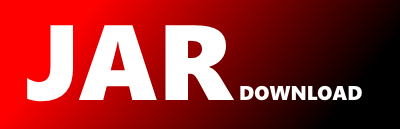
com.speedment.runtime.compute.internal.expression.MapperUtil Maven / Gradle / Ivy
/*
*
* Copyright (c) 2006-2020, Speedment, Inc. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"); You may not
* use this file except in compliance with the License. You may obtain a copy of
* the License at:
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS, WITHOUT
* WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. See the
* License for the specific language governing permissions and limitations under
* the License.
*/
package com.speedment.runtime.compute.internal.expression;
import static java.util.Objects.requireNonNull;
import com.speedment.common.function.BooleanToDoubleFunction;
import com.speedment.common.function.BooleanUnaryOperator;
import com.speedment.common.function.ByteToDoubleFunction;
import com.speedment.common.function.ByteUnaryOperator;
import com.speedment.common.function.CharUnaryOperator;
import com.speedment.common.function.FloatToDoubleFunction;
import com.speedment.common.function.FloatUnaryOperator;
import com.speedment.common.function.ShortToDoubleFunction;
import com.speedment.common.function.ShortUnaryOperator;
import com.speedment.runtime.compute.ToBigDecimal;
import com.speedment.runtime.compute.ToBoolean;
import com.speedment.runtime.compute.ToByte;
import com.speedment.runtime.compute.ToChar;
import com.speedment.runtime.compute.ToDouble;
import com.speedment.runtime.compute.ToEnum;
import com.speedment.runtime.compute.ToFloat;
import com.speedment.runtime.compute.ToInt;
import com.speedment.runtime.compute.ToLong;
import com.speedment.runtime.compute.ToShort;
import com.speedment.runtime.compute.ToString;
import com.speedment.runtime.compute.expression.Expression;
import com.speedment.runtime.compute.expression.MapperExpression;
import java.math.BigDecimal;
import java.util.Objects;
import java.util.function.DoubleUnaryOperator;
import java.util.function.IntToDoubleFunction;
import java.util.function.IntUnaryOperator;
import java.util.function.LongToDoubleFunction;
import java.util.function.LongUnaryOperator;
import java.util.function.ToDoubleFunction;
import java.util.function.UnaryOperator;
/**
* Utility class used to create expressions that map from one value to another.
*
* @author Emil Forslund
* @since 3.1.0
*/
public final class MapperUtil {
/**
* Returns an expression that first applies the specified expression to get
* a value, then applies the specified mapping operation to that value to
* get the final result.
*
* @param expression the expression to apply to the input
* @param mapper the mapper to apply to the result
* @param the input type
* @return the new expression
*/
public static ToBoolean mapBoolean(ToBoolean expression, BooleanUnaryOperator mapper) {
return new ToBooleanMapper, BooleanUnaryOperator>(expression, mapper) {
@Override
public boolean applyAsBoolean(T object) {
return this.mapper.applyAsBoolean(this.inner.applyAsBoolean(object));
}
@Override
public MapperType mapperType() {
return MapperType.BOOLEAN_TO_BOOLEAN;
}
};
}
/**
* Returns an expression that first applies the specified expression to get
* a value, then applies the specified mapping operation to that value to
* get the final result.
*
* @param expression the expression to apply to the input
* @param mapper the mapper to apply to the result
* @param the input type
* @return the new expression
*/
public static ToDouble mapBooleanToDouble(ToBoolean expression, BooleanToDoubleFunction mapper) {
return new ToDoubleMapper, BooleanToDoubleFunction>(expression, mapper) {
@Override
public double applyAsDouble(T object) {
return this.mapper.applyAsDouble(this.inner.applyAsBoolean(object));
}
@Override
public MapperType mapperType() {
return MapperType.BOOLEAN_TO_DOUBLE;
}
};
}
/**
* Returns an expression that first applies the specified expression to get
* a value, then applies the specified mapping operation to that value to
* get the final result.
*
* @param expression the expression to apply to the input
* @param mapper the mapper to apply to the result
* @param the input type
* @return the new expression
*/
public static ToChar mapChar(ToChar expression, CharUnaryOperator mapper) {
return new ToCharMapper, CharUnaryOperator>(expression, mapper) {
@Override
public char applyAsChar(T object) {
return this.mapper.applyAsChar(this.inner.applyAsChar(object));
}
@Override
public MapperType mapperType() {
return MapperType.CHAR_TO_CHAR;
}
};
}
/**
* Returns an expression that first applies the specified expression to get
* a value, then applies the specified mapping operation to that value to
* get the final result.
*
* @param expression the expression to apply to the input
* @param mapper the mapper to apply to the result
* @param the input type
* @return the new expression
*/
public static ToByte mapByte(ToByte expression, ByteUnaryOperator mapper) {
return new ToByteMapper, ByteUnaryOperator>(expression, mapper) {
@Override
public byte applyAsByte(T object) {
return this.mapper.applyAsByte(this.inner.applyAsByte(object));
}
@Override
public MapperType mapperType() {
return MapperType.BYTE_TO_BYTE;
}
};
}
/**
* Returns an expression that first applies the specified expression to get
* a value, then applies the specified mapping operation to that value to
* get the final result.
*
* @param expression the expression to apply to the input
* @param mapper the mapper to apply to the result
* @param the input type
* @return the new expression
*/
public static ToDouble mapByteToDouble(ToByte expression, ByteToDoubleFunction mapper) {
return new ToDoubleMapper, ByteToDoubleFunction>(expression, mapper) {
@Override
public double applyAsDouble(T object) {
return this.mapper.applyAsDouble(this.inner.applyAsByte(object));
}
@Override
public MapperType mapperType() {
return MapperType.BYTE_TO_DOUBLE;
}
};
}
/**
* Returns an expression that first applies the specified expression to get
* a value, then applies the specified mapping operation to that value to
* get the final result.
*
* @param expression the expression to apply to the input
* @param mapper the mapper to apply to the result
* @param the input type
* @return the new expression
*/
public static ToShort mapShort(ToShort expression, ShortUnaryOperator mapper) {
return new ToShortMapper, ShortUnaryOperator>(expression, mapper) {
@Override
public short applyAsShort(T object) {
return this.mapper.applyAsShort(this.inner.applyAsShort(object));
}
@Override
public MapperType mapperType() {
return MapperType.SHORT_TO_SHORT;
}
};
}
/**
* Returns an expression that first applies the specified expression to get
* a value, then applies the specified mapping operation to that value to
* get the final result.
*
* @param expression the expression to apply to the input
* @param mapper the mapper to apply to the result
* @param the input type
* @return the new expression
*/
public static ToDouble mapShortToDouble(ToShort expression, ShortToDoubleFunction mapper) {
return new ToDoubleMapper, ShortToDoubleFunction>(expression, mapper) {
@Override
public double applyAsDouble(T object) {
return this.mapper.applyAsDouble(this.inner.applyAsShort(object));
}
@Override
public MapperType mapperType() {
return MapperType.SHORT_TO_DOUBLE;
}
};
}
/**
* Returns an expression that first applies the specified expression to get
* a value, then applies the specified mapping operation to that value to
* get the final result.
*
* @param expression the expression to apply to the input
* @param mapper the mapper to apply to the result
* @param the input type
* @return the new expression
*/
public static ToInt mapInt(ToInt expression, IntUnaryOperator mapper) {
return new ToIntMapper, IntUnaryOperator>(expression, mapper) {
@Override
public int applyAsInt(T object) {
return this.mapper.applyAsInt(this.inner.applyAsInt(object));
}
@Override
public MapperType mapperType() {
return MapperType.INT_TO_INT;
}
};
}
/**
* Returns an expression that first applies the specified expression to get
* a value, then applies the specified mapping operation to that value to
* get the final result.
*
* @param expression the expression to apply to the input
* @param mapper the mapper to apply to the result
* @param the input type
* @return the new expression
*/
public static ToDouble mapIntToDouble(ToInt expression, IntToDoubleFunction mapper) {
return new ToDoubleMapper, IntToDoubleFunction>(expression, mapper) {
@Override
public double applyAsDouble(T object) {
return this.mapper.applyAsDouble(this.inner.applyAsInt(object));
}
@Override
public MapperType mapperType() {
return MapperType.INT_TO_DOUBLE;
}
};
}
/**
* Returns an expression that first applies the specified expression to get
* a value, then applies the specified mapping operation to that value to
* get the final result.
*
* @param expression the expression to apply to the input
* @param mapper the mapper to apply to the result
* @param the input type
* @return the new expression
*/
public static ToLong mapLong(ToLong expression, LongUnaryOperator mapper) {
return new ToLongMapper, LongUnaryOperator>(expression, mapper) {
@Override
public long applyAsLong(T object) {
return this.mapper.applyAsLong(this.inner.applyAsLong(object));
}
@Override
public MapperType mapperType() {
return MapperType.LONG_TO_LONG;
}
};
}
/**
* Returns an expression that first applies the specified expression to get
* a value, then applies the specified mapping operation to that value to
* get the final result.
*
* @param expression the expression to apply to the input
* @param mapper the mapper to apply to the result
* @param the input type
* @return the new expression
*/
public static ToDouble mapLongToDouble(ToLong expression, LongToDoubleFunction mapper) {
return new ToDoubleMapper, LongToDoubleFunction>(expression, mapper) {
@Override
public double applyAsDouble(T object) {
return this.mapper.applyAsDouble(this.inner.applyAsLong(object));
}
@Override
public MapperType mapperType() {
return MapperType.LONG_TO_DOUBLE;
}
};
}
/**
* Returns an expression that first applies the specified expression to get
* a value, then applies the specified mapping operation to that value to
* get the final result.
*
* @param expression the expression to apply to the input
* @param mapper the mapper to apply to the result
* @param the input type
* @return the new expression
*/
public static ToFloat mapFloat(ToFloat expression, FloatUnaryOperator mapper) {
return new ToFloatMapper, FloatUnaryOperator>(expression, mapper) {
@Override
public float applyAsFloat(T object) {
return this.mapper.applyAsFloat(this.inner.applyAsFloat(object));
}
@Override
public MapperType mapperType() {
return MapperType.FLOAT_TO_FLOAT;
}
};
}
/**
* Returns an expression that first applies the specified expression to get
* a value, then applies the specified mapping operation to that value to
* get the final result.
*
* @param expression the expression to apply to the input
* @param mapper the mapper to apply to the result
* @param the input type
* @return the new expression
*/
public static ToDouble mapFloatToDouble(ToFloat expression, FloatToDoubleFunction mapper) {
return new ToDoubleMapper, FloatToDoubleFunction>(expression, mapper) {
@Override
public double applyAsDouble(T object) {
return this.mapper.applyAsDouble(this.inner.applyAsFloat(object));
}
@Override
public MapperType mapperType() {
return MapperType.FLOAT_TO_DOUBLE;
}
};
}
/**
* Returns an expression that first applies the specified expression to get
* a value, then applies the specified mapping operation to that value to
* get the final result.
*
* @param expression the expression to apply to the input
* @param mapper the mapper to apply to the result
* @param the input type
* @return the new expression
*/
public static ToDouble mapDouble(ToDouble expression, DoubleUnaryOperator mapper) {
return new ToDoubleMapper, DoubleUnaryOperator>(expression, mapper) {
@Override
public double applyAsDouble(T object) {
return this.mapper.applyAsDouble(this.inner.applyAsDouble(object));
}
@Override
public MapperType mapperType() {
return MapperType.DOUBLE_TO_DOUBLE;
}
};
}
/**
* Returns an expression that first applies the specified expression to get
* a value, then applies the specified mapping operation to that value to
* get the final result.
*
* @param expression the expression to apply to the input
* @param mapper the mapper to apply to the result
* @param the input type
* @return the new expression
*/
public static ToString mapString(ToString expression, UnaryOperator mapper) {
return new ToStringMapper, UnaryOperator>(expression, mapper) {
@Override
public String apply(T object) {
return this.mapper.apply(this.inner.apply(object));
}
@Override
public MapperType mapperType() {
return MapperType.STRING_TO_STRING;
}
};
}
/**
* Returns an expression that first applies the specified expression to get
* a value, then applies the specified mapping operation to that value to
* get the final result.
*
* @param expression the expression to apply to the input
* @param mapper the mapper to apply to the result
* @param the input type
* @return the new expression
*/
public static > ToEnum mapEnum(ToEnum expression, UnaryOperator mapper) {
return new ToEnumMapper, UnaryOperator>(expression, mapper) {
@Override
public E apply(T object) {
return this.mapper.apply(this.inner.apply(object));
}
@Override
public Class enumClass() {
return this.inner.enumClass();
}
@Override
public MapperType mapperType() {
return MapperType.ENUM_TO_ENUM;
}
};
}
/**
* Returns an expression that first applies the specified expression to get
* a value, then applies the specified mapping operation to that value to
* get the final result.
*
* @param expression the expression to apply to the input
* @param mapper the mapper to apply to the result
* @param the input type
* @return the new expression
*/
public static ToBigDecimal mapBigDecimal(ToBigDecimal expression, UnaryOperator mapper) {
return new ToBigDecimalMapper, UnaryOperator>(expression, mapper) {
@Override
public BigDecimal apply(T object) {
return this.mapper.apply(this.inner.apply(object));
}
@Override
public MapperType mapperType() {
return MapperType.BIG_DECIMAL_TO_BIG_DECIMAL;
}
};
}
/**
* Returns an expression that first applies the specified expression to get
* a value, then applies the specified mapping operation to that value to
* get the final result.
*
* @param expression the expression to apply to the input
* @param mapper the mapper to apply to the result
* @param the input type
* @return the new expression
*/
public static ToDouble mapBigDecimalToDouble(ToBigDecimal expression, ToDoubleFunction mapper) {
return new ToDoubleMapper, ToDoubleFunction>(expression, mapper) {
@Override
public double applyAsDouble(T object) {
return this.mapper.applyAsDouble(this.inner.apply(object));
}
@Override
public MapperType mapperType() {
return MapperType.BIG_DECIMAL_TO_DOUBLE;
}
};
}
/**
* Abstract base for a mapping operation that results in a {@code byte}.
*
* @param the input type
* @param the inner expression type
* @param the mapping functional interface
*/
abstract static class ToByteMapper, MAPPER>
extends AbstractMapper implements ToByte {
ToByteMapper(INNER inner, MAPPER mapper) {
super(inner, mapper);
}
}
/**
* Abstract base for a mapping operation that results in a {@code short}.
*
* @param the input type
* @param the inner expression type
* @param the mapping functional interface
*/
abstract static class ToShortMapper, MAPPER>
extends AbstractMapper implements ToShort {
ToShortMapper(INNER inner, MAPPER mapper) {
super(inner, mapper);
}
}
/**
* Abstract base for a mapping operation that results in a {@code int}.
*
* @param the input type
* @param the inner expression type
* @param the mapping functional interface
*/
abstract static class ToIntMapper, MAPPER>
extends AbstractMapper implements ToInt {
ToIntMapper(INNER inner, MAPPER mapper) {
super(inner, mapper);
}
}
/**
* Abstract base for a mapping operation that results in a {@code long}.
*
* @param the input type
* @param the inner expression type
* @param the mapping functional interface
*/
abstract static class ToLongMapper, MAPPER>
extends AbstractMapper implements ToLong {
ToLongMapper(INNER inner, MAPPER mapper) {
super(inner, mapper);
}
}
/**
* Abstract base for a mapping operation that results in a {@code float}.
*
* @param the input type
* @param the inner expression type
* @param the mapping functional interface
*/
abstract static class ToFloatMapper, MAPPER>
extends AbstractMapper implements ToFloat {
ToFloatMapper(INNER inner, MAPPER mapper) {
super(inner, mapper);
}
}
/**
* Abstract base for a mapping operation that results in a {@code double}.
*
* @param the input type
* @param the inner expression type
* @param the mapping functional interface
*/
abstract static class ToDoubleMapper, MAPPER>
extends AbstractMapper implements ToDouble {
ToDoubleMapper(INNER inner, MAPPER mapper) {
super(inner, mapper);
}
}
/**
* Abstract base for a mapping operation that results in a {@code char}.
*
* @param the input type
* @param the inner expression type
* @param the mapping functional interface
*/
abstract static class ToCharMapper, MAPPER>
extends AbstractMapper implements ToChar {
ToCharMapper(INNER inner, MAPPER mapper) {
super(inner, mapper);
}
}
/**
* Abstract base for a mapping operation that results in a {@code boolean}.
*
* @param the input type
* @param the inner expression type
* @param the mapping functional interface
*/
abstract static class ToBooleanMapper, MAPPER>
extends AbstractMapper implements ToBoolean {
ToBooleanMapper(INNER inner, MAPPER mapper) {
super(inner, mapper);
}
}
/**
* Abstract base for a mapping operation that results in a {@code enum}.
*
* @param the input type
* @param the enum type
* @param the inner expression type
* @param the mapping functional interface
*/
abstract static class ToEnumMapper, INNER extends Expression, MAPPER>
extends AbstractMapper implements ToEnum {
ToEnumMapper(INNER inner, MAPPER mapper) {
super(inner, mapper);
}
}
/**
* Abstract base for a mapping operation that results in a {@code string}.
*
* @param the input type
* @param the inner expression type
* @param the mapping functional interface
*/
abstract static class ToStringMapper, MAPPER>
extends AbstractMapper implements ToString {
ToStringMapper(INNER inner, MAPPER mapper) {
super(inner, mapper);
}
}
/**
* Abstract base for a mapping operation that results in a {@code bigDecimal}.
*
* @param the input type
* @param the inner expression type
* @param the mapping functional interface
*/
abstract static class ToBigDecimalMapper, MAPPER>
extends AbstractMapper implements ToBigDecimal {
ToBigDecimalMapper(INNER inner, MAPPER mapper) {
super(inner, mapper);
}
}
/**
* Abstract base for a mapping operation.
*
* @param the input type
* @param the inner expression type
* @param the mapping functional interface
*/
abstract static class AbstractMapper, MAPPER>
implements MapperExpression {
final INNER inner;
final MAPPER mapper;
AbstractMapper(INNER inner, MAPPER mapper) {
this.inner = requireNonNull(inner);
this.mapper = requireNonNull(mapper);
}
@Override
public final INNER inner() {
return inner;
}
@Override
public final MAPPER mapper() {
return mapper;
}
@Override
public final boolean equals(Object o) {
if (this == o) return true;
else if (!(o instanceof MapperExpression)) return false;
final MapperExpression, ?, ?> that = (MapperExpression, ?, ?>) o;
return Objects.equals(inner(), that.inner()) &&
Objects.equals(mapper(), that.mapper()) &&
Objects.equals(mapperType(), that.mapperType());
}
@Override
public final int hashCode() {
return Objects.hash(inner(), mapper(), mapperType());
}
}
/**
* Utility classes should not be instantiated.
*/
private MapperUtil() {}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy