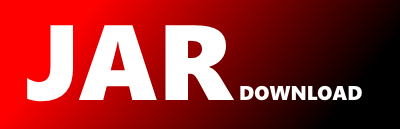
com.speedment.runtime.compute.internal.expression.OrElseThrowUtil Maven / Gradle / Ivy
/*
*
* Copyright (c) 2006-2020, Speedment, Inc. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"); You may not
* use this file except in compliance with the License. You may obtain a copy of
* the License at:
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS, WITHOUT
* WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. See the
* License for the specific language governing permissions and limitations under
* the License.
*/
package com.speedment.runtime.compute.internal.expression;
import static java.util.Objects.requireNonNull;
import com.speedment.runtime.compute.ToBigDecimalNullable;
import com.speedment.runtime.compute.ToBooleanNullable;
import com.speedment.runtime.compute.ToByteNullable;
import com.speedment.runtime.compute.ToCharNullable;
import com.speedment.runtime.compute.ToDoubleNullable;
import com.speedment.runtime.compute.ToEnumNullable;
import com.speedment.runtime.compute.ToFloatNullable;
import com.speedment.runtime.compute.ToIntNullable;
import com.speedment.runtime.compute.ToLongNullable;
import com.speedment.runtime.compute.ToShortNullable;
import com.speedment.runtime.compute.ToStringNullable;
import com.speedment.runtime.compute.expression.Expression;
import com.speedment.runtime.compute.expression.NonNullableExpression;
import com.speedment.runtime.compute.expression.orelse.OrElseThrowExpression;
import com.speedment.runtime.compute.expression.orelse.ToBigDecimalOrThrow;
import com.speedment.runtime.compute.expression.orelse.ToBooleanOrThrow;
import com.speedment.runtime.compute.expression.orelse.ToByteOrThrow;
import com.speedment.runtime.compute.expression.orelse.ToCharOrThrow;
import com.speedment.runtime.compute.expression.orelse.ToDoubleOrThrow;
import com.speedment.runtime.compute.expression.orelse.ToEnumOrThrow;
import com.speedment.runtime.compute.expression.orelse.ToFloatOrThrow;
import com.speedment.runtime.compute.expression.orelse.ToIntOrThrow;
import com.speedment.runtime.compute.expression.orelse.ToLongOrThrow;
import com.speedment.runtime.compute.expression.orelse.ToShortOrThrow;
import com.speedment.runtime.compute.expression.orelse.ToStringOrThrow;
import java.math.BigDecimal;
import java.util.Objects;
/**
* Utility class used to create expressions that wrap a nullable expression and
* handles any {@code null}-values by throwing an exception.
*
* @author Emil Forslund
* @since 3.1.0
*/
public final class OrElseThrowUtil {
/**
* Returns an expression that returns the same value as the specified
* expression if it is not {@code null}, and if the wrapped expression
* returns {@code null}, throws an exception.
*
* @param expression the nullable expression to wrap
* @param the input entity type
* @return the non-nullable expression
*/
public static ToDoubleOrThrow
doubleOrElseThrow(ToDoubleNullable expression) {
return new ToDoubleOrElseThrowImpl<>(expression);
}
/**
* Internal class used when calling {@code ToDoubleNullable.doubleOrElse(double)}.
*
* @param the input entity type
*/
private static final class ToDoubleOrElseThrowImpl
extends AbstractNonNullable>
implements ToDoubleOrThrow {
private ToDoubleOrElseThrowImpl(ToDoubleNullable inner) {
super(inner);
}
@Override
public double applyAsDouble(T object) {
return inner.applyAsDouble(object);
}
}
/**
* Returns an expression that returns the same value as the specified
* expression if it is not {@code null}, and if the wrapped expression
* returns {@code null}, throws an exception.
*
* @param expression the nullable expression to wrap
* @param the input entity type
* @return the non-nullable expression
*/
public static ToFloatOrThrow
floatOrElseThrow(ToFloatNullable expression) {
return new ToFloatOrElseThrowImpl<>(expression);
}
/**
* Internal class used when calling {@code ToFloatNullable.doubleOrElse(float)}.
*
* @param the input entity type
*/
private static final class ToFloatOrElseThrowImpl
extends AbstractNonNullable>
implements ToFloatOrThrow {
private ToFloatOrElseThrowImpl(ToFloatNullable inner) {
super(inner);
}
@Override
public float applyAsFloat(T object) {
return inner.applyAsFloat(object);
}
}
/**
* Returns an expression that returns the same value as the specified
* expression if it is not {@code null}, and if the wrapped expression
* returns {@code null}, throws an exception.
*
* @param expression the nullable expression to wrap
* @param the input entity type
* @return the non-nullable expression
*/
public static ToLongOrThrow
longOrElseThrow(ToLongNullable expression) {
return new ToLongOrElseThrowImpl<>(expression);
}
/**
* Internal class used when calling {@code ToLongNullable.doubleOrElse(long)}.
*
* @param the input entity type
*/
private static final class ToLongOrElseThrowImpl
extends AbstractNonNullable>
implements ToLongOrThrow {
private ToLongOrElseThrowImpl(ToLongNullable inner) {
super(inner);
}
@Override
public long applyAsLong(T object) {
return inner.applyAsLong(object);
}
}
/**
* Returns an expression that returns the same value as the specified
* expression if it is not {@code null}, and if the wrapped expression
* returns {@code null}, throws an exception.
*
* @param expression the nullable expression to wrap
* @param the input entity type
* @return the non-nullable expression
*/
public static ToIntOrThrow
intOrElseThrow(ToIntNullable expression) {
return new ToIntOrElseThrowImpl<>(expression);
}
/**
* Internal class used when calling {@code ToIntNullable.doubleOrElse(int)}.
*
* @param the input entity type
*/
private static final class ToIntOrElseThrowImpl
extends AbstractNonNullable>
implements ToIntOrThrow {
private ToIntOrElseThrowImpl(ToIntNullable inner) {
super(inner);
}
@Override
public int applyAsInt(T object) {
return inner.applyAsInt(object);
}
}
/**
* Returns an expression that returns the same value as the specified
* expression if it is not {@code null}, and if the wrapped expression
* returns {@code null}, throws an exception.
*
* @param expression the nullable expression to wrap
* @param the input entity type
* @return the non-nullable expression
*/
public static ToShortOrThrow
shortOrElseThrow(ToShortNullable expression) {
return new ToShortOrElseThrowImpl<>(expression);
}
/**
* Internal class used when calling {@code ToShortNullable.doubleOrElse(short)}.
*
* @param the input entity type
*/
private static final class ToShortOrElseThrowImpl
extends AbstractNonNullable>
implements ToShortOrThrow {
private ToShortOrElseThrowImpl(ToShortNullable inner) {
super(inner);
}
@Override
public short applyAsShort(T object) {
return inner.applyAsShort(object);
}
}
/**
* Returns an expression that returns the same value as the specified
* expression if it is not {@code null}, and if the wrapped expression
* returns {@code null}, throws an exception.
*
* @param expression the nullable expression to wrap
* @param the input entity type
* @return the non-nullable expression
*/
public static ToByteOrThrow
byteOrElseThrow(ToByteNullable expression) {
return new ToByteOrElseThrowImpl<>(expression);
}
/**
* Internal class used when calling {@code ToByteNullable.doubleOrElse(byte)}.
*
* @param the input entity type
*/
private static final class ToByteOrElseThrowImpl
extends AbstractNonNullable>
implements ToByteOrThrow {
private ToByteOrElseThrowImpl(ToByteNullable inner) {
super(inner);
}
@Override
public byte applyAsByte(T object) {
return inner.applyAsByte(object);
}
}
/**
* Returns an expression that returns the same value as the specified
* expression if it is not {@code null}, and if the wrapped expression
* returns {@code null}, throws an exception.
*
* @param expression the nullable expression to wrap
* @param the input entity type
* @return the non-nullable expression
*/
public static ToCharOrThrow
charOrElseThrow(ToCharNullable expression) {
return new ToCharOrElseThrowImpl<>(expression);
}
/**
* Internal class used when calling {@code ToCharNullable.doubleOrElse(char)}.
*
* @param the input entity type
*/
private static final class ToCharOrElseThrowImpl
extends AbstractNonNullable>
implements ToCharOrThrow {
private ToCharOrElseThrowImpl(ToCharNullable inner) {
super(inner);
}
@Override
public char applyAsChar(T object) {
return inner.applyAsChar(object);
}
}
/**
* Returns an expression that returns the same value as the specified
* expression if it is not {@code null}, and if the wrapped expression
* returns {@code null}, throws an exception.
*
* @param expression the nullable expression to wrap
* @param the input entity type
* @return the non-nullable expression
*/
public static ToBooleanOrThrow
booleanOrElseThrow(ToBooleanNullable expression) {
return new ToBooleanOrElseThrowImpl<>(expression);
}
/**
* Internal class used when calling {@code ToBooleanNullable.doubleOrElse(boolean)}.
*
* @param the input entity type
*/
private static final class ToBooleanOrElseThrowImpl
extends AbstractNonNullable>
implements ToBooleanOrThrow {
private ToBooleanOrElseThrowImpl(ToBooleanNullable inner) {
super(inner);
}
@Override
public boolean applyAsBoolean(T object) {
return inner.applyAsBoolean(object);
}
}
/**
* Returns an expression that returns the same value as the specified
* expression if it is not {@code null}, and if the wrapped expression
* returns {@code null}, throws an exception.
*
* @param expression the nullable expression to wrap
* @param the input entity type
* @return the non-nullable expression
*/
public static ToStringOrThrow
stringOrElseThrow(ToStringNullable expression) {
return new ToStringOrElseThrowImpl<>(expression);
}
/**
* Internal class used when calling {@code ToStringNullable.doubleOrElse(String)}.
*
* @param the input entity type
*/
private static final class ToStringOrElseThrowImpl
extends AbstractNonNullable>
implements ToStringOrThrow {
private ToStringOrElseThrowImpl(ToStringNullable inner) {
super(inner);
}
@Override
public String apply(T object) {
return requireNonNull(inner.apply(object));
}
}
/**
* Returns an expression that returns the same value as the specified
* expression if it is not {@code null}, and if the wrapped expression
* returns {@code null}, throws an exception.
*
* @param expression the nullable expression to wrap
* @param the input entity type
* @return the non-nullable expression
*/
public static ToBigDecimalOrThrow
bigDecimalOrElseThrow(ToBigDecimalNullable expression) {
return new ToBigDecimalOrElseThrowImpl<>(expression);
}
/**
* Internal class used when calling
* {@code ToBigDecimalNullable.doubleOrElse(BigDecimal)}.
*
* @param the input entity type
*/
private static final class ToBigDecimalOrElseThrowImpl
extends AbstractNonNullable>
implements ToBigDecimalOrThrow {
private ToBigDecimalOrElseThrowImpl(ToBigDecimalNullable inner) {
super(inner);
}
@Override
public BigDecimal apply(T object) {
return requireNonNull(inner.apply(object));
}
}
/**
* Returns an expression that returns the same value as the specified
* expression if it is not {@code null}, and if the wrapped expression
* returns {@code null}, throws an exception.
*
* @param expression the nullable expression to wrap
* @param the input entity type
* @param the enum type
* @return the non-nullable expression
*/
public static > ToEnumOrThrow
enumOrElseThrow(ToEnumNullable expression) {
return new ToEnumOrElseThrowImpl<>(expression);
}
/**
* Internal class used when calling {@code ToEnumNullable.doubleOrElse(Enum)}.
*
* @param the input entity type
* @param the enum type
*/
private static final class ToEnumOrElseThrowImpl>
extends AbstractNonNullable>
implements ToEnumOrThrow {
private ToEnumOrElseThrowImpl(ToEnumNullable inner) {
super(inner);
}
@Override
public E apply(T object) {
return requireNonNull(inner.apply(object));
}
}
/**
* Abstract base for a {@link NonNullableExpression}.
*
* @param the input type
* @param the wrapped nullable expression type
*/
abstract static class AbstractNonNullable>
implements OrElseThrowExpression {
final INNER inner;
AbstractNonNullable(INNER inner) {
this.inner = requireNonNull(inner);
}
@Override
public final INNER innerNullable() {
return inner;
}
@Override
public final boolean equals(Object o) {
if (this == o) return true;
if (!(o instanceof NonNullableExpression)) return false;
final NonNullableExpression, ?> that = (NonNullableExpression, ?>) o;
return Objects.equals(inner, that.innerNullable()) &&
Objects.equals(nullStrategy(), that.nullStrategy());
}
@Override
public final int hashCode() {
return Objects.hash(inner);
}
}
/**
* Utility classes should not be instantiated.
*/
private OrElseThrowUtil() {}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy