Exclude the specified field from this encoder. If the field was not
* already included in the encoder, this method has no effect.
*
* @param field the field to remove
* @return a reference to this encoder
*/
JsonEncoder remove(Field field);
/**************************************************************************/
/* Encode */
/**************************************************************************/
/**
* Encodes the specified entity using this encoder.
*
* @param entity the entity to encode
* @return the JSON encoded string
*/
String apply(ENTITY entity);
/**
* Returns a collector that will use this encoder to encode any incoming
* entities.
*
* @return the collector
*/
JsonCollector collector();
}