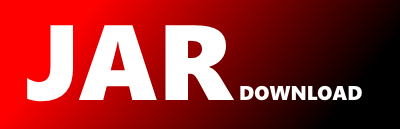
com.spotify.mobius.extras.Program Maven / Gradle / Ivy
/*
* -\-\-
* Mobius
* --
* Copyright (c) 2017-2020 Spotify AB
* --
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
* -/-/-
*/
package com.spotify.mobius.extras;
import com.google.auto.value.AutoValue;
import com.spotify.mobius.Connectable;
import com.spotify.mobius.EventSource;
import com.spotify.mobius.Init;
import com.spotify.mobius.Mobius;
import com.spotify.mobius.MobiusLoop;
import com.spotify.mobius.Update;
import javax.annotation.Nonnull;
import javax.annotation.Nullable;
/**
* This class defines a Mobius program. It holds you your {@link Init}, {@link Update}, {@link
* EventSource}, and {@link Connectable} definitions, as well as the tag you would like to use for
* identifying your program in logs. It is primarily meant to be used for composing programs, in
* most cases you should prefer to use {@link Mobius#loop(Update, Connectable)}.
*/
@AutoValue
public abstract class Program {
@Nonnull
public abstract Update update();
@Nonnull
public abstract Connectable effectHandler();
@Nullable
public abstract Init init();
@Nullable
public abstract EventSource eventSource();
@Nullable
public abstract String loggingTag();
/** Returns a {@link MobiusLoop.Builder} based on this program */
public MobiusLoop.Builder createLoop() {
MobiusLoop.Builder builder = Mobius.loop(update(), effectHandler());
Init init = init();
if (init != null) {
builder = builder.init(init);
}
EventSource eventSource = eventSource();
if (eventSource != null) {
builder = builder.eventSource(eventSource);
}
String loggingTag = loggingTag();
if (loggingTag != null) {
builder = builder.logger(SLF4JLogger.withTag(loggingTag));
}
return builder;
}
public static Builder builder() {
return new AutoValue_Program.Builder<>();
}
@AutoValue.Builder
public abstract static class Builder {
public abstract Builder update(Update update);
public abstract Builder effectHandler(Connectable effectHandler);
public abstract Builder init(Init init);
public abstract Builder eventSource(EventSource eventSource);
public abstract Builder loggingTag(String loggingTag);
public abstract Program build();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy