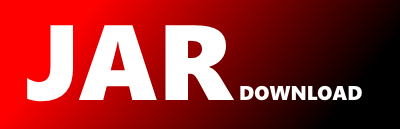
com.speedment.runtime.application.AbstractSpeedment Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of runtime-application Show documentation
Show all versions of runtime-application Show documentation
The runtime bundle of Speedment that all projects must depend on to use
the framework.
The newest version!
/*
*
* Copyright (c) 2006-2020, Speedment, Inc. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"); You may not
* use this file except in compliance with the License. You may obtain a copy of
* the License at:
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS, WITHOUT
* WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. See the
* License for the specific language governing permissions and limitations under
* the License.
*/
package com.speedment.runtime.application;
import com.speedment.common.injector.Injector;
import com.speedment.common.injector.State;
import com.speedment.common.injector.annotation.ExecuteBefore;
import com.speedment.common.logger.Logger;
import com.speedment.common.logger.LoggerManager;
import com.speedment.runtime.core.Speedment;
import com.speedment.runtime.core.component.StreamSupplierComponent;
import com.speedment.runtime.core.exception.SpeedmentException;
import com.speedment.runtime.core.manager.Manager;
import com.speedment.runtime.core.manager.ManagerConfigurator;
import java.util.Optional;
import static java.util.Objects.requireNonNull;
/**
* An abstract base implementation of the {@link Speedment} interface.
*
* @author Emil Forslund
* @since 3.0.0
*/
public abstract class AbstractSpeedment implements Speedment {
private static final Logger LOGGER = LoggerManager.getLogger(AbstractSpeedment.class);
private Injector injector;
protected AbstractSpeedment() {}
@ExecuteBefore(State.INITIALIZED)
public final void setInjector(Injector injector) {
this.injector = requireNonNull(injector);
}
@Override
public Optional get(Class type) {
return injector.get(type);
}
@Override
public T getOrThrow(Class componentClass) {
try {
return injector.getOrThrow(componentClass);
} catch (final Exception ex) {
logTypicalError(componentClass);
throw new SpeedmentException(
"Specified component '" + componentClass.getSimpleName()
+ "' is not installed in the platform.", ex
);
}
}
@Override
public ManagerConfigurator configure(Class extends Manager> managerClass) {
requireNonNull(managerClass);
return ManagerConfigurator.create(getOrThrow(StreamSupplierComponent.class), getOrThrow(managerClass));
}
@Override
public void stop() {
injector.stop();
}
private void logTypicalError(Class componentClass) {
final String componentSimpleName = componentClass.getSimpleName();
if ("JoinComponent".equals(componentSimpleName)) {
LOGGER.error(
"Since 3.2.0, The JoinBundle (that contains the JoinComponent) is optional. " +
"Make sure that you have installed the JoinBundle by invoking .withBundle(JoinBundle.class) in your application builder " +
"and add 'requires com.speedment.runtime.join;' in your module-info.java file (if any)."
);
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy