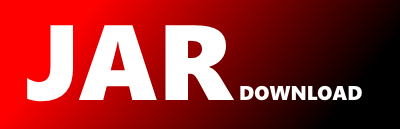
com.spotify.apollo.route.AutoValue_RouteImpl Maven / Gradle / Ivy
package com.spotify.apollo.route;
import java.util.Optional;
import javax.annotation.Generated;
@Generated("com.google.auto.value.processor.AutoValueProcessor")
final class AutoValue_RouteImpl extends RouteImpl {
private final String method;
private final String uri;
private final H handler;
private final Optional docString;
AutoValue_RouteImpl(
String method,
String uri,
H handler,
Optional docString) {
if (method == null) {
throw new NullPointerException("Null method");
}
this.method = method;
if (uri == null) {
throw new NullPointerException("Null uri");
}
this.uri = uri;
if (handler == null) {
throw new NullPointerException("Null handler");
}
this.handler = handler;
if (docString == null) {
throw new NullPointerException("Null docString");
}
this.docString = docString;
}
@Override
public String method() {
return method;
}
@Override
public String uri() {
return uri;
}
@Override
public H handler() {
return handler;
}
@Override
public Optional docString() {
return docString;
}
@Override
public String toString() {
return "RouteImpl{"
+ "method=" + method + ", "
+ "uri=" + uri + ", "
+ "handler=" + handler + ", "
+ "docString=" + docString
+ "}";
}
@Override
public boolean equals(Object o) {
if (o == this) {
return true;
}
if (o instanceof RouteImpl) {
RouteImpl> that = (RouteImpl>) o;
return (this.method.equals(that.method()))
&& (this.uri.equals(that.uri()))
&& (this.handler.equals(that.handler()))
&& (this.docString.equals(that.docString()));
}
return false;
}
@Override
public int hashCode() {
int h = 1;
h *= 1000003;
h ^= method.hashCode();
h *= 1000003;
h ^= uri.hashCode();
h *= 1000003;
h ^= handler.hashCode();
h *= 1000003;
h ^= docString.hashCode();
return h;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy