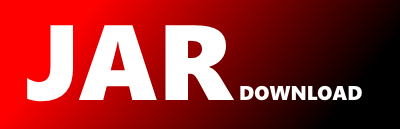
com.spotify.mobius.MobiusLoopController Maven / Gradle / Ivy
/*
* -\-\-
* Mobius
* --
* Copyright (c) 2017-2018 Spotify AB
* --
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
* -/-/-
*/
package com.spotify.mobius;
import static com.spotify.mobius.internal_util.Preconditions.checkNotNull;
import com.spotify.mobius.functions.Consumer;
import com.spotify.mobius.runners.WorkRunner;
import javax.annotation.Nonnull;
import javax.annotation.Nullable;
class MobiusLoopController
implements MobiusLoop.Controller, ControllerActions {
private final MobiusLoop.Factory loopFactory;
private final M defaultModel;
@Nullable private final Init init;
private final WorkRunner mainThreadRunner;
private ControllerStateBase currentState;
MobiusLoopController(
MobiusLoop.Factory loopFactory,
M defaultModel,
@Nullable Init init,
WorkRunner mainThreadRunner) {
this.loopFactory = checkNotNull(loopFactory);
this.defaultModel = checkNotNull(defaultModel);
this.init = init;
this.mainThreadRunner = checkNotNull(mainThreadRunner);
goToStateInit(defaultModel);
}
@Override
public synchronized boolean isRunning() {
return currentState.isRunning();
}
private synchronized void dispatchEvent(E event) {
currentState.onDispatchEvent(event);
}
private synchronized void updateView(M model) {
currentState.onUpdateView(model);
}
@Override
public synchronized void connect(Connectable view) {
currentState.onConnect(checkNotNull(view));
}
@Override
public synchronized void disconnect() {
currentState.onDisconnect();
}
@Override
public synchronized void start() {
currentState.onStart();
}
@Override
public synchronized void stop() {
currentState.onStop();
}
@Override
public synchronized void replaceModel(M model) {
checkNotNull(model);
currentState.onReplaceModel(model);
}
@Override
@Nonnull
public synchronized M getModel() {
return currentState.onGetModel();
}
public void postUpdateView(final M model) {
mainThreadRunner.post(
new Runnable() {
@Override
public void run() {
updateView(model);
}
});
}
@Override
public synchronized void goToStateInit(M nextModelToStartFrom) {
currentState = new ControllerStateInit<>(this, nextModelToStartFrom);
}
@Override
public synchronized void goToStateCreated(
Connection renderer, @Nullable M nextModelToStartFrom) {
if (nextModelToStartFrom == null) {
nextModelToStartFrom = defaultModel;
}
currentState = new ControllerStateCreated(this, renderer, nextModelToStartFrom);
}
@Override
public void goToStateCreated(Connectable view, M nextModelToStartFrom) {
SafeConnectable safeModelHandler = new SafeConnectable<>(checkNotNull(view));
Connection modelConnection =
safeModelHandler.connect(
new Consumer() {
@Override
public void accept(E event) {
dispatchEvent(event);
}
});
goToStateCreated(checkNotNull(modelConnection), nextModelToStartFrom);
}
@Override
public synchronized void goToStateRunning(Connection renderer, M nextModelToStartFrom) {
ControllerStateRunning stateRunning =
new ControllerStateRunning<>(this, renderer, loopFactory, nextModelToStartFrom, init);
currentState = stateRunning;
stateRunning.start();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy