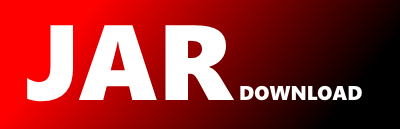
com.spotify.google.cloud.pubsub.client.RequestInfoBuilder Maven / Gradle / Ivy
package com.spotify.google.cloud.pubsub.client;
import io.norberg.automatter.AutoMatter;
import javax.annotation.Generated;
@Generated("io.norberg.automatter.processor.AutoMatterProcessor")
public final class RequestInfoBuilder {
private String operation;
private String method;
private String uri;
private long payloadSize;
public RequestInfoBuilder() {
}
private RequestInfoBuilder(RequestInfo v) {
this.operation = v.operation();
this.method = v.method();
this.uri = v.uri();
this.payloadSize = v.payloadSize();
}
private RequestInfoBuilder(RequestInfoBuilder v) {
this.operation = v.operation;
this.method = v.method;
this.uri = v.uri;
this.payloadSize = v.payloadSize;
}
public String operation() {
return operation;
}
public RequestInfoBuilder operation(String operation) {
if (operation == null) {
throw new NullPointerException("operation");
}
this.operation = operation;
return this;
}
public String method() {
return method;
}
public RequestInfoBuilder method(String method) {
if (method == null) {
throw new NullPointerException("method");
}
this.method = method;
return this;
}
public String uri() {
return uri;
}
public RequestInfoBuilder uri(String uri) {
if (uri == null) {
throw new NullPointerException("uri");
}
this.uri = uri;
return this;
}
public long payloadSize() {
return payloadSize;
}
public RequestInfoBuilder payloadSize(long payloadSize) {
this.payloadSize = payloadSize;
return this;
}
public RequestInfo build() {
return new Value(operation, method, uri, payloadSize);
}
public static RequestInfoBuilder from(RequestInfo v) {
return new RequestInfoBuilder(v);
}
public static RequestInfoBuilder from(RequestInfoBuilder v) {
return new RequestInfoBuilder(v);
}
private static final class Value implements RequestInfo {
private final String operation;
private final String method;
private final String uri;
private final long payloadSize;
private Value(@AutoMatter.Field("operation") String operation, @AutoMatter.Field("method") String method, @AutoMatter.Field("uri") String uri, @AutoMatter.Field("payloadSize") long payloadSize) {
if (operation == null) {
throw new NullPointerException("operation");
}
if (method == null) {
throw new NullPointerException("method");
}
if (uri == null) {
throw new NullPointerException("uri");
}
this.operation = operation;
this.method = method;
this.uri = uri;
this.payloadSize = payloadSize;
}
@AutoMatter.Field
@Override
public String operation() {
return operation;
}
@AutoMatter.Field
@Override
public String method() {
return method;
}
@AutoMatter.Field
@Override
public String uri() {
return uri;
}
@AutoMatter.Field
@Override
public long payloadSize() {
return payloadSize;
}
public RequestInfoBuilder builder() {
return new RequestInfoBuilder(this);
}
@Override
public boolean equals(Object o) {
if (this == o) {
return true;
}
if (!(o instanceof RequestInfo)) {
return false;
}
final RequestInfo that = (RequestInfo) o;
if (operation != null ? !operation.equals(that.operation()) : that.operation() != null) {
return false;
}
if (method != null ? !method.equals(that.method()) : that.method() != null) {
return false;
}
if (uri != null ? !uri.equals(that.uri()) : that.uri() != null) {
return false;
}
if (payloadSize != that.payloadSize()) {
return false;
}
return true;
}
@Override
public int hashCode() {
int result = 1;
long temp;
result = 31 * result + (operation != null ? operation.hashCode() : 0);
result = 31 * result + (method != null ? method.hashCode() : 0);
result = 31 * result + (uri != null ? uri.hashCode() : 0);
result = 31 * result + (int) (payloadSize ^ (payloadSize >>> 32));
return result;
}
@Override
public String toString() {
return "RequestInfo{" +
"operation=" + operation +
", method=" + method +
", uri=" + uri +
", payloadSize=" + payloadSize +
'}';
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy