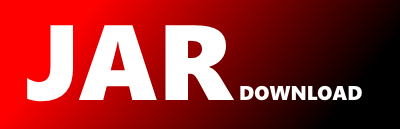
com.spotify.mobius.extras.CompositeLogger Maven / Gradle / Ivy
/*
* -\-\-
* Mobius
* --
* Copyright (c) 2017-2020 Spotify AB
* --
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
* -/-/-
*/
package com.spotify.mobius.extras;
import static com.spotify.mobius.internal_util.Preconditions.checkNotNull;
import com.spotify.mobius.First;
import com.spotify.mobius.MobiusLoop.Logger;
import com.spotify.mobius.Next;
import java.util.ArrayList;
import java.util.Collections;
import java.util.List;
/**
* A {@link Logger} that delegates all logging to a list of provided loggers. Useful if you have
* different types of loggers that you would like to us simultaneously while maintaining single
* responsibility per logger implementation
*
* @param The loop's Model type
* @param The loop's Event type
* @param The loop's Effect type
*/
public class CompositeLogger implements Logger {
@SafeVarargs
public static Logger from(Logger logger, Logger... loggers) {
List> allLoggers = new ArrayList<>();
allLoggers.add(checkNotNull(logger));
for (Logger lg : loggers) {
allLoggers.add(checkNotNull(lg));
}
return new CompositeLogger<>(allLoggers);
}
private final List> loggers;
private final List> loggersReversed;
private CompositeLogger(List> loggers) {
this.loggers = loggers;
this.loggersReversed = new ArrayList<>(loggers);
Collections.reverse(loggersReversed);
}
@Override
public void beforeInit(M model) {
for (Logger logger : loggers) {
logger.beforeInit(model);
}
}
@Override
public void afterInit(M model, First result) {
for (Logger logger : loggersReversed) {
logger.afterInit(model, result);
}
}
@Override
public void exceptionDuringInit(M model, Throwable exception) {
for (Logger logger : loggersReversed) {
logger.exceptionDuringInit(model, exception);
}
}
@Override
public void beforeUpdate(M model, E event) {
for (Logger logger : loggers) {
logger.beforeUpdate(model, event);
}
}
@Override
public void afterUpdate(M model, E event, Next result) {
for (Logger logger : loggersReversed) {
logger.afterUpdate(model, event, result);
}
}
@Override
public void exceptionDuringUpdate(M model, E event, Throwable exception) {
for (Logger logger : loggersReversed) {
logger.exceptionDuringUpdate(model, event, exception);
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy