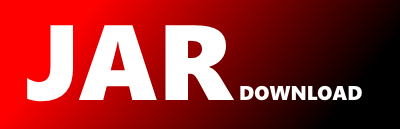
com.speedment.runtime.field.trait.HasStringOperators Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of runtime-field Show documentation
Show all versions of runtime-field Show documentation
Partly generated model of the fields that represent columns in the
database. Fields can be used to produce special predicates and functions
that contain metadata that Speedment can analyze runtime.
/**
*
* Copyright (c) 2006-2017, Speedment, Inc. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"); You may not
* use this file except in compliance with the License. You may obtain a copy of
* the License at:
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS, WITHOUT
* WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. See the
* License for the specific language governing permissions and limitations under
* the License.
*/
package com.speedment.runtime.field.trait;
import java.util.function.Predicate;
/**
* A representation of an Entity field that is a {@code String} type. String
* fields have additional methods that makes it easier to create string-related
* predicates.
*
* @param the entity type
* @param the database type
*
* @author Per Minborg
* @author Emil Forslund
* @since 2.2.0
*/
public interface HasStringOperators {
/**
* Returns a {@link java.util.function.Predicate} that will evaluate to
* {@code true}, if and only if this Field is equal to the given
* value while ignoring the case of the Strings that are compared.
*
* @param value to compare
* @return a Predicate that will evaluate to {@code true}, if and only if
* this Field is equal to the given value while ignoring the case
* of the Strings that are compared
*
* @see String#compareToIgnoreCase(java.lang.String)
*/
Predicate equalIgnoreCase(String value);
/**
* Returns a {@link java.util.function.Predicate} that will evaluate to
* {@code true}, if and only if this Field is not equal to the
* given value while ignoring the case of the Strings that are compared.
*
* @param value to compare
* @return a Predicate that will evaluate to {@code true}, if and only if
* this Field is not equal to the given value while ignoring the
* case of the Strings that are compared
*
* @see String#compareToIgnoreCase(java.lang.String)
*/
default Predicate notEqualIgnoreCase(String value) {
return equalIgnoreCase(value).negate();
}
/**
* Returns a {@link java.util.function.Predicate} that will evaluate to
* {@code true}, if and only if this Field starts with the given
* value.
*
* @param value to compare
* @return a Predicate that will evaluate to {@code true}, if and only if
* this Field starts with the given value
*
* @see String#startsWith(java.lang.String)
*/
Predicate startsWith(String value);
/**
* Returns a {@link java.util.function.Predicate} that will evaluate to
* {@code true}, if and only if this Field not starts with the
* given value.
*
* @param value to compare
* @return a Predicate that will evaluate to {@code true}, if and only if
* this Field not starts with the given value
*
* @see String#startsWith(java.lang.String)
*/
default Predicate notStartsWith(String value) {
return startsWith(value).negate();
}
/**
* Returns a {@link java.util.function.Predicate} that will evaluate to
* {@code true}, if and only if this Field ends with the given
* value.
*
* @param value to compare
* @return a Predicate that will evaluate to {@code true}, if and only if
* this Field ends with the given value
*
* @see String#endsWith(java.lang.String)
*/
Predicate endsWith(String value);
/**
* Returns a {@link java.util.function.Predicate} that will evaluate to
* {@code true}, if and only if this Field not ends with the given
* value.
*
* @param value to compare
* @return a Predicate that will evaluate to {@code true}, if and only if
* this Field not ends with the given value
*
* @see String#endsWith(java.lang.String)
*/
default Predicate notEndsWith(String value) {
return endsWith(value).negate();
}
/**
* Returns a {@link java.util.function.Predicate} that will evaluate to
* {@code true}, if and only if this Field contains the given
* value.
*
* @param value to compare
* @return a Predicate that will evaluate to {@code true}, if and only if
* this Field contains the given value
*
* @see String#contains(java.lang.CharSequence)
*/
Predicate contains(String value);
/**
* Returns a {@link java.util.function.Predicate} that will evaluate to
* {@code true}, if and only if this Field not contains the given
* value.
*
* @param value to compare
* @return a Predicate that will evaluate to {@code true}, if and only if
* this Field not contains the given value
*
* @see String#contains(java.lang.CharSequence)
*/
default Predicate notContains(String value) {
return contains(value).negate();
}
/**
* Returns a {@link java.util.function.Predicate} that will evaluate to
* {@code true}, if and only if this Field is empty. An empty Field
* contains a String with length zero.
*
* @return a Predicate that will evaluate to {@code true}, if and only if
* this Field is empty
*
* @see String#isEmpty()
*/
Predicate isEmpty();
/**
* Returns a {@link java.util.function.Predicate} that will evaluate to
* {@code true}, if and only if this Field is not empty. An empty
* Field contains a String with length zero.
*
* @return a Predicate that will evaluate to {@code true}, if and only if
* this Field is not empty
*
* @see String#isEmpty()
*/
default Predicate isNotEmpty() {
return isEmpty().negate();
}
/**
* Returns a {@link java.util.function.Predicate} that will evaluate to
* {@code true}, if and only if this Field starts with the given
* value while ignoring the case of the Strings that are compared.
*
* @param value to compare
* @return a Predicate that will evaluate to {@code true}, if and only if
* this Field starts with the given value while ignoring the case
* of the Strings that are compared
*
* @see String#startsWith(java.lang.String)
*/
Predicate startsWithIgnoreCase(String value);
/**
* Returns a {@link java.util.function.Predicate} that will evaluate to
* {@code true}, if and only if this Field not starts with the
* given value while ignoring the case of the Strings that are compared.
*
* @param value to compare
* @return a Predicate that will evaluate to {@code true}, if and only if
* this Field not starts with the given value while ignoring the
* case of the Strings that are compared
*
* @see String#startsWith(java.lang.String)
*/
default Predicate notStartsWithIgnoreCase(String value) {
return startsWithIgnoreCase(value).negate();
}
/**
* Returns a {@link java.util.function.Predicate} that will evaluate to
* {@code true}, if and only if this Field ends with the given
* value while ignoring the case of the Strings that are compared.
*
* @param value to compare
* @return a Predicate that will evaluate to {@code true}, if and only if
* this Field ends with the given value while ignoring the case of
* the Strings that are compared
*
* @see String#endsWith(java.lang.String)
*/
Predicate endsWithIgnoreCase(String value);
/**
* Returns a {@link java.util.function.Predicate} that will evaluate to
* {@code true}, if and only if this Field not ends with the given
* value while ignoring the case of the Strings that are compared.
*
* @param value to compare
* @return a Predicate that will evaluate to {@code true}, if and only if
* this Field not ends with the given value while ignoring the case
* of the Strings that are compared
*
* @see String#startsWith(java.lang.String)
*/
default Predicate notEndsWithIgnoreCase(String value) {
return endsWithIgnoreCase(value).negate();
}
/**
* Returns a {@link java.util.function.Predicate} that will evaluate to
* {@code true}, if and only if this Field contains the given value
* while ignoring the case of the Strings that are compared.
*
* @param value to compare
* @return a Predicate that will evaluate to {@code true}, if and only if
* this Field contains the given value while ignoring the case of
* the Strings that are compared
*
* @see String#contains(java.lang.CharSequence)
*/
Predicate containsIgnoreCase(String value);
/**
* Returns a {@link java.util.function.Predicate} that will evaluate to
* {@code true}, if and only if this Field does not contain the
* given value while ignoring the case of the Strings that are compared.
*
* @param value to compare
* @return a Predicate that will evaluate to {@code true}, if and only if
* this Field does not contain the given value while ignoring the
* case of the Strings that are compared
*
* @see String#contains(java.lang.CharSequence)
*/
default Predicate notContainsIgnoreCase(String value) {
return containsIgnoreCase(value).negate();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy