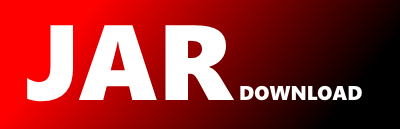
com.spotify.mobius.rx.FlatMapCompletable Maven / Gradle / Ivy
Show all versions of mobius-rx Show documentation
/*
* -\-\-
* Mobius
* --
* Copyright (c) 2017-2020 Spotify AB
* --
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
* -/-/-
*/
package com.spotify.mobius.rx;
import static com.spotify.mobius.internal_util.Preconditions.checkNotNull;
import javax.annotation.Nullable;
import rx.Completable;
import rx.Observable;
import rx.Scheduler;
import rx.functions.Action0;
import rx.functions.Action1;
import rx.functions.Func1;
/**
* Dispatches and executes an Action1 when upstream emits a value.
*
* This is very similar to AsyncDoOnNext, the difference being that this transformer doesn't emit
* any items. This means that you're free to choose what the output type will be - it doesn't matter
* what it is as no items will be emitted.
*
*
Note: as always, be very careful with performing side-effects from inside an Observable
* stream, it is often (but not always) a sign of having overlooked something in how you design the
* chain. Try to push side-effects either to the beginning or the end of the chain.
*/
class FlatMapCompletable implements Observable.Transformer {
private final Func1 func;
@Nullable private final Scheduler scheduler;
private FlatMapCompletable(Func1 func, @Nullable Scheduler scheduler) {
this.func = func;
this.scheduler = scheduler;
}
static FlatMapCompletable createForAction(
final Action1 action, @Nullable Scheduler scheduler) {
return create(
new Func1() {
@Override
public Completable call(final T t) {
return Completable.fromAction(
new Action0() {
@Override
public void call() {
action.call(t);
}
});
}
},
scheduler);
}
static FlatMapCompletable createForAction(final Action1 action) {
return createForAction(action, null);
}
static FlatMapCompletable create(Func1 func) {
return create(func, null);
}
static FlatMapCompletable create(
Func1 func, @Nullable Scheduler scheduler) {
return new FlatMapCompletable<>(checkNotNull(func), scheduler);
}
@Override
public Observable call(Observable observable) {
return observable.flatMap(
new Func1>() {
@Override
public Observable call(final T value) {
Completable completable = func.call(value);
if (scheduler != null) {
completable = completable.subscribeOn(scheduler);
}
return completable
.toObservable()
.ignoreElements()
.map(
new Func1