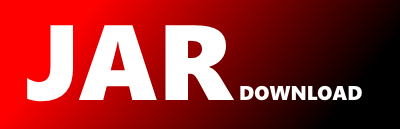
com.spotify.google.cloud.pubsub.client.MessageBuilder Maven / Gradle / Ivy
package com.spotify.google.cloud.pubsub.client;
import io.norberg.automatter.AutoMatter;
import java.time.Instant;
import java.util.Collections;
import java.util.HashMap;
import java.util.Map;
import java.util.Optional;
import javax.annotation.Generated;
@Generated("io.norberg.automatter.processor.AutoMatterProcessor")
public final class MessageBuilder {
private String data;
private Map attributes;
private Optional messageId;
private Optional publishTime;
public MessageBuilder() {
this.messageId = Optional.empty();
this.publishTime = Optional.empty();
}
private MessageBuilder(Message v) {
this.data = v.data();
Map extends String, ? extends String> _attributes = v.attributes();
this.attributes = (_attributes == null) ? null : new HashMap(_attributes);
this.messageId = v.messageId();
this.publishTime = v.publishTime();
}
private MessageBuilder(MessageBuilder v) {
this.data = v.data;
this.attributes = (v.attributes == null) ? null : new HashMap(v.attributes);
this.messageId = v.messageId;
this.publishTime = v.publishTime;
}
public String data() {
return data;
}
public MessageBuilder data(String data) {
if (data == null) {
throw new NullPointerException("data");
}
this.data = data;
return this;
}
public Map attributes() {
if (this.attributes == null) {
this.attributes = new HashMap();
}
return attributes;
}
public MessageBuilder attributes(Map extends String, ? extends String> attributes) {
if (attributes == null) {
throw new NullPointerException("attributes");
}
for (Map.Entry extends String, ? extends String> entry : attributes.entrySet()) {
if (entry.getKey() == null) {
throw new NullPointerException("attributes: null key");
}
if (entry.getValue() == null) {
throw new NullPointerException("attributes: null value");
}
}
this.attributes = new HashMap(attributes);
return this;
}
public MessageBuilder attributes(String k1, String v1) {
if (k1 == null) {
throw new NullPointerException("attributes: k1");
}
if (v1 == null) {
throw new NullPointerException("attributes: v1");
}
attributes = new HashMap();
attributes.put(k1, v1);
return this;
}
public MessageBuilder attributes(String k1, String v1, String k2, String v2) {
attributes(k1, v1);
if (k2 == null) {
throw new NullPointerException("attributes: k2");
}
if (v2 == null) {
throw new NullPointerException("attributes: v2");
}
attributes.put(k2, v2);
return this;
}
public MessageBuilder attributes(String k1, String v1, String k2, String v2, String k3, String v3) {
attributes(k1, v1, k2, v2);
if (k3 == null) {
throw new NullPointerException("attributes: k3");
}
if (v3 == null) {
throw new NullPointerException("attributes: v3");
}
attributes.put(k3, v3);
return this;
}
public MessageBuilder attributes(String k1, String v1, String k2, String v2, String k3, String v3, String k4, String v4) {
attributes(k1, v1, k2, v2, k3, v3);
if (k4 == null) {
throw new NullPointerException("attributes: k4");
}
if (v4 == null) {
throw new NullPointerException("attributes: v4");
}
attributes.put(k4, v4);
return this;
}
public MessageBuilder attributes(String k1, String v1, String k2, String v2, String k3, String v3, String k4, String v4, String k5, String v5) {
attributes(k1, v1, k2, v2, k3, v3, k4, v4);
if (k5 == null) {
throw new NullPointerException("attributes: k5");
}
if (v5 == null) {
throw new NullPointerException("attributes: v5");
}
attributes.put(k5, v5);
return this;
}
public MessageBuilder putAttribute(String key, String value) {
if (key == null) {
throw new NullPointerException("attribute: key");
}
if (value == null) {
throw new NullPointerException("attribute: value");
}
if (this.attributes == null) {
this.attributes = new HashMap();
}
attributes.put(key, value);
return this;
}
public Optional messageId() {
return messageId;
}
public MessageBuilder messageId(String messageId) {
return messageId(Optional.ofNullable(messageId));
}
public MessageBuilder messageId(Optional extends String> messageId) {
if (messageId == null) {
throw new NullPointerException("messageId");
}
this.messageId = (Optional)messageId;
return this;
}
public Optional publishTime() {
return publishTime;
}
public MessageBuilder publishTime(Instant publishTime) {
return publishTime(Optional.ofNullable(publishTime));
}
public MessageBuilder publishTime(Optional extends Instant> publishTime) {
if (publishTime == null) {
throw new NullPointerException("publishTime");
}
this.publishTime = (Optional)publishTime;
return this;
}
public Message build() {
Map _attributes = (attributes != null) ? Collections.unmodifiableMap(new HashMap(attributes)) : Collections.emptyMap();
return new Value(data, _attributes, messageId, publishTime);
}
public static MessageBuilder from(Message v) {
return new MessageBuilder(v);
}
public static MessageBuilder from(MessageBuilder v) {
return new MessageBuilder(v);
}
private static final class Value implements Message {
private final String data;
private final Map attributes;
private final Optional messageId;
private final Optional publishTime;
private Value(@AutoMatter.Field("data") String data, @AutoMatter.Field("attributes") Map attributes, @AutoMatter.Field("messageId") Optional messageId, @AutoMatter.Field("publishTime") Optional publishTime) {
if (data == null) {
throw new NullPointerException("data");
}
if (messageId == null) {
throw new NullPointerException("messageId");
}
if (publishTime == null) {
throw new NullPointerException("publishTime");
}
this.data = data;
this.attributes = (attributes != null) ? attributes : Collections.emptyMap();
this.messageId = messageId;
this.publishTime = publishTime;
}
@AutoMatter.Field
@Override
public String data() {
return data;
}
@AutoMatter.Field
@Override
public Map attributes() {
return attributes;
}
@AutoMatter.Field
@Override
public Optional messageId() {
return messageId;
}
@AutoMatter.Field
@Override
public Optional publishTime() {
return publishTime;
}
public MessageBuilder builder() {
return new MessageBuilder(this);
}
@Override
public boolean equals(Object o) {
if (this == o) {
return true;
}
if (!(o instanceof Message)) {
return false;
}
final Message that = (Message) o;
if (data != null ? !data.equals(that.data()) : that.data() != null) {
return false;
}
if (attributes != null ? !attributes.equals(that.attributes()) : that.attributes() != null) {
return false;
}
if (messageId != null ? !messageId.equals(that.messageId()) : that.messageId() != null) {
return false;
}
if (publishTime != null ? !publishTime.equals(that.publishTime()) : that.publishTime() != null) {
return false;
}
return true;
}
@Override
public int hashCode() {
int result = 1;
long temp;
result = 31 * result + (data != null ? data.hashCode() : 0);
result = 31 * result + (attributes != null ? attributes.hashCode() : 0);
result = 31 * result + (messageId != null ? messageId.hashCode() : 0);
result = 31 * result + (publishTime != null ? publishTime.hashCode() : 0);
return result;
}
@Override
public String toString() {
return "Message{" +
"data=" + data +
", attributes=" + attributes +
", messageId=" + messageId +
", publishTime=" + publishTime +
'}';
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy