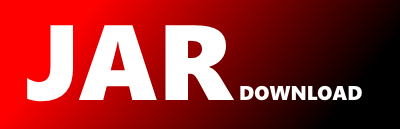
com.speedment.runtime.compute.internal.expression.MinusUtil Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of runtime-compute Show documentation
Show all versions of runtime-compute Show documentation
Functional interfaces and utility class used to express computations on
Speedment entities.
/**
*
* Copyright (c) 2006-2019, Speedment, Inc. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"); You may not
* use this file except in compliance with the License. You may obtain a copy of
* the License at:
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS, WITHOUT
* WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. See the
* License for the specific language governing permissions and limitations under
* the License.
*/
package com.speedment.runtime.compute.internal.expression;
import com.speedment.runtime.compute.ToByte;
import com.speedment.runtime.compute.ToDouble;
import com.speedment.runtime.compute.ToFloat;
import com.speedment.runtime.compute.ToInt;
import com.speedment.runtime.compute.ToLong;
import com.speedment.runtime.compute.ToShort;
import com.speedment.runtime.compute.expression.BinaryExpression;
import com.speedment.runtime.compute.expression.BinaryObjExpression;
import com.speedment.runtime.compute.expression.Expression;
import java.util.Objects;
import static java.util.Objects.requireNonNull;
/**
* Utility class used to construct expression that gives the sum of two
* expressions.
*
* @author Emil Forslund
* @since 3.1.0
*/
public final class MinusUtil {
/**
* Creates and returns an expression that takes the result of the expression
* and adds a constant to it.
*
* @param first the first input expression
* @param second the second input constant
* @param the input type
* @return the new expression
*/
public static ToShort byteMinusByte(ToByte first, byte second) {
class ByteMinusByte extends AbstractMinusByte> implements ToShort {
private ByteMinusByte(ToByte first, byte second) {
super(first, second);
}
@Override
public short applyAsShort(T object) {
return (short) (firstInner.applyAsByte(object) - secondInner);
}
}
return new ByteMinusByte(first, second);
}
/**
* Creates and returns an expression that takes the result of the expression
* and adds a constant to it.
*
* @param first the first input expression
* @param second the second input constant
* @param the input type
* @return the new expression
*/
public static ToInt byteMinusInt(ToByte first, int second) {
class ByteMinusInt extends AbstractMinusInt> implements ToInt {
private ByteMinusInt(ToByte first, int second) {
super(first, second);
}
@Override
public int applyAsInt(T object) {
return firstInner.applyAsByte(object) - secondInner;
}
}
return new ByteMinusInt(first, second);
}
/**
* Creates and returns an expression that takes the result of the expression
* and adds a constant to it.
*
* @param first the first input expression
* @param second the second input constant
* @param the input type
* @return the new expression
*/
public static ToLong byteMinusLong(ToByte first, long second) {
class ByteMinusLong extends AbstractMinusLong> implements ToLong {
private ByteMinusLong(ToByte first, long second) {
super(first, second);
}
@Override
public long applyAsLong(T object) {
return firstInner.applyAsByte(object) - secondInner;
}
}
return new ByteMinusLong(first, second);
}
/**
* Creates and returns an expression that takes the result of the two
* expressions and add them together.
*
* @param first the first input expression
* @param second the second input expression
* @param the input type
* @return the new expression
*/
public static ToShort byteMinusByte(ToByte first, ToByte second) {
class ByteMinusByte extends AbstractMinus, ToByte> implements ToShort {
private ByteMinusByte(ToByte first, ToByte second) {
super(first, second);
}
@Override
public short applyAsShort(T object) {
return (short) (firstInner.applyAsByte(object)
- secondInner.applyAsByte(object));
}
}
return new ByteMinusByte(first, second);
}
/**
* Creates and returns an expression that takes the result of the expression
* and adds a constant to it.
*
* @param first the first input expression
* @param second the second input constant
* @param the input type
* @return the new expression
*/
public static ToInt shortMinusByte(ToShort first, byte second) {
class ShortMinusShort extends AbstractMinusByte> implements ToInt {
private ShortMinusShort(ToShort first, byte second) {
super(first, second);
}
@Override
public int applyAsInt(T object) {
return firstInner.applyAsShort(object) - secondInner;
}
}
return new ShortMinusShort(first, second);
}
/**
* Creates and returns an expression that takes the result of the expression
* and adds a constant to it.
*
* @param first the first input expression
* @param second the second input constant
* @param the input type
* @return the new expression
*/
public static ToInt shortMinusInt(ToShort first, int second) {
class ShortMinusInt extends AbstractMinusInt> implements ToInt {
private ShortMinusInt(ToShort first, int second) {
super(first, second);
}
@Override
public int applyAsInt(T object) {
return firstInner.applyAsShort(object) - secondInner;
}
}
return new ShortMinusInt(first, second);
}
/**
* Creates and returns an expression that takes the result of the expression
* and adds a constant to it.
*
* @param first the first input expression
* @param second the second input constant
* @param the input type
* @return the new expression
*/
public static ToLong shortMinusLong(ToShort first, long second) {
class ShortMinusLong extends AbstractMinusLong> implements ToLong {
private ShortMinusLong(ToShort first, long second) {
super(first, second);
}
@Override
public long applyAsLong(T object) {
return firstInner.applyAsShort(object) - secondInner;
}
}
return new ShortMinusLong(first, second);
}
/**
* Creates and returns an expression that takes the result of the two
* expressions and add them together.
*
* @param first the first input expression
* @param second the second input expression
* @param the input type
* @return the new expression
*/
public static ToShort shortMinusShort(ToShort first, ToShort second) {
class ShortMinusShort extends AbstractMinus, ToShort> implements ToShort {
private ShortMinusShort(ToShort first, ToShort second) {
super(first, second);
}
@Override
public short applyAsShort(T object) {
return (short) (firstInner.applyAsShort(object)
- secondInner.applyAsShort(object));
}
}
return new ShortMinusShort(first, second);
}
/**
* Creates and returns an expression that takes the result of the expression
* and adds a constant to it.
*
* @param first the first input expression
* @param second the second input constant
* @param the input type
* @return the new expression
*/
public static ToInt intMinusByte(ToInt first, byte second) {
class IntMinusByte extends AbstractMinusByte> implements ToInt {
private IntMinusByte(ToInt first, byte second) {
super(first, second);
}
@Override
public int applyAsInt(T object) {
return firstInner.applyAsInt(object) - secondInner;
}
}
return new IntMinusByte(first, second);
}
/**
* Creates and returns an expression that takes the result of the expression
* and adds a constant to it.
*
* @param first the first input expression
* @param second the second input constant
* @param the input type
* @return the new expression
*/
public static ToInt intMinusInt(ToInt first, int second) {
class IntMinusInt extends AbstractMinusInt> implements ToInt {
private IntMinusInt(ToInt first, int second) {
super(first, second);
}
@Override
public int applyAsInt(T object) {
return firstInner.applyAsInt(object) - secondInner;
}
}
return new IntMinusInt(first, second);
}
/**
* Creates and returns an expression that takes the result of the expression
* and adds a constant to it.
*
* @param first the first input expression
* @param second the second input constant
* @param the input type
* @return the new expression
*/
public static ToLong intMinusLong(ToInt first, long second) {
class IntMinusLong extends AbstractMinusLong> implements ToLong {
private IntMinusLong(ToInt first, long second) {
super(first, second);
}
@Override
public long applyAsLong(T object) {
return firstInner.applyAsInt(object) - secondInner;
}
}
return new IntMinusLong(first, second);
}
/**
* Creates and returns an expression that takes the result of the two
* expressions and add them together.
*
* @param first the first input expression
* @param second the second input expression
* @param the input type
* @return the new expression
*/
public static ToInt intMinusByte(ToInt first, ToByte second) {
class IntMinusByte extends AbstractMinus, ToByte> implements ToInt {
private IntMinusByte(ToInt first, ToByte second) {
super(first, second);
}
@Override
public int applyAsInt(T object) {
return firstInner.applyAsInt(object) -
secondInner.applyAsByte(object);
}
}
return new IntMinusByte(first, second);
}
/**
* Creates and returns an expression that takes the result of the two
* expressions and add them together.
*
* @param first the first input expression
* @param second the second input expression
* @param the input type
* @return the new expression
*/
public static ToInt intMinusInt(ToInt first, ToInt second) {
class IntMinusInt extends AbstractMinus, ToInt> implements ToInt {
private IntMinusInt(ToInt first, ToInt second) {
super(first, second);
}
@Override
public int applyAsInt(T object) {
return firstInner.applyAsInt(object) -
secondInner.applyAsInt(object);
}
}
return new IntMinusInt(first, second);
}
/**
* Creates and returns an expression that takes the result of the expression
* and adds a constant to it.
*
* @param first the first input expression
* @param second the second input constant
* @param the input type
* @return the new expression
*/
public static ToLong longMinusByte(ToLong first, byte second) {
class LongMinusLong extends AbstractMinusByte> implements ToLong {
private LongMinusLong(ToLong first, byte second) {
super(first, second);
}
@Override
public long applyAsLong(T object) {
return firstInner.applyAsLong(object) - secondInner;
}
}
return new LongMinusLong(first, second);
}
/**
* Creates and returns an expression that takes the result of the expression
* and adds a constant to it.
*
* @param first the first input expression
* @param second the second input constant
* @param the input type
* @return the new expression
*/
public static ToLong longMinusInt(ToLong first, int second) {
class LongMinusInt extends AbstractMinusInt> implements ToLong {
private LongMinusInt(ToLong first, int second) {
super(first, second);
}
@Override
public long applyAsLong(T object) {
return firstInner.applyAsLong(object) - secondInner;
}
}
return new LongMinusInt(first, second);
}
/**
* Creates and returns an expression that takes the result of the expression
* and adds a constant to it.
*
* @param first the first input expression
* @param second the second input constant
* @param the input type
* @return the new expression
*/
public static ToLong longMinusLong(ToLong first, long second) {
class LongMinusLong extends AbstractMinusLong> implements ToLong {
private LongMinusLong(ToLong first, long second) {
super(first, second);
}
@Override
public long applyAsLong(T object) {
return firstInner.applyAsLong(object) - secondInner;
}
}
return new LongMinusLong(first, second);
}
/**
* Creates and returns an expression that takes the result of the two
* expressions and add them together.
*
* @param first the first input expression
* @param second the second input expression
* @param the input type
* @return the new expression
*/
public static ToLong longMinusInt(ToLong first, ToInt second) {
class LongMinusInt extends AbstractMinus, ToInt> implements ToLong {
private LongMinusInt(ToLong first, ToInt second) {
super(first, second);
}
@Override
public long applyAsLong(T object) {
return firstInner.applyAsLong(object)
- secondInner.applyAsInt(object);
}
}
return new LongMinusInt(first, second);
}
/**
* Creates and returns an expression that takes the result of the two
* expressions and add them together.
*
* @param first the first input expression
* @param second the second input expression
* @param the input type
* @return the new expression
*/
public static ToLong longMinusLong(ToLong first, ToLong second) {
class LongMinusLong extends AbstractMinus, ToLong> implements ToLong {
private LongMinusLong(ToLong first, ToLong second) {
super(first, second);
}
@Override
public long applyAsLong(T object) {
return firstInner.applyAsLong(object)
- secondInner.applyAsLong(object);
}
}
return new LongMinusLong(first, second);
}
/**
* Creates and returns an expression that takes the result of the expression
* and adds a constant to it.
*
* @param first the first input expression
* @param second the second input constant
* @param the input type
* @return the new expression
*/
public static ToFloat floatMinusInt(ToFloat first, int second) {
class FloatMinusInt extends AbstractMinusInt> implements ToFloat {
private FloatMinusInt(ToFloat first, int second) {
super(first, second);
}
@Override
public float applyAsFloat(T object) {
return firstInner.applyAsFloat(object) - secondInner;
}
}
return new FloatMinusInt(first, second);
}
/**
* Creates and returns an expression that takes the result of the expression
* and adds a constant to it.
*
* @param first the first input expression
* @param second the second input constant
* @param the input type
* @return the new expression
*/
public static ToDouble floatMinusLong(ToFloat first, long second) {
class FloatMinusLong extends AbstractMinusLong> implements ToDouble {
private FloatMinusLong(ToFloat first, long second) {
super(first, second);
}
@Override
public double applyAsDouble(T object) {
return firstInner.applyAsFloat(object) - secondInner;
}
}
return new FloatMinusLong(first, second);
}
/**
* Creates and returns an expression that takes the result of the expression
* and adds a constant to it.
*
* @param first the first input expression
* @param second the second input constant
* @param the input type
* @return the new expression
*/
public static ToFloat floatMinusFloat(ToFloat first, float second) {
class FloatMinusFloat extends AbstractMinusFloat> implements ToFloat {
private FloatMinusFloat(ToFloat first, float second) {
super(first, second);
}
@Override
public float applyAsFloat(T object) {
return firstInner.applyAsFloat(object) - secondInner;
}
}
return new FloatMinusFloat(first, second);
}
/**
* Creates and returns an expression that takes the result of the two
* expressions and add them together.
*
* @param first the first input expression
* @param second the second input expression
* @param the input type
* @return the new expression
*/
public static ToFloat floatMinusInt(ToFloat first, ToInt second) {
class FloatMinusInt extends AbstractMinus, ToInt> implements ToFloat {
private FloatMinusInt(ToFloat first, ToInt second) {
super(first, second);
}
@Override
public float applyAsFloat(T object) {
return firstInner.applyAsFloat(object)
- secondInner.applyAsInt(object);
}
}
return new FloatMinusInt(first, second);
}
/**
* Creates and returns an expression that takes the result of the two
* expressions and add them together.
*
* @param first the first input expression
* @param second the second input expression
* @param the input type
* @return the new expression
*/
public static ToDouble floatMinusLong(ToFloat first, ToLong second) {
class FloatMinusLong extends AbstractMinus, ToLong> implements ToDouble {
private FloatMinusLong(ToFloat first, ToLong second) {
super(first, second);
}
@Override
public double applyAsDouble(T object) {
return firstInner.applyAsFloat(object)
- secondInner.applyAsLong(object);
}
}
return new FloatMinusLong(first, second);
}
/**
* Creates and returns an expression that takes the result of the two
* expressions and add them together.
*
* @param first the first input expression
* @param second the second input expression
* @param the input type
* @return the new expression
*/
public static ToFloat floatMinusFloat(ToFloat first, ToFloat second) {
class FloatMinusFloat extends AbstractMinus, ToFloat> implements ToFloat {
private FloatMinusFloat(ToFloat first, ToFloat second) {
super(first, second);
}
@Override
public float applyAsFloat(T object) {
return firstInner.applyAsFloat(object)
- secondInner.applyAsFloat(object);
}
}
return new FloatMinusFloat(first, second);
}
/**
* Creates and returns an expression that takes the result of the expression
* and adds a constant to it.
*
* @param first the first input expression
* @param second the second input constant
* @param the input type
* @return the new expression
*/
public static ToDouble doubleMinusInt(ToDouble first, int second) {
class DoubleMinusInt extends AbstractMinusInt> implements ToDouble {
private DoubleMinusInt(ToDouble first, int second) {
super(first, second);
}
@Override
public double applyAsDouble(T object) {
return firstInner.applyAsDouble(object) - secondInner;
}
}
return new DoubleMinusInt(first, second);
}
/**
* Creates and returns an expression that takes the result of the expression
* and adds a constant to it.
*
* @param first the first input expression
* @param second the second input constant
* @param the input type
* @return the new expression
*/
public static ToDouble doubleMinusLong(ToDouble first, long second) {
class DoubleMinusLong extends AbstractMinusLong> implements ToDouble {
private DoubleMinusLong(ToDouble first, long second) {
super(first, second);
}
@Override
public double applyAsDouble(T object) {
return firstInner.applyAsDouble(object) - secondInner;
}
}
return new DoubleMinusLong(first, second);
}
/**
* Creates and returns an expression that takes the result of the expression
* and adds a constant to it.
*
* @param first the first input expression
* @param second the second input constant
* @param the input type
* @return the new expression
*/
public static ToDouble doubleMinusDouble(ToDouble first, double second) {
class DoubleMinusDouble extends AbstractMinusDouble> implements ToDouble {
private DoubleMinusDouble(ToDouble first, double second) {
super(first, second);
}
@Override
public double applyAsDouble(T object) {
return firstInner.applyAsDouble(object) - secondInner;
}
}
return new DoubleMinusDouble(first, second);
}
/**
* Creates and returns an expression that takes the result of the two
* expressions and add them together.
*
* @param first the first input expression
* @param second the second input expression
* @param the input type
* @return the new expression
*/
public static ToDouble doubleMinusInt(ToDouble first, ToInt second) {
class DoubleMinusInt extends AbstractMinus, ToInt> implements ToDouble {
private DoubleMinusInt(ToDouble first, ToInt second) {
super(first, second);
}
@Override
public double applyAsDouble(T object) {
return firstInner.applyAsDouble(object)
- secondInner.applyAsInt(object);
}
}
return new DoubleMinusInt(first, second);
}
/**
* Creates and returns an expression that takes the result of the two
* expressions and add them together.
*
* @param first the first input expression
* @param second the second input expression
* @param the input type
* @return the new expression
*/
public static ToDouble doubleMinusLong(ToDouble first, ToLong second) {
class DoubleMinusLong extends AbstractMinus, ToLong> implements ToDouble {
private DoubleMinusLong(ToDouble first, ToLong second) {
super(first, second);
}
@Override
public double applyAsDouble(T object) {
return firstInner.applyAsDouble(object)
- secondInner.applyAsLong(object);
}
}
return new DoubleMinusLong(first, second);
}
/**
* Creates and returns an expression that takes the result of the two
* expressions and add them together.
*
* @param first the first input expression
* @param second the second input expression
* @param the input type
* @return the new expression
*/
public static ToDouble doubleMinusDouble(ToDouble first, ToDouble second) {
class DoubleMinusDouble extends AbstractMinus, ToDouble> implements ToDouble {
private DoubleMinusDouble(ToDouble first, ToDouble second) {
super(first, second);
}
@Override
public double applyAsDouble(T object) {
return firstInner.applyAsDouble(object)
- secondInner.applyAsDouble(object);
}
}
return new DoubleMinusDouble(first, second);
}
/**
* Abstract base for a minus operation.
*
* @param the input type
* @param the first operand expression type
* @param the second operand expression type
*/
private static abstract class AbstractMinus, SECOND extends Expression