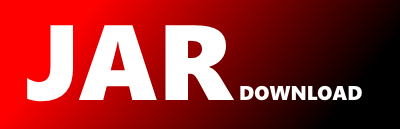
com.speedment.runtime.field.trait.HasComparableOperators Maven / Gradle / Ivy
Show all versions of runtime-field Show documentation
/**
*
* Copyright (c) 2006-2017, Speedment, Inc. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"); You may not
* use this file except in compliance with the License. You may obtain a copy of
* the License at:
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS, WITHOUT
* WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. See the
* License for the specific language governing permissions and limitations under
* the License.
*/
package com.speedment.runtime.field.trait;
import com.speedment.runtime.field.Field;
import com.speedment.runtime.field.comparator.FieldComparator;
import com.speedment.runtime.field.predicate.Inclusion;
import java.util.Collection;
import java.util.Comparator;
import java.util.function.Predicate;
import java.util.stream.Stream;
import static java.util.stream.Collectors.toSet;
/**
* A representation of an Entity field that is a reference type (e.g.
* {@code Integer} and not {@code int}) and that implements {@link Comparable}.
*
* @param the entity type
* @param the field value type
*
* @author Per Minborg
* @author Emil Forslund
* @since 2.2.0
*/
public interface HasComparableOperators>
extends Field {
/**
* Returns a {@link Comparator} that will compare to this field using this
* fields natural order.
*
* @return a {@link Comparator} that will compare to this field using this
* fields natural order
* @throws NullPointerException if a field is null
*/
FieldComparator comparator();
/**
* Returns a {@link Comparator} that will compare to this field using this
* fields natural order, null fields are sorted first.
*
* @return a {@link Comparator} that will compare to this field using this
* fields natural order, null fields are sorted first
*/
FieldComparator comparatorNullFieldsFirst();
/**
* Returns a {@link Comparator} that will compare to this field using this
* fields natural order, null fields are sorted last.
*
* @return a {@link Comparator} that will compare to this field using this
* fields natural order, null fields are sorted last
*
*
* @deprecated This method is the same as comparator(). Use that method
* instead. This method will be removed in coming API versions.
*/
@Deprecated
default FieldComparator comparatorNullFieldsLast() {
return comparator();
}
/**
* Returns a {@link java.util.function.Predicate} that will evaluate to
* {@code true}, if and only if this Field is equal to the given
* value.
*
* @param value to compare
* @return a Predicate that will evaluate to {@code true}, if and only if
* this Field is equal to the given value
*/
Predicate equal(V value);
/**
* Returns a {@link java.util.function.Predicate} that will evaluate to
* {@code true}, if and only if this Field is not equal to the
* given value.
*
* @param value to compare
* @return a Predicate that will evaluate to {@code true}, if and only if
* this Field is not equal to the given value
*/
Predicate notEqual(V value);
/**
* Returns a {@link java.util.function.Predicate} that will evaluate to
* {@code true}, if and only if this Field is less than the given
* value.
*
* If the specified value is {@code null}, the returned predicate will
* always return {@code false}.
*
* @param value to compare
* @return a Predicate that will evaluate to {@code true}, if and only if
* this Field is less than the given value
*/
Predicate lessThan(V value);
/**
* Returns a {@link java.util.function.Predicate} that will evaluate to
* {@code true}, if and only if this Field is less than or equal
* to the given value.
*
* If the specified value is {@code null}, the returned predicate will only
* return {@code true} for {@code null} values.
*
* @param value to compare
* @return a Predicate that will evaluate to {@code true}, if and only if
* this Field is less than or equal to the given value
*/
Predicate lessOrEqual(V value);
/**
* Returns a {@link java.util.function.Predicate} that will evaluate to
* {@code true}, if and only if this Field is greater than
* the given value. If the specified value is {@code null}, the returned
* predicate will always return {@code false}.
*
* @param value to compare
* @return a Predicate that will evaluate to {@code true}, if and only if
* this Field is greater than the given value
*/
Predicate greaterThan(V value);
/**
* Returns a {@link java.util.function.Predicate} that will evaluate to
* {@code true}, if and only if this Field is greater than or equal
* to the given value.
*
* If the specified value is {@code null}, the returned predicate will only
* return {@code true} for {@code null} values.
*
* @param value to compare
* @return a Predicate that will evaluate to {@code true}, if and only if
* this Field is greater than or equal to the given value
*/
Predicate greaterOrEqual(V value);
/**
* Returns a {@link java.util.function.Predicate} that will evaluate to
* {@code true}, if and only if this Field is between
* the given values (inclusive the start value but exclusive the end value).
*
* N.B. if the start value is greater or equal to the end value, then the
* returned Predicate will always evaluate to {@code false}.
*
* @param start to compare as a start value
* @param end to compare as an end value
* @return a Predicate that will evaluate to {@code true}, if and only if
* this Field is between the given values (inclusive the start
* value but exclusive the end value)
*/
default Predicate between(V start, V end) {
return between(start, end, Inclusion.START_INCLUSIVE_END_EXCLUSIVE);
}
/**
* Returns a {@link java.util.function.Predicate} that will evaluate to
* {@code true}, if and only if this Field is between
* the given values and taking the Inclusion parameter into account when
* determining if either of the end points shall be included in the Field
* range or not.
*
* N.B. if the start value is greater than the end value, then the returned
* Predicate will always evaluate to {@code false}
*
* @param start to compare as a start value
* @param end to compare as an end value
* @param inclusion determines if the end points is included in the Field
* range.
* @return a Predicate that will evaluate to {@code true}, if and only if
* this Field is between the given values and taking the Inclusion
* parameter into account when determining if either of the end points shall
* be included in the Field range or not
*/
Predicate between(V start, V end, Inclusion inclusion);
/**
* Returns a {@link java.util.function.Predicate} that will evaluate to
* {@code true}, if and only if this Field is not between
* the given values (inclusive the start value but exclusive the end value).
*
* N.B. if the start value is greater than the end value, then the returned
* Predicate will always evaluate to {@code true}
*
* @param start to compare as a start value
* @param end to compare as an end value
* @return a Predicate that will evaluate to {@code true}, if and only if
* this Field is not between the given values (inclusive the start
* value but exclusive the end value)
*/
default Predicate notBetween(V start, V end) {
return notBetween(start, end, Inclusion.START_INCLUSIVE_END_EXCLUSIVE);
}
/**
* Returns a {@link java.util.function.Predicate} that will evaluate to
* {@code true}, if and only if this Field is not between
* the given values and taking the Inclusion parameter into account when
* determining if either of the end points shall be included in the Field
* range or not.
*
* N.B. if the start value is greater than the end value, then the returned
* Predicate will always evaluate to {@code true}
*
* @param start to compare as a start value
* @param end to compare as an end value
* @param inclusion determines if the end points is included in the Field
* range.
* @return a Predicate that will evaluate to {@code true}, if and only if
* this Field is not between the given values and taking the
* Inclusion parameter into account when determining if either of the end
* points shall be included in the Field range or not
*/
Predicate notBetween(V start, V end, Inclusion inclusion);
/**
* Returns a {@link java.util.function.Predicate} that will evaluate to
* {@code true}, if and only if this Field is in the set of given
* values.
*
* N.B. if no values are given, then the returned Predicate will always
* evaluate to {@code false}
*
* @param values the set of values to match towards this Field
* @return a Predicate that will evaluate to {@code true}, if and only if
* this Field is in the set of given values
*/
@SuppressWarnings("unchecked")
default Predicate in(V... values) {
return in(Stream.of(values).collect(toSet()));
}
/**
* Returns a {@link java.util.function.Predicate} that will evaluate to
* {@code true}, if and only if this Field is in the given set. (If
* the collection is not a set, then a set will be created temporarily from
* the values of the collection).
*
* N.B. if the Set is empty, then the returned Predicate will always
* evaluate to {@code false}
*
* @param values the set of values to match towards this Field
* @return a Predicate that will evaluate to {@code true}, if and only if
* this Field is in the given Set
*/
Predicate in(Collection values);
/**
* Returns a {@link java.util.function.Predicate} that will evaluate to
* {@code true}, if and only if this Field is not in the set of
* given values.
*
* N.B. if no values are given, then the returned Predicate will always
* evaluate to {@code true}
*
* @param values the set of values to match towards this Field
* @return a Predicate that will evaluate to {@code true}, if and only if
* this Field is not in the set of given values
*/
@SuppressWarnings("unchecked")
default Predicate notIn(V... values) {
return notIn(Stream.of(values).collect(toSet()));
}
/**
* Returns a {@link java.util.function.Predicate} that will evaluate to
* {@code true}, if and only if this Field is not in the given Set.
* (If the collection is not a set, then a set will be created temporarily
* from the values of the collection).
*
* N.B. if the Set is empty, then the returned Predicate will always
* evaluate to {@code true}
*
* @param values the set of values to match towards this Field
* @return a Predicate that will evaluate to {@code true}, if and only if
* this Field is not in the given Set
*/
Predicate notIn(Collection values);
}