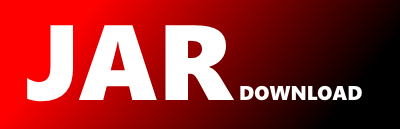
com.speedment.runtime.field.internal.predicate.bytes.ByteBetweenPredicate Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of runtime-field Show documentation
Show all versions of runtime-field Show documentation
Partly generated model of the fields that represent columns in the
database. Fields can be used to produce special predicates and functions
that contain metadata that Speedment can analyze runtime.
/**
*
* Copyright (c) 2006-2017, Speedment, Inc. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"); You may not
* use this file except in compliance with the License. You may obtain a copy of
* the License at:
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS, WITHOUT
* WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. See the
* License for the specific language governing permissions and limitations under
* the License.
*/
package com.speedment.runtime.field.internal.predicate.bytes;
import com.speedment.common.annotation.GeneratedCode;
import com.speedment.common.tuple.Tuple2;
import com.speedment.runtime.field.internal.predicate.AbstractFieldPredicate;
import com.speedment.runtime.field.internal.predicate.BetweenPredicate;
import com.speedment.runtime.field.predicate.Inclusion;
import com.speedment.runtime.field.predicate.PredicateType;
import com.speedment.runtime.field.trait.HasByteValue;
import static java.util.Objects.requireNonNull;
/**
* A predicate that evaluates if a value is between two bytes.
*
* @param entity type
* @param database type
*
* @author Emil Forslund
* @since 3.0.0
*/
@GeneratedCode(value = "Speedment")
public final class ByteBetweenPredicate
extends AbstractFieldPredicate>
implements BetweenPredicate,
Tuple2 {
private final byte start;
private final byte end;
private final Inclusion inclusion;
public ByteBetweenPredicate(
HasByteValue field,
byte start,
byte end,
Inclusion inclusion) {
super(PredicateType.BETWEEN, field, entity -> {
final byte fieldValue = field.getAsByte(entity);
switch (inclusion) {
case START_EXCLUSIVE_END_EXCLUSIVE :
return (start < fieldValue && end > fieldValue);
case START_EXCLUSIVE_END_INCLUSIVE :
return (start < fieldValue && end >= fieldValue);
case START_INCLUSIVE_END_EXCLUSIVE :
return (start <= fieldValue && end > fieldValue);
case START_INCLUSIVE_END_INCLUSIVE :
return (start <= fieldValue && end >= fieldValue);
default : throw new IllegalStateException("Inclusion unknown: " + inclusion);
}
});
this.start = start;
this.end = end;
this.inclusion = requireNonNull(inclusion);
}
@Override
public Byte get0() {
return start;
}
@Override
public Byte get1() {
return end;
}
@Override
public Inclusion getInclusion() {
return inclusion;
}
@Override
public ByteNotBetweenPredicate negate() {
return new ByteNotBetweenPredicate<>(getField(), start, end, inclusion);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy