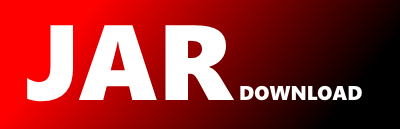
com.speedment.runtime.compute.internal.expression.CastUtil Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of runtime-compute Show documentation
Show all versions of runtime-compute Show documentation
Functional interfaces and utility class used to express computations on
Speedment entities.
/**
*
* Copyright (c) 2006-2019, Speedment, Inc. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"); You may not
* use this file except in compliance with the License. You may obtain a copy of
* the License at:
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS, WITHOUT
* WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. See the
* License for the specific language governing permissions and limitations under
* the License.
*/
package com.speedment.runtime.compute.internal.expression;
import com.speedment.runtime.compute.ToBigDecimal;
import com.speedment.runtime.compute.ToBoolean;
import com.speedment.runtime.compute.ToByte;
import com.speedment.runtime.compute.ToChar;
import com.speedment.runtime.compute.ToDouble;
import com.speedment.runtime.compute.ToFloat;
import com.speedment.runtime.compute.ToInt;
import com.speedment.runtime.compute.ToLong;
import com.speedment.runtime.compute.ToShort;
import com.speedment.runtime.compute.expression.Expression;
import com.speedment.runtime.compute.expression.UnaryExpression;
import java.util.Objects;
import static java.util.Objects.requireNonNull;
/**
* Utility class for creating expression that casts the result of another
* expression into some type, preserving as much metadata as possible.
*
* @author Emil Forslund
* @since 3.1.0
*/
public final class CastUtil {
////////////////////////////////////////////////////////////////////////////
// ToDouble //
////////////////////////////////////////////////////////////////////////////
/**
* Returns an expression that wraps the specified expression and casts the
* result from it into a {@code double}.
*
* @param original the original expression
* @param the input type
* @return the new {@link ToDouble} expression
*/
public static ToDouble castByteToDouble(ToByte original) {
class ByteToDouble extends CastToDouble> {
private ByteToDouble(ToByte tToByte) {
super(tToByte);
}
@Override
public double applyAsDouble(T object) {
return inner.applyAsByte(object);
}
}
return new ByteToDouble(original);
}
/**
* Returns an expression that wraps the specified expression and casts the
* result from it into a {@code double}.
*
* @param original the original expression
* @param the input type
* @return the new {@link ToDouble} expression
*/
public static ToDouble castShortToDouble(ToShort original) {
class ShortToDouble extends CastToDouble> {
private ShortToDouble(ToShort tToShort) {
super(tToShort);
}
@Override
public double applyAsDouble(T object) {
return inner.applyAsShort(object);
}
}
return new ShortToDouble(original);
}
/**
* Returns an expression that wraps the specified expression and casts the
* result from it into a {@code double}.
*
* @param original the original expression
* @param the input type
* @return the new {@link ToDouble} expression
*/
public static ToDouble castIntToDouble(ToInt original) {
class IntToDouble extends CastToDouble> {
private IntToDouble(ToInt tToInt) {
super(tToInt);
}
@Override
public double applyAsDouble(T object) {
return inner.applyAsInt(object);
}
}
return new IntToDouble(original);
}
/**
* Returns an expression that wraps the specified expression and casts the
* result from it into a {@code double}.
*
* @param original the original expression
* @param the input type
* @return the new {@link ToDouble} expression
*/
public static ToDouble castLongToDouble(ToLong original) {
class LongToDouble extends CastToDouble> {
private LongToDouble(ToLong tToLong) {
super(tToLong);
}
@Override
public double applyAsDouble(T object) {
return inner.applyAsLong(object);
}
}
return new LongToDouble(original);
}
/**
* Returns an expression that wraps the specified expression and casts the
* result from it into a {@code double}.
*
* @param original the original expression
* @param the input type
* @return the new {@link ToDouble} expression
*/
public static ToDouble castFloatToDouble(ToFloat original) {
class FloatToDouble extends CastToDouble> {
private FloatToDouble(ToFloat tToFloat) {
super(tToFloat);
}
@Override
public double applyAsDouble(T object) {
return inner.applyAsFloat(object);
}
}
return new FloatToDouble(original);
}
/**
* Returns an expression that wraps the specified expression and casts the
* result from it into a {@code double}.
*
* @param original the original expression
* @param the input type
* @return the new {@link ToDouble} expression
*/
public static ToDouble castBigDecimalToDouble(ToBigDecimal original) {
class BigDecimalToDouble extends CastToDouble> {
private BigDecimalToDouble(ToBigDecimal tToBigDecimal) {
super(tToBigDecimal);
}
@Override
public double applyAsDouble(T object) {
return inner.apply(object).doubleValue();
}
}
return new BigDecimalToDouble(original);
}
/**
* Returns an expression that wraps the specified expression and casts the
* result from it into a {@code double}.
*
* @param original the original expression
* @param the input type
* @return the new {@link ToDouble} expression
*/
public static ToDouble castBooleanToDouble(ToBoolean original) {
class BooleanToDouble extends CastToDouble> {
private BooleanToDouble(ToBoolean tToBoolean) {
super(tToBoolean);
}
@Override
public double applyAsDouble(T object) {
return inner.applyAsBoolean(object) ? 1 : 0;
}
}
return new BooleanToDouble(original);
}
/**
* Returns an expression that wraps the specified expression and casts the
* result from it into a {@code double}.
*
* @param original the original expression
* @param the input type
* @return the new {@link ToDouble} expression
*/
public static ToDouble castCharToDouble(ToChar original) {
class CharToDouble extends CastToDouble> {
private CharToDouble(ToChar tToChar) {
super(tToChar);
}
@Override
public double applyAsDouble(T object) {
return inner.applyAsChar(object);
}
}
return new CharToDouble(original);
}
////////////////////////////////////////////////////////////////////////////
// ToInt //
////////////////////////////////////////////////////////////////////////////
/**
* Returns an expression that wraps the specified expression and casts the
* result from it into a {@code int}.
*
* @param original the original expression
* @param the input type
* @return the new {@link ToInt} expression
*/
public static ToInt castByteToInt(ToByte original) {
class ByteToInt extends CastToInt> {
private ByteToInt(ToByte tToByte) {
super(tToByte);
}
@Override
public int applyAsInt(T object) {
return inner.applyAsByte(object);
}
}
return new ByteToInt(original);
}
/**
* Returns an expression that wraps the specified expression and casts the
* result from it into a {@code int}.
*
* @param original the original expression
* @param the input type
* @return the new {@link ToInt} expression
*/
public static ToInt castShortToInt(ToShort original) {
class ShortToInt extends CastToInt> {
private ShortToInt(ToShort tToShort) {
super(tToShort);
}
@Override
public int applyAsInt(T object) {
return inner.applyAsShort(object);
}
}
return new ShortToInt(original);
}
/**
* Returns an expression that wraps the specified expression and casts the
* result from it into a {@code int}.
*
* @param original the original expression
* @param the input type
* @return the new {@link ToInt} expression
*/
public static ToInt castLongToInt(ToLong original) {
class LongToInt extends CastToInt> {
private LongToInt(ToLong tToLong) {
super(tToLong);
}
@Override
public int applyAsInt(T object) {
return (int) inner.applyAsLong(object);
}
}
return new LongToInt(original);
}
/**
* Returns an expression that wraps the specified expression and casts the
* result from it into a {@code int}.
*
* @param original the original expression
* @param the input type
* @return the new {@link ToInt} expression
*/
public static ToInt castFloatToInt(ToFloat original) {
class FloatToInt extends CastToInt> {
private FloatToInt(ToFloat tToFloat) {
super(tToFloat);
}
@Override
public int applyAsInt(T object) {
return (int) inner.applyAsFloat(object);
}
}
return new FloatToInt(original);
}
/**
* Returns an expression that wraps the specified expression and casts the
* result from it into a {@code int}.
*
* @param original the original expression
* @param the input type
* @return the new {@link ToInt} expression
*/
public static ToInt castDoubleToInt(ToDouble original) {
class DoubleToInt extends CastToInt> {
private DoubleToInt(ToDouble tToDouble) {
super(tToDouble);
}
@Override
public int applyAsInt(T object) {
return (int) inner.applyAsDouble(object);
}
}
return new DoubleToInt(original);
}
/**
* Returns an expression that wraps the specified expression and casts the
* result from it into a {@code int}.
*
* @param original the original expression
* @param the input type
* @return the new {@link ToInt} expression
*/
public static ToInt castBigDecimalToInt(ToBigDecimal original) {
class BigDecimalToInt extends CastToInt> {
private BigDecimalToInt(ToBigDecimal tToBigDecimal) {
super(tToBigDecimal);
}
@Override
public int applyAsInt(T object) {
return inner.apply(object).intValueExact();
}
}
return new BigDecimalToInt(original);
}
/**
* Returns an expression that wraps the specified expression and casts the
* result from it into a {@code int}.
*
* @param original the original expression
* @param the input type
* @return the new {@link ToInt} expression
*/
public static ToInt castBooleanToInt(ToBoolean original) {
class BooleanToInt extends CastToInt> {
private BooleanToInt(ToBoolean tToBoolean) {
super(tToBoolean);
}
@Override
public int applyAsInt(T object) {
return inner.applyAsBoolean(object) ? 1 : 0;
}
}
return new BooleanToInt(original);
}
/**
* Returns an expression that wraps the specified expression and casts the
* result from it into a {@code int}.
*
* @param original the original expression
* @param the input type
* @return the new {@link ToInt} expression
*/
public static ToInt castCharToInt(ToChar original) {
class CharToInt extends CastToInt> {
private CharToInt(ToChar tToChar) {
super(tToChar);
}
@Override
public int applyAsInt(T object) {
return inner.applyAsChar(object);
}
}
return new CharToInt(original);
}
////////////////////////////////////////////////////////////////////////////
// ToLong //
////////////////////////////////////////////////////////////////////////////
/**
* Returns an expression that wraps the specified expression and casts the
* result from it into a {@code long}.
*
* @param original the original expression
* @param the input type
* @return the new {@link ToLong} expression
*/
public static ToLong castByteToLong(ToByte original) {
class ByteToLong extends CastToLong> {
private ByteToLong(ToByte tToByte) {
super(tToByte);
}
@Override
public long applyAsLong(T object) {
return inner.applyAsByte(object);
}
}
return new ByteToLong(original);
}
/**
* Returns an expression that wraps the specified expression and casts the
* result from it into a {@code long}.
*
* @param original the original expression
* @param the input type
* @return the new {@link ToLong} expression
*/
public static ToLong castShortToLong(ToShort original) {
class ShortToLong extends CastToLong> {
private ShortToLong(ToShort tToShort) {
super(tToShort);
}
@Override
public long applyAsLong(T object) {
return inner.applyAsShort(object);
}
}
return new ShortToLong(original);
}
/**
* Returns an expression that wraps the specified expression and casts the
* result from it into a {@code long}.
*
* @param original the original expression
* @param the input type
* @return the new {@link ToLong} expression
*/
public static ToLong castIntToLong(ToInt original) {
class IntToLong extends CastToLong> {
private IntToLong(ToInt tToInt) {
super(tToInt);
}
@Override
public long applyAsLong(T object) {
return inner.applyAsInt(object);
}
}
return new IntToLong(original);
}
/**
* Returns an expression that wraps the specified expression and casts the
* result from it into a {@code long}.
*
* @param original the original expression
* @param the input type
* @return the new {@link ToLong} expression
*/
public static ToLong castFloatToLong(ToFloat original) {
class FloatToLong extends CastToLong> {
private FloatToLong(ToFloat tToFloat) {
super(tToFloat);
}
@Override
public long applyAsLong(T object) {
return (long) inner.applyAsFloat(object);
}
}
return new FloatToLong(original);
}
/**
* Returns an expression that wraps the specified expression and casts the
* result from it into a {@code long}.
*
* @param original the original expression
* @param the input type
* @return the new {@link ToLong} expression
*/
public static ToLong castDoubleToLong(ToDouble original) {
class DoubleToLong extends CastToLong> {
private DoubleToLong(ToDouble tToDouble) {
super(tToDouble);
}
@Override
public long applyAsLong(T object) {
return (long) inner.applyAsDouble(object);
}
}
return new DoubleToLong(original);
}
/**
* Returns an expression that wraps the specified expression and casts the
* result from it into a {@code long}.
*
* @param original the original expression
* @param the input type
* @return the new {@link ToInt} expression
*/
public static ToLong castBigDecimalToLong(ToBigDecimal original) {
class BigDecimalToLong extends CastToLong> {
private BigDecimalToLong(ToBigDecimal tToBigDecimal) {
super(tToBigDecimal);
}
@Override
public long applyAsLong(T object) {
return inner.apply(object).longValueExact();
}
}
return new BigDecimalToLong(original);
}
/**
* Returns an expression that wraps the specified expression and casts the
* result from it into a {@code long}.
*
* @param original the original expression
* @param the input type
* @return the new {@link ToLong} expression
*/
public static ToLong castBooleanToLong(ToBoolean original) {
class BooleanToLong extends CastToLong> {
private BooleanToLong(ToBoolean tToBoolean) {
super(tToBoolean);
}
@Override
public long applyAsLong(T object) {
return inner.applyAsBoolean(object) ? 1 : 0;
}
}
return new BooleanToLong(original);
}
/**
* Returns an expression that wraps the specified expression and casts the
* result from it into a {@code long}.
*
* @param original the original expression
* @param the input type
* @return the new {@link ToLong} expression
*/
public static ToLong castCharToLong(ToChar original) {
class CharToLong extends CastToLong> {
private CharToLong(ToChar tToChar) {
super(tToChar);
}
@Override
public long applyAsLong(T object) {
return inner.applyAsChar(object);
}
}
return new CharToLong(original);
}
////////////////////////////////////////////////////////////////////////////
// Internal //
////////////////////////////////////////////////////////////////////////////
/**
* Internal abstract implementation of the cast operation that casts to a
* {@code double}.
*
* @param the input type
* @param the inner expression type
*/
private abstract static class CastToDouble>
extends AbstractCast implements ToDouble {
CastToDouble(INNER inner) {
super(inner);
}
}
/**
* Internal abstract implementation of the cast operation that casts to an
* {@code int}.
*
* @param the input type
* @param the inner expression type
*/
private abstract static class CastToInt>
extends AbstractCast implements ToInt {
CastToInt(INNER inner) {
super(inner);
}
}
/**
* Internal abstract implementation of the cast operation that casts to a
* {@code long}.
*
* @param the input type
* @param the inner expression type
*/
private abstract static class CastToLong>
extends AbstractCast implements ToLong {
CastToLong(INNER inner) {
super(inner);
}
}
/**
* Internal abstract implementation of the cast operation.
*
* @param the input entity type
* @param the inner expression type
*/
private abstract static class AbstractCast>
implements UnaryExpression {
final INNER inner;
AbstractCast(INNER inner) {
this.inner = requireNonNull(inner);
}
@Override
public final INNER inner() {
return inner;
}
@Override
public final Operator operator() {
return Operator.CAST;
}
@Override
public final boolean equals(Object o) {
if (this == o) return true;
else if (!(o instanceof UnaryExpression)) return false;
final UnaryExpression, ?> that = (UnaryExpression, ?>) o;
return Objects.equals(inner(), that.inner())
&& operator().equals(that.operator());
}
@Override
public final int hashCode() {
return Objects.hash(inner(), operator());
}
}
/**
* Utility classes should not be instantiated.
*/
private CastUtil() {}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy