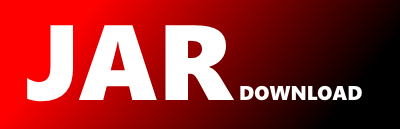
com.speedment.runtime.field.internal.DoubleForeignKeyFieldImpl Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of runtime-field Show documentation
Show all versions of runtime-field Show documentation
Partly generated model of the fields that represent columns in the
database. Fields can be used to produce special predicates and functions
that contain metadata that Speedment can analyze runtime.
/**
*
* Copyright (c) 2006-2017, Speedment, Inc. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"); You may not
* use this file except in compliance with the License. You may obtain a copy of
* the License at:
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS, WITHOUT
* WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. See the
* License for the specific language governing permissions and limitations under
* the License.
*/
package com.speedment.runtime.field.internal;
import com.speedment.common.annotation.GeneratedCode;
import com.speedment.runtime.config.identifier.ColumnIdentifier;
import com.speedment.runtime.config.identifier.TableIdentifier;
import com.speedment.runtime.field.DoubleField;
import com.speedment.runtime.field.DoubleForeignKeyField;
import com.speedment.runtime.field.internal.comparator.DoubleFieldComparator;
import com.speedment.runtime.field.internal.comparator.DoubleFieldComparatorImpl;
import com.speedment.runtime.field.internal.method.BackwardFinderImpl;
import com.speedment.runtime.field.internal.method.FindFromDouble;
import com.speedment.runtime.field.internal.method.GetDoubleImpl;
import com.speedment.runtime.field.internal.predicate.doubles.DoubleBetweenPredicate;
import com.speedment.runtime.field.internal.predicate.doubles.DoubleEqualPredicate;
import com.speedment.runtime.field.internal.predicate.doubles.DoubleGreaterOrEqualPredicate;
import com.speedment.runtime.field.internal.predicate.doubles.DoubleGreaterThanPredicate;
import com.speedment.runtime.field.internal.predicate.doubles.DoubleInPredicate;
import com.speedment.runtime.field.internal.predicate.doubles.DoubleLessOrEqualPredicate;
import com.speedment.runtime.field.internal.predicate.doubles.DoubleLessThanPredicate;
import com.speedment.runtime.field.internal.predicate.doubles.DoubleNotBetweenPredicate;
import com.speedment.runtime.field.internal.predicate.doubles.DoubleNotEqualPredicate;
import com.speedment.runtime.field.internal.predicate.doubles.DoubleNotInPredicate;
import com.speedment.runtime.field.method.BackwardFinder;
import com.speedment.runtime.field.method.DoubleGetter;
import com.speedment.runtime.field.method.DoubleSetter;
import com.speedment.runtime.field.method.FindFrom;
import com.speedment.runtime.field.method.GetDouble;
import com.speedment.runtime.field.predicate.FieldPredicate;
import com.speedment.runtime.field.predicate.Inclusion;
import com.speedment.runtime.typemapper.TypeMapper;
import java.util.Collection;
import java.util.function.Predicate;
import java.util.function.Supplier;
import java.util.stream.Stream;
import static com.speedment.runtime.field.internal.util.CollectionUtil.collectionToSet;
import static java.util.Objects.requireNonNull;
/**
* Default implementation of the {@link DoubleField}-interface.
*
* @param entity type
* @param database type
* @param foreign entity type
*
* @author Emil Forslund
* @since 3.0.0
*/
@GeneratedCode(value = "Speedment")
public final class DoubleForeignKeyFieldImpl implements DoubleField, DoubleForeignKeyField {
private final ColumnIdentifier identifier;
private final GetDouble getter;
private final DoubleSetter setter;
private final DoubleField referenced;
private final TypeMapper typeMapper;
private final boolean unique;
public DoubleForeignKeyFieldImpl(
ColumnIdentifier identifier,
DoubleGetter getter,
DoubleSetter setter,
DoubleField referenced,
TypeMapper typeMapper,
boolean unique) {
this.identifier = requireNonNull(identifier);
this.getter = new GetDoubleImpl<>(this, getter);
this.setter = requireNonNull(setter);
this.referenced = requireNonNull(referenced);
this.typeMapper = requireNonNull(typeMapper);
this.unique = unique;
}
@Override
public ColumnIdentifier identifier() {
return identifier;
}
@Override
public DoubleSetter setter() {
return setter;
}
@Override
public GetDouble getter() {
return getter;
}
@Override
public DoubleField getReferencedField() {
return referenced;
}
@Override
public BackwardFinder backwardFinder(TableIdentifier identifier, Supplier> streamSupplier) {
return new BackwardFinderImpl<>(this, identifier, streamSupplier);
}
@Override
public FindFrom finder(TableIdentifier identifier, Supplier> streamSupplier) {
return new FindFromDouble<>(this, referenced, identifier, streamSupplier);
}
@Override
public TypeMapper typeMapper() {
return typeMapper;
}
@Override
public boolean isUnique() {
return unique;
}
@Override
public DoubleFieldComparator comparator() {
return new DoubleFieldComparatorImpl<>(this);
}
@Override
public DoubleFieldComparator comparatorNullFieldsFirst() {
return comparator();
}
@Override
public DoubleFieldComparator comparatorNullFieldsLast() {
return comparator();
}
@Override
public FieldPredicate equal(Double value) {
return new DoubleEqualPredicate<>(this, value);
}
@Override
public FieldPredicate greaterThan(Double value) {
return new DoubleGreaterThanPredicate<>(this, value);
}
@Override
public FieldPredicate greaterOrEqual(Double value) {
return new DoubleGreaterOrEqualPredicate<>(this, value);
}
@Override
public FieldPredicate between(Double start, Double end, Inclusion inclusion) {
return new DoubleBetweenPredicate<>(this, start, end, inclusion);
}
@Override
public FieldPredicate in(Collection values) {
return new DoubleInPredicate<>(this, collectionToSet(values));
}
@Override
public Predicate notEqual(Double value) {
return new DoubleNotEqualPredicate<>(this, value);
}
@Override
public Predicate lessOrEqual(Double value) {
return new DoubleLessOrEqualPredicate<>(this, value);
}
@Override
public Predicate lessThan(Double value) {
return new DoubleLessThanPredicate<>(this, value);
}
@Override
public Predicate notBetween(Double start, Double end, Inclusion inclusion) {
return new DoubleNotBetweenPredicate<>(this, start, end, inclusion);
}
@Override
public Predicate notIn(Collection values) {
return new DoubleNotInPredicate<>(this, collectionToSet(values));
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy