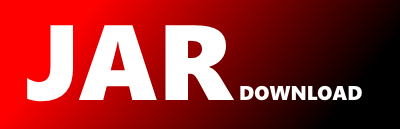
com.spotify.docker.client.messages.swarm.AutoValue_SwarmSpec Maven / Gradle / Ivy
package com.spotify.docker.client.messages.swarm;
import com.fasterxml.jackson.annotation.JsonProperty;
import com.google.common.collect.ImmutableMap;
import javax.annotation.Generated;
import javax.annotation.Nullable;
@Generated("com.google.auto.value.processor.AutoValueProcessor")
final class AutoValue_SwarmSpec extends SwarmSpec {
private final String name;
private final ImmutableMap labels;
private final OrchestrationConfig orchestration;
private final RaftConfig raft;
private final DispatcherConfig dispatcher;
private final CaConfig caConfig;
private final TaskDefaults taskDefaults;
AutoValue_SwarmSpec(
String name,
@Nullable ImmutableMap labels,
OrchestrationConfig orchestration,
RaftConfig raft,
DispatcherConfig dispatcher,
CaConfig caConfig,
TaskDefaults taskDefaults) {
if (name == null) {
throw new NullPointerException("Null name");
}
this.name = name;
this.labels = labels;
if (orchestration == null) {
throw new NullPointerException("Null orchestration");
}
this.orchestration = orchestration;
if (raft == null) {
throw new NullPointerException("Null raft");
}
this.raft = raft;
if (dispatcher == null) {
throw new NullPointerException("Null dispatcher");
}
this.dispatcher = dispatcher;
if (caConfig == null) {
throw new NullPointerException("Null caConfig");
}
this.caConfig = caConfig;
if (taskDefaults == null) {
throw new NullPointerException("Null taskDefaults");
}
this.taskDefaults = taskDefaults;
}
@JsonProperty(value = "Name")
@Override
public String name() {
return name;
}
@Nullable
@JsonProperty(value = "Labels")
@Override
public ImmutableMap labels() {
return labels;
}
@JsonProperty(value = "Orchestration")
@Override
public OrchestrationConfig orchestration() {
return orchestration;
}
@JsonProperty(value = "Raft")
@Override
public RaftConfig raft() {
return raft;
}
@JsonProperty(value = "Dispatcher")
@Override
public DispatcherConfig dispatcher() {
return dispatcher;
}
@JsonProperty(value = "CAConfig")
@Override
public CaConfig caConfig() {
return caConfig;
}
@JsonProperty(value = "TaskDefaults")
@Override
public TaskDefaults taskDefaults() {
return taskDefaults;
}
@Override
public String toString() {
return "SwarmSpec{"
+ "name=" + name + ", "
+ "labels=" + labels + ", "
+ "orchestration=" + orchestration + ", "
+ "raft=" + raft + ", "
+ "dispatcher=" + dispatcher + ", "
+ "caConfig=" + caConfig + ", "
+ "taskDefaults=" + taskDefaults
+ "}";
}
@Override
public boolean equals(Object o) {
if (o == this) {
return true;
}
if (o instanceof SwarmSpec) {
SwarmSpec that = (SwarmSpec) o;
return (this.name.equals(that.name()))
&& ((this.labels == null) ? (that.labels() == null) : this.labels.equals(that.labels()))
&& (this.orchestration.equals(that.orchestration()))
&& (this.raft.equals(that.raft()))
&& (this.dispatcher.equals(that.dispatcher()))
&& (this.caConfig.equals(that.caConfig()))
&& (this.taskDefaults.equals(that.taskDefaults()));
}
return false;
}
@Override
public int hashCode() {
int h = 1;
h *= 1000003;
h ^= this.name.hashCode();
h *= 1000003;
h ^= (labels == null) ? 0 : this.labels.hashCode();
h *= 1000003;
h ^= this.orchestration.hashCode();
h *= 1000003;
h ^= this.raft.hashCode();
h *= 1000003;
h ^= this.dispatcher.hashCode();
h *= 1000003;
h ^= this.caConfig.hashCode();
h *= 1000003;
h ^= this.taskDefaults.hashCode();
return h;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy