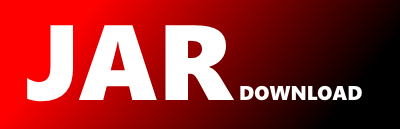
com.spotify.helios.common.descriptors.HostStatus Maven / Gradle / Ivy
/*
* Copyright (c) 2014 Spotify AB.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing,
* software distributed under the License is distributed on an
* "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY
* KIND, either express or implied. See the License for the
* specific language governing permissions and limitations
* under the License.
*/
package com.spotify.helios.common.descriptors;
import com.google.common.base.Joiner;
import com.fasterxml.jackson.annotation.JsonIgnoreProperties;
import com.fasterxml.jackson.annotation.JsonProperty;
import org.jetbrains.annotations.Nullable;
import java.util.Collections;
import java.util.Map;
import static com.google.common.base.Optional.fromNullable;
import static com.google.common.base.Preconditions.checkNotNull;
/**
* Gives the Helios host status for the agent, which includes all jobs, their statuses, as well
* as host and agent information.
*
* {
* "agentInfo" : { #... see the description of AgentInfo },
* "environment" : {
* "SYSLOG_HOST_PORT" : "10.99.0.1:514",
* },
* "hostInfo" : { #... see the description of HostInfo },
* "jobs" : {
* "myservice:0.5:3539b7bc2235d53f79e6e8511942bbeaa8816265" : {
* "goal" : "START",
* "jobId" : "myservice:0.5:3539b7bc2235d53f79e6e8511942bbeaa8816265",
* }
* },
* "labels" : {
* "role" : "foo",
* "xyz" : "123"
* },
* "status" : "UP",
* "statuses" : {
* "elva:0.0.4:9f64cf43353c55c36276b7df76b066584f9c49aa" : {
* "containerId" : "5a31d4fd48b5b4349980175e2f865494146704e684d89b6a95a9a766cc2f43a3",
* "env" : {
* "SYSLOG_HOST_PORT" : "10.99.0.1:514",
* },
* "goal" : "START",
* "job" : { #... See definition of Job },
* "state" : "RUNNING",
* "throttled" : "NO"
* }
* }
* }
*
*/
@JsonIgnoreProperties(ignoreUnknown = true)
public class HostStatus extends Descriptor {
public enum Status {
UP,
DOWN
}
private final Status status;
private final HostInfo hostInfo;
private final AgentInfo agentInfo;
private final Map jobs;
private final Map statuses;
private final Map environment;
private final Map labels;
/**
* Constructor.
* @param jobs Map of jobs and their deployments for this host.
* @param statuses the statuses of jobs on this host.
* @param status The up/down status of this host.
* @param hostInfo The host information.
* @param agentInfo The agent information.
* @param environment The environment provided to the agent on it's command line.
* @param labels The labels assigned to the agent.
*/
public HostStatus(@JsonProperty("jobs") final Map jobs,
@JsonProperty("statuses") final Map statuses,
@JsonProperty("status") final Status status,
@JsonProperty("hostInfo") final HostInfo hostInfo,
@JsonProperty("agentInfo") final AgentInfo agentInfo,
@JsonProperty("environment") final Map environment,
@JsonProperty("labels") final Map labels) {
this.status = checkNotNull(status, "status");
this.jobs = checkNotNull(jobs, "jobs");
this.statuses = checkNotNull(statuses, "statuses");
// Host, runtime info and environment might not be available
this.hostInfo = hostInfo;
this.agentInfo = agentInfo;
this.environment = fromNullable(environment).or(Collections.emptyMap());
this.labels = fromNullable(labels).or(Collections.emptyMap());
}
public Map getEnvironment() {
return environment;
}
public Map getLabels() {
return labels;
}
public Status getStatus() {
return status;
}
@Nullable
public HostInfo getHostInfo() {
return hostInfo;
}
@Nullable
public AgentInfo getAgentInfo() {
return agentInfo;
}
public Map getJobs() {
return jobs;
}
public Map getStatuses() {
return statuses;
}
public static Builder newBuilder() {
return new Builder();
}
public static class Builder {
private Map jobs;
private Map statuses;
private Status status;
private HostInfo hostInfo;
private AgentInfo agentInfo;
private Map environment;
private Map labels;
public Builder setJobs(final Map jobs) {
this.jobs = jobs;
return this;
}
public Builder setStatuses(final Map statuses) {
this.statuses = statuses;
return this;
}
public Builder setStatus(final Status status) {
this.status = status;
return this;
}
public Builder setHostInfo(final HostInfo hostInfo) {
this.hostInfo = hostInfo;
return this;
}
public Builder setAgentInfo(final AgentInfo agentInfo) {
this.agentInfo = agentInfo;
return this;
}
public Builder setEnvironment(final Map environment) {
this.environment = environment;
return this;
}
public Builder setLabels(final Map labels) {
this.labels = labels;
return this;
}
public HostStatus build() {
return new HostStatus(jobs, statuses, status, hostInfo, agentInfo, environment, labels);
}
}
@Override
public boolean equals(final Object o) {
if (this == o) {
return true;
}
if (o == null || getClass() != o.getClass()) {
return false;
}
final HostStatus that = (HostStatus) o;
if (hostInfo != null ? !hostInfo.equals(that.hostInfo) : that.hostInfo != null) {
return false;
}
if (jobs != null ? !jobs.equals(that.jobs) : that.jobs != null) {
return false;
}
if (agentInfo != null ? !agentInfo.equals(that.agentInfo) : that.agentInfo != null) {
return false;
}
if (status != that.status) {
return false;
}
if (statuses != null ? !statuses.equals(that.statuses) : that.statuses != null) {
return false;
}
if (environment != null ? !environment.equals(that.environment) : that.environment != null) {
return false;
}
if (labels != null ? !labels.equals(that.labels) : that.labels != null) {
return false;
}
return true;
}
@Override
public int hashCode() {
int result = status != null ? status.hashCode() : 0;
result = 31 * result + (hostInfo != null ? hostInfo.hashCode() : 0);
result = 31 * result + (agentInfo != null ? agentInfo.hashCode() : 0);
result = 31 * result + (jobs != null ? jobs.hashCode() : 0);
result = 31 * result + (statuses != null ? statuses.hashCode() : 0);
result = 31 * result + (environment != null ? environment.hashCode() : 0);
result = 31 * result + (labels != null ? labels.hashCode() : 0);
return result;
}
@Override
public String toString() {
return "HostStatus{" +
"status=" + status +
", hostInfo=" + hostInfo +
", agentInfo=" + agentInfo +
", jobs=" + jobs +
", statuses=" + statuses +
", environment=" + stringMapToString(environment) +
", labels=" + stringMapToString(labels) +
'}';
}
private static String stringMapToString(final Map map) {
return "{" + Joiner.on(", ").withKeyValueSeparator("=").join(map) + "}";
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy