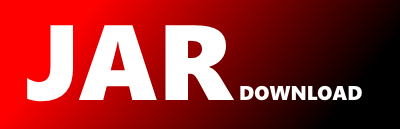
com.spotify.dbeam.options.AutoValue_KmsDecrypter Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of dbeam-core Show documentation
Show all versions of dbeam-core Show documentation
Top level DBeam core implementation
package com.spotify.dbeam.options;
import com.google.api.client.http.HttpTransport;
import com.google.auth.Credentials;
import java.util.Optional;
import javax.annotation.Generated;
@Generated("com.google.auto.value.processor.AutoValueProcessor")
final class AutoValue_KmsDecrypter extends KmsDecrypter {
private final String location;
private final String keyring;
private final String key;
private final Optional project;
private final Optional transport;
private final Optional credentials;
private AutoValue_KmsDecrypter(
String location,
String keyring,
String key,
Optional project,
Optional transport,
Optional credentials) {
this.location = location;
this.keyring = keyring;
this.key = key;
this.project = project;
this.transport = transport;
this.credentials = credentials;
}
@Override
String location() {
return location;
}
@Override
String keyring() {
return keyring;
}
@Override
String key() {
return key;
}
@Override
Optional project() {
return project;
}
@Override
Optional transport() {
return transport;
}
@Override
Optional credentials() {
return credentials;
}
@Override
public String toString() {
return "KmsDecrypter{"
+ "location=" + location + ", "
+ "keyring=" + keyring + ", "
+ "key=" + key + ", "
+ "project=" + project + ", "
+ "transport=" + transport + ", "
+ "credentials=" + credentials
+ "}";
}
@Override
public boolean equals(Object o) {
if (o == this) {
return true;
}
if (o instanceof KmsDecrypter) {
KmsDecrypter that = (KmsDecrypter) o;
return this.location.equals(that.location())
&& this.keyring.equals(that.keyring())
&& this.key.equals(that.key())
&& this.project.equals(that.project())
&& this.transport.equals(that.transport())
&& this.credentials.equals(that.credentials());
}
return false;
}
@Override
public int hashCode() {
int h$ = 1;
h$ *= 1000003;
h$ ^= location.hashCode();
h$ *= 1000003;
h$ ^= keyring.hashCode();
h$ *= 1000003;
h$ ^= key.hashCode();
h$ *= 1000003;
h$ ^= project.hashCode();
h$ *= 1000003;
h$ ^= transport.hashCode();
h$ *= 1000003;
h$ ^= credentials.hashCode();
return h$;
}
@Override
public KmsDecrypter.Builder builder() {
return new Builder(this);
}
static final class Builder extends KmsDecrypter.Builder {
private String location;
private String keyring;
private String key;
private Optional project = Optional.empty();
private Optional transport = Optional.empty();
private Optional credentials = Optional.empty();
Builder() {
}
private Builder(KmsDecrypter source) {
this.location = source.location();
this.keyring = source.keyring();
this.key = source.key();
this.project = source.project();
this.transport = source.transport();
this.credentials = source.credentials();
}
@Override
public KmsDecrypter.Builder location(String location) {
if (location == null) {
throw new NullPointerException("Null location");
}
this.location = location;
return this;
}
@Override
public KmsDecrypter.Builder keyring(String keyring) {
if (keyring == null) {
throw new NullPointerException("Null keyring");
}
this.keyring = keyring;
return this;
}
@Override
public KmsDecrypter.Builder key(String key) {
if (key == null) {
throw new NullPointerException("Null key");
}
this.key = key;
return this;
}
@Override
public KmsDecrypter.Builder project(Optional project) {
if (project == null) {
throw new NullPointerException("Null project");
}
this.project = project;
return this;
}
@Override
public KmsDecrypter.Builder transport(HttpTransport transport) {
this.transport = Optional.of(transport);
return this;
}
@Override
public KmsDecrypter.Builder credentials(Credentials credentials) {
this.credentials = Optional.of(credentials);
return this;
}
@Override
public KmsDecrypter build() {
if (this.location == null
|| this.keyring == null
|| this.key == null) {
StringBuilder missing = new StringBuilder();
if (this.location == null) {
missing.append(" location");
}
if (this.keyring == null) {
missing.append(" keyring");
}
if (this.key == null) {
missing.append(" key");
}
throw new IllegalStateException("Missing required properties:" + missing);
}
return new AutoValue_KmsDecrypter(
this.location,
this.keyring,
this.key,
this.project,
this.transport,
this.credentials);
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy