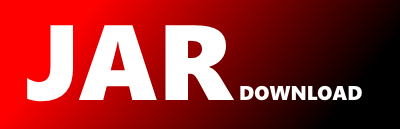
com.spotify.dbeam.args.AutoValue_JdbcAvroArgs Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of dbeam-core Show documentation
Show all versions of dbeam-core Show documentation
Top level DBeam core implementation
package com.spotify.dbeam.args;
import java.util.List;
import javax.annotation.Generated;
import javax.annotation.Nullable;
@Generated("com.google.auto.value.processor.AutoValueProcessor")
final class AutoValue_JdbcAvroArgs extends JdbcAvroArgs {
private final JdbcConnectionArgs jdbcConnectionConfiguration;
private final JdbcAvroArgs.StatementPreparator statementPreparator;
private final int fetchSize;
private final String avroCodec;
private final List preCommand;
private AutoValue_JdbcAvroArgs(
JdbcConnectionArgs jdbcConnectionConfiguration,
@Nullable JdbcAvroArgs.StatementPreparator statementPreparator,
int fetchSize,
String avroCodec,
List preCommand) {
this.jdbcConnectionConfiguration = jdbcConnectionConfiguration;
this.statementPreparator = statementPreparator;
this.fetchSize = fetchSize;
this.avroCodec = avroCodec;
this.preCommand = preCommand;
}
@Override
public JdbcConnectionArgs jdbcConnectionConfiguration() {
return jdbcConnectionConfiguration;
}
@Nullable
@Override
public JdbcAvroArgs.StatementPreparator statementPreparator() {
return statementPreparator;
}
@Override
public int fetchSize() {
return fetchSize;
}
@Override
public String avroCodec() {
return avroCodec;
}
@Override
public List preCommand() {
return preCommand;
}
@Override
public String toString() {
return "JdbcAvroArgs{"
+ "jdbcConnectionConfiguration=" + jdbcConnectionConfiguration + ", "
+ "statementPreparator=" + statementPreparator + ", "
+ "fetchSize=" + fetchSize + ", "
+ "avroCodec=" + avroCodec + ", "
+ "preCommand=" + preCommand
+ "}";
}
@Override
public boolean equals(Object o) {
if (o == this) {
return true;
}
if (o instanceof JdbcAvroArgs) {
JdbcAvroArgs that = (JdbcAvroArgs) o;
return this.jdbcConnectionConfiguration.equals(that.jdbcConnectionConfiguration())
&& (this.statementPreparator == null ? that.statementPreparator() == null : this.statementPreparator.equals(that.statementPreparator()))
&& this.fetchSize == that.fetchSize()
&& this.avroCodec.equals(that.avroCodec())
&& this.preCommand.equals(that.preCommand());
}
return false;
}
@Override
public int hashCode() {
int h$ = 1;
h$ *= 1000003;
h$ ^= jdbcConnectionConfiguration.hashCode();
h$ *= 1000003;
h$ ^= (statementPreparator == null) ? 0 : statementPreparator.hashCode();
h$ *= 1000003;
h$ ^= fetchSize;
h$ *= 1000003;
h$ ^= avroCodec.hashCode();
h$ *= 1000003;
h$ ^= preCommand.hashCode();
return h$;
}
private static final long serialVersionUID = 774966612L;
@Override
JdbcAvroArgs.Builder builder() {
return new Builder(this);
}
static final class Builder extends JdbcAvroArgs.Builder {
private JdbcConnectionArgs jdbcConnectionConfiguration;
private JdbcAvroArgs.StatementPreparator statementPreparator;
private Integer fetchSize;
private String avroCodec;
private List preCommand;
Builder() {
}
private Builder(JdbcAvroArgs source) {
this.jdbcConnectionConfiguration = source.jdbcConnectionConfiguration();
this.statementPreparator = source.statementPreparator();
this.fetchSize = source.fetchSize();
this.avroCodec = source.avroCodec();
this.preCommand = source.preCommand();
}
@Override
JdbcAvroArgs.Builder setJdbcConnectionConfiguration(JdbcConnectionArgs jdbcConnectionConfiguration) {
if (jdbcConnectionConfiguration == null) {
throw new NullPointerException("Null jdbcConnectionConfiguration");
}
this.jdbcConnectionConfiguration = jdbcConnectionConfiguration;
return this;
}
@Override
JdbcAvroArgs.Builder setStatementPreparator(JdbcAvroArgs.StatementPreparator statementPreparator) {
this.statementPreparator = statementPreparator;
return this;
}
@Override
JdbcAvroArgs.Builder setFetchSize(int fetchSize) {
this.fetchSize = fetchSize;
return this;
}
@Override
JdbcAvroArgs.Builder setAvroCodec(String avroCodec) {
if (avroCodec == null) {
throw new NullPointerException("Null avroCodec");
}
this.avroCodec = avroCodec;
return this;
}
@Override
JdbcAvroArgs.Builder setPreCommand(List preCommand) {
if (preCommand == null) {
throw new NullPointerException("Null preCommand");
}
this.preCommand = preCommand;
return this;
}
@Override
JdbcAvroArgs build() {
if (this.jdbcConnectionConfiguration == null
|| this.fetchSize == null
|| this.avroCodec == null
|| this.preCommand == null) {
StringBuilder missing = new StringBuilder();
if (this.jdbcConnectionConfiguration == null) {
missing.append(" jdbcConnectionConfiguration");
}
if (this.fetchSize == null) {
missing.append(" fetchSize");
}
if (this.avroCodec == null) {
missing.append(" avroCodec");
}
if (this.preCommand == null) {
missing.append(" preCommand");
}
throw new IllegalStateException("Missing required properties:" + missing);
}
return new AutoValue_JdbcAvroArgs(
this.jdbcConnectionConfiguration,
this.statementPreparator,
this.fetchSize,
this.avroCodec,
this.preCommand);
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy