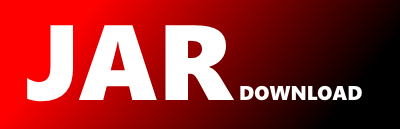
com.spotify.asyncdatastoreclient.Value Maven / Gradle / Ivy
/*
* Copyright (c) 2011-2015 Spotify AB
*
* Licensed under the Apache License, Version 2.0 (the "License"); you may not
* use this file except in compliance with the License. You may obtain a copy of
* the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS, WITHOUT
* WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. See the
* License for the specific language governing permissions and limitations under
* the License.
*/
package com.spotify.asyncdatastoreclient;
import com.google.common.collect.ImmutableList;
import com.google.datastore.v1.ArrayValue;
import com.google.protobuf.ByteString;
import com.google.protobuf.Timestamp;
import java.time.Instant;
import java.util.Date;
import java.util.List;
import java.util.Objects;
import java.util.stream.Collectors;
/**
* Represents an entity property value.
*
* A value is immutable; use {@code Value.builder()} to construct new
* {@code Value} instances.
*/
public final class Value {
private final com.google.datastore.v1.Value value;
private Value(final com.google.datastore.v1.Value value) {
this.value = value;
}
public static final class Builder {
private final com.google.datastore.v1.Value.Builder value;
private boolean excludeFromIndexes;
private Builder(com.google.datastore.v1.Value.Builder builder) {
this.value = builder;
}
private Builder() {
this.value = com.google.datastore.v1.Value.newBuilder();
this.excludeFromIndexes = false;
}
private Builder(final Value value) {
this(value.getPb());
}
private Builder(final com.google.datastore.v1.Value value) {
this.value = com.google.datastore.v1.Value.newBuilder(value);
this.excludeFromIndexes = value.getExcludeFromIndexes();
}
/**
* Creates a new {@code Value}.
*
* @return an immutable value.
*/
public Value build() {
return new Value(value.setExcludeFromIndexes(excludeFromIndexes).build());
}
/**
* Set the value for this {@code Value}.
*
* The supplied value must comply with the data types supported by Datastore.
*
* @param value the value to set.
* @return this value builder.
* @throws IllegalArgumentException if supplied {@code value} is not recognised.
* @deprecated Use type-specific builders instead, like {@code Value#fromString(String)}.
*/
public Builder value(final Object value) {
if (value instanceof String) {
this.value.setStringValue((String) value);
} else if (value instanceof Boolean) {
this.value.setBooleanValue((Boolean) value);
} else if (value instanceof Date) {
this.value.setTimestampValue(toTimestamp((Date) value));
} else if (value instanceof ByteString) {
this.value.setBlobValue((ByteString) value);
} else if (value instanceof Entity) {
this.value.setEntityValue(((Entity) value).getPb()).setExcludeFromIndexes(true);
} else if (value instanceof Key) {
this.value.setKeyValue(((Key) value).getPb());
} else if (value instanceof Double) {
this.value.setDoubleValue((Double) value);
} else if (value instanceof Long) {
this.value.setIntegerValue((Long) value);
} else if (value instanceof Float) {
this.value.setDoubleValue(((Float) value).doubleValue());
} else if (value instanceof Integer) {
this.value.setIntegerValue(((Integer) value).longValue());
} else {
throw new IllegalArgumentException("Invalid value type: " + value.getClass());
}
return this;
}
/**
* Set a list of values for this {@code Value}.
*
* The supplied value items must comply with the data types supported by Datastore.
*
* @param values a list of values to set.
* @return this value builder.
* @throws IllegalArgumentException if supplied {@code values} contains types
* that are not recognised.
*/
public Builder value(final List