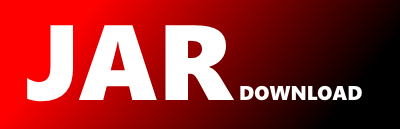
com.spotify.apollo.AutoValue_RequestValue Maven / Gradle / Ivy
package com.spotify.apollo;
import java.time.Duration;
import java.util.List;
import java.util.Map;
import java.util.Optional;
import javax.annotation.Generated;
import okio.ByteString;
@Generated("com.google.auto.value.processor.AutoValueProcessor")
final class AutoValue_RequestValue extends RequestValue {
private final String method;
private final String uri;
private final Map> parameters;
private final Optional service;
private final Optional payload;
private final Headers internalHeadersImpl;
private final Optional ttl;
AutoValue_RequestValue(
String method,
String uri,
Map> parameters,
Optional service,
Optional payload,
Headers internalHeadersImpl,
Optional ttl) {
if (method == null) {
throw new NullPointerException("Null method");
}
this.method = method;
if (uri == null) {
throw new NullPointerException("Null uri");
}
this.uri = uri;
if (parameters == null) {
throw new NullPointerException("Null parameters");
}
this.parameters = parameters;
if (service == null) {
throw new NullPointerException("Null service");
}
this.service = service;
if (payload == null) {
throw new NullPointerException("Null payload");
}
this.payload = payload;
if (internalHeadersImpl == null) {
throw new NullPointerException("Null internalHeadersImpl");
}
this.internalHeadersImpl = internalHeadersImpl;
if (ttl == null) {
throw new NullPointerException("Null ttl");
}
this.ttl = ttl;
}
@Override
public String method() {
return method;
}
@Override
public String uri() {
return uri;
}
@Override
public Map> parameters() {
return parameters;
}
@Override
public Optional service() {
return service;
}
@Override
public Optional payload() {
return payload;
}
@Override
Headers internalHeadersImpl() {
return internalHeadersImpl;
}
@Override
public Optional ttl() {
return ttl;
}
@Override
public String toString() {
return "RequestValue{"
+ "method=" + method + ", "
+ "uri=" + uri + ", "
+ "parameters=" + parameters + ", "
+ "service=" + service + ", "
+ "payload=" + payload + ", "
+ "internalHeadersImpl=" + internalHeadersImpl + ", "
+ "ttl=" + ttl
+ "}";
}
@Override
public boolean equals(Object o) {
if (o == this) {
return true;
}
if (o instanceof RequestValue) {
RequestValue that = (RequestValue) o;
return (this.method.equals(that.method()))
&& (this.uri.equals(that.uri()))
&& (this.parameters.equals(that.parameters()))
&& (this.service.equals(that.service()))
&& (this.payload.equals(that.payload()))
&& (this.internalHeadersImpl.equals(that.internalHeadersImpl()))
&& (this.ttl.equals(that.ttl()));
}
return false;
}
@Override
public int hashCode() {
int h$ = 1;
h$ *= 1000003;
h$ ^= method.hashCode();
h$ *= 1000003;
h$ ^= uri.hashCode();
h$ *= 1000003;
h$ ^= parameters.hashCode();
h$ *= 1000003;
h$ ^= service.hashCode();
h$ *= 1000003;
h$ ^= payload.hashCode();
h$ *= 1000003;
h$ ^= internalHeadersImpl.hashCode();
h$ *= 1000003;
h$ ^= ttl.hashCode();
return h$;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy