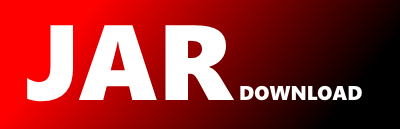
com.spotify.google.cloud.pubsub.client.TopicListBuilder Maven / Gradle / Ivy
package com.spotify.google.cloud.pubsub.client;
import io.norberg.automatter.AutoMatter;
import java.util.ArrayList;
import java.util.Arrays;
import java.util.Collection;
import java.util.Collections;
import java.util.Iterator;
import java.util.List;
import java.util.Optional;
import javax.annotation.Generated;
@Generated("io.norberg.automatter.processor.AutoMatterProcessor")
public final class TopicListBuilder {
private List topics;
private Optional nextPageToken;
public TopicListBuilder() {
this.nextPageToken = Optional.empty();
}
private TopicListBuilder(TopicList v) {
List extends Topic> _topics = v.topics();
this.topics = (_topics == null) ? null : new ArrayList(_topics);
this.nextPageToken = v.nextPageToken();
}
private TopicListBuilder(TopicListBuilder v) {
this.topics = (v.topics == null) ? null : new ArrayList(v.topics);
this.nextPageToken = v.nextPageToken;
}
public List topics() {
if (this.topics == null) {
this.topics = new ArrayList();
}
return topics;
}
public TopicListBuilder topics(List extends Topic> topics) {
return topics((Collection extends Topic>) topics);
}
public TopicListBuilder topics(Collection extends Topic> topics) {
if (topics == null) {
throw new NullPointerException("topics");
}
for (Topic item : topics) {
if (item == null) {
throw new NullPointerException("topics: null item");
}
}
this.topics = new ArrayList(topics);
return this;
}
public TopicListBuilder topics(Iterable extends Topic> topics) {
if (topics == null) {
throw new NullPointerException("topics");
}
if (topics instanceof Collection) {
return topics((Collection extends Topic>) topics);
}
return topics(topics.iterator());
}
public TopicListBuilder topics(Iterator extends Topic> topics) {
if (topics == null) {
throw new NullPointerException("topics");
}
this.topics = new ArrayList();
while (topics.hasNext()) {
Topic item = topics.next();
if (item == null) {
throw new NullPointerException("topics: null item");
}
this.topics.add(item);
}
return this;
}
@SafeVarargs
public final TopicListBuilder topics(Topic... topics) {
if (topics == null) {
throw new NullPointerException("topics");
}
return topics(Arrays.asList(topics));
}
public TopicListBuilder addTopic(Topic topic) {
if (topic == null) {
throw new NullPointerException("topic");
}
if (this.topics == null) {
this.topics = new ArrayList();
}
topics.add(topic);
return this;
}
public Optional nextPageToken() {
return nextPageToken;
}
public TopicListBuilder nextPageToken(String nextPageToken) {
return nextPageToken(Optional.ofNullable(nextPageToken));
}
@SuppressWarnings("unchecked")
public TopicListBuilder nextPageToken(Optional extends String> nextPageToken) {
if (nextPageToken == null) {
throw new NullPointerException("nextPageToken");
}
this.nextPageToken = (Optional)nextPageToken;
return this;
}
public TopicList build() {
List _topics = (topics != null) ? Collections.unmodifiableList(new ArrayList(topics)) : Collections.emptyList();
return new Value(_topics, nextPageToken);
}
public static TopicListBuilder from(TopicList v) {
return new TopicListBuilder(v);
}
public static TopicListBuilder from(TopicListBuilder v) {
return new TopicListBuilder(v);
}
private static final class Value implements TopicList {
private final List topics;
private final Optional nextPageToken;
private Value(@AutoMatter.Field("topics") List topics, @AutoMatter.Field("nextPageToken") Optional nextPageToken) {
if (nextPageToken == null) {
throw new NullPointerException("nextPageToken");
}
this.topics = (topics != null) ? topics : Collections.emptyList();
this.nextPageToken = nextPageToken;
}
@AutoMatter.Field
@Override
public List topics() {
return topics;
}
@AutoMatter.Field
@Override
public Optional nextPageToken() {
return nextPageToken;
}
public TopicListBuilder builder() {
return new TopicListBuilder(this);
}
@Override
public boolean equals(Object o) {
if (this == o) {
return true;
}
if (!(o instanceof TopicList)) {
return false;
}
final TopicList that = (TopicList) o;
if (topics != null ? !topics.equals(that.topics()) : that.topics() != null) {
return false;
}
if (nextPageToken != null ? !nextPageToken.equals(that.nextPageToken()) : that.nextPageToken() != null) {
return false;
}
return true;
}
@Override
public int hashCode() {
int result = 1;
long temp;
result = 31 * result + (topics != null ? topics.hashCode() : 0);
result = 31 * result + (nextPageToken != null ? nextPageToken.hashCode() : 0);
return result;
}
@Override
public String toString() {
return "TopicList{" +
"topics=" + topics +
", nextPageToken=" + nextPageToken +
'}';
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy