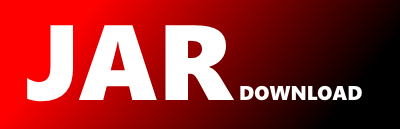
com.spotify.google.cloud.pubsub.client.AcknowledgeRequestBuilder Maven / Gradle / Ivy
package com.spotify.google.cloud.pubsub.client;
import io.norberg.automatter.AutoMatter;
import java.util.ArrayList;
import java.util.Arrays;
import java.util.Collection;
import java.util.Collections;
import java.util.Iterator;
import java.util.List;
import javax.annotation.Generated;
@Generated("io.norberg.automatter.processor.AutoMatterProcessor")
final class AcknowledgeRequestBuilder {
private List ackIds;
public AcknowledgeRequestBuilder() {
}
private AcknowledgeRequestBuilder(AcknowledgeRequest v) {
List extends String> _ackIds = v.ackIds();
this.ackIds = (_ackIds == null) ? null : new ArrayList(_ackIds);
}
private AcknowledgeRequestBuilder(AcknowledgeRequestBuilder v) {
this.ackIds = (v.ackIds == null) ? null : new ArrayList(v.ackIds);
}
public List ackIds() {
if (this.ackIds == null) {
this.ackIds = new ArrayList();
}
return ackIds;
}
public AcknowledgeRequestBuilder ackIds(List extends String> ackIds) {
return ackIds((Collection extends String>) ackIds);
}
public AcknowledgeRequestBuilder ackIds(Collection extends String> ackIds) {
if (ackIds == null) {
throw new NullPointerException("ackIds");
}
for (String item : ackIds) {
if (item == null) {
throw new NullPointerException("ackIds: null item");
}
}
this.ackIds = new ArrayList(ackIds);
return this;
}
public AcknowledgeRequestBuilder ackIds(Iterable extends String> ackIds) {
if (ackIds == null) {
throw new NullPointerException("ackIds");
}
if (ackIds instanceof Collection) {
return ackIds((Collection extends String>) ackIds);
}
return ackIds(ackIds.iterator());
}
public AcknowledgeRequestBuilder ackIds(Iterator extends String> ackIds) {
if (ackIds == null) {
throw new NullPointerException("ackIds");
}
this.ackIds = new ArrayList();
while (ackIds.hasNext()) {
String item = ackIds.next();
if (item == null) {
throw new NullPointerException("ackIds: null item");
}
this.ackIds.add(item);
}
return this;
}
public AcknowledgeRequestBuilder ackIds(String... ackIds) {
if (ackIds == null) {
throw new NullPointerException("ackIds");
}
return ackIds(Arrays.asList(ackIds));
}
public AcknowledgeRequestBuilder addAckId(String ackId) {
if (ackId == null) {
throw new NullPointerException("ackId");
}
if (this.ackIds == null) {
this.ackIds = new ArrayList();
}
ackIds.add(ackId);
return this;
}
public AcknowledgeRequest build() {
List _ackIds = (ackIds != null) ? Collections.unmodifiableList(new ArrayList(ackIds)) : Collections.emptyList();
return new Value(_ackIds);
}
public static AcknowledgeRequestBuilder from(AcknowledgeRequest v) {
return new AcknowledgeRequestBuilder(v);
}
public static AcknowledgeRequestBuilder from(AcknowledgeRequestBuilder v) {
return new AcknowledgeRequestBuilder(v);
}
private static final class Value implements AcknowledgeRequest {
private final List ackIds;
private Value(@AutoMatter.Field("ackIds") List ackIds) {
this.ackIds = (ackIds != null) ? ackIds : Collections.emptyList();
}
@AutoMatter.Field
@Override
public List ackIds() {
return ackIds;
}
public AcknowledgeRequestBuilder builder() {
return new AcknowledgeRequestBuilder(this);
}
@Override
public boolean equals(Object o) {
if (this == o) {
return true;
}
if (!(o instanceof AcknowledgeRequest)) {
return false;
}
final AcknowledgeRequest that = (AcknowledgeRequest) o;
if (ackIds != null ? !ackIds.equals(that.ackIds()) : that.ackIds() != null) {
return false;
}
return true;
}
@Override
public int hashCode() {
int result = 1;
long temp;
result = 31 * result + (ackIds != null ? ackIds.hashCode() : 0);
return result;
}
@Override
public String toString() {
return "AcknowledgeRequest{" +
"ackIds=" + ackIds +
'}';
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy