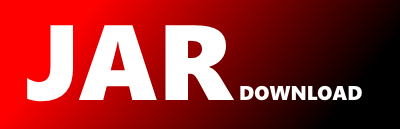
com.speedment.runtime.compute.internal.expression.DivideUtil Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of runtime-compute Show documentation
Show all versions of runtime-compute Show documentation
Functional interfaces and utility class used to express computations on
Speedment entities.
/**
*
* Copyright (c) 2006-2019, Speedment, Inc. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"); You may not
* use this file except in compliance with the License. You may obtain a copy of
* the License at:
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS, WITHOUT
* WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. See the
* License for the specific language governing permissions and limitations under
* the License.
*/
package com.speedment.runtime.compute.internal.expression;
import com.speedment.runtime.compute.ToByte;
import com.speedment.runtime.compute.ToDouble;
import com.speedment.runtime.compute.ToFloat;
import com.speedment.runtime.compute.ToInt;
import com.speedment.runtime.compute.ToLong;
import com.speedment.runtime.compute.ToShort;
import com.speedment.runtime.compute.expression.BinaryExpression;
import com.speedment.runtime.compute.expression.BinaryObjExpression;
import com.speedment.runtime.compute.expression.Expression;
import java.util.Objects;
import static java.util.Objects.requireNonNull;
/**
* Utility class for creating expressions that computes the result of a wrapped
* expression divided by either a constant or another expression.
*
* @author Emil Forslund
* @since 3.1.0
*/
public final class DivideUtil {
////////////////////////////////////////////////////////////////////////////
// ToByte //
////////////////////////////////////////////////////////////////////////////
/**
* Returns an expression that takes the result from the first expression and
* divides it with the result of the second expression. The second
* expression must never return {@code 0}!
*
* @param first the first expression
* @param second the second expression
* @param the input entity type
* @return the division expression
*/
public static ToDouble byteDivideInt(ToByte first, int second) {
return new DivideObjToDouble, Integer>(first) {
@Override
public double applyAsDouble(T object) {
return (double) this.first.applyAsByte(object)
/ (double) second;
}
@Override
public Integer second() {
return second;
}
};
}
/**
* Returns an expression that takes the result from the first expression and
* divides it with the result of the second expression. The second
* expression must never return {@code 0}!
*
* @param first the first expression
* @param second the second expression
* @param the input entity type
* @return the division expression
*/
public static ToDouble byteDivideLong(ToByte first, long second) {
return new DivideObjToDouble, Long>(first) {
@Override
public double applyAsDouble(T object) {
return (double) this.first.applyAsByte(object)
/ (double) second;
}
@Override
public Long second() {
return second;
}
};
}
/**
* Returns an expression that takes the result from the first expression and
* divides it with the result of the second expression. The second
* expression must never return {@code 0}!
*
* @param first the first expression
* @param second the second expression
* @param the input entity type
* @return the division expression
*/
public static ToDouble byteDivideDouble(ToByte first, double second) {
return new DivideObjToDouble, Double>(first) {
@Override
public double applyAsDouble(T object) {
return (double) this.first.applyAsByte(object) / second;
}
@Override
public Double second() {
return second;
}
};
}
/**
* Returns an expression that takes the result from the first expression and
* divides it with the result of the second expression. The second
* expression must never return {@code 0}!
*
* @param first the first expression
* @param second the second expression
* @param the input entity type
* @return the division expression
*/
public static ToDouble byteDivideInt(ToByte first, ToInt second) {
return new DivideToDouble, ToInt>(first, second) {
@Override
public double applyAsDouble(T object) {
return (double) this.first.applyAsByte(object)
/ (double) this.second.applyAsInt(object);
}
};
}
/**
* Returns an expression that takes the result from the first expression and
* divides it with the result of the second expression. The second
* expression must never return {@code 0}!
*
* @param first the first expression
* @param second the second expression
* @param the input entity type
* @return the division expression
*/
public static ToDouble byteDivideLong(ToByte first, ToLong second) {
return new DivideToDouble, ToLong>(first, second) {
@Override
public double applyAsDouble(T object) {
return (double) this.first.applyAsByte(object)
/ (double) this.second.applyAsLong(object);
}
};
}
/**
* Returns an expression that takes the result from the first expression and
* divides it with the result of the second expression. The second
* expression must never return {@code 0}!
*
* @param first the first expression
* @param second the second expression
* @param the input entity type
* @return the division expression
*/
public static ToDouble byteDivideDouble(ToByte first, ToDouble second) {
return new DivideToDouble, ToDouble>(first, second) {
@Override
public double applyAsDouble(T object) {
return (double) this.first.applyAsByte(object)
/ this.second.applyAsDouble(object);
}
};
}
////////////////////////////////////////////////////////////////////////////
// ToShort //
////////////////////////////////////////////////////////////////////////////
/**
* Returns an expression that takes the result from the first expression and
* divides it with the result of the second expression. The second
* expression must never return {@code 0}!
*
* @param first the first expression
* @param second the second expression
* @param the input entity type
* @return the division expression
*/
public static ToDouble shortDivideInt(ToShort first, int second) {
return new DivideObjToDouble, Integer>(first) {
@Override
public double applyAsDouble(T object) {
return (double) this.first.applyAsShort(object)
/ (double) second;
}
@Override
public Integer second() {
return second;
}
};
}
/**
* Returns an expression that takes the result from the first expression and
* divides it with the result of the second expression. The second
* expression must never return {@code 0}!
*
* @param first the first expression
* @param second the second expression
* @param the input entity type
* @return the division expression
*/
public static ToDouble shortDivideLong(ToShort first, long second) {
return new DivideObjToDouble, Long>(first) {
@Override
public double applyAsDouble(T object) {
return (double) this.first.applyAsShort(object)
/ (double) second;
}
@Override
public Long second() {
return second;
}
};
}
/**
* Returns an expression that takes the result from the first expression and
* divides it with the result of the second expression. The second
* expression must never return {@code 0}!
*
* @param first the first expression
* @param second the second expression
* @param the input entity type
* @return the division expression
*/
public static ToDouble shortDivideDouble(ToShort first, double second) {
return new DivideObjToDouble, Double>(first) {
@Override
public double applyAsDouble(T object) {
return (double) this.first.applyAsShort(object) / second;
}
@Override
public Double second() {
return second;
}
};
}
/**
* Returns an expression that takes the result from the first expression and
* divides it with the result of the second expression. The second
* expression must never return {@code 0}!
*
* @param first the first expression
* @param second the second expression
* @param the input entity type
* @return the division expression
*/
public static ToDouble shortDivideInt(ToShort first, ToInt second) {
return new DivideToDouble, ToInt>(first, second) {
@Override
public double applyAsDouble(T object) {
return (double) this.first.applyAsShort(object)
/ (double) this.second.applyAsInt(object);
}
};
}
/**
* Returns an expression that takes the result from the first expression and
* divides it with the result of the second expression. The second
* expression must never return {@code 0}!
*
* @param first the first expression
* @param second the second expression
* @param the input entity type
* @return the division expression
*/
public static ToDouble shortDivideLong(ToShort first, ToLong second) {
return new DivideToDouble, ToLong>(first, second) {
@Override
public double applyAsDouble(T object) {
return (double) this.first.applyAsShort(object)
/ (double) this.second.applyAsLong(object);
}
};
}
/**
* Returns an expression that takes the result from the first expression and
* divides it with the result of the second expression. The second
* expression must never return {@code 0}!
*
* @param first the first expression
* @param second the second expression
* @param the input entity type
* @return the division expression
*/
public static ToDouble shortDivideDouble(ToShort first, ToDouble second) {
return new DivideToDouble, ToDouble>(first, second) {
@Override
public double applyAsDouble(T object) {
return (double) this.first.applyAsShort(object)
/ this.second.applyAsDouble(object);
}
};
}
////////////////////////////////////////////////////////////////////////////
// ToInt //
////////////////////////////////////////////////////////////////////////////
/**
* Returns an expression that takes the result from the first expression and
* divides it with the result of the second expression. The second
* expression must never return {@code 0}!
*
* @param first the first expression
* @param second the second expression
* @param the input entity type
* @return the division expression
*/
public static ToDouble intDivideInt(ToInt first, int second) {
return new DivideObjToDouble, Integer>(first) {
@Override
public double applyAsDouble(T object) {
return (double) this.first.applyAsInt(object)
/ (double) second;
}
@Override
public Integer second() {
return second;
}
};
}
/**
* Returns an expression that takes the result from the first expression and
* divides it with the result of the second expression. The second
* expression must never return {@code 0}!
*
* @param first the first expression
* @param second the second expression
* @param the input entity type
* @return the division expression
*/
public static ToDouble intDivideLong(ToInt first, long second) {
return new DivideObjToDouble, Long>(first) {
@Override
public double applyAsDouble(T object) {
return (double) this.first.applyAsInt(object)
/ (double) second;
}
@Override
public Long second() {
return second;
}
};
}
/**
* Returns an expression that takes the result from the first expression and
* divides it with the result of the second expression. The second
* expression must never return {@code 0}!
*
* @param first the first expression
* @param second the second expression
* @param the input entity type
* @return the division expression
*/
public static ToDouble intDivideDouble(ToInt first, double second) {
return new DivideObjToDouble, Double>(first) {
@Override
public double applyAsDouble(T object) {
return (double) this.first.applyAsInt(object) / second;
}
@Override
public Double second() {
return second;
}
};
}
/**
* Returns an expression that takes the result from the first expression and
* divides it with the result of the second expression. The second
* expression must never return {@code 0}!
*
* @param first the first expression
* @param second the second expression
* @param the input entity type
* @return the division expression
*/
public static ToDouble intDivideInt(ToInt first, ToInt second) {
return new DivideToDouble, ToInt>(first, second) {
@Override
public double applyAsDouble(T object) {
return (double) this.first.applyAsInt(object)
/ (double) this.second.applyAsInt(object);
}
};
}
/**
* Returns an expression that takes the result from the first expression and
* divides it with the result of the second expression. The second
* expression must never return {@code 0}!
*
* @param first the first expression
* @param second the second expression
* @param the input entity type
* @return the division expression
*/
public static ToDouble intDivideLong(ToInt first, ToLong second) {
return new DivideToDouble, ToLong>(first, second) {
@Override
public double applyAsDouble(T object) {
return (double) this.first.applyAsInt(object)
/ (double) this.second.applyAsLong(object);
}
};
}
/**
* Returns an expression that takes the result from the first expression and
* divides it with the result of the second expression. The second
* expression must never return {@code 0}!
*
* @param first the first expression
* @param second the second expression
* @param the input entity type
* @return the division expression
*/
public static ToDouble intDivideDouble(ToInt first, ToDouble second) {
return new DivideToDouble, ToDouble>(first, second) {
@Override
public double applyAsDouble(T object) {
return (double) this.first.applyAsInt(object)
/ this.second.applyAsDouble(object);
}
};
}
////////////////////////////////////////////////////////////////////////////
// ToLong //
////////////////////////////////////////////////////////////////////////////
/**
* Returns an expression that takes the result from the first expression and
* divides it with the result of the second expression. The second
* expression must never return {@code 0}!
*
* @param first the first expression
* @param second the second expression
* @param the input entity type
* @return the division expression
*/
public static ToDouble longDivideInt(ToLong first, int second) {
return new DivideObjToDouble, Integer>(first) {
@Override
public double applyAsDouble(T object) {
return (double) this.first.applyAsLong(object)
/ (double) second;
}
@Override
public Integer second() {
return second;
}
};
}
/**
* Returns an expression that takes the result from the first expression and
* divides it with the result of the second expression. The second
* expression must never return {@code 0}!
*
* @param first the first expression
* @param second the second expression
* @param the input entity type
* @return the division expression
*/
public static ToDouble longDivideLong(ToLong first, long second) {
return new DivideObjToDouble, Long>(first) {
@Override
public double applyAsDouble(T object) {
return (double) this.first.applyAsLong(object)
/ (double) second;
}
@Override
public Long second() {
return second;
}
};
}
/**
* Returns an expression that takes the result from the first expression and
* divides it with the result of the second expression. The second
* expression must never return {@code 0}!
*
* @param first the first expression
* @param second the second expression
* @param the input entity type
* @return the division expression
*/
public static ToDouble longDivideDouble(ToLong first, double second) {
return new DivideObjToDouble, Double>(first) {
@Override
public double applyAsDouble(T object) {
return (double) this.first.applyAsLong(object) / second;
}
@Override
public Double second() {
return second;
}
};
}
/**
* Returns an expression that takes the result from the first expression and
* divides it with the result of the second expression. The second
* expression must never return {@code 0}!
*
* @param first the first expression
* @param second the second expression
* @param the input entity type
* @return the division expression
*/
public static ToDouble longDivideInt(ToLong first, ToInt second) {
return new DivideToDouble, ToInt>(first, second) {
@Override
public double applyAsDouble(T object) {
return (double) this.first.applyAsLong(object)
/ (double) this.second.applyAsInt(object);
}
};
}
/**
* Returns an expression that takes the result from the first expression and
* divides it with the result of the second expression. The second
* expression must never return {@code 0}!
*
* @param first the first expression
* @param second the second expression
* @param the input entity type
* @return the division expression
*/
public static ToDouble longDivideLong(ToLong first, ToLong second) {
return new DivideToDouble, ToLong>(first, second) {
@Override
public double applyAsDouble(T object) {
return (double) this.first.applyAsLong(object)
/ (double) this.second.applyAsLong(object);
}
};
}
/**
* Returns an expression that takes the result from the first expression and
* divides it with the result of the second expression. The second
* expression must never return {@code 0}!
*
* @param first the first expression
* @param second the second expression
* @param the input entity type
* @return the division expression
*/
public static ToDouble longDivideDouble(ToLong first, ToDouble second) {
return new DivideToDouble, ToDouble>(first, second) {
@Override
public double applyAsDouble(T object) {
return (double) this.first.applyAsLong(object)
/ this.second.applyAsDouble(object);
}
};
}
////////////////////////////////////////////////////////////////////////////
// ToFloat //
////////////////////////////////////////////////////////////////////////////
/**
* Returns an expression that takes the result from the first expression and
* divides it with the result of the second expression. The second
* expression must never return {@code 0}!
*
* @param first the first expression
* @param second the second expression
* @param the input entity type
* @return the division expression
*/
public static ToDouble floatDivideInt(ToFloat first, int second) {
return new DivideObjToDouble, Integer>(first) {
@Override
public double applyAsDouble(T object) {
return (double) this.first.applyAsFloat(object)
/ (double) second;
}
@Override
public Integer second() {
return second;
}
};
}
/**
* Returns an expression that takes the result from the first expression and
* divides it with the result of the second expression. The second
* expression must never return {@code 0}!
*
* @param first the first expression
* @param second the second expression
* @param the input entity type
* @return the division expression
*/
public static ToDouble floatDivideLong(ToFloat first, long second) {
return new DivideObjToDouble, Long>(first) {
@Override
public double applyAsDouble(T object) {
return (double) this.first.applyAsFloat(object)
/ (double) second;
}
@Override
public Long second() {
return second;
}
};
}
/**
* Returns an expression that takes the result from the first expression and
* divides it with the result of the second expression. The second
* expression must never return {@code 0}!
*
* @param first the first expression
* @param second the second expression
* @param the input entity type
* @return the division expression
*/
public static ToDouble floatDivideDouble(ToFloat first, double second) {
return new DivideObjToDouble, Double>(first) {
@Override
public double applyAsDouble(T object) {
return (double) this.first.applyAsFloat(object) / second;
}
@Override
public Double second() {
return second;
}
};
}
/**
* Returns an expression that takes the result from the first expression and
* divides it with the result of the second expression. The second
* expression must never return {@code 0}!
*
* @param first the first expression
* @param second the second expression
* @param the input entity type
* @return the division expression
*/
public static ToDouble floatDivideInt(ToFloat first, ToInt second) {
return new DivideToDouble, ToInt>(first, second) {
@Override
public double applyAsDouble(T object) {
return (double) this.first.applyAsFloat(object)
/ (double) this.second.applyAsInt(object);
}
};
}
/**
* Returns an expression that takes the result from the first expression and
* divides it with the result of the second expression. The second
* expression must never return {@code 0}!
*
* @param first the first expression
* @param second the second expression
* @param the input entity type
* @return the division expression
*/
public static ToDouble floatDivideLong(ToFloat first, ToLong second) {
return new DivideToDouble, ToLong>(first, second) {
@Override
public double applyAsDouble(T object) {
return (double) this.first.applyAsFloat(object)
/ (double) this.second.applyAsLong(object);
}
};
}
/**
* Returns an expression that takes the result from the first expression and
* divides it with the result of the second expression. The second
* expression must never return {@code 0}!
*
* @param first the first expression
* @param second the second expression
* @param the input entity type
* @return the division expression
*/
public static ToDouble floatDivideDouble(ToFloat first, ToDouble second) {
return new DivideToDouble, ToDouble>(first, second) {
@Override
public double applyAsDouble(T object) {
return (double) this.first.applyAsFloat(object)
/ this.second.applyAsDouble(object);
}
};
}
////////////////////////////////////////////////////////////////////////////
// ToDouble //
////////////////////////////////////////////////////////////////////////////
/**
* Returns an expression that takes the result from the first expression and
* divides it with the result of the second expression. The second
* expression must never return {@code 0}!
*
* @param first the first expression
* @param second the second expression
* @param the input entity type
* @return the division expression
*/
public static ToDouble doubleDivideInt(ToDouble first, int second) {
return new DivideObjToDouble, Integer>(first) {
@Override
public double applyAsDouble(T object) {
return this.first.applyAsDouble(object)
/ (double) second;
}
@Override
public Integer second() {
return second;
}
};
}
/**
* Returns an expression that takes the result from the first expression and
* divides it with the result of the second expression. The second
* expression must never return {@code 0}!
*
* @param first the first expression
* @param second the second expression
* @param the input entity type
* @return the division expression
*/
public static ToDouble doubleDivideLong(ToDouble first, long second) {
return new DivideObjToDouble, Long>(first) {
@Override
public double applyAsDouble(T object) {
return this.first.applyAsDouble(object)
/ (double) second;
}
@Override
public Long second() {
return second;
}
};
}
/**
* Returns an expression that takes the result from the first expression and
* divides it with the result of the second expression. The second
* expression must never return {@code 0}!
*
* @param first the first expression
* @param second the second expression
* @param the input entity type
* @return the division expression
*/
public static ToDouble doubleDivideDouble(ToDouble first, double second) {
return new DivideObjToDouble, Double>(first) {
@Override
public double applyAsDouble(T object) {
return this.first.applyAsDouble(object) / second;
}
@Override
public Double second() {
return second;
}
};
}
/**
* Returns an expression that takes the result from the first expression and
* divides it with the result of the second expression. The second
* expression must never return {@code 0}!
*
* @param first the first expression
* @param second the second expression
* @param the input entity type
* @return the division expression
*/
public static ToDouble doubleDivideInt(ToDouble first, ToInt second) {
return new DivideToDouble, ToInt>(first, second) {
@Override
public double applyAsDouble(T object) {
return this.first.applyAsDouble(object)
/ (double) this.second.applyAsInt(object);
}
};
}
/**
* Returns an expression that takes the result from the first expression and
* divides it with the result of the second expression. The second
* expression must never return {@code 0}!
*
* @param first the first expression
* @param second the second expression
* @param the input entity type
* @return the division expression
*/
public static ToDouble doubleDivideLong(ToDouble first, ToLong second) {
return new DivideToDouble, ToLong>(first, second) {
@Override
public double applyAsDouble(T object) {
return this.first.applyAsDouble(object)
/ (double) this.second.applyAsLong(object);
}
};
}
/**
* Returns an expression that takes the result from the first expression and
* divides it with the result of the second expression. The second
* expression must never return {@code 0}!
*
* @param first the first expression
* @param second the second expression
* @param the input entity type
* @return the division expression
*/
public static ToDouble doubleDivideDouble(ToDouble first, ToDouble second) {
return new DivideToDouble, ToDouble>(first, second) {
@Override
public double applyAsDouble(T object) {
return this.first.applyAsDouble(object)
/ this.second.applyAsDouble(object);
}
};
}
////////////////////////////////////////////////////////////////////////////
// Internal Base Implementations //
////////////////////////////////////////////////////////////////////////////
/**
* Abstract base for a division operation that takes two other expressions
* and returns a {@code double} value.
*
* @param the input entity type
* @param the first expression type
* @param the second expression type
*/
private static abstract class DivideToDouble
, SECOND extends Expression>
extends Divide implements ToDouble {
DivideToDouble(FIRST first, SECOND second) {
super(first, second);
}
@Override
public Operator operator() {
return Operator.DIVIDE;
}
}
/**
* Abstract base for a division operation that takes two other expressions.
*
* @param the input type
* @param the first expression type
* @param the second expression type
*/
private static abstract class Divide
, SECOND extends Expression>
implements BinaryExpression {
final FIRST first;
final SECOND second;
Divide(FIRST first, SECOND second) {
this.first = requireNonNull(first);
this.second = requireNonNull(second);
}
@Override
public FIRST first() {
return first;
}
@Override
public SECOND second() {
return second;
}
@Override
public boolean equals(Object o) {
if (this == o) return true;
if (!(o instanceof BinaryExpression)) return false;
final BinaryExpression, ?, ?> divide = (BinaryExpression, ?, ?>) o;
return Objects.equals(first(), divide.first()) &&
Objects.equals(second(), divide.second()) &&
Objects.equals(operator(), divide.operator());
}
@Override
public int hashCode() {
return Objects.hash(first(), second(), operator());
}
}
/**
* Abstract base for a division operation that takes two other expressions
* and returns a {@code double} value.
*
* @param the input entity type
* @param the first expression type
* @param the second expression type
*/
private static abstract class DivideObjToDouble
, N>
extends DivideObj implements ToDouble {
DivideObjToDouble(INNER first) {
super(first);
}
@Override
public Operator operator() {
return Operator.DIVIDE;
}
@Override
public final boolean equals(Object o) {
if (o == null) return false;
else if (this == o) return true;
else if (!(o instanceof BinaryObjExpression)) return false;
final BinaryObjExpression, ?, ?> that = (BinaryObjExpression, ?, ?>) o;
return Objects.equals(first, that.first()) &&
Objects.equals(second(), that.second()) &&
Objects.equals(operator(), that.operator());
}
@Override
public final int hashCode() {
return Objects.hash(first, second(), operator());
}
}
/**
* Abstract base for a division operation that takes two other expressions.
*
* @param the input type
* @param the type of the expression to divide
* @param the value to divide with
*/
private static abstract class DivideObj
, N>
implements BinaryObjExpression {
final INNER first;
DivideObj(INNER first) {
this.first = requireNonNull(first);
}
@Override
public final INNER first() {
return first;
}
}
/**
* Utility classes should not be instantiated.
*/
private DivideUtil() {}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy