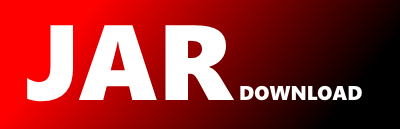
com.sporniket.libre.test.utils.ResourceHelper Maven / Gradle / Ivy
/**
*
*/
package com.sporniket.libre.test.utils;
import static java.lang.String.format;
import java.io.File;
import java.io.InputStream;
/**
* Helpers to load a ressource located in a folder next to the source class.
*
*
* For a class with the full name com.foo.GreatTest
, the files will be located in a folder named
* com/foo/GreatTest_data
, starting from the classloader root.
*
*
*
*
* © Copyright 2020 David Sporn
*
*
*
*
* This file is part of The Sporniket Testing Library – utils.
*
*
* The Sporniket Testing Library – utils is free software: you can redistribute it and/or modify it under the terms of
* the GNU Lesser General Public License as published by the Free Software Foundation, either version 3 of the License, or (at your
* option) any later version.
*
*
* The Sporniket Testing Library – utils is distributed in the hope that it will be useful, but WITHOUT ANY WARRANTY;
* without even the implied warranty of MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the GNU Lesser General Public
* License for more details.
*
*
* You should have received a copy of the GNU Lesser General Public License along with The Sporniket Testing Library –
* utils. If not, see http://www.gnu.org/licenses/. 2
*
*
*
* @author David SPORN
* @version 20.04.00
* @since 20.04.00
*/
public class ResourceHelper
{
private static final String FORMAT__DATA_FILE_PATH = "%s_data/%s";
/**
* Load a resource located in a folder next to the location of the client class.
*
*
* For a client that is an instance of a class with the full name com.foo.GreatTest
, the files will be located in a
* folder named com/foo/GreatTest_data
, starting from the classloader root.
*
*
* @param client
* either the object, or the class of the object.
* @param resourceName
* the name of the file
* @return an input stream from the file, or null
if the file was not found.
*/
public static InputStream getDataResource(final Object client, final String resourceName)
{
final Class extends Object> _class = (client instanceof Class>) ? (Class>) client : client.getClass();
return getDataResourceForClass(_class, resourceName);
}
/**
* Load a resource located in a folder next to the location of the given class.
*
*
*
* For a class with the full name com.foo.GreatTest
, the files will be located in a folder named
* com/foo/GreatTest_data
, starting from the classloader root.
*
*
* This will be the only way to load a resource file for java.lang.Class
.
*
*
* @param clientClass
* the class of the client object.
* @param resourceName
* the name of the file
* @return an input stream from the file, or null
if the file was not found.
*/
public static InputStream getDataResourceForClass(final Class extends Object> clientClass, String resourceName)
{
final String _name = clientClass.getName();
final String _path = format(FORMAT__DATA_FILE_PATH, _name.replace('.', File.separatorChar), resourceName);
return clientClass.getClassLoader().getResourceAsStream(_path);
}
}